ThreadLocal: Unraveling Its Hidden Performance Issues
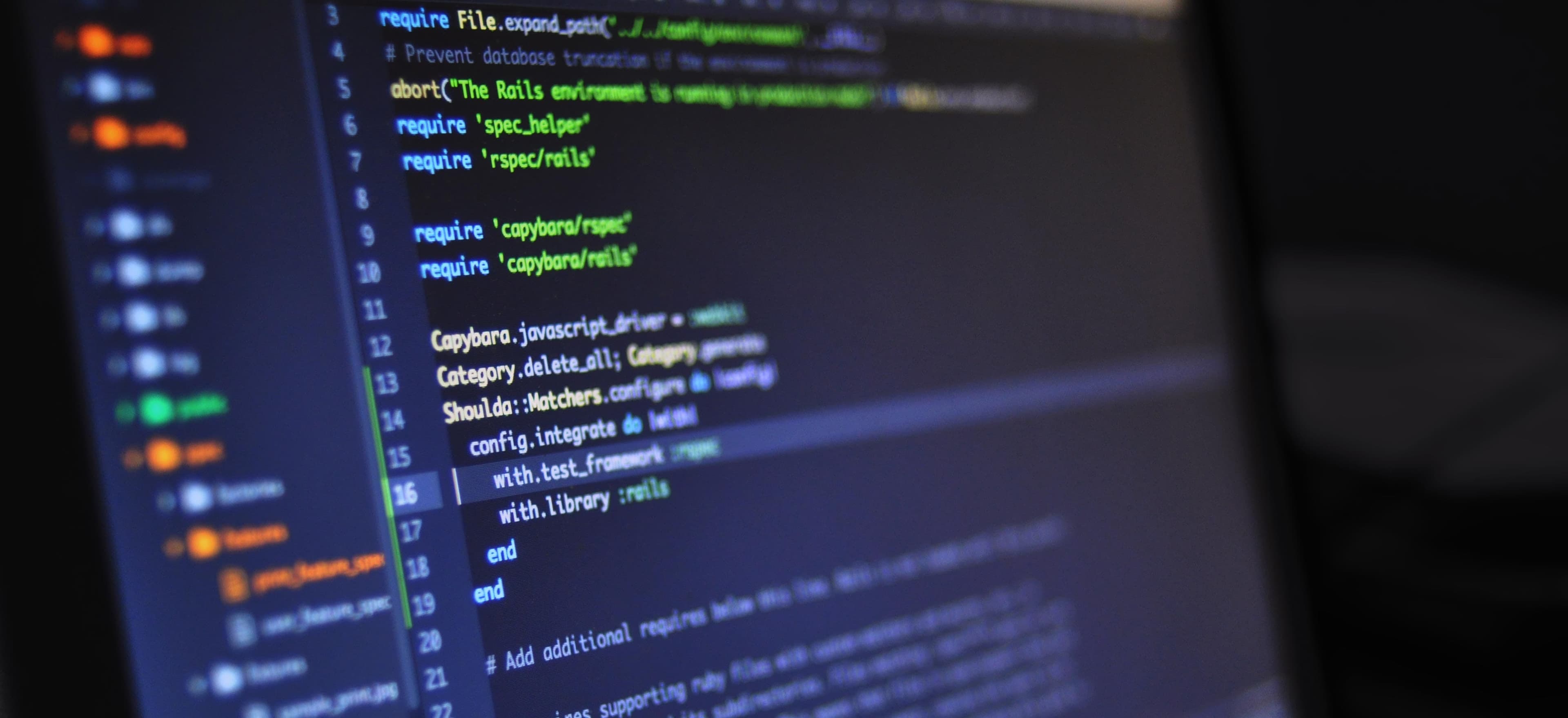
- Published on
ThreadLocal: Unraveling Its Hidden Performance Issues
Java offers various tools and features designed to enhance developer productivity and improve application performance. One of these features, ThreadLocal
, provides a way to maintain thread-specific values, facilitating concurrent programming. While ThreadLocal
can significantly improve performance in certain contexts, it comes with its pitfalls. In this post, we will untangle the complications tied to ThreadLocal
, its underlying mechanics, common use cases, and hidden performance issues that can manifest in your applications.
What Is ThreadLocal?
ThreadLocal
is a class in Java that provides thread-local variables. Each thread accessing a ThreadLocal
variable has its own, independent copy. This means that changes made by one thread do not affect the value in another thread.
Basic example of ThreadLocal usage
public class ThreadLocalExample {
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 1);
public static void main(String[] args) {
Runnable task1 = () -> {
System.out.println(Thread.currentThread().getName() + " initial: " + threadLocalValue.get());
threadLocalValue.set(10);
System.out.println(Thread.currentThread().getName() + " modified: " + threadLocalValue.get());
};
Runnable task2 = () -> {
System.out.println(Thread.currentThread().getName() + " initial: " + threadLocalValue.get());
threadLocalValue.set(20);
System.out.println(Thread.currentThread().getName() + " modified: " + threadLocalValue.get());
};
Thread thread1 = new Thread(task1);
Thread thread2 = new Thread(task2);
thread1.start();
thread2.start();
}
}
Explanation of the Code
- ThreadLocal Variable: We declare a
ThreadLocal
variable calledthreadLocalValue
, initialized to1
. - Runnable Tasks: Two tasks print the initial and modified values of
threadLocalValue
. Each thread modifies its own instance without affecting others. - Threads: We instantiate and start two threads that execute different tasks simultaneously.
Use Cases for ThreadLocal
Many scenarios benefit from using ThreadLocal
, including:
- Database Connections: To manage database connections in a thread-safe way.
- User Sessions: Storing user context during web requests.
- Transaction Management: Implementing thread-specific transactional states.
Why Use ThreadLocal?
Using ThreadLocal
can improve performance by limiting the need for synchronization. Since threads maintain their own copies of variables, you can avoid costly context switches, locking, and contention among threads, making your applications respond more quickly, especially in multi-threaded environments.
Key takeaway:
ThreadLocal
can enhance efficiency when used correctly but requires an understanding of when and where it is viable.
Hidden Performance Issues
Despite its benefits, ThreadLocal
can introduce hidden performance issues, mainly due to memory leaks and the misallocation of resources.
Memory Leaks
The most prominent issue revolves around memory leaks. When a ThreadLocal
instance is no longer needed, it needs to be properly cleaned up, as ThreadLocal
variables are stored in thread-specific maps. If the threads remain alive, they will hold references to ThreadLocal
variables, preventing garbage collection.
The Garbage Collector Problem
Java's garbage collector cannot reclaim the memory used by ThreadLocal
variables if the respective thread is alive but idle. As a result, over time, this can lead to excessive memory utilization.
Example of Memory Leak
public class MemoryLeakExample {
private static ThreadLocal<List<String>> threadLocalList = ThreadLocal.withInitial(ArrayList::new);
public static void main(String[] args) {
Runnable task = () -> {
try {
while (true) {
List<String> list = threadLocalList.get();
list.add("Some Value"); // Simulating an operation
Thread.sleep(100); // Sleep to imitate processing
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
};
Thread thread = new Thread(task);
thread.start();
}
}
Explanation of the Memory Leak Code
- The thread keeps running indefinitely and continuously adds values to a
List
managed throughThreadLocal
. - If we don't clean up or end this thread properly, it retains references to the
List
, resulting in a memory leak.
How to Mitigate Memory Leaks
A simple practice for mitigating memory leaks with ThreadLocal
is to clear the values once they are no longer required. This can be achieved by using the remove()
method.
public class CleanUpExample {
private static ThreadLocal<String> threadLocalString = ThreadLocal.withInitial(() -> "Hello");
public static void main(String[] args) {
Runnable task = () -> {
System.out.println(Thread.currentThread().getName() + ": " + threadLocalString.get());
threadLocalString.remove(); // Clean-up to prevent memory leak
};
Thread thread = new Thread(task);
thread.start();
}
}
The Cost of Maintenance
Using ThreadLocal
also involves additional code for proper management. The overhead can accumulate when you have multiple threads accessing numerous ThreadLocal
variables. This complexity can detract from the potential performance benefits.
Alternatives to ThreadLocal
In specific scenarios, consider the following alternatives to ThreadLocal
:
- Thread-Safe Collections: Use concurrent collections, which provide thread-safe access to data shared among multiple threads.
- Immutable Data: Create immutable data structures that can be easily shared among threads without modifying the state.
- Dependency Injection: Rely on frameworks (like Spring) that manage the lifecycle of components and their contexts, avoiding the pitfalls of manual memory management.
Key Takeaways
ThreadLocal
is a powerful tool designed to enhance performance in Java applications. However, it is crucial to understand its hidden performance issues, such as memory leaks and maintenance overheads, to utilize it effectively.
By following best practices like using remove()
for cleanup and considering alternatives, you can mitigate these risks. Always assess the viability of ThreadLocal
in your application's context and maintain a balance between performance gains and resource management.
For more insights on Java concurrency topics, check out Java Concurrency in Practice and Oracle's Guide to ThreadLocal.
By arming yourself with knowledge about ThreadLocal
, you can pave the way for robust and efficient Java applications. Happy coding!