Overcoming Common Pitfalls in Continuous Testing Strategies
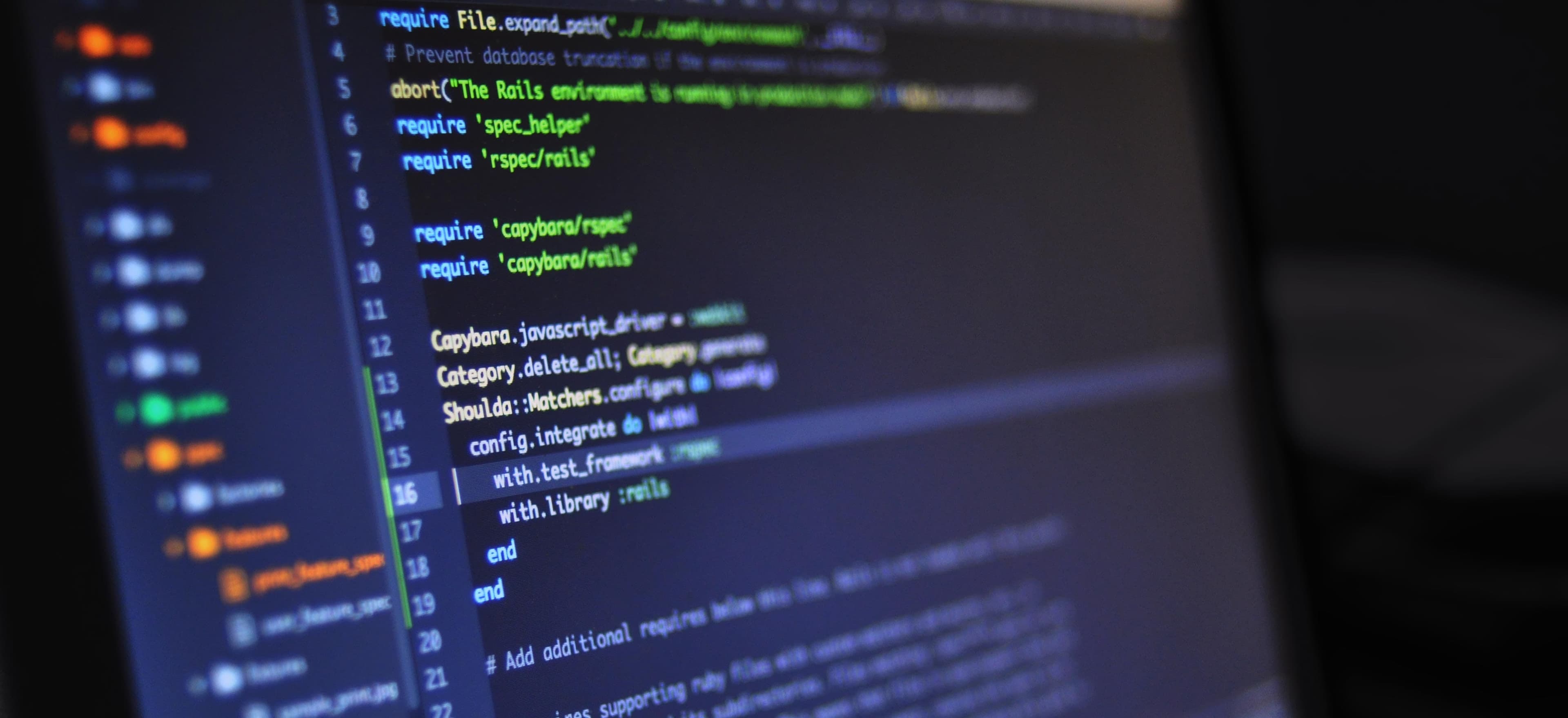
- Published on
Overcoming Common Pitfalls in Continuous Testing Strategies
Continuous testing is a vital component of modern software development life cycles, particularly for teams applying agile and DevOps methodologies. It allows teams to identify issues quickly, reduce time to market, and improve overall software quality. However, several common pitfalls can hinder the effectiveness of continuous testing strategies. In this blog post, we'll explore these pitfalls and provide actionable insights to help you overcome them.
Understanding Continuous Testing
Continuous testing refers to the practice of executing automated tests as part of the software delivery pipeline. This means that tests are run frequently and provide feedback throughout the development process. By integrating testing into this cycle, teams can ensure that they deliver reliable software swiftly and efficiently.
Why Continuous Testing Matters
- Faster Feedback Loops: Developers receive instant feedback about the quality of their code.
- Reduced Risk: Continuous testing helps identify defects before they escalate into critical issues.
- Cost Efficiency: Early detection of bugs is less expensive than fixing them after production release.
Common Pitfalls in Continuous Testing
1. Lack of Test Automation
A common misconception is that continuous testing requires a fully automated test suite from the start. While automation is critical, expecting to automate every test immediately can lead to overwhelming complexity.
Solution: Start small. Focus on automating the most critical tests first, such as regression tests or those high-impact areas of your application. As you gain confidence and understanding of your testing needs, gradually expand your automated test coverage.
Code Insight
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3)); // Simple addition test
}
}
In this example, we've automated a simple addition test for a Calculator
class. Quick wins like this make for a strong foundation in test automation without overwhelming your initial efforts.
2. Overlooking Test Maintenance
Over time, as code evolves, tests can become outdated or irrelevant. Neglecting test maintenance can lead to "test rot,” where tests fail not due to actual issues but because they are based on outdated implementations.
Solution: Implement a routine for reviewing and updating test cases. Use features like tag-based execution to run specific tests that may require refreshing or to exclude those failing tests that are no longer relevant.
3. Insufficient Test Coverage
While automated tests can provide fast feedback, if the tests do not cover all critical functionalities, your continuous testing strategy will likely leave gaps.
Solution: Conduct regular coverage analysis using tools such as JaCoCo. These tools can provide insights into which parts of the codebase are tested and which are not.
Code Insight
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
public class TestRunner {
public static void main(String[] args) {
Result result = JUnitCore.runClasses(CalculatorTest.class);
System.out.println("Number of tests run: "+ result.getRunCount());
System.out.println("Failures: "+ result.getFailureCount());
}
}
In this runner, we can see how many tests were executed, providing insight into our test coverage efforts. Regularly check the outputs from such tools to maintain robust coverage.
4. Testing in Isolation
Testing in isolation without considering the entire system can lead to deceptive results. Tests can pass in local environments but fail when integrated into the production system due to missed dependencies.
Solution: Adopt a collaborative testing approach involving developers, testers, and operations teams. Integration tests should replicate how various components interact in a real-world scenario.
5. Poorly Defined Test Cases
Ambiguous test cases can lead to confusion among testing teams. Clear, concise test cases that are easy to understand are crucial for effective communication and execution.
Solution: Use Behavior Driven Development (BDD) frameworks such as Cucumber or JBehave, which promote writing test cases in a natural language format.
Steps to a More Effective Continuous Testing Strategy
Embrace the Shift Left Approach
The "Shift Left" strategy emphasizes testing earlier in the software development cycle. The earlier feedback is received, the better the chances of resolving issues quickly.
- Encourage collaboration during requirement gathering.
- Define acceptance criteria upfront.
- Run tests against early builds and integrations.
Develop a Robust Test Strategy
- Identify critical business functionalities and ensure they have sufficient coverage.
- Regularly review and refine your test cases to ensure they remain relevant.
- Leverage mocking frameworks to isolate components and speed up the testing process.
Utilize Continuous Integration (CI) and Continuous Delivery (CD) Tools
Integrating testing with CI/CD tools allows for a streamlined approach to deploying code upon successful test executions. Tools like Jenkins, CircleCI, and TravisCI can automate your testing pipelines effectively.
Monitor and Analyze Testing Metrics
Using metrics is essential for understanding the effectiveness of your testing strategy. Track:
- Test pass/fail rates
- Time taken to run tests
- Test execution frequency
These metrics can provide insights into your testing health and help identify areas for improvement.
Wrapping Up
Continuous testing is an evolving discipline that plays a pivotal role in maintaining software quality in fast-paced development environments. By addressing common pitfalls, employing effective strategies, and continuously refining your approach, you can enhance your continuous testing practices.
For further reading, consider diving deeper into Agile Testing Strategies or exploring Best Practices for Automated Testing.
By systematically identifying these pitfalls and allowing for proper planning and execution of a robust testing strategy, teams can overcome obstacles and succeed in delivering high-quality software. The road may be challenging, but with the right approach, the payoff is significant. Happy testing!