How to Fix Resizable Canvas Issues in JavaFX
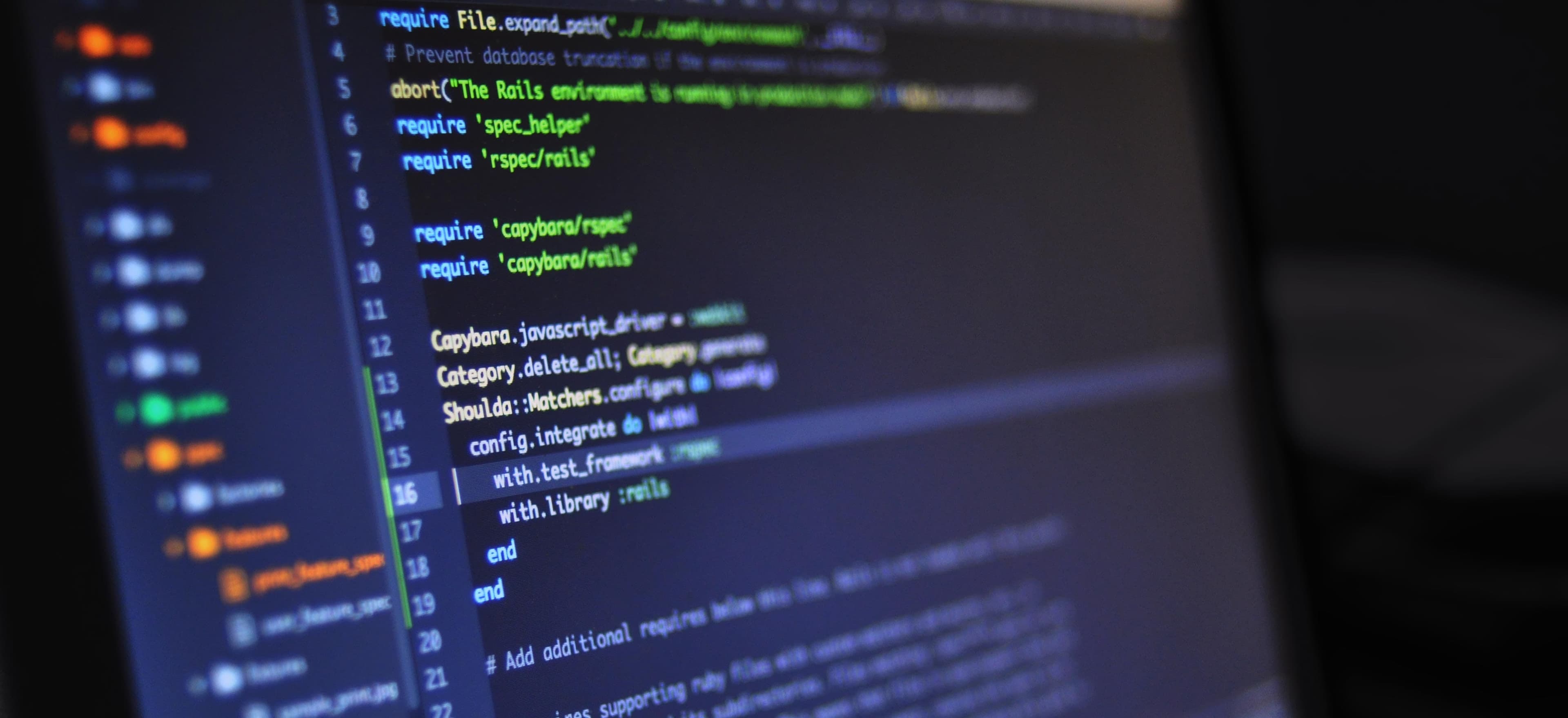
- Published on
How to Fix Resizable Canvas Issues in JavaFX
JavaFX is a powerful framework for building rich client applications, particularly in gaming and interactive applications. One common issue developers face when building applications using JavaFX is managing a resizable canvas. In this blog post, we will explore how to create a responsive canvas that adapts to window resizing without distorting the content.
Understanding the Challenge
When you create a canvas in JavaFX, it is often a fixed size. Resizing the window does not automatically adjust the canvas size or the content drawn on it. This leads to several complications, including:
- Distortion of Graphics: As the window resizes, the graphics may appear stretched or squished.
- Re-drawing: The content may need to be redrawn every time the window size changes.
- Performance Issues: Continuous resizing events can lead to performance bottlenecks.
The Solution
To tackle these issues, we need to execute a few key concepts:
- Listening to Resize Events: We should listen to the scene's size changes so we can react accordingly.
- Adjusting Canvas Size: We will resize the canvas to match the current window size.
- Redrawing Content: After resizing, we will redraw the content on the canvas to ensure it maintains its aspect ratio.
Example Code
Here is a simple example of how to implement a resizable canvas in JavaFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ResizableCanvasExample extends Application {
private Canvas canvas;
@Override
public void start(Stage primaryStage) {
canvas = new Canvas(400, 400);
GraphicsContext gc = canvas.getGraphicsContext2D();
drawShapes(gc, 400, 400); // Initial drawing
// Setup resize listener
primaryStage.widthProperty().addListener((obs, oldVal, newVal) -> resizeCanvas());
primaryStage.heightProperty().addListener((obs, oldVal, newVal) -> resizeCanvas());
StackPane root = new StackPane();
root.getChildren().add(canvas);
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("Resizable Canvas Example");
primaryStage.setScene(scene);
primaryStage.show();
}
private void resizeCanvas() {
double width = canvas.getWidth();
double height = canvas.getHeight();
// Create a new canvas with the updated size
Canvas tempCanvas = new Canvas(canvas.getScene().getWidth(), canvas.getScene().getHeight());
GraphicsContext gc = tempCanvas.getGraphicsContext2D();
// Redraw shapes with new size
drawShapes(gc, tempCanvas.getWidth(), tempCanvas.getHeight());
// Replace old canvas with new canvas
((StackPane) canvas.getParent()).getChildren().set(0, tempCanvas);
canvas = tempCanvas;
}
private void drawShapes(GraphicsContext gc, double width, double height) {
// Clear the canvas
gc.clearRect(0, 0, width, height);
// Draw some shapes, using width and height for responsiveness
gc.fillRect(10, 10, width / 4, height / 4); // A rectangle
gc.fillOval(width / 2, height / 2, width / 4, height / 4); // A circle
gc.fillText("Resizable Graphics", width / 4, height / 4);
}
public static void main(String[] args) {
launch(args);
}
}
Code Walkthrough
-
Canvas Creation: Initially, we create a canvas with a specified width and height.
-
Listener for Resize Events: We set a listener on the width and height properties of the primary stage. Whenever the window resizes, the
resizeCanvas()
method is invoked. -
Resizing the Canvas: Within
resizeCanvas()
, we create a new canvas. This canvas has dimensions that correspond to the current stage width and height. -
Redrawing Graphics: After creating a new canvas, the
drawShapes()
method is called to render the current graphical elements based on the new dimensions. This ensures that the shapes maintain their relative positions and sizes.
Ensuring Aspect Ratio
In some cases, you may want to maintain the aspect ratio of the graphics regardless of the resizing. To achieve this, you can modify the resizeCanvas()
method to include conditions that respect the aspect ratio.
private void resizeCanvas() {
double aspectRatio = 1.0; // Maintain Aspect Ratio
double newWidth = canvas.getScene().getWidth();
double newHeight = newWidth / aspectRatio;
if (newHeight > canvas.getScene().getHeight()) {
newHeight = canvas.getScene().getHeight();
newWidth = newHeight * aspectRatio;
}
Canvas tempCanvas = new Canvas(newWidth, newHeight);
GraphicsContext gc = tempCanvas.getGraphicsContext2D();
drawShapes(gc, tempCanvas.getWidth(), tempCanvas.getHeight());
((StackPane) canvas.getParent()).getChildren().set(0, tempCanvas);
canvas = tempCanvas;
}
Performance Considerations
Creating a new canvas and redrawing shapes can become resource-intensive, especially with large and intricate graphics. Below are some strategies to optimize performance:
- Throttling Resize Events: Instead of redrawing on every resize event, consider implementing a throttle mechanism that only triggers a redraw after resizing is complete.
- Using a Buffer: Store images that can be reused instead of redrawing basic shapes repeatedly.
- Control Redraw Frequency: Utilize a dedicated method to redraw at intervals rather than every change in size, providing a smoother experience.
Closing Remarks
Creating a responsive, resizable canvas in JavaFX might seem challenging, but with the right strategies, it's entirely manageable. This technique allows you to create dynamic and user-friendly applications that can adapt to various screen sizes.
For further exploration of graphics and JavaFX, consider visiting the official JavaFX documentation for a healthier grasp on the subject.
By implementing these practices, you can deliver an enhanced user experience that matches the high standards of modern applications. Happy coding!