Understanding Deferred Execution in Java: Common Pitfalls
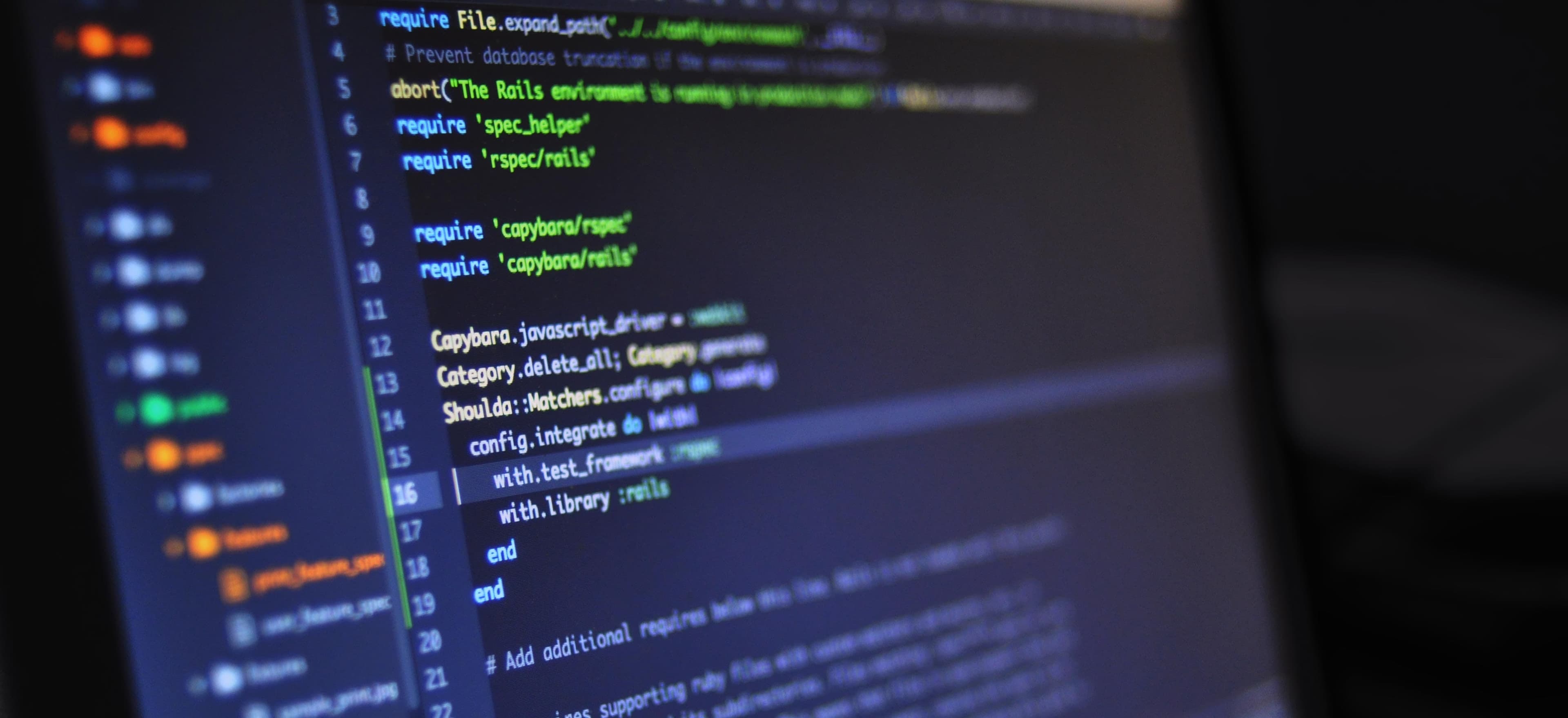
- Published on
Understanding Deferred Execution in Java: Common Pitfalls
Deferred execution, often referred to in the context of programming with Java, can significantly impact the way developers manage performance and resource allocation within their applications. Understanding how deferred execution works can help developers build more efficient code, but it can also lead to common pitfalls if not handled with care.
In this post, we will explain the concept of deferred execution, its implications in Java, and outline several common mistakes developers make. We will include code snippets, linking to relevant concepts for further study to ensure a comprehensive understanding of deferred execution in Java.
What is Deferred Execution?
Deferred execution is a programming paradigm where the execution of code is postponed until its results are actually needed. In Java, this is primarily seen in stream processing and lazy evaluation. The principle behind this approach is to optimize performance and resource utilization by delaying computation until you have sufficient data to execute.
Example of Deferred Execution with Streams
Java 8 introduced the Stream
API, which utilizes deferred execution. Here is a simple example highlighting this feature:
import java.util.List;
import java.util.Arrays;
public class DeferredExecutionExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Edward");
long count = names.stream()
.filter(name -> name.startsWith("A"))
.count(); // Execution happens here
System.out.println("Names starting with 'A': " + count);
}
}
Commentary
- Stream Creation: The
stream()
method creates a stream of names. - Filtering: The
filter
method is invoked, defining conditions to filter the stream items. At this stage, nothing happens as the stream is not yet executed. - Execution: The
count()
method triggers the processing. Now the stream operations will run, counting how many names start with 'A'.
The key takeaway here is that the filtering operation does not execute until the terminal operation (count()
) is called. This means we can set up complex chain-call operations without immediate performance costs.
Benefits of Deferred Execution
- Improved Performance: By executing only what is necessary, deferred execution can reduce processing time and memory usage.
- Easier to Understand: The code tends to be more readable, as it describes the intent rather than the mechanics of execution.
- Flexibility: It allows for more complex data processing setups since execution is not moored to the point of definition.
Common Pitfalls of Deferred Execution
While deferred execution offers remarkable advantages, it is essential to approach it carefully. Here are several common pitfalls that developers often encounter.
1. State Changes Between Setup and Execution
One of the main pitfalls arises when a stream's underlying data structure is modified after defining the stream but before executing it. This can lead to unexpected results.
import java.util.ArrayList;
import java.util.List;
public class PitfallExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>(List.of("Alice", "Bob", "Charlie"));
var stream = names.stream().filter(name -> name.startsWith("A"));
// Modifying the list after creating the stream
names.add("Andrew"); // The list now has an additional name
long count = stream.count(); // Execution happens here
System.out.println("Names starting with 'A': " + count);
}
}
Result Analysis
In this example, the expected output is 2 (for Alice and Andrew), but if you forget the modification, the result will be misleading since the stream was created before the change. It's crucial to avoid altering the underlying collection after stream creation.
2. Performance Misconceptions
Deferred execution can lead to misconceptions regarding performance. Developers might assume that because an operation isn’t executed immediately, it doesn’t incur any cost. In reality, the operation is deferred until it is triggered by a terminal operation, which can lead to a sudden spike in resource usage.
Example performance issue:
import java.util.stream.IntStream;
public class PerformanceMisconception {
public static void main(String[] args) {
var stream = IntStream.range(1, 1_000_000)
.filter(n -> n % 2 == 0)
.map(n -> n * 2);
// The filter and map operations haven’t been executed yet.
System.out.println("About to trigger execution...");
long count = stream.count(); // Execution happens here
System.out.println("Count of even numbers: " + count);
}
}
My Closing Thoughts on the Matter About Performance
A sudden demand for resources during execution can throttle system performance unexpectedly. Always be mindful of the context in which you are working, especially regarding memory consumption and CPU cycles due to deferred execution setups.
3. Ignoring Short-Circuiting
Java Stream API provides short-circuiting operations like findFirst()
and anyMatch()
. These methods can optimize operations because they can return results without traversing the entire stream. However, if overlooked, you may end up with less performant code.
import java.util.stream.IntStream;
public class ShortCircuitExample {
public static void main(String[] args) {
boolean anyEven = IntStream.range(1, 1_000_000)
.filter(n -> n % 2 == 0)
.findFirst() // Short-circuits here
.isPresent();
System.out.println("Is there any even number? " + anyEven);
}
}
4. Not Reusing Streams
Streams in Java are designed to be consumed once, which can lead to errors if you try to reuse a stream after it has already been processed. Attempting to reuse a stream can lead to IllegalStateException
.
import java.util.List;
import java.util.Arrays;
public class ReuseStreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
var stream = names.stream().filter(name -> name.startsWith("A"));
stream.count(); // First use, valid execution
// Attempting to reuse the same stream
long countAgain = stream.count(); // This will throw an exception
}
}
Practical Tip for Reusing Streams
Always create a new stream for independent operations:
long countA = names.stream().filter(name -> name.startsWith("A")).count();
long countB = names.stream().filter(name -> name.startsWith("B")).count();
My Closing Thoughts on the Matter
Understanding deferred execution is vital for Java developers aiming to optimize their code's performance and maintain clarity. While it offers numerous benefits, neglecting to recognize common pitfalls can lead to inefficiencies and bugs.
Keep these common issues in mind:
- Be cautious of state changes after creating streams.
- Have a clear strategy regarding performance expectations.
- Utilize short-circuiting methods to improve efficiency.
- Remember that streams cannot be reused after consumption.
For further information, you may want to check out the following resources:
- Java Platform SE 8 - Stream Documentation
- The Basics of Lambda Expressions
By following these guidelines, you can harness the power of deferred execution effectively in your Java projects. Happy coding!
Checkout our other articles