Streamline Bug Tracking: Overcoming Common Team Challenges
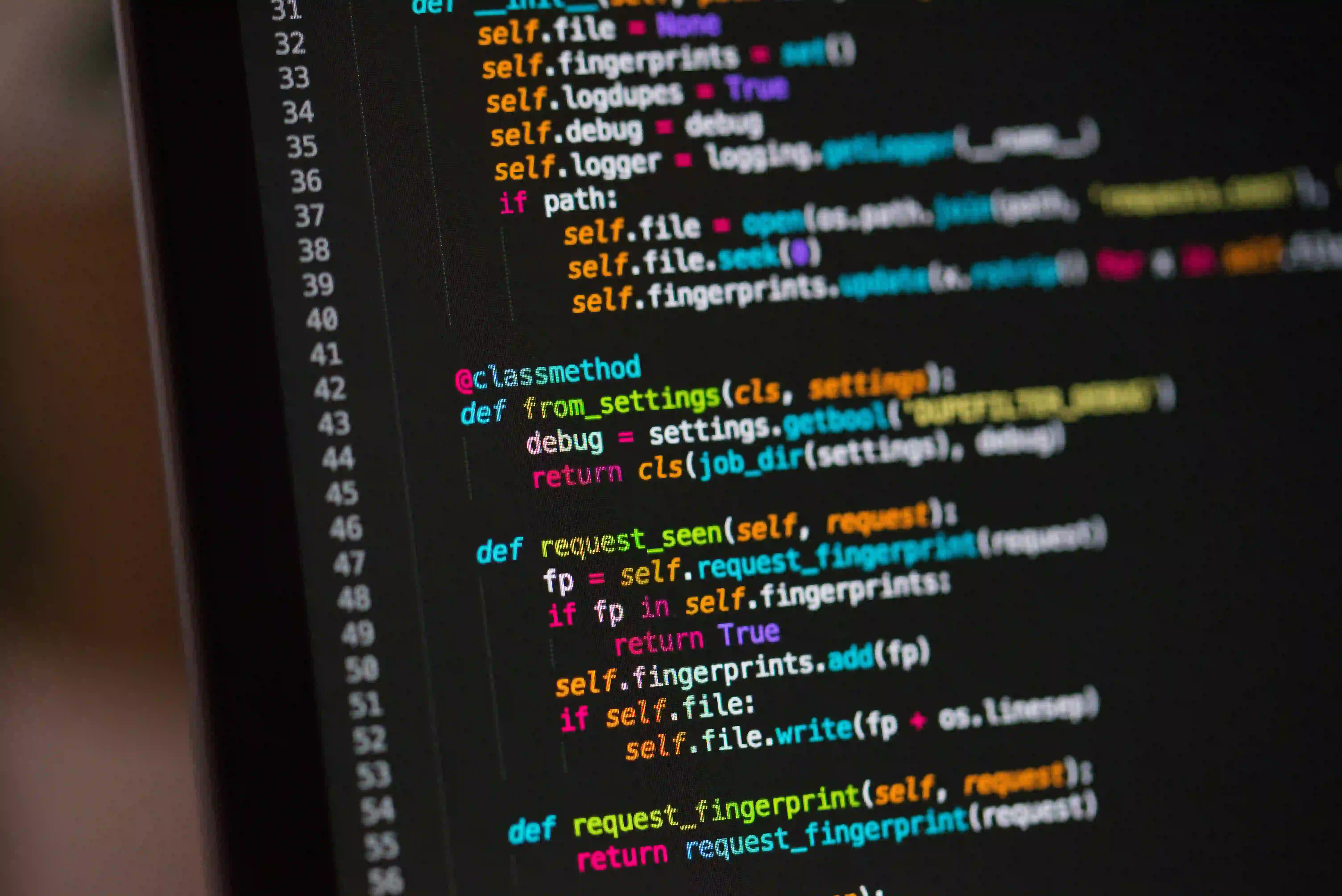
Streamline Bug Tracking: Overcoming Common Team Challenges
Bug tracking is a critical process in software development. It plays a pivotal role in ensuring that applications are free of glitches that could hinder user experience or render functionality useless. However, bug tracking can also present significant challenges for teams. In this blog post, we'll explore common challenges teams face in bug tracking and strategies to overcome them, ultimately improving efficiency and productivity.
Why Bug Tracking is Important
Before diving into the challenges, let's clarify why bug tracking is essential. A robust bug tracking system:
- Enhances Communication: It fosters better communication among team members, ensuring everyone is on the same page.
- Prioritizes Issues: Teams can prioritize which bugs to fix based on their severity and impact on the application.
- Improves Product Quality: By systematically addressing bugs, teams can deliver higher-quality products to users.
Common Challenges in Bug Tracking
1. Lack of Standardized Processes
One of the first issues many teams encounter is the absence of a standardized process for bug reporting and tracking. Without this, bugs may be reported inconsistently, making it difficult to prioritize and address them effectively.
Solution: Establish a Standardized Bug Reporting Template
Implement a standardized bug reporting template that includes critical fields such as:
- Title: A concise summary of the bug.
- Description: A detailed description including steps to reproduce.
- Severity: An assessment of how it impacts the user experience.
- Status: Current state of the bug (e.g., Open, In Progress, Closed).
Here’s an exemplary template:
## Bug Report Template
### Title:
[Write a concise title]
### Description:
[Explain the issue, ideally step-by-step]
### Steps to Reproduce:
1. [Step-by-step guide]
2. [Additional steps if necessary]
### Expected Result:
[Describe what should happen]
### Actual Result:
[Describe what actually happens]
### Severity:
[Low, Medium, High, Critical]
### Status:
[Open, In Progress, Closed]
2. Poor Communication Among Team Members
Communication breakdowns are common, especially in larger teams or when teams work in silos. This often results in duplicate bug reports or missed fixes.
Solution: Implement a Centralized Bug Tracking Tool
Using a centralized bug tracking tool can help streamline communication. Tools such as JIRA, Bugzilla, or MantisBT allow all team members to view and update bugs in real time. This creates a single source of truth that can significantly reduce miscommunications.
3. Overwhelming Volume of Bugs
As development progresses, the number of reported bugs can quickly spiral. An overwhelming volume can lead to important issues being overlooked.
Solution: Prioritize Bugs Effectively Using a Severity Matrix
Adopting a severity matrix helps teams focus on high-impact bugs first. Here's a simple code snippet that demonstrates how you might approach prioritizing bugs programmatically:
import java.util.*;
class Bug {
private String title;
private String severity;
public Bug(String title, String severity) {
this.title = title;
this.severity = severity;
}
public String getSeverity() {
return severity;
}
public String getTitle() {
return title;
}
}
public class BugTracker {
private List<Bug> bugs;
public BugTracker() {
bugs = new ArrayList<>();
}
public void addBug(Bug bug) {
bugs.add(bug);
}
public List<Bug> prioritizeBugs() {
// Sort bugs based on severity (High -> Medium -> Low)
bugs.sort(Comparator.comparing(this::getSeverityRank).reversed());
return bugs;
}
private int getSeverityRank(Bug bug) {
switch (bug.getSeverity()) {
case "High":
return 3;
case "Medium":
return 2;
case "Low":
return 1;
default:
return 0;
}
}
public static void main(String[] args) {
BugTracker tracker = new BugTracker();
tracker.addBug(new Bug("UI Crash on Load", "High"));
tracker.addBug(new Bug("Typo in Button Label", "Low"));
tracker.addBug(new Bug("Slow Performance on Login", "Medium"));
List<Bug> prioritizedBugs = tracker.prioritizeBugs();
prioritizedBugs.forEach(bug -> System.out.println("Bug Title: " + bug.getTitle()));
}
}
4. Incomplete Bug Fixes
Even when bugs are addressed, they might not be fixed completely. This typically happens when developers only focus on the symptoms rather than the underlying causes.
Solution: Conduct Root Cause Analysis (RCA)
Encouraging the team to perform a Root Cause Analysis (RCA) can help identify underlying issues. This process involves asking "why" multiple times (the “5 Whys” technique is a common approach) until the root cause is uncovered.
5. Inefficient Workflows
Many teams struggle with inefficient workflows that prolong the bug fixing process. The result is delays in delivery and frustrated stakeholders.
Solution: Automate Where Possible
Automation can significantly streamline workflows. Consider implementing:
- Automated testing: Tools like Selenium and JUnit can automate test cases, reducing human error.
- Continuous Integration (CI): CI tools like Jenkins and GitHub Actions can help integrate code changes rapidly, enabling quick detection of bugs as they arise.
6. Limited Insights and Metrics
Without proper metrics, teams may struggle to understand the effectiveness of their bug tracking processes, leading to repetitive mistakes.
Solution: Track Key Performance Indicators (KPIs)
Use KPIs such as:
- Time to Resolve: How quickly bugs are fixed.
- Number of Bugs Opened vs. Closed: A ratio to measure productivity.
- Reopened Bugs: Indicates the efficiency of fixes.
Text these metrics in a dashboard to provide real-time insights into your bug tracking efforts.
The Closing Argument
Overcoming the common challenges of bug tracking isn’t just about adopting new tools or methodologies; it's about fostering a culture of communication, collaboration, and continuous improvement. By standardizing processes, using effective tools, prioritizing issues, conducting root cause analysis, automating workflows, and tracking metrics, teams can streamline their bug tracking efforts significantly.
For further reading on this topic, you might find the following resources useful:
- Effective Bug Tracking Techniques
- Best Practices in Software Testing and QA
By embracing these strategies, you will not only improve efficiency but also enhance the overall quality of your software products. Remember, every bug fixed is one step closer to a polished, user-friendly experience. Happy bug tracking!