Is Garbage Collection Logging Slowing Your Java App?
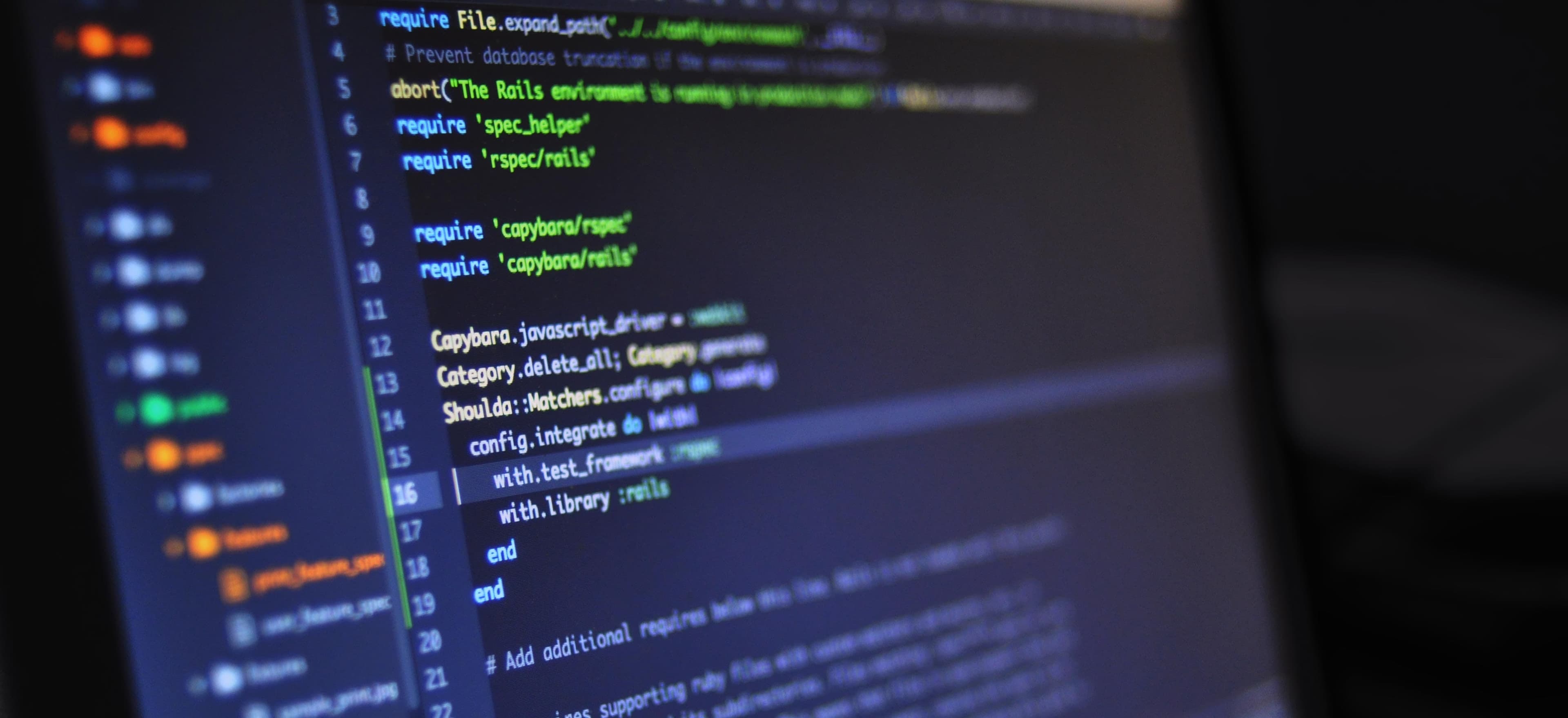
- Published on
Is Garbage Collection Logging Slowing Your Java App?
Garbage collection (GC) is a crucial automatism in Java that manages memory automatically by reclaiming memory taken by objects that are no longer in use. For developers upgrading their applications or fine-tuning performance, understanding Garbage Collection is vital. However, GC logging can introduce performance challenges. In this blog post, we'll dive deep into what garbage collection logging is, how it affects performance, and strategies to optimize your Java application without sacrificing visibility.
Understanding Garbage Collection in Java
Garbage Collection in Java is a process that automatically identifies and disposes of objects that are no longer referenced in the application. This is an intrinsic feature of Java and greatly simplifies memory management for developers. However, this process does come at a cost. The garbage collector needs CPU time for the collection and can lead to application pause times that affect performance.
Key GC Terms
- Minor GC: Cleans up the young generation where new objects are allocated.
- Major GC: Cleans up the old generation – it is usually more expensive in terms of time.
- Full GC: Cleans both the young and old generation and can cause significant pauses.
To learn more about the types of garbage collectors available in Java, you can refer to the Oracle Documentation.
What is Garbage Collection Logging?
GC logging involves emitting logs that track GC events. The data can be valuable for performance tuning - providing insights into when and why collections occur.
However, logging itself may result in overhead and impact application performance. To put it simply, logging generates additional workload, and if you're not careful, this can lead to slower response times and increased latency.
Common GC Logging Flags
To enable GC logging, you typically use the following flags:
-XX:+PrintGCDetails
-XX:+PrintGCDateStamps
-XX:+PrintGCTimeStamps
-XX:+PrintTenuringDistribution
These options provide a wide variety of information about the garbage collection process, including timestamps, duration of the collection, and the memory freed. However, the more logging options you enable, the more overhead you may introduce.
Does Garbage Collection Logging Slow Down Your Application?
The Overhead of Logging
When you enable GC logging, it may indeed slow down your Java application. Here are the main reasons why:
- CPU Usage: Each log entry requires CPU cycles. More extensive logging means your application spends more time logging instead of executing core business logic.
- I/O Blocking: Writing logs to disk can be a blocking operation, especially if logs are written synchronously. This can lead to delays in application response.
- Increased Memory Usage: The logging framework may also consume extra memory for output buffers, potentially aggravating the memory footprint.
Measuring the Impact
To measure the impact of GC logging on your application, you can use Java's built-in monitoring tools like JVisualVM or Java Flight Recorder. Here’s an example of how to activate it:
java -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr -jar YourApp.jar
This allows you to record application performance metrics and examine the impact of GC logging during runtime.
Profiling Your Application
Before disabling GC logging entirely, you should profile your application to determine the root cause of any slowdowns. Profiling tools can help you isolate problems related to garbage collection versus other potential issues, such as thread contention or inefficient algorithms.
A code snippet to help profile GC events:
public class GCDemo {
public static void main(String[] args) {
// Adjust these parameters to observe performance changes
int numberOfIterations = 500_000;
// Create a large number of temporary objects
for (int i = 0; i < numberOfIterations; i++) {
String tempString = new String("Garbage Collection Test " + i);
}
// Force Garbage Collection
System.gc();
// Output to confirm completion
System.out.println("Completed iterations and requested garbage collection.");
}
}
Why This Works
This simple program creates a large number of temporary strings and explicitly invokes garbage collection towards the end. This will generate enough load to observe how the Garbage Collector performs differently with and without logging.
Strategies to Optimize GC Logging
If you've identified that GC logging is indeed impeding performance, consider the following strategies to optimize it:
1. Adjust Logging Levels
Instead of maximizing log details, focus on concise yet meaningful logs. For example, use only -XX:+PrintGC
to minimize logged data while still receiving essential information.
2. Log to a File Asynchronously
By configuring your application to log to a file asynchronously, you can prevent I/O blocking. Here’s a simple example using Log4J:
<Configuration>
<Appenders>
<Async name="AsyncLogger">
<File name="FileLogger" fileName="gc.log">
<PatternLayout pattern="%d{HH:mm:ss.SSS} %-5p %c{1} - %m%n"/>
</File>
</Async>
</Appenders>
</Configuration>
By using an asynchronous appender, your application can continue operating while logging occurs in the background.
3. Profile Regularly
Integrating a profiling mechanism during your development lifecycle can help mitigate issues before they escalate. Regular detecting and optimizing of GC behavior will ensure you don't hit performance roadblocks as your codebase evolves.
My Closing Thoughts on the Matter
In summary, while garbage collection logging can provide essential insights for performance tuning your Java application, it can also introduce overhead that slows down your app. Balancing visibility and performance is key to maintaining a healthy Java application. Always remember that effective profiling and optimization save you time and resources.
For further reading on optimizing your Java application, check out the following essential resources:
- Java Performance Tuning
- Understanding Garbage Collection in Java
Take Action
As a Java developer, it's essential to leverage garbage collection logging effectively while monitoring its impact on your application's performance. Implement the suggested strategies, and consider regularly profiling your application to maintain optimal performance. Happy coding!
Checkout our other articles