Managing Circular Dependencies in Spring Inheritance Trees
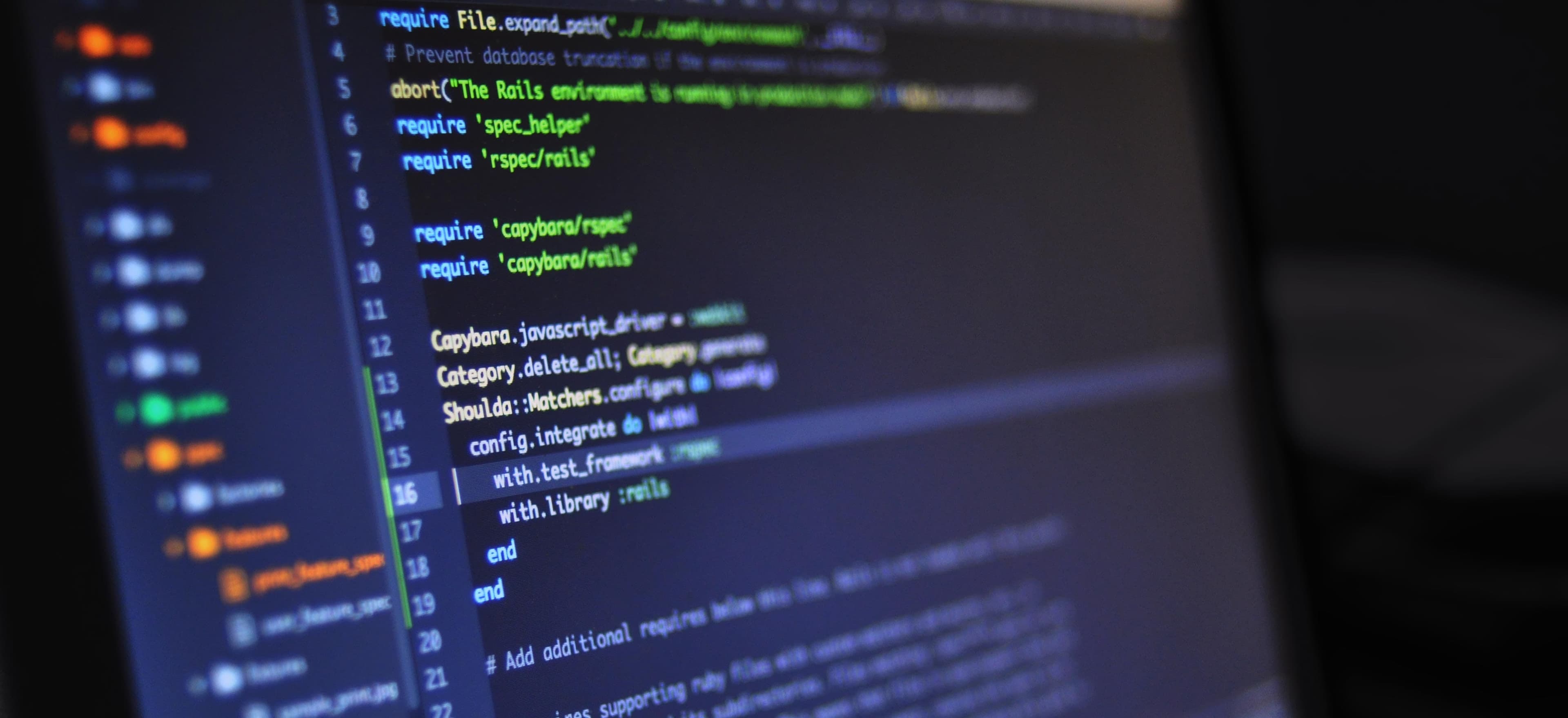
- Published on
Managing Circular Dependencies in Spring Inheritance Trees
Circular dependencies can sometimes seem like a daunting hurdle in the world of Spring Framework, particularly when dealing with inheritance trees. Just as an architect ensures that a building’s design avoids any structural flaws, developers must be vigilant to prevent circular dependencies in application architecture. This blog post will delve deep into how to effectively manage circular dependencies in Spring inheritance trees, ensuring your applications remain maintainable and efficient.
Understanding Circular Dependencies
In basic terms, a circular dependency occurs when two or more beans depend on each other. For instance, if ClassA
depends on ClassB
, and simultaneously, ClassB
depends on ClassA
, we have a classic case of a circular dependency.
The Need for Caution
While Spring manages many forms of dependency resolution, circular dependencies can lead to BeanCurrentlyInCreationException
, which hampers application functionality and crashes startup processes. Therefore, it's crucial to avoid these pitfalls from the outset.
Spring Inheritance and Dependency Injection
Spring supports inheritance, allowing one bean to inherit properties and dependencies from a parent bean. However, if a child bean of one class depends on a sibling bean from another class that eventually points back to the parent, you have a potential circular dependency.
Example Scenario
Suppose we have classes Parent
, ChildA
, and ChildB
:
public class Parent {
private ChildA childA;
public Parent(ChildA childA) {
this.childA = childA;
}
}
public class ChildA extends Parent {
private ChildB childB;
public ChildA(ChildB childB) {
super(childB);
this.childB = childB;
}
}
public class ChildB extends Parent {
private ChildA childA;
public ChildB(ChildA childA) {
super(childA);
this.childA = childA;
}
}
In the code above, ChildA
and ChildB
both have circular dependencies on each other, leading to potential startup failures.
Resolving Circular Dependencies
1. Use of Setter Injection
Setter injection can resolve circular dependencies since it allows Spring to first create the bean without fully initializing it, thus breaking the circular dependency chain.
public class Parent {
private ChildA childA;
public void setChildA(ChildA childA) {
this.childA = childA;
}
}
public class ChildA extends Parent {
private ChildB childB;
public ChildA() {}
public void setChildB(ChildB childB) {
this.childB = childB;
}
}
public class ChildB extends Parent {
private ChildA childA;
public ChildB() {}
public void setChildA(ChildA childA) {
this.childA = childA;
}
}
In this modification, Spring will create beans ChildA
and ChildB
first and inject their dependencies afterward. This avoids immediate circular reference during creation.
2. Using @Lazy
Annotations
Another powerful solution for breaking circular dependencies comes from the @Lazy
annotation. This annotation allows Spring to lazily resolve the dependency, which means it will only initialize the bean when it is required.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Lazy;
public class Parent {
private ChildA childA;
@Autowired
public Parent(@Lazy ChildA childA) {
this.childA = childA;
}
}
public class ChildA extends Parent {
private ChildB childB;
@Autowired
public ChildA(@Lazy ChildB childB) {
super(childB);
this.childB = childB;
}
}
public class ChildB extends Parent {
private ChildA childA;
@Autowired
public ChildB(@Lazy ChildA childA) {
super(childA);
this.childA = childA;
}
}
In this case, by using @Lazy
, you inform Spring that it can instantiate the class once it is needed, effectively managing the cycle.
3. Refactor the Design
Restructuring your classes is often necessary. Consider breaking down tightly coupled classes into separate, well-defined components or use interfaces for your applications.
This can lead to better separation of concerns, making your application easier to maintain and test.
public interface ParentService {
void manage();
}
public class ChildAService implements ParentService {
@Override
public void manage() {
// Logic for managing ChildA
}
}
public class ChildBService implements ParentService {
@Override
public void manage() {
// Logic for managing ChildB
}
}
By defining an interface, you encapsulate behavior that doesn’t depend directly on specific classes, helping to erase circular dependencies.
4. Application Context Configuration
By using one of Spring’s configuration methods like XML configuration or Java-based configuration, you can define the beans and their dependencies more explicitly. This controlled setup can prevent accidental circular dependencies that may arise in auto-wiring scenarios.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public Parent parent(ChildA childA) {
return new Parent(childA);
}
@Bean
public ChildA childA(ChildB childB) {
return new ChildA(childB);
}
@Bean
public ChildB childB(ChildA childA) {
return new ChildB(childA);
}
}
Explicitly defining your beans outlines the relationships—similar to an architect creating a blueprint of a building, ensuring there are no surprises during construction.
Final Thoughts
Managing circular dependencies in Spring inheritance trees is not just about applying workarounds; it is a part of developing clean and maintainable software design. By leveraging setter injection, lazy initialization, proper refactoring, and organized application context configuration, you not only resolve immediate issues but also enhance the robustness of your application’s architecture.
When faced with circular dependencies, remember that the goal is clarity and maintainability. As you improve your design, the effectiveness of your software will reflect in its performance and ease of use.
For further reading on dependency management in Spring, consider checking out the Spring Framework documentation.
Taking the time to address these architectural concerns now saves headaches down the line. Happy coding!
Checkout our other articles