Preventing Common JDBC Security Vulnerabilities
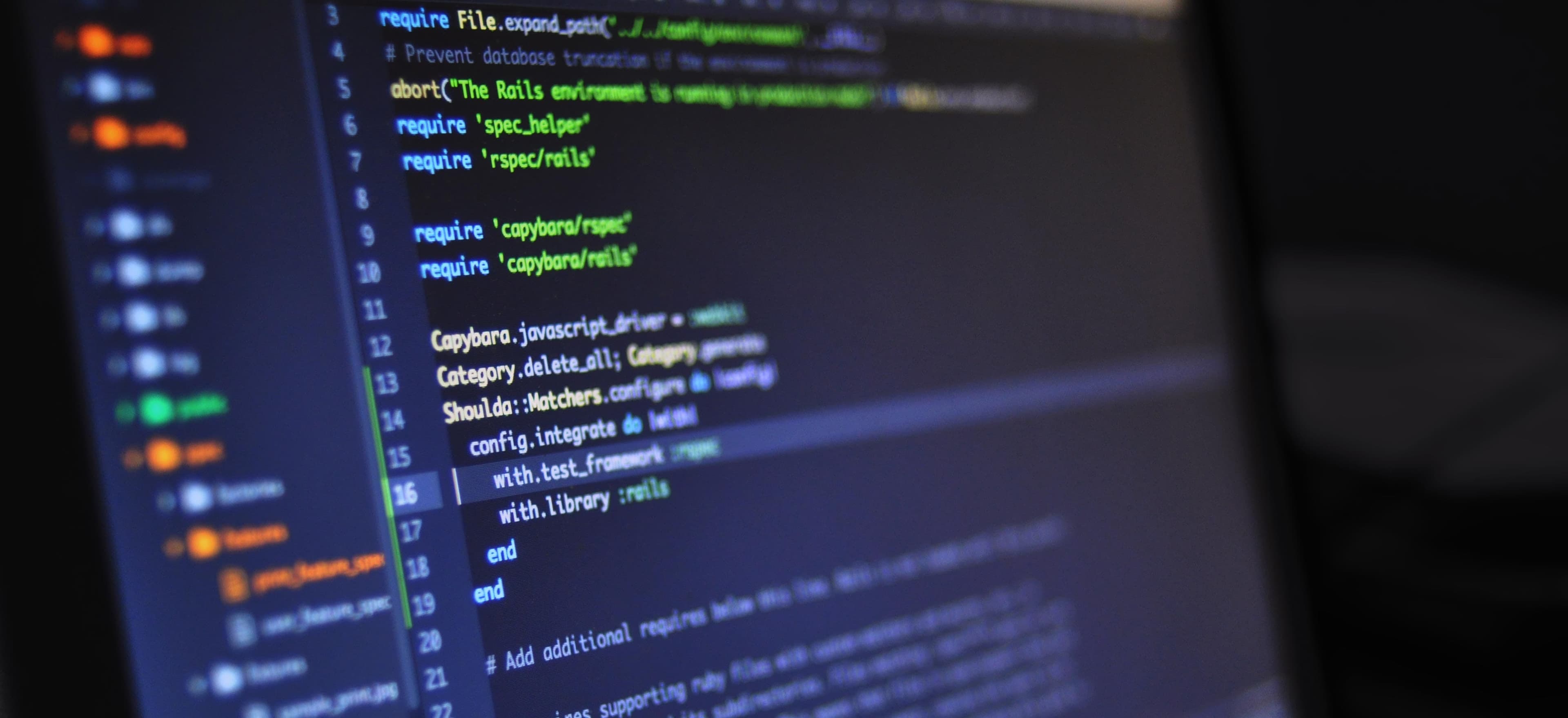
- Published on
Preventing Common JDBC Security Vulnerabilities
Java Database Connectivity (JDBC) is a powerful API that allows Java applications to interact with databases. However, with great power comes great responsibility, especially in terms of security. JDBC applications are susceptible to various vulnerabilities that can lead to serious breaches. In this post, we will explore these vulnerabilities, best practices to prevent them, and provide code snippets that illustrate how to write secure JDBC code.
Understanding JDBC Vulnerabilities
1. SQL Injection
One of the most prevalent vulnerabilities in any database application is SQL Injection. This occurs when untrusted data is included in SQL statements without adequate filtering. It can allow an attacker to execute arbitrary SQL code, potentially compromising the entire database.
Example of SQL Injection:
String userId = request.getParameter("userId");
String query = "SELECT * FROM users WHERE id = '" + userId + "'";
Statement stmt = connection.createStatement();
ResultSet rs = stmt.executeQuery(query);
In the example above, if the user enters a malicious string like 1 OR 1=1
, the query would yield all rows in the users
table.
Prevention: Use Prepared Statements
Prepared statements allow you to embed user input safely.
String userId = request.getParameter("userId");
String query = "SELECT * FROM users WHERE id = ?";
PreparedStatement pstmt = connection.prepareStatement(query);
pstmt.setString(1, userId);
ResultSet rs = pstmt.executeQuery();
Prepared statements not only prevent SQL injection but also improve performance because the SQL query is compiled once and can be reused multiple times.
2. Exposing Database Credentials
Hardcoding database credentials or exposing them in the codebase is a significant security risk. An attacker gaining access to your source code could retrieve these credentials.
Prevention: External Configuration
Store database credentials in external configuration files, environment variables, or use a secrets management tool like AWS Secrets Manager or HashiCorp Vault.
Properties properties = new Properties();
try (InputStream input = new FileInputStream("config.properties")) {
properties.load(input);
String dbUser = properties.getProperty("db.user");
String dbPassword = properties.getProperty("db.password");
String dbUrl = properties.getProperty("db.url");
connection = DriverManager.getConnection(dbUrl, dbUser, dbPassword);
}
3. Inefficient Error Handling
Poor error handling can leak sensitive information through stack traces or detailed error messages, aiding attackers in exploiting vulnerabilities.
Prevention: Generic Error Messages
Implement logging responsibly and return generic error messages to users.
try {
// Database operations
} catch (SQLException e) {
LOGGER.error("Database error occurred.");
throw new RuntimeException("A database error has occurred. Please try again later.");
}
In the example, the error message logged provides no details to the user, but it can help developers diagnose the issue.
4. Insecure Network Connections
When accessing databases over the network, insecure connections can expose sensitive data to interception.
Prevention: Use SSL/TLS
If your database supports it, always use SSL/TLS to encrypt data in transit. Most JDBC drivers allow you to specify SSL parameters in the connection URL.
String dbUrl = "jdbc:mysql://localhost:3306/mydatabase?useSSL=true&requireSSL=true";
Connection connection = DriverManager.getConnection(dbUrl, dbUser, dbPassword);
5. Lack of Proper Input Validation
Failing to validate user inputs can lead to various attacks, including buffer overflows and data corruption.
Prevention: Input Validation
Always validate input data to ensure it meets expected formats.
String userId = request.getParameter("userId");
if (!userId.matches("\\d+")) {
throw new IllegalArgumentException("Invalid user ID format.");
}
6. Insufficient Logging and Monitoring
Failing to log database operations and access attempts can leave your application vulnerable since you won’t have records to determine if a breach occurred.
Prevention: Implement Logging and Monitoring
Log all significant database operations, user access, and errors. Use monitoring tools to detect unusual patterns.
// Logging a successful login attempt
LOGGER.info("User {} logged in successfully.", userId);
// Log data changes
LOGGER.info("User {} updated their profile.", userId);
7. Inadequate Permissions
Granting users excessive database permissions can lead to misuse. A non-administrative user may gain access to administrative functions.
Prevention: Principle of Least Privilege
Limit database user permissions to the least necessary for performing their tasks. Create a user with limited rights for your application.
GRANT SELECT, INSERT, UPDATE ON mydatabase.* TO 'myappuser'@'localhost';
8. Not Closing Database Connections
Open connections can consume resources and degrade performance. They can also lead to a Denial of Service (DoS) if the maximum number of connections is reached.
Prevention: Connection Management
Always close database connections in a finally block or use try-with-resources.
try (Connection connection = DriverManager.getConnection(dbUrl, dbUser, dbPassword);
PreparedStatement pstmt = connection.prepareStatement(query)) {
// Database operations
} catch (SQLException e) {
LOGGER.error("Database operation failed.", e);
}
// Connection and PreparedStatement are automatically closed.
A Final Look
Preventing common JDBC security vulnerabilities requires a proactive approach. This includes using prepared statements, managing credentials securely, implementing strict error handling, ensuring secure connections, validating inputs, providing adequate logging, adhering to the principle of least privilege, and managing database connections efficiently.
Pursuing these best practices not only enhances the security of your application but also builds trust with your users. For more detailed security practices, consider visiting resources like the OWASP JDBC Security project.
By following the advice and code snippets shared in this post, you can significantly reduce the risks associated with JDBC vulnerabilities. A secure application is always a step towards safeguarding sensitive data and maintaining the integrity of your systems.