Mastering Java Switch Expressions with Lambda Syntax
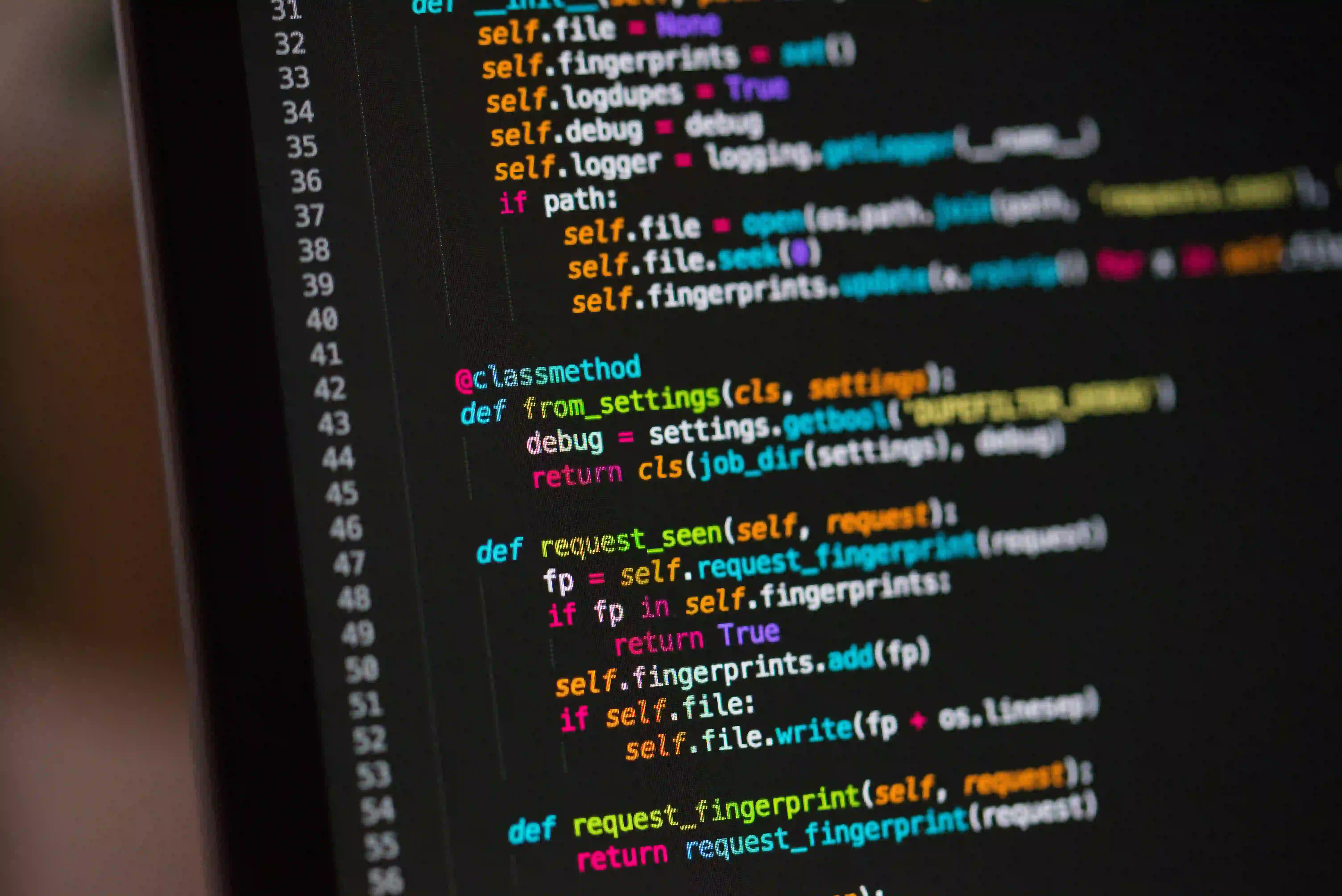
Mastering Java Switch Expressions with Lambda Syntax
As software developers, we are constantly evolving our coding practices and adapting to new features in the languages we work with. Java, with its rich set of functionalities, continues to inspire programmers. Among its recent developments, switch expressions introduced in Java 12, followed by enhancements in later versions, stand out as remarkable transformations to traditional switch statements. In this post, we will explore how to leverage switch expressions with lambda syntax effectively, enriching your coding practices and making your applications more concise and maintainable.
Understanding Switch Expressions
Switch statements in Java have long been a staple for decision-making but have limitations when it comes to returning values or managing control flows more cleanly. In Java 12, switch expressions emerged as a new way to simplify the control flow and enhance the readability of the code. Unlike traditional switch statements, switch expressions can return a value. Here's a brief comparison of the two:
-
Switch Statement: Used primarily for executing multiple paths of operation without returning a value. It’s limited in flexibility and often requires the use of break statements to prevent fall-through.
-
Switch Expression: Can return a value directly. It enhances readability, reduces boilerplate code, and eliminates the risk of fall-through since it's a more expressive construct.
Basic Example of a Switch Expression
Let's start with a basic example to underline the differences. The following code snippet illustrates how to use a switch expression:
public class SwitchExpressionExample {
public static void main(String[] args) {
String day = "MONDAY";
String dayType = switch (day) {
case "MONDAY", "FRIDAY", "SUNDAY" -> "Weekend";
case "TUESDAY", "WEDNESDAY", "THURSDAY" -> "Weekday";
default -> throw new IllegalArgumentException("Invalid day: " + day);
};
System.out.println("Type of day: " + dayType);
}
}
Explanation of the Code
-
Switch Expression: Instead of multiple case statements, expressions can return values using a concise syntax. This is particularly helpful in reducing boilerplate.
-
Case Labels: This example combines multiple case labels into a single line;
case "MONDAY", "FRIDAY", "SUNDAY"
will all lead to "Weekend". -
Return Value: Unlike the traditional switch, which required additional declarations to return a value, the switch expression directly evaluates and returns a string indicating whether the day is a "Weekend" or "Weekday".
-
Default Case: Instead of a simple print statement, we leverage
throw
to handle invalid cases more effectively, thus promoting better error handling.
Enhancements: Using Lambda Syntax in Switch Expressions
Java 14 introduced new capabilities for switch expressions, allowing us to improve code quality even further by utilizing lambda syntax and pattern matching. These functional programming techniques can lead to cleaner and more maintainable code.
Example with Lambda Syntax
Here’s a more advanced example demonstrating how we can use switch expressions in conjunction with lambda syntax:
import java.util.function.Function;
public class LambdaSwitchExample {
public static void main(String[] args) {
Function<String, String> dayTypeMapper = day -> switch (day) {
case "MONDAY", "FRIDAY", "SUNDAY" -> "Weekend";
case "TUESDAY", "WEDNESDAY", "THURSDAY" -> "Weekday";
default -> "Unknown Day";
};
String day = "WEDNESDAY";
String dayType = dayTypeMapper.apply(day);
System.out.println("Type of day: " + dayType);
}
}
Breakdown of This Example
-
Functional Interface: We define a
Function<String, String>
to encapsulate our day-to-type mapping logic. This showcases how lambdas can make our code more functional and reusable. -
Using Lambda: The lambda expression succinctly maps days to their types. This aligns with the principles of functional programming, simplifying how we manage operations on data.
-
Interacting with the Function: We call the
apply
method to get the corresponding type for the day. This abstracts the logic and keeps our main method clean and focused.
Benefits of Switch Expressions
1. Readability and Maintainability
Using switch expressions results in cleaner, more concise code. Enhanced readability allows developers to understand the logic quicker, which is essential for teamwork and long-term maintenance.
2. Reduced Boilerplate Code
The elimination of break statements and reduced verbosity mean that the essential logic takes center stage without unnecessary distractions.
3. Error Handling
Switch expressions shine with their ability to handle multiple cases and defaults using concise structures that make code error handling clearer.
For additional technical information, you can check out the official Java Documentation on Switch Expressions.
Practical Applications of Switch Expressions and Lambdas
Example: Calculating Areas
Let’s leverage switch expressions and lambda syntax to compute the area of different geometric shapes:
import java.util.function.Function;
public class AreaCalculator {
public static void main(String[] args) {
Function<String, Double> areaCalculator = shape -> switch (shape) {
case "CIRCLE" -> (radius) -> Math.PI * Math.pow(radius, 2);
case "SQUARE" -> (side) -> Math.pow(side, 2);
default -> throw new IllegalArgumentException("Unknown shape: " + shape);
};
double circleArea = areaCalculator.apply("CIRCLE").apply(5); // Radius of 5
double squareArea = areaCalculator.apply("SQUARE").apply(4); // Side of 4
System.out.println("Area of Circle: " + circleArea);
System.out.println("Area of Square: " + squareArea);
}
}
Code Explanation
-
Area Calculation Logic: The same switch expression structure employs parameterized lambdas which allow dynamic area computations based on user-input values.
-
Shape as Keys: Shapes are treated as keys in the switch structure, returning specific lambdas for area calculations.
-
Realization of Different Functions: The return of a lambda function allows users the flexibility to calculate areas with ease.
To Wrap Things Up
By mastering switch expressions coupled with lambda syntax, Java programmers significantly optimize their coding experience. The enhanced readability, reduced boilerplate, and powerful error handling mechanisms make switch expressions indispensable tools in modern Java development.
As you incorporate this knowledge into your own projects, be sure to consider how switch expressions can simplify your control flow and improve code quality. For more advanced insights, feel free to explore Java's Lambda Expressions Documentation.
With continued practice, you'll find that these concepts not only streamline your Java applications but also pave the way for writing robust, maintainable code in your development journey. Happy coding!