Overcoming Java Module System Compatibility Issues
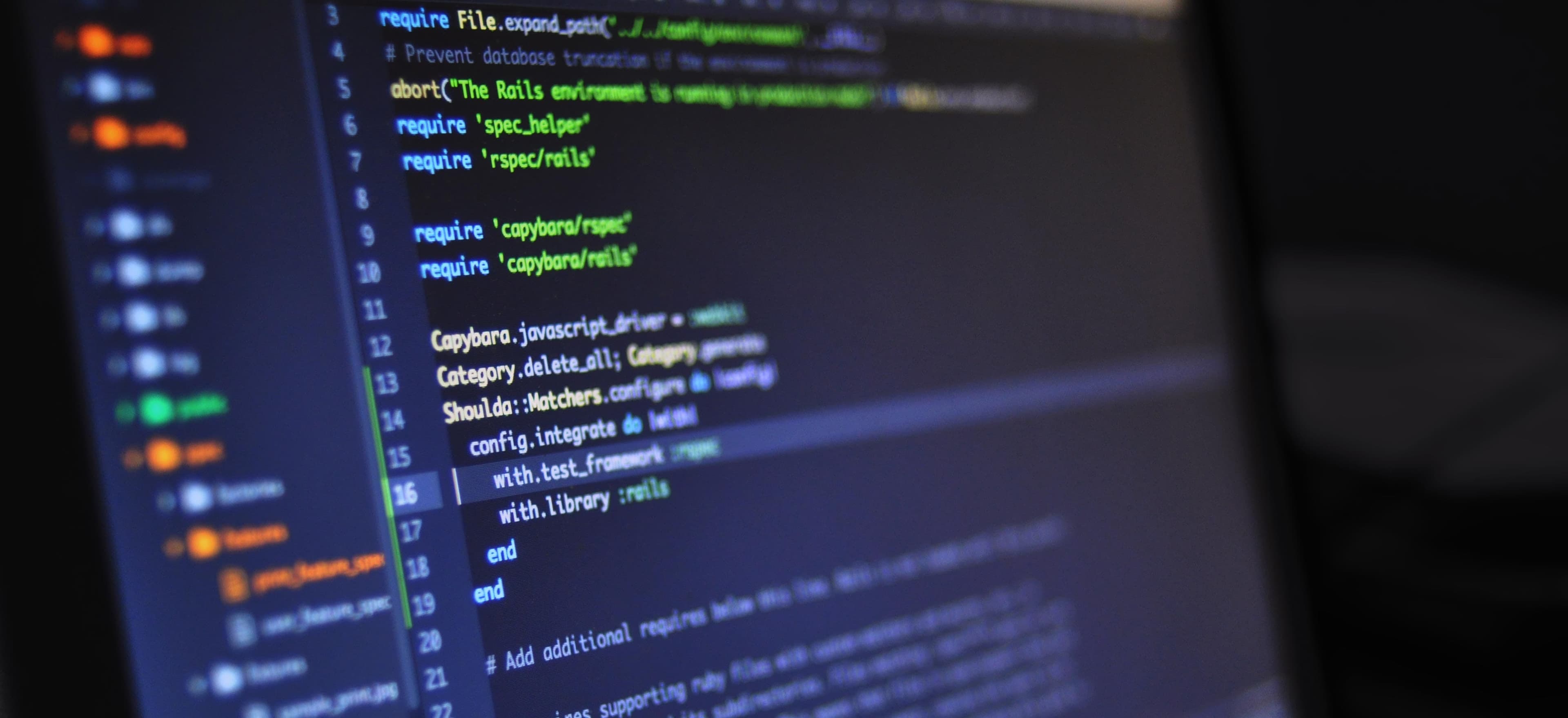
- Published on
Overcoming Java Module System Compatibility Issues
In the landscape of Java development, the introduction of the Java Platform Module System (JPMS) with Java 9 marked a significant shift in how we manage dependencies and modularize applications. While the module system brings many advantages, it also introduces compatibility challenges, especially for projects transitioning from older versions of Java. This blog post delves into the common issues developers face when adopting the module system, offers practical solutions, and highlights best practices.
Understanding the Java Module System
Before diving into the compatibility issues, it is essential to grasp the core concept of the Java Module System, which provides a way to group related packages and resources into modules. This system brings several benefits:
- Encapsulation: Modules can hide internal packages, exposing only what is necessary.
- Reduced Namespace Conflicts: Modules allow for better management of package namespaces.
- Improved Performance: The JVM can optimize module loading, improving startup time.
However, the transition to JPMS can cause compatibility issues, especially with older libraries that were not designed with modularity in mind.
Common Compatibility Issues
1. Non-modular Libraries
Many third-party libraries are not modularized and do not provide module-info.java
files. This can lead to problems, such as:
- Visibility Issues: Non-modularized libraries might not expose the necessary classes when run in a modular environment.
- Reflection Warnings: Any reflective access to classes and methods might trigger warnings due to encapsulation rules.
Solution: Use the --add-modules
and --add-exports
command-line options to explicitly add non-modular libraries to your module path.
java --module-path my-library.jar --add-modules my.module --add-exports my.module/com.example.myclass=my.other.module -jar my-app.jar
This command lets you access the specific packages within non-modular libraries, ensuring they work seamlessly with your modularized application.
2. Split Packages
In JPMS, a split package occurs when two different modules contain the same package name. This can lead to class-loading conflicts.
Solution: Refactor your code to ensure that each package is contained within a single module. This might require merging libraries or adjusting package structures. Here's a refactoring example:
// Before: Split package example
// Module A
package com.example.utils;
public class UtilityA {}
// Module B
package com.example.utils;
public class UtilityB {}
// After: Refactoring to avoid split packages
// Module C
package com.example.utilities;
public class Utility { ... }
3. Access Controls and Encapsulation
With the introduction of modules, encapsulation becomes more robust. A package that is not exported cannot be accessed by other modules. This can be a challenge when dealing with legacy codebases.
Solution: Identify the required packages and use the exports
directive in your module-info.java
to expose the necessary classes. For example:
module com.example.myapp {
exports com.example.myapp.services;
exports com.example.myapp.utils;
}
4. Classpath vs. Module Path Confusion
Developers often mix classpath and module path, leading to unexpected behavior. The module path is where modules are located, while the classpath is used for traditional Jar files.
Solution: Always clearly specify whether you're using the classpath or module path when running your application or tests. By adhering to a consistent structure, you can avoid these mix-ups:
// Running with module path
java --module-path mods -m com.example.myapp/com.example.Main
// Running with classpath
java -cp "libs/*:." com.example.Main
5. Transitive Dependencies
In modular systems, declaring dependencies becomes crucial. If one module relies on another, you must ensure that it can access that module's exports.
Solution: Use the requires transitive
directive to propagate dependencies down to modules that depend on your module.
module com.example.moduleA {
requires transitive com.example.moduleB;
}
This way, com.example.moduleC
, which requires com.example.moduleA
, will automatically have access to com.example.moduleB
.
Transition Planning
Transitioning to JPMS requires careful planning and execution. Here are some strategies to consider:
-
Audit Your Dependencies: Assess which libraries your application utilizes and their compatibility with JPMS. Tools like jdeps can help.
-
Incremental Migration: Begin the migration process with small modules, allowing you to identify issues without impacting the entire codebase.
-
Documentation and Training: Educate your team about modular programming principles, JPMS, and its best practices to ensure a smooth transition.
-
Test Thoroughly: Ensure you have a robust testing framework in place to catch any issues resulting from modularization.
To Wrap Things Up
The Java Module System offers a powerful means to better organize and encapsulate your code. However, transitioning to this new paradigm presents its own set of challenges. By understanding the common compatibility issues and following the proposed solutions, you can navigate the complexities of JPMS effectively.
By embracing modularity, you will not only enhance code maintainability and clarity but also future-proof your applications against the evolving needs of the Java ecosystem. As Java continues to evolve, keeping up with these advancements will ensure you're well-equipped to build robust, modular applications.
For additional reading, consider checking out the official JPMS documentation and resources like Effective Java by Joshua Bloch for best practices in Java programming.
By implementing these strategies, your journey through the Java Module System will be smoother, positioning you as a forward-thinking Java developer ready to tackle the challenges of the modern programming landscape.
Checkout our other articles