Common Spring Batch Pitfalls and How to Avoid Them
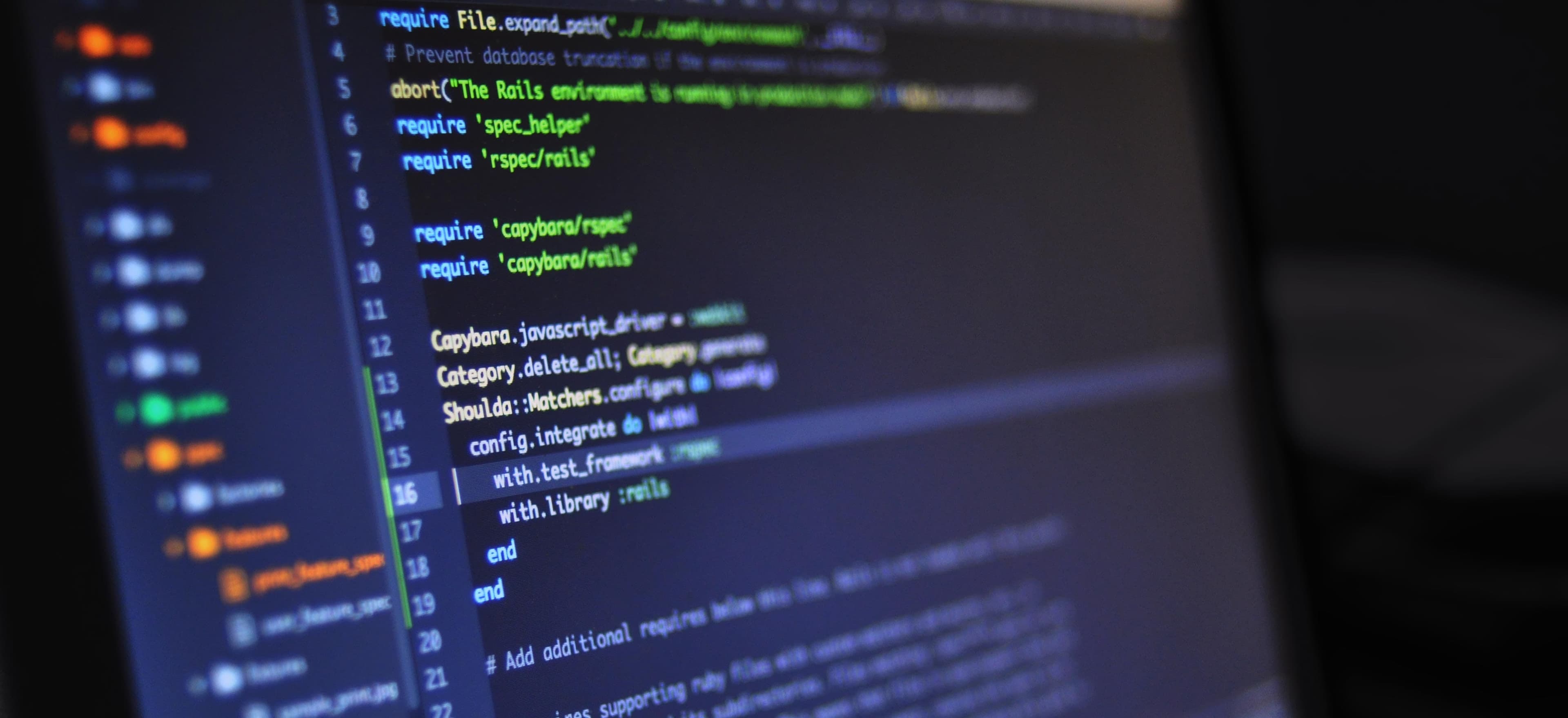
- Published on
Common Spring Batch Pitfalls and How to Avoid Them
Spring Batch is a powerful framework for processing large volumes of data. With its comprehensive set of tools and features, developers can create robust batch applications with ease. However, even experienced developers can run into pitfalls during the implementation phase. In this article, we will explore some common Spring Batch pitfalls and provide you with strategies to avoid them.
1. Not Understanding Batch Processing Concepts
Batch processing is inherently different from real-time processing. Understanding these differences is crucial for implementing a successful batch job. A common mistake developers make is treating batch jobs like standard web applications.
Why It's Important
Knowing the lifecycle of a batch job—including the steps, flows, and listeners—will help you design better applications and troubleshoot issues effectively. Segregating the batch architecture can lead to issues such as long-running transactions or performance bottlenecks.
Best Practices
- Learn the Basics: Familiarize yourself with key concepts such as ItemReader, ItemProcessor, and ItemWriter.
- Use Spring Batch Documentation: The Spring Batch documentation is well-structured and comprehensive.
2. Ignoring Transaction Management
Transaction management in Spring Batch is vital but often overlooked. Failing to configure transactions can lead to inconsistent data states, especially when processing large volumes of records.
What Can Go Wrong
Batch processes can fail due to exceptions in one of the step processes (Reader, Processor, or Writer). If transactions are not configured properly, you may end up with partially processed records, making the state unclear.
Solutions
- Use Step-scoped Transactions: Spring Batch allows each step to have its own transaction scope. This allows you to roll back only the transactions that are part of the failed step.
@Bean
public Step myStep() {
return stepBuilderFactory.get("myStep")
.<ItemType, AnotherItemType>chunk(10)
.reader(itemReader())
.processor(itemProcessor())
.writer(itemWriter())
.transactionManager(transactionManager())
.build();
}
Why This Works
Setting transactions at the step level ensures that for each chunk processed (10 in this case), if an error occurs, all operations in that chunk will be rolled back while others can still succeed.
3. Improper Exception Handling
Exception handling can become tricky in batch processing due to its asynchronous nature. Uncaught exceptions can halt the entire process without informing you of the exact reason.
The Downside
When an exception occurs, it can either cause the job to fail or be retried infinitely without logging meaningful information. This can lead to loss of time and resources.
Recommendations
- Implement Exception Listeners: These allow you to manage errors more gracefully.
public class MyJobExecutionListener implements JobExecutionListener {
@Override
public void beforeJob(JobExecution jobExecution) {
// Initialization before job starts
}
@Override
public void afterJob(JobExecution jobExecution) {
if (jobExecution.getStatus() == BatchStatus.FAILED) {
// Log detailed error
}
}
}
Why Use Listeners
Listeners give you hooks to inspect the job at critical points, allowing you to react appropriately to failures.
4. Overloading a Single Step
While it might seem efficient to combine multiple processes into one step, this practice can lead to significant performance degradation.
The Problem
Overloaded steps can become a bottleneck, slowing down the entire job. They may also complicate debugging, as narrowing down issues will be more challenging.
Strategy to Avoid
- Break Up Your Steps: Isolate functionalities into different steps.
@Bean
public Job myJob() {
return jobBuilderFactory.get("myJob")
.incrementer(new RunIdIncrementer())
.flow(stepOne())
.next(stepTwo())
.end()
.build();
}
Advantages
This approach improves performance because it allows for more focused chunk processing and easier maintenance.
5. Misconfiguring Job Repository
Job repositories handle the persistence of job metadata. Misconfiguration can lead to issues such as overwriting job history or losing important execution data.
Problems You May Encounter
If the job repository is not accurately configured, you may find that jobs do not restart as expected or metadata is not preserved across app restarts.
Solutions
- Configure a Proper Job Repository:
@Bean
public JobRepository jobRepository() throws Exception {
return new JobRepositoryFactoryBean()
.setDataSource(dataSource())
.setTransactionManager(transactionManager())
.setIsolationLevelForCreate("ISOLATION_DEFAULT")
.afterPropertiesSet()
.getObject();
}
Why This Matters
A correctly configured job repository ensures that all essential job metadata is stored and retrievable, allowing for proper restart and tracking.
6. Not Monitoring Jobs
Failing to implement logging and monitoring can make it challenging to identify issues as they arise. You might find yourself in the dark about the job's status until it fails.
Importance of Monitoring
Real-time monitoring allows you to intervene proactively to stop a failing job before it causes major issues.
How To Implement It
-
Use Spring Batch Admin: Spring Batch Admin provides a clean interface to monitor batch jobs.
-
Integrate with Logging Libraries: Use loggers like log4j or slf4j to log the details of job execution and handle different levels of logging.
public class MyJobExecutionListener implements JobExecutionListener {
private static final Logger logger = LoggerFactory.getLogger(MyJobExecutionListener.class);
@Override
public void beforeJob(JobExecution jobExecution) {
logger.info("Job {} started", jobExecution.getJobInstance().getJobName());
}
}
Why This Will Help
An effective logging strategy can illuminate issues immediately and can save time in diagnosing problems.
Key Takeaways
Spring Batch is an incredibly versatile framework, but pitfalls can obstruct its potential. Understanding batch processing concepts, managing transactions, setting up proper exception handling, breaking down steps, configuring job repositories, and implementing monitoring practices can significantly improve your batch jobs' performance and reliability.
As you embark on your Spring Batch journey, keep these best practices in mind, and you will be well on your way to mastering batch processing.
For more in-depth information, feel free to explore these resources:
With these insights, you can tackle Spring Batch jobs confidently and effectively!
Checkout our other articles