Overcoming Flyway Migration Issues in JOOQ Development
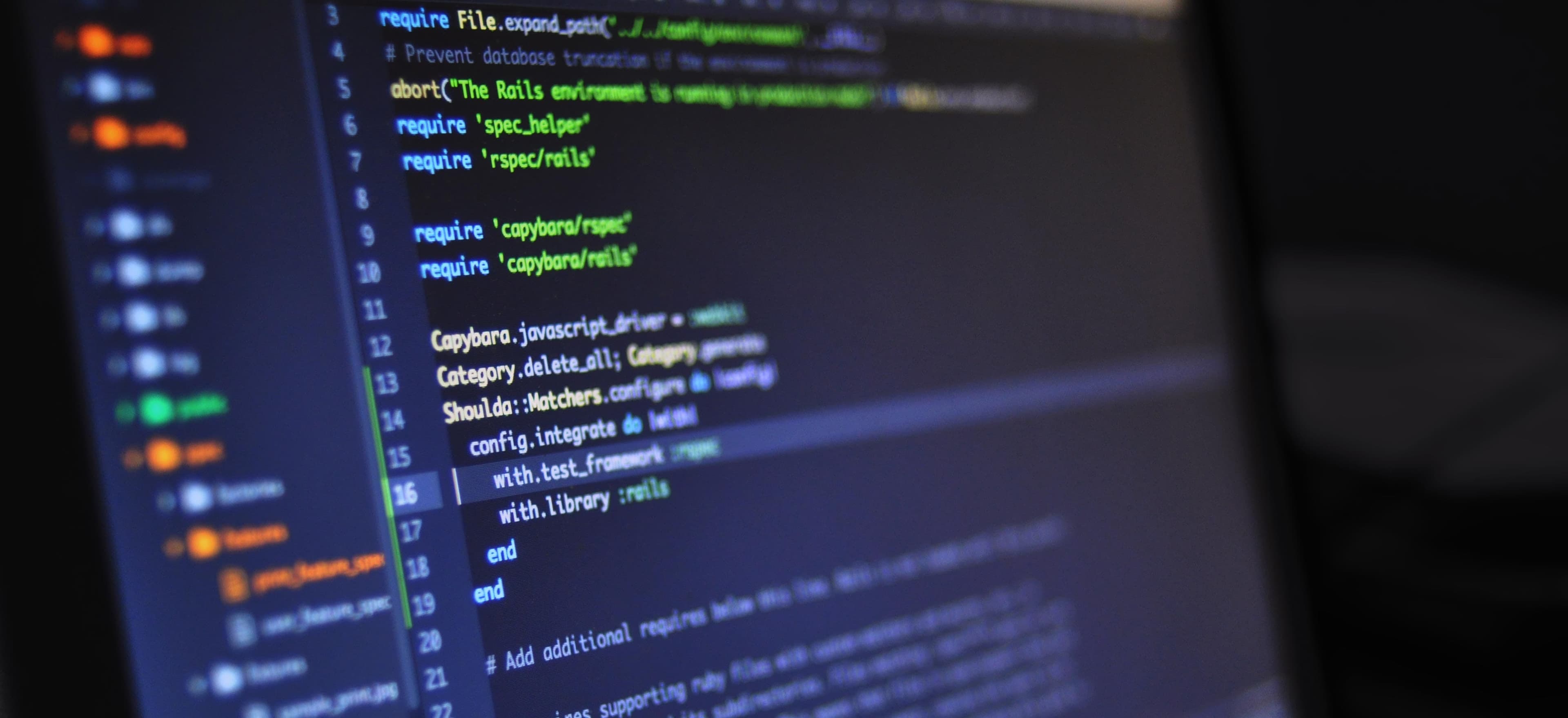
- Published on
Overcoming Flyway Migration Issues in JOOQ Development
In the world of Java development, database migrations play a crucial role in ensuring the smooth evolution of your application's schema. Flyway is a popular tool to manage database migrations, while JOOQ (Java Object Oriented Querying) is a powerful library for building SQL queries in a type-safe manner. However, integrating Flyway with JOOQ can sometimes lead to issues that may hinder your development process. This post aims to explore common Flyway migration problems in JOOQ development and provides strategies to overcome them.
Understanding Flyway and JOOQ
Before diving into the issues, let’s take a moment to define Flyway and JOOQ.
What is Flyway?
Flyway is an open-source database migration tool that allows you to version control your database schema. It uses SQL scripts or Java migrations to keep track of changes in your database. Flyway is highly effective, as it supports multiple databases and provides a simple mechanism to apply and undo migrations.
What is JOOQ?
JOOQ is a Java library that facilitates building type-safe SQL queries. It generates Java code from your database schema, enabling developers to write queries directly using Java, rather than relying on string-based queries. JOOQ enriches the development experience by providing compile-time safety, which significantly reduces runtime errors in SQL execution.
Common Issues in Flyway-JOOQ Integration
1. Migration Order
One of the most common issues faced when using Flyway with JOOQ is managing the order of migrations. Flyway executes migrations based on version numbers, and if these are not correctly incremented or defined, migrations can execute out of order.
Solution
Always ensure that your migration files are consistently numbered. For example, follow the convention:
V1__create_user_table.sql
V2__add_email_to_user.sql
V3__create_orders_table.sql
By following a clear and systematic numbering, you ensure Flyway executes them in the intended order.
2. Rollbacks
Another issue developers encounter is rollback management. Flyway does not support rollbacks natively for all types of migrations. This can lead to inconsistencies when JOOQ tries to populate new tables that have been partially rolled back.
Solution
Implement "Undo" migrations where possible. For instance, if you have added a new table, create an undo script to drop it:
-- V2__create_user_table.sql
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(100),
password VARCHAR(100)
);
-- U1__drop_user_table.sql
DROP TABLE IF EXISTS users;
This way, if a migration fails, you can revert back to a known state.
3. Different Environments
Development, testing, and production environments often have different database schemas. If Flyway migrations are not synchronized across environments, you may run into discrepancies that can break your JOOQ queries.
Solution
Maintain database migrations in a source-control system and use database seeding to bring all environments to a consistent state. Ensure that migrations are always applied in the same sequence across all environments before running your application.
4. Compatibility Issues
Flyway Migrations can also have compatibility issues with JOOQ if the generated classes become outdated due to changes in the database schema.
Solution
Automate the Code Generation
Integrate JOOQ code generation into your build process (e.g., Maven or Gradle), which allows updating the generated classes whenever a migration is applied. This is how you can set it up in Maven:
<build>
<plugins>
<plugin>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen-maven</artifactId>
<version>${jooq.version}</version>
<executions>
<execution>
<goals>
<goal>generate</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
5. Version Conflicts
Version conflicts occur when multiple migrations attempt to modify the same database object (like a table or column) simultaneously. This can lead to failed migrations or corrupted states.
Solution
Establish a clear communication policy among developers regarding schema changes. Consider using a Branching strategy such as Git Flow, ensuring that developers can discuss and merge migration files intelligently.
Sample JOOQ Code Snippet
Suppose you want to retrieve all users from the database using JOOQ. The following code snippet illustrates this:
// Setting up the DSLContext for JOOQ
DSLContext create = DSL.using(connection, SQLDialect.MYSQL);
// Fetching users from the database
Result<Record> result = create.select()
.from("users")
.fetch();
// Processing the results
for (Record record : result) {
Integer id = record.getValue("id", Integer.class);
String username = record.getValue("username", String.class);
System.out.println("User ID: " + id + ", Username: " + username);
}
Why Use JOOQ for Database Operations?
- Type Safety: Avoids runtime errors by catching SQL errors at compile time.
- Fluent API: Enables easy building of complex SQL queries.
- Database Independence: Works across various SQL databases with minimal changes.
- Integration with Java: Seamless integration with existing Java applications.
Bringing It All Together
Integrating Flyway with JOOQ offers powerful capabilities to manage your database schema safely and efficiently. By understanding common migration issues and implementing the provided strategies, you can avoid pitfalls and enhance your development workflow.
For further reading, consult the following resources:
Both tools are incredibly useful, and with the right strategies, you can leverage them to create robust, maintainable applications. Whether you are a seasoned developer or just beginning, mastering these tools will greatly enhance your database management capabilities in Java development.
Checkout our other articles