Common Pitfalls in Using Custom Java Collectors
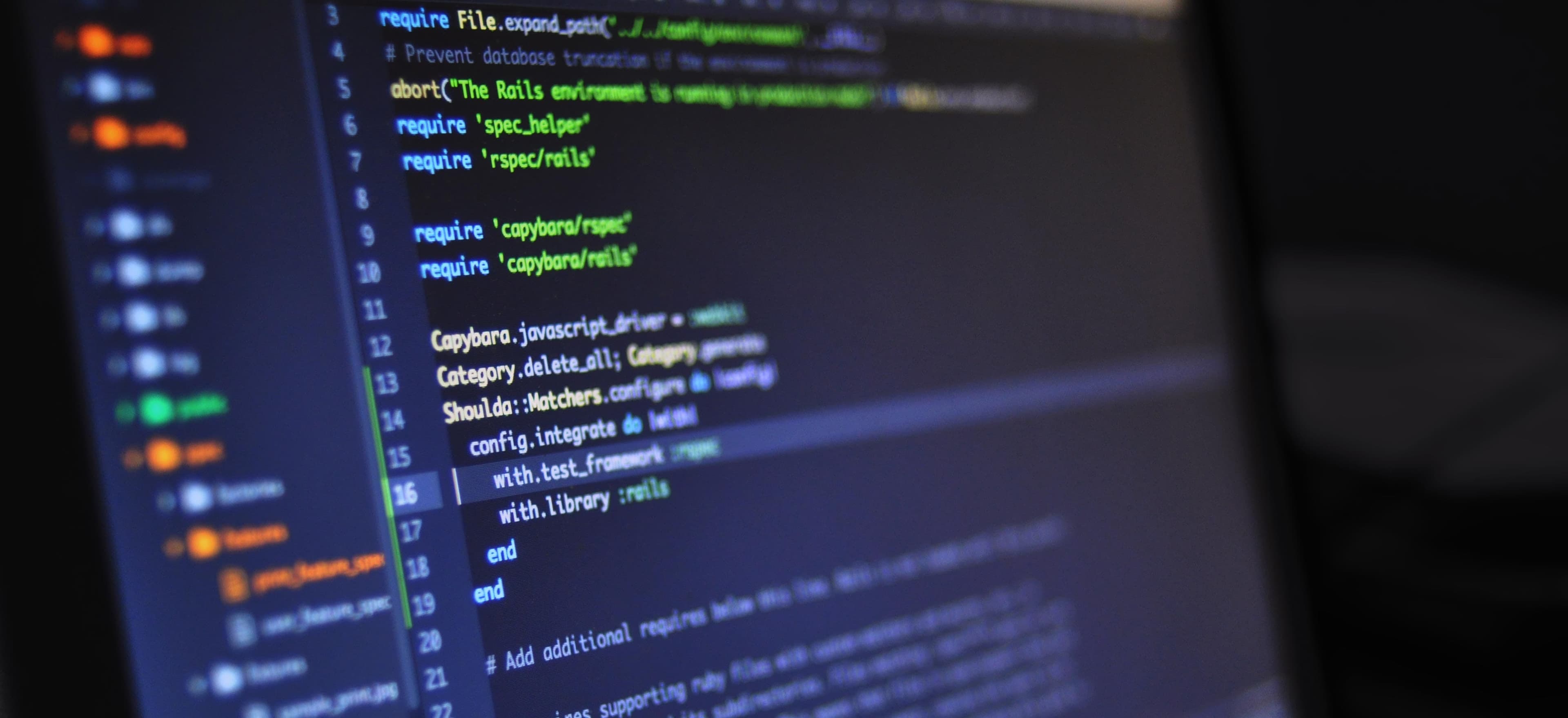
- Published on
Common Pitfalls in Using Custom Java Collectors
Java's Stream API has revolutionized the way we process collections of data, introducing a functional style of programming to the language. One of the powerful features of the Stream API is the ability to create custom collectors. However, this power comes with its own set of challenges and pitfalls. In this blog post, we will explore common mistakes that developers make when implementing custom collectors in Java, along with best practices to ensure your code is both efficient and effective.
What is a Collector?
Before diving into the pitfalls, it's essential to understand what a collector is. A Collector
in Java is an interface that builds a result based on the elements of a stream. The Collector
interface provides methods to transform data structures in a flexible and efficient manner. Here is a basic example of using a built-in collector:
import java.util.List;
import java.util.stream.Collectors;
public class BasicCollectorExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
String result = names.stream().collect(Collectors.joining(", "));
System.out.println(result); // Output: Alice, Bob, Charlie
}
}
The above snippet shows the use of the joining
collector to concatenate strings. Now let's dive into the pitfalls of creating custom collectors.
Common Pitfalls
1. Not Implementing the supplier
Correctly
One of the critical components of a custom collector is the supplier
, which provides an initial container for the results. A common mistake is to have side effects in the supplier.
Example of a Mistake
import java.util.stream.Collector;
import java.util.ArrayList;
public class IncorrectCollector {
public static Collector<String, ArrayList<String>, ArrayList<String>> toArrayList() {
return Collector.of(ArrayList::new,
(list, item) -> list.add(item), // Side effects
(left, right) -> {
left.addAll(right);
return left;
});
}
}
Commentary
In the above example, while the supplier
method is correctly returning a new ArrayList
, the side effect occurs in the accumulator method. The accumulator shouldn't have effects outside of its operation. To resolve this, consider focusing on just returning values without side effects:
Corrected Version
public class CorrectedCollector {
public static Collector<String, List<String>, List<String>> toArrayList() {
return Collector.of(ArrayList::new,
List::add,
(left, right) -> {
left.addAll(right);
return left;
});
}
}
2. Ignoring the Characteristics of the Collector
Every collector should define its characteristics, which helps the runtime optimize its behavior. Ignoring this can lead to unexpected performance issues.
Example of Ignoring Characteristics
public class IgnoringCharacteristicsCollector {
public static Collector<String, ?, List<String>> toList() {
return Collector.of(ArrayList::new,
List::add,
List::addAll); // Missing characteristics
}
}
Commentary
Not specifying characteristics can lead to a collector being treated as unordered, which could affect performance. A simple fix is to make use of the Collector.Characteristics
:
import java.util.stream.Collector;
public class WithCharacteristics {
public static Collector<String, ?, List<String>> toList() {
return Collector.of(ArrayList::new,
List::add,
List::addAll,
Collector.Characteristics.IDENTITY_FINISH);
}
}
3. Poorly Implemented Merging Function
When dealing with parallel streams, the merging function plays an important role in combining results. A nonsensical merging function can lead to lost data or incorrect results.
Example of Pitfall
public class PoorMergingCollector {
public static Collector<String, ?, List<String>> joiningCollector() {
return Collector.of(ArrayList::new,
List::add,
(left, right) -> {
left.addAll(right);
return left;
});
}
}
Commentary
If the merging function modifies the left container when it shouldn’t, it might lead to unpredictable results when used in a parallel stream. It's advisable to return a new instance rather than modifying the left or right container.
Corrected Merging
public class CorrectMergingCollector {
public static Collector<String, ?, List<String>> joiningCollector() {
return Collector.of(ArrayList::new,
List::add,
(left, right) -> {
List<String> newList = new ArrayList<>(left);
newList.addAll(right);
return newList;
});
}
}
4. Not Handling Nulls
Ignoring potential null values in streams can result in NullPointerExceptions
. A robust collector should handle nulls gracefully.
Example of Neglected Null Handling
public class NullHandlingCollector {
public static Collector<String, ?, List<String>> safeCollector() {
return Collector.of(ArrayList::new,
(list, item) -> list.add(item),
List::addAll);
}
}
Commentary
The above collector will fail if any of the items added are null. A better approach would be to add a null check in the accumulator:
public class NullSafeCollector {
public static Collector<String, ?, List<String>> safeCollector() {
return Collector.of(ArrayList::new,
(list, item) -> {
if (item != null) list.add(item);
},
List::addAll);
}
}
5. Overly Complicated Logic
Keep custom collectors as simple as possible. Complicated logic can lead to significant maintenance overhead and can be a nightmare for debugging.
Overcomplicated Example
public class OverlyComplexCollector {
public static Collector<String, ?, List<String>> complexCollector() {
return Collector.of(ArrayList::new,
(list, item) -> {
if (item.length() > 5) {
// Complex logic...
} else {
// More complex logic...
}
},
(left, right) -> {
// Complex merging logic...
return left;
});
}
}
Commentary
Keep the logic focused. Aim for simplicity and clarity, and extract more complicated behavior into separate methods where necessary.
public class SimplerCollector {
public static Collector<String, ?, List<String>> simpleCollector() {
return Collector.of(ArrayList::new,
List::add,
List::addAll);
}
}
In Conclusion, Here is What Matters
Creating custom collectors in Java can be a powerful tool in your programming arsenal. However, it’s crucial to avoid some common pitfalls that can lead to unexpected behavior and confusion.
- Ensure
supplier
methods are side-effect-free. - Don’t ignore collector characteristics.
- Implement robust merging functions.
- Handle nulls proactively.
- Keep the logic simple.
By following the guidelines in this post, you will find that your custom collectors not only function better, but they will also be easier to maintain and understand.
For more information on Java Streams and Collectors, check out the official Java documentation. Happy coding!