Boost CPU Performance by Mastering Garbage Collection Techniques
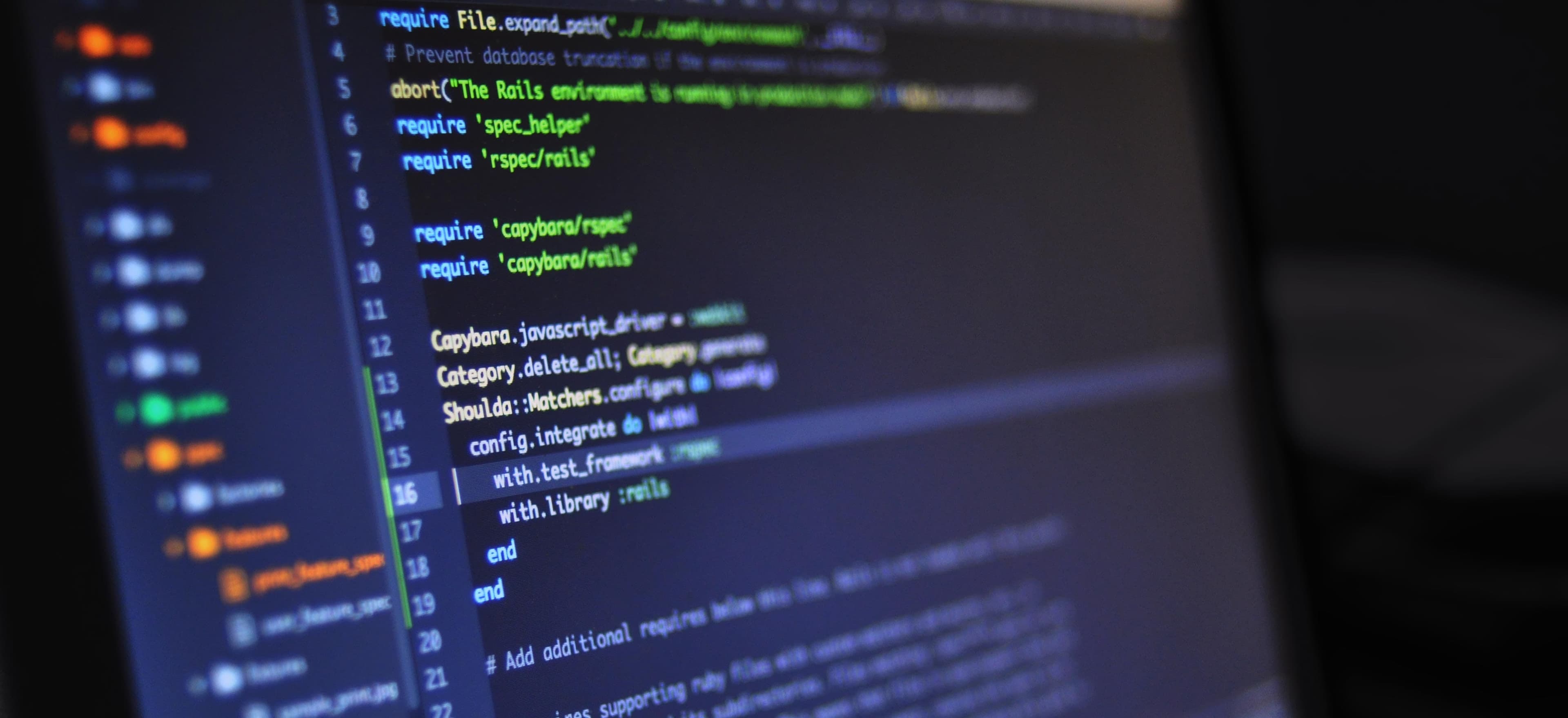
- Published on
Boost CPU Performance by Mastering Garbage Collection Techniques
Garbage Collection (GC) is an essential part of Java's memory management. It's designed to help programmers manage memory more efficiently, but understanding how it works can unlock substantial performance improvements, particularly for CPU-bound applications. In this blog post, we'll dive deep into various Garbage Collection techniques, their implications on CPU performance, and practical tips for optimizing Java applications.
What is Garbage Collection?
In the context of Java, Garbage Collection refers to the automated process that identifies and discards memory that is no longer in use, freeing it up for future allocations. This can prevent memory leaks and excessive memory consumption, which can severely impact application performance.
Why GC Matters for CPU Performance
While Java developers often focus on CPU usage, it's crucial to understand that inefficient use of memory can lead to increased CPU overhead. This occurs because when memory is not efficiently managed, it can lead to higher CPU cycles spent on GC processes, ultimately degrading performance.
Key Garbage Collection Techniques
Java provides several garbage collection algorithms. Each has its strengths and weaknesses. Let's discuss them in detail.
1. Serial Garbage Collector
The Serial Garbage Collector is the simplest GC implementation, working on a single thread. It's suitable for small applications or single-threaded environments.
-XX:+UseSerialGC
Why this matters:
- It stops application threads while executing garbage collection.
- Therefore, it can lead to longer pauses in applications with heavy loads.
2. Parallel Garbage Collector
The Parallel GC uses multiple threads to perform GC operations, significantly reducing the time it takes for the application to recover memory.
-XX:+UseParallelGC
Why this matters:
- Parallel GC can outperform the Serial Collector in multi-threaded applications, as it minimizes the time spent on memory cleanup.
- It is particularly effective in CPU-intensive applications where vacuuming garbage quickly is paramount.
3. Concurrent Mark-Sweep (CMS)
CMS aims for shorter GC pauses by performing most of its work concurrently with the application threads.
-XX:+UseConcMarkSweepGC
Why this matters:
- By running concurrently, CMS can greatly reduce pause times, thus improving performance for applications that require low-latency responses.
4. G1 Garbage Collector
The G1 GC is designed for applications with large heaps (greater than a gigabyte) where lower pause times are critical. It divides the heap into regions and prioritizes the collection of areas that hold the most garbage.
-XX:+UseG1GC
Why this matters:
- G1 GC can provide predictable pause times and is designed to scale well as more memory is added.
Choosing the Right GC for Your Application
Selecting the right garbage collector can have dramatic effects on your application's CPU performance.
Testing and Observation
Before making definitive choices, always test with different GC options. Use tools like Java VisualVM or JConsole to monitor GC activity.
Example command:
java -XX:+UseG1GC -verbose:gc -Xloggc:gc.log -jar YourApplication.jar
This will help you monitor how frequently garbage collection occurs and how much time it takes.
Profiling Memory Usage
Understanding how your application manages memory is essential. Make use of profiling libraries to pinpoint areas where memory usage can be reduced.
- Java Flight Recorder: Provides insights into application performance, including memory usage.
- JProfiler or YourKit: Third-party profiler tools that offer in-depth GC monitoring.
Managing Heap Sizes
Configuring heap sizes can also greatly affect CPU performance.
Initial and Maximum Heap Size
Finding the right balance in setting the initial heap size and the maximum heap size can help control how often GC is triggered. The flags -Xms
(initial) and -Xmx
(maximum) can be adjusted accordingly.
Example:
java -Xms512m -Xmx2048m -jar YourApplication.jar
This initial allocation provides a good starting point without wasting memory.
Best Practices for Optimizing Garbage Collection
Below are some best practices to optimize GC for better CPU performance:
1. Minimize Object Creation
Frequent object creation can lead to increased garbage collection. To reduce this:
- Reuse objects where possible.
- Use object pools for frequently used objects.
2. Prefer Primitive Types
Using primitive types instead of boxed types can decrease memory consumption, allowing for fewer allocations and less garbage to collect.
Example:
Instead of using Integer
, use int
when possible.
3. Use Collections Wisely
Be cautious when selecting collections. For example:
- Prefer
ArrayList
for random access. - Use
LinkedList
for applications focused on frequent insertions and deletions.
4. Tune GC Parameters
Garbage collectors have several parameters that can be fine-tuned. Some commonly adjusted parameters are:
-XX:GCTimeRatio=<value>
-XX:MaxGCPauseMillis=<value>
Make sure to document changes and iterate based on application performance.
Real-World Linkages
For more insights on how specific garbage collection methods can impact different types of applications, refer to the following resources:
- Oracle's Garbage Collection Tuning Guide
- Baeldung's Guide to Java Garbage Collection
The Closing Argument
Understanding and mastering Garbage Collection techniques can have a tremendous impact on the CPU performance of your Java applications. By recognizing the characteristics of the various GC algorithms, monitoring memory usage, and following best coding practices, you can develop applications that are both efficient and responsive.
Efficient memory management leads to reduced CPU overhead, better application performance, and enhanced user experience. Start implementing these strategies, and witness the difference in your application's performance.
Final Thoughts
Garbage Collection is a complex topic, but with proper understanding and application of techniques, Java developers can optimize their applications significantly, leading to increased efficiency and reduced latency. Happy coding!
Checkout our other articles