Boosting Microservice Performance with Chronicle Map in Java
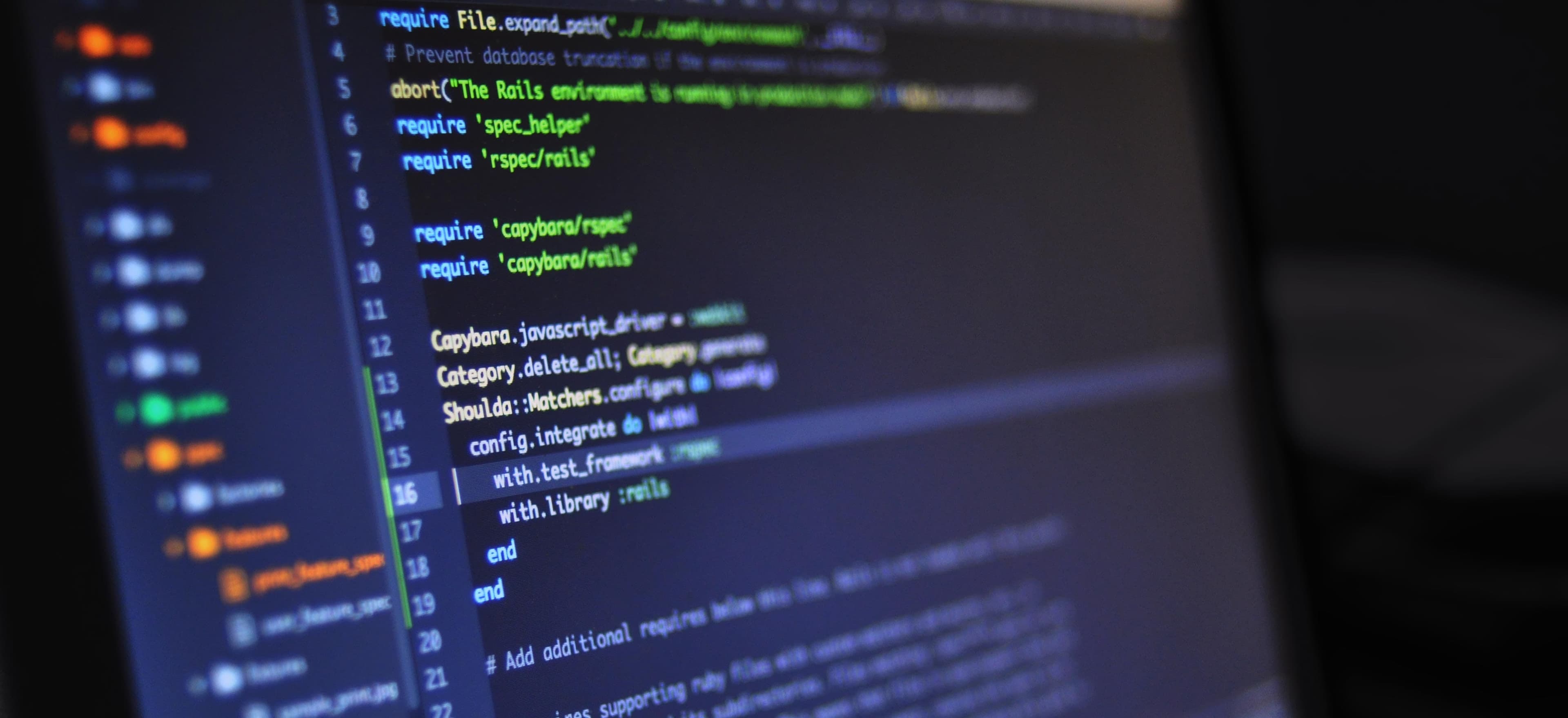
- Published on
Boosting Microservice Performance with Chronicle Map in Java
In the evolving landscape of microservices architecture, performance optimization is at the forefront of every developer's mind. Microservices, which break down applications into smaller, independent services, can end up feeling sluggish due to various challenges like data access latency or inefficient data structure management. One efficient way to address these challenges is by leveraging Chronicle Map, a high-performance Java library designed for managing key-value pairs directly in memory.
In this blog post, we will delve into what Chronicle Map is, its advantages, how to implement it in a microservices architecture, and explore real-world examples with code snippets.
What is Chronicle Map?
Chronicle Map is designed to provide high-throughput, low-latency data access in Java applications. Unlike traditional maps, which store data on the heap, Chronicle Map offers features like:
- Support for larger-than-memory storage: It can store massive datasets without running into memory constraints.
- Asynchronous operations: Designed for high performance, it includes capabilities for non-blocking data access.
- Durability: It can save its state to disk, providing a layer of persistence that standard in-memory structures do not.
All these features make Chronicle Map a compelling choice for microservices that require fast data retrieval and storage without compromising on scalability.
Why Use Chronicle Map in Microservices?
-
Performance Optimization: Microservices often communicate with databases over a network, which can add latency. Chronicle Map minimizes this communication overhead by allowing services to cache frequently accessed data.
-
Reduced Memory Footprint: Since Chronicle Map allows larger-than-memory storage, it efficiently handles large datasets by mapping file storage directly into Java memory. This can significantly reduce garbage collection pauses in your applications.
-
Simplicity in Management: Setting up and managing a Chronicle Map instance is straightforward. This simplicity helps lower development and operational overhead in microservice architectures.
Getting Started with Chronicle Map
To get started with Chronicle Map, you'll first need to add it to your Maven project. Here’s how you can do that:
<dependency>
<groupId>net.openhft</groupId>
<artifactId>chronicle-map</artifactId>
<version>3.18.19</version>
</dependency>
Implementing Chronicle Map
Let's consider a simple example of using Chronicle Map within a microservice that manages user sessions. Here’s how you'd set it up:
- Create a Chronicle Map Instance:
import net.openhft.chronicle.map.ChronicleMap;
ChronicleMap<String, UserSession> userSessionMap = ChronicleMap.of(String.class, UserSession.class)
.name("userSessions")
.entries(100_000) // Maximum entries
.averageKeySize(40) // Average key size for optimization
.create(); // Create instance
Why this matters: By setting up the key and value types, along with the estimated number of entries, Chronicle Map optimizes its internal structures for better performance from the get-go.
- Storing Data in Chronicle Map:
UserSession session = new UserSession("user123", Instant.now());
userSessionMap.put(session.getUserId(), session);
Explanation: Here, we create a new UserSession
object and store it using the user ID as the key. This allows for quick access to user session data.
- Retrieving Data:
UserSession retrievedSession = userSessionMap.get("user123");
if (retrievedSession != null) {
System.out.println("Session retrieved: " + retrievedSession);
} else {
System.out.println("Session not found.");
}
Why this is effective: Accessing user sessions becomes highly efficient by directly looking them up in memory, reducing latency compared to traditional database queries.
- Handling Expiry or Removal:
To remove sessions that are expired, you can set up a scheduled task:
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
scheduler.scheduleAtFixedRate(() -> {
userSessionMap.forEach((key, value) -> {
if (value.isExpired()) {
userSessionMap.remove(key);
}
});
}, 0, 1, TimeUnit.MINUTES);
Explanation: This ensures that expired sessions are regularly cleaned up, thus preventing the map from growing indefinitely. An efficient cache management strategy is crucial in any microservice.
- Shutting Down the Instance:
It's always good practice to close the map when it's no longer needed:
userSessionMap.close();
Performance Testing and Benchmarking
Integrating performance testing into your workflow is a vital aspect of using Chronicle Map. Tools like JMH (Java Microbenchmark Harness) can help assess how Chronicle Map stacks up against other data stores in your microservice.
Example of a Simple Benchmark
import org.openjdk.jmh.annotations.*;
@State(Scope.Thread)
public class ChronicleMapBenchmark {
private ChronicleMap<String, UserSession> map;
@Setup
public void setup() {
map = ChronicleMap.of(String.class, UserSession.class).name("benchmarkMap").entries(100_000).create();
// Populate the map with test data
}
@Benchmark
public void testPut() {
map.put("user1", new UserSession("user1", Instant.now()));
}
@Benchmark
public void testGet() {
map.get("user1");
}
@TearDown
public void tearDown() {
map.close();
}
}
Explanation: By benchmarking both put
and get
operations, you can analyze performance under different load conditions and optimize accordingly.
The Bottom Line
In a tightly connected ecosystem of microservices, optimizing data access through in-memory structures like Chronicle Map can yield significant performance benefits. The customizability, durability, and simplicity of implementing Chronicle Map make it a formidable tool for any developer looking to enhance the capabilities of their microservices.
If you want to learn more about key-value stores, check out the Chronicle Map documentation for advanced use cases and additional features.
By integrating Chronicle Map into your microservice architecture, you can minimize latency and maximize throughput, ultimately leading to a better user experience and a more efficient application overall.
Checkout our other articles