Troubleshooting Robolectric and Robotium Integration Issues
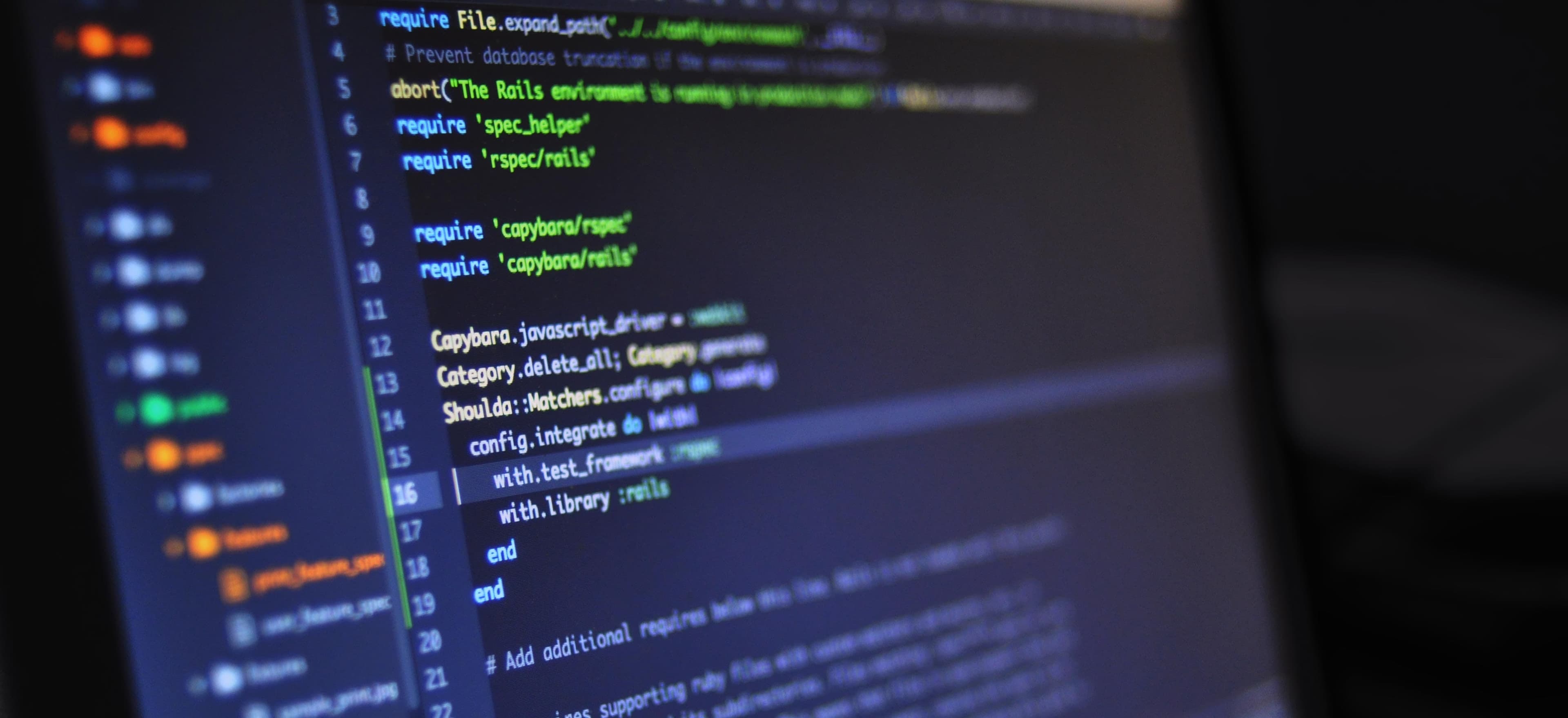
- Published on
Troubleshooting Robolectric and Robotium Integration Issues
As mobile application development continues to evolve, so do the methods employed for effective testing. This is where Robolectric and Robotium come into play. Both tools serve significant roles in Android testing, yet integrating them can lead to myriad challenges. In this blog post, we will explore these tools, their integration issues, and effective troubleshooting steps.
What are Robolectric and Robotium?
Robolectric
Robolectric is a powerful framework for Android unit testing that enables developers to run tests directly on the JVM without needing an emulator, thus speeding up the testing process. It simulates Android components and allows for faster feedback cycles during development.
Robotium
Robotium, on the other hand, is a testing framework specifically designed for Android UI testing. It allows you to write both automatic functional and acceptance tests for Android apps. Unlike Robolectric, which focuses on unit tests, Robotium is utilized for UI tests that check how your components interact in real devices.
Why Use Both Frameworks?
Integrating Robolectric with Robotium can provide a well-rounded testing suite. While Robolectric covers unit tests, Robotium ensures that the user interface aspects are functioning as expected. Combining these tools can lead to better coverage and more reliable applications.
However, integrating these frameworks can lead to several issues. In this article, we’ll identify common problems and provide troubleshooting guidance.
Common Issues When Integrating Robolectric and Robotium
-
Classpath Issues
- Sometimes, mismatches in classpath can cause tests to fail. These might relate to dependency versions in your build configuration.
-
Activity Lifecycle Management
- Robolectric runs tests in a simulated environment, which can lead to discrepancies in the Activity lifecycle when mixed with Robotium's instrumentation testing.
-
Resource Access Failures
- There can be inconsistencies in accessing resources when transitioning between Robolectric and Robotium tests.
-
Threading Issues
- Running tests engaging UI threads can also lead to flakiness in tests as Robolectric runs on the JVM and Robotium on a device/emulator.
-
Configuration Problems
- Incorrectly configured build tools or test configurations can amplify the integration issues.
Troubleshooting Steps
Step 1: Resolving Classpath Issues
Check your build configuration to ensure there are no conflicts. Here is an example of how you might set up your build.gradle
:
dependencies {
testImplementation 'org.robolectric:robolectric:4.7.3'
androidTestImplementation 'com.robotium.solo:robotium-solo:5.6.3'
}
Ensure that all libraries are compatible in terms of versions. The same applies to your Gradle plugin and Android SDK versions. Using the latest versions might resolve several issues immediately.
Step 2: Manage Activity Lifecycle
Ensure that the Activities you are testing are properly set up before the tests begin. In Robolectric you can use @Config
to set configurations on your Activity tests. For example:
@RunWith(RobolectricTestRunner.class)
@Config(sdk = Build.VERSION_CODES.P)
public class MainActivityTest {
@Test
public void testActivityLaunches() {
MainActivity activity = Robolectric.buildActivity(MainActivity.class).create().start().resume().get();
assertNotNull(activity);
}
}
Using the above method, manage the lifecycle events correctly before transitioning to Robotium tests.
Step 3: Handling Resource Access
Ensure any resources you are accessing in your tests are correct. If resources are defined in different directories or are missing, your tests might crash.
In Robolectric, use the @Config
annotation to specify the resource directory if necessary:
@Config(manifest = Config.NONE) // Use when no manifest is required
Step 4: Keeping Threads in Check
Robolectric runs all tests on a single thread, however, Robotium operates with multiple threads. Be aware of this when performing operations that depend on UI updates. Consider using Robolectric.runOnUiThread()
when linking the two to avoid threading-related issues.
Example Code for UI Testing with Robotium
public class RobotiumTest extends ActivityInstrumentationTestCase2<MainActivity> {
private Solo solo;
public RobotiumTest() {
super(MainActivity.class);
}
@Override
protected void setUp() throws Exception {
solo = new Solo(getInstrumentation(), getActivity());
}
public void testButtonClick() {
solo.clickOnButton("Click Me");
assertTrue(solo.searchText("Button Clicked"));
}
@Override
protected void tearDown() throws Exception {
solo.finishOpenedActivities();
}
}
In the code example above, the solo
object is an instance of the Robotium class that allows you to interact with UI elements easily. After clicking the button, we assert that the text "Button Clicked" appears, ensuring that our UI behaves as expected.
Step 5: Double-check Configuration Settings
Be diligent about your configuration settings in AndroidManifest.xml
. This can dramatically affect how Robolectric and Robotium execute tests together.
Final Thoughts
Integrating Robolectric and Robotium might seem daunting, but by understanding and addressing these common issues, you can streamline your testing process significantly. Testing forms the backbone of a reliable application, and with robust integration, you can ensure that both your backend logic and UI operations are performing effectively.
For further reading on the best practices with Robolectric and Robotium, be sure to check their official documentation.
By leveraging the strengths of both frameworks, you can foster a comprehensive testing approach for Android applications, ensuring a smoother and more confident release process.
Happy testing!