Overcoming Common Deployment Issues with Tomcat and JSF
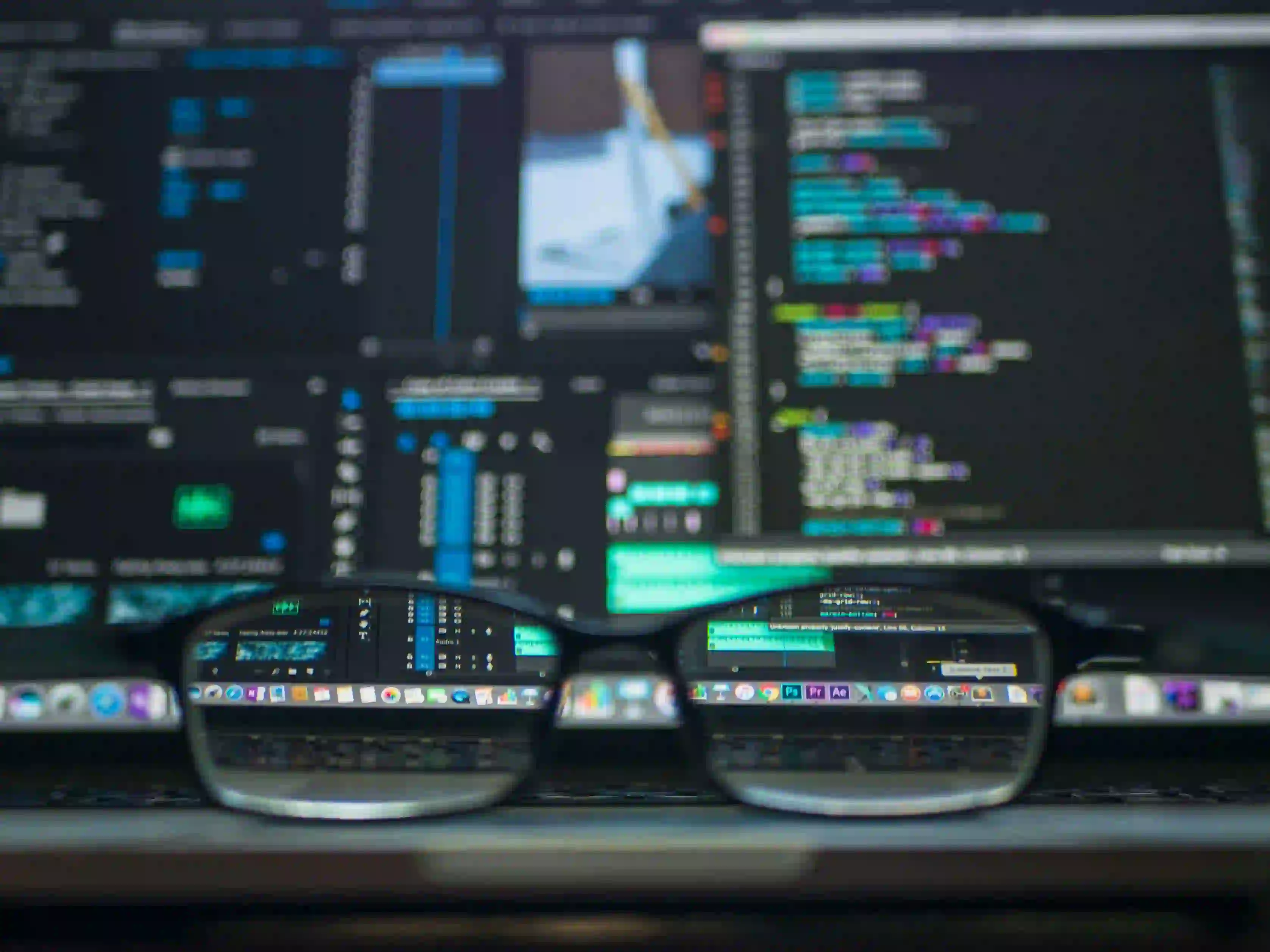
Overcoming Common Deployment Issues with Tomcat and JSF
When working with Java-based applications, the deployment process can sometimes feel like stumbling through a maze. Among the various frameworks and servers, Apache Tomcat and JavaServer Faces (JSF) stand out as popular choices. However, their integration may lead to some common deployment issues that developers must navigate. In this blog post, we’ll discuss these deployment challenges and how to overcome them effectively.
Understanding Tomcat and JSF
Before we dive into solutions, let’s briefly examine what Tomcat and JSF are.
Tomcat is an open-source implementation of the Java Servlet, JavaServer Pages (JSP), and Java Expression Language technologies. It serves as a lightweight server and is a popular choice for Java applications.
JavaServer Faces (JSF) is a Java specification for building component-based user interfaces for web applications. It simplifies UI development by enabling developers to work with reusable UI components.
Essential Configuration Steps
When deploying an application that uses Tomcat and JSF, there are a few essential configuration steps to keep in mind. Proper configuration avoids a slew of common deployment pitfalls.
-
Install Tomcat: Ensure you have the latest version of Tomcat installed. You can download the server from the Apache Tomcat website.
-
Set Up Your Project Structure: A typical Maven project structure may look like this:
my-jsf-app/
|-- src/
| |-- main/
| |-- java/
| |-- resources/
| |-- webapp/
| |-- WEB-INF/
| |-- web.xml
|-- pom.xml
- Dependency Management with Maven: Utilize a
pom.xml
file to manage your project’s dependencies. For JSF, you might include:
<dependency>
<groupId>javax.faces</groupId>
<artifactId>javax.faces-api</artifactId>
<version>2.3</version>
<scope>provided</scope>
</dependency>
Common Deployment Issues
Now that we have the basics down, let's address some common deployment issues.
1. Class Not Found Exception
One of the most frequent errors is the ClassNotFoundException
. This indicates that a required class cannot be found at runtime.
Solution: Verify your dependency management in pom.xml
. Make sure all required libraries are included and that Maven has pulled them correctly, particularly those for JSF. Here’s how you can check if your dependencies are in place:
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.faces</artifactId>
<version>2.3.10</version>
</dependency>
Another common pitfall comes from incorrect packaging. If dependencies are marked scope provided
, they will not be packaged in the WAR file. Ensure that libraries not provided by the server are included.
2. Incorrect Faces Configuration
Often, developers face issues related to the JSF configuration not being recognized. This typically leads to the inability to resolve JSF pages.
Solution: Check that your web.xml
includes the necessary faces servlet configuration and mapping:
<servlet>
<servlet-name>FacesServlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>FacesServlet</servlet-name>
<url-pattern>*.faces</url-pattern>
</servlet-mapping>
Tips for Effective Deployment
Now, let’s look at some best practices that can help ensure smooth deployment.
-
Testing Locally: Before pushing to production, always test your application locally on Tomcat. Use
localhost:8080/my-jsf-app
to check for any visible issues. -
Logging and Error Handling: Set up logging in your application to capture any application-level errors. Utilizing libraries like SLF4J with Logback or Log4j can be beneficial for debugging.
-
Directory Structure: Follow standard directory and naming conventions. A well-structured project saves a lot of time during deployment and troubleshooting.
-
Tuning Tomcat: Adjust Tomcat’s
server.xml
orcontext.xml
files as needed. For example, tweaking thread pool settings can significantly improve performance.
Additional Resources
If you are looking to dive deeper into Tomcat and JSF, the following resources can be tremendously helpful:
To Wrap Things Up
Deployment issues can be frustrating, especially when they occur in complex environments such as those built with Tomcat and JSF. However, understanding common problems and their solutions can empower developers to streamline the deployment process efficiently.
With careful attention to detail, a solid understanding of configuration, and an emphasis on testing, you can navigate these deployment challenges successfully. Next time you’re deploying a JSF application on Tomcat, keep these insights in mind to enhance your experience and ensure a smooth rollout.