Maximizing Performance: Common Hashing Strategy Pitfalls
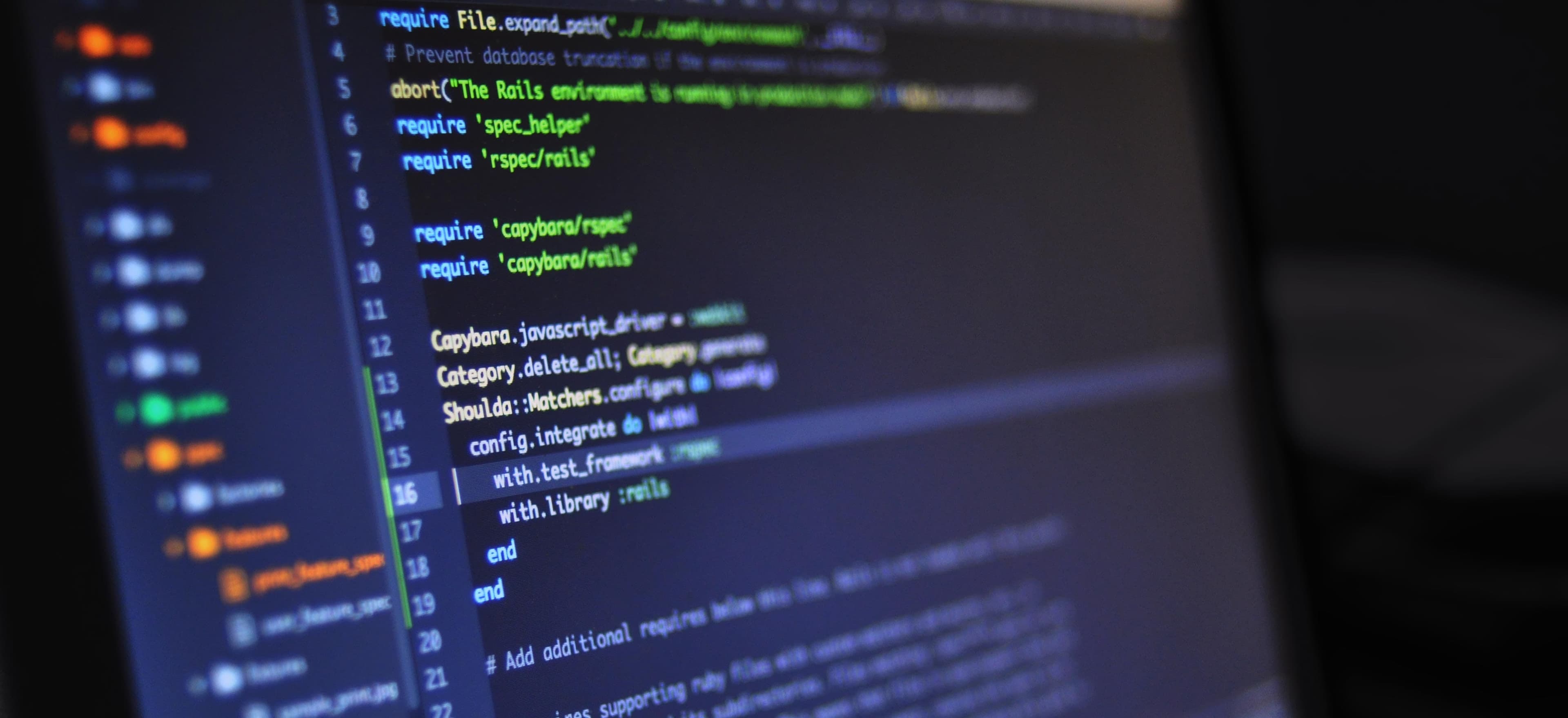
- Published on
Maximizing Performance: Common Hashing Strategy Pitfalls
Hashing is a crucial concept in computer science and software engineering, especially when dealing with data retrieval, storage, and performance optimization. However, many developers stumble over common pitfalls associated with hashing strategies. In this blog post, we will explore these pitfalls, illustrating with Java examples, and provide best practices to help maximize performance.
Understanding Hashing
Before diving into common pitfalls, let's quickly revisit what hashing is. A hash function transforms an input (or 'key') into a fixed-size string of bytes. The output is typically in a compact numerical format, allowing for efficient data manipulation and retrieval.
Why Hashing?
Hashing contributes significantly to performance in various operations, such as:
- Quick data lookup: Allows almost instantaneous data retrieval from structures like hash tables.
- Data integrity verification: Ensures that data has not been altered during transmission.
- Efficient storage: Reduces data size for faster storage and retrieval.
Pitfall 1: Poor Hash Function Design
The choice of a hash function is critical. A poorly designed hash function can lead to an uneven distribution of hashed values, causing collisions. A collision occurs when multiple inputs produce the same hash output.
Code Example
Here's a simplistic hash function that demonstrates this pitfall:
public int simpleHash(String key) {
return key.length() % 10;
}
Why This is Poor Design: This function only considers the length of the string, meaning different strings of the same length will collide. For instance:
- "abc" and "xyz" both hash to 3.
- "hello" and "world" both hash to 5.
Best Practice
A good hash function should:
- Use all bits of the input.
- Distribute hashed values uniformly across the hash space.
Consider using built-in libraries, such as java.util.Objects
, which provide robust implementations.
public int improvedHash(String key) {
return Objects.hash(key);
}
The Last Word
Investing time in selecting or designing an effective hash function is essential for maintaining performance and reducing collision occurrences.
Pitfall 2: Ignoring Load Factor
Load factor refers to the ratio of the number of entries in a hash table to the number of slots in the table. A high load factor can deteriorate performance due to increased collisions, which often lead to clustering.
Code Example
Here's an example of a hash table implementation that doesn't consider load factor:
public class SimpleHashTable {
private int size = 10;
private List<String>[] table;
public SimpleHashTable() {
table = new List[size];
for (int i = 0; i < size; i++) {
table[i] = new ArrayList<>();
}
}
public void put(String key) {
int index = improvedHash(key) % size; // Using improved hash function
table[index].add(key);
}
}
Why This Matters: If you are consistently adding elements without resizing the hash table, your load factor will increase and retrieval times will slow significantly.
Best Practice
Implement resizing logic in your hash table. For example, double the size of the array when the load factor exceeds 0.75.
private void resize() {
int newSize = size * 2;
List<String>[] newTable = new List[newSize];
for (int i = 0; i < newSize; i++) {
newTable[i] = new ArrayList<>();
}
for (List<String> bucket : table) {
for (String key : bucket) {
int newIndex = improvedHash(key) % newSize;
newTable[newIndex].add(key);
}
}
size = newSize;
table = newTable;
}
The Last Word
Monitor your load factor actively and resize your hash table as necessary. This practice significantly enhances performance, especially with dynamic datasets.
Pitfall 3: Static vs. Dynamic Arrays
When implementing a hash table, using a static array can be limiting. Once the array fills up, the only option is to resize and rehash. This operation can be costly in terms of performance.
Code Example
Consider this initial implementation with a static array:
private List<String>[] table;
public SimpleHashTable(int initialSize) {
table = new List[initialSize];
}
Why This is Problematic: Once the array is at capacity, you are constrained until you perform the costly resizing operation.
Best Practice
Utilize a dynamic array, such as ArrayList
, that automatically resizes as needed without requiring extensive manual intervention.
public class DynamicHashTable {
private List<String> table;
public DynamicHashTable() {
table = new ArrayList<>();
}
}
The Last Word
Dynamic arrays simplify management, reduce the risk of capacity issues, and inherently improve performance.
Pitfall 4: Not Handling Null Inputs
Many developers neglect to handle null
inputs when implementing a hash function. This can lead to NullPointerException
and cause abrupt application failures.
Code Example
Here is a hash function that doesn’t account for null:
public int unsafeHash(String key) {
return key.hashCode() % size;
}
Why This is Dangerous: Calling hashCode()
on a null object will throw an exception.
Best Practice
Always check for null inputs and handle them gracefully.
public int safeHash(String key) {
return key == null ? 0 : key.hashCode() % size;
}
The Last Word
It is important to ensure that your hash functions can handle null values to maintain application stability.
Pitfall 5: Neglecting Thread Safety
In a multi-threaded environment, handling shared hash tables can introduce concurrency issues. Without proper synchronization, data inconsistency may arise.
Code Example
Here’s how a basic hash table may look without thread safety:
public void put(String key) {
int index = improvedHash(key) % size;
table[index].add(key);
}
Why This is Risky: If multiple threads access put()
simultaneously, they could collide while modifying the same bucket list.
Best Practice
Utilize synchronization techniques like synchronized
methods or ReentrantLock
for managing access.
public synchronized void put(String key) {
int index = improvedHash(key) % size;
table[index].add(key);
}
The Last Word
Implementing thread safety in your hash table designs is essential to prevent data inconsistencies and ensure robust performance.
The Last Word
Hashing is an essential tool for optimizing program performance. However, it is easy to fall into pitfalls that can degrade efficiency and scalability. By understanding the impacts of hash function design, load factors, data structure choices, and concurrency control, developers can enhance their hashing strategies.
For further reading on hashing strategies, check out the following resources:
By adhering to best practices and avoiding common pitfalls, you can ensure high performance in your applications that rely on efficient data handling.