Struggling with MyBatis 3 and Spring Integration? Here's Help!
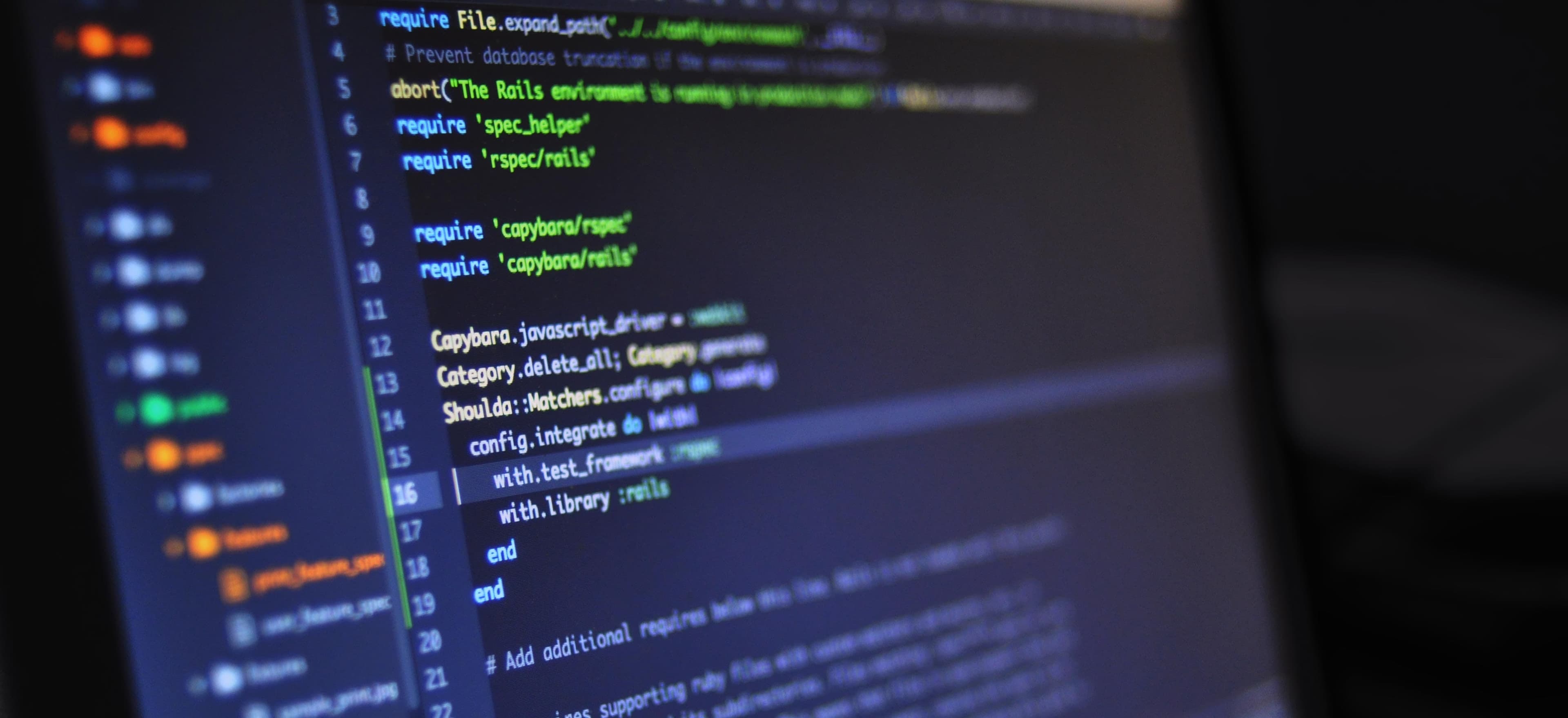
- Published on
Struggling with MyBatis 3 and Spring Integration? Here's Help!
Integrating MyBatis 3 with Spring can be daunting, especially for developers new to these technologies. Both frameworks offer powerful capabilities, but their combination often leads to confusion. By breaking down their integration into simpler components, this guide aims to clarify the process and improve your understanding.
What are MyBatis and Spring?
MyBatis
MyBatis is a persistence framework that facilitates the interaction between application code and relational databases. Unlike full-fledged Object-Relational Mapping (ORM) libraries like Hibernate, MyBatis uses SQL directly while still allowing a flexible mapping of SQL results to Java objects.
Spring Framework
Spring is a comprehensive framework that provides various tools for enterprise Java development. Spring’s primary goal is to promote good programming practices for developing Java applications, making it easier to manage business objects.
When used together, MyBatis and Spring allow developers to harness the strengths of both frameworks while simplifying resource management and database transactions.
Setting Up Your Project
Using Maven, you can create a Spring and MyBatis project quickly. Here’s how you can structure your pom.xml
dependencies:
<dependencies>
<!-- Spring Context -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.6</version>
</dependency>
<!-- Database Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.25</version>
</dependency>
</dependencies>
Make sure to adjust your versions to maintain compatibility.
Configuration
Spring Configuration
You need to configure Spring’s ApplicationContext
to manage your MyBatis sessions and custom mappers effectively. Here’s a simple configuration:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="org.apache.commons.dbcp2.BasicDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/testdb" />
<property name="username" value="user" />
<property name="password" value="password" />
</bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
</bean>
<bean id="sqlSessionTemplate" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg ref="sqlSessionFactory" />
</bean>
</beans>
Explanation
- Data Source: This is required to establish a connection with the MySQL database.
- SqlSessionFactory: This is responsible for creating MyBatis
SqlSession
instances. - SqlSessionTemplate: This template class simplifies interactions with
SqlSession
.
MyBatis Mapper Configuration
Create an XML file for your MyBatis mappers, for instance, UserMapper.xml
:
<mapper namespace="com.example.mapper.UserMapper">
<select id="getUserById" parameterType="int" resultType="com.example.model.User">
SELECT * FROM users WHERE id = #{id}
</select>
</mapper>
In this XML file:
- We define a select operation to retrieve a user by their ID.
Java Model and Mapper Interface
Define your User
model:
package com.example.model;
public class User {
private int id;
private String name;
// Getters and Setters
}
And the corresponding mapper interface:
package com.example.mapper;
import com.example.model.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper {
User getUserById(int id);
}
Service Layer
Now let's create a service class to utilize our mapper:
package com.example.service;
import com.example.mapper.UserMapper;
import com.example.model.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserMapper userMapper;
@Autowired
public UserService(UserMapper userMapper) {
this.userMapper = userMapper;
}
public User retrieveUser(int id) {
return userMapper.getUserById(id);
}
}
Controller Layer
To expose the service through a RESTful API, you could add a controller like this:
package com.example.controller;
import com.example.model.User;
import com.example.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public User getUser(@PathVariable int id) {
return userService.retrieveUser(id);
}
}
Testing
To ensure your setup is correct, you may want to create a test case. Here's an example using JUnit and Spring Test:
package com.example;
import static org.assertj.core.api.Assertions.assertThat;
import com.example.model.User;
import com.example.service.UserService;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class UserServiceTests {
@Autowired
private UserService userService;
@Test
public void testGetUserById() {
User user = userService.retrieveUser(1);
assertThat(user).isNotNull();
assertThat(user.getName()).isEqualTo("John Doe");
}
}
Explanation
- This test checks whether the
retrieveUser
method returns a validUser
object for a given ID.
Common Challenges and Solutions
Session Management Issues
A common pitfall during integration is improper session management. Ensure that you manage MyBatis sessions properly, especially in a multi-threaded environment.
- Solution: Always utilize the SqlSessionTemplate to manage your sessions, as it is thread-safe.
SQL and Object Mapping Errors
Make sure your SQL queries perfectly align with your domain models. Mismatches can lead to runtime exceptions.
- Solution: Use MyBatis Mapper XML for complex queries to ensure that your mappings are effectively declared.
The Closing Argument
Integrating MyBatis 3 with Spring is not an insurmountable challenge. By following best practices and leveraging Spring’s capabilities, you can create a robust application that handles database interactions efficiently.
As you dive deeper into MyBatis and Spring, consider exploring more advanced features such as batch processing and caching strategies. For further reading, check out the official documentation for Spring and MyBatis.
Feel free to ask questions or share your experiences in the comments. Happy coding!