Common Mistakes Beginner Java Programmers Make
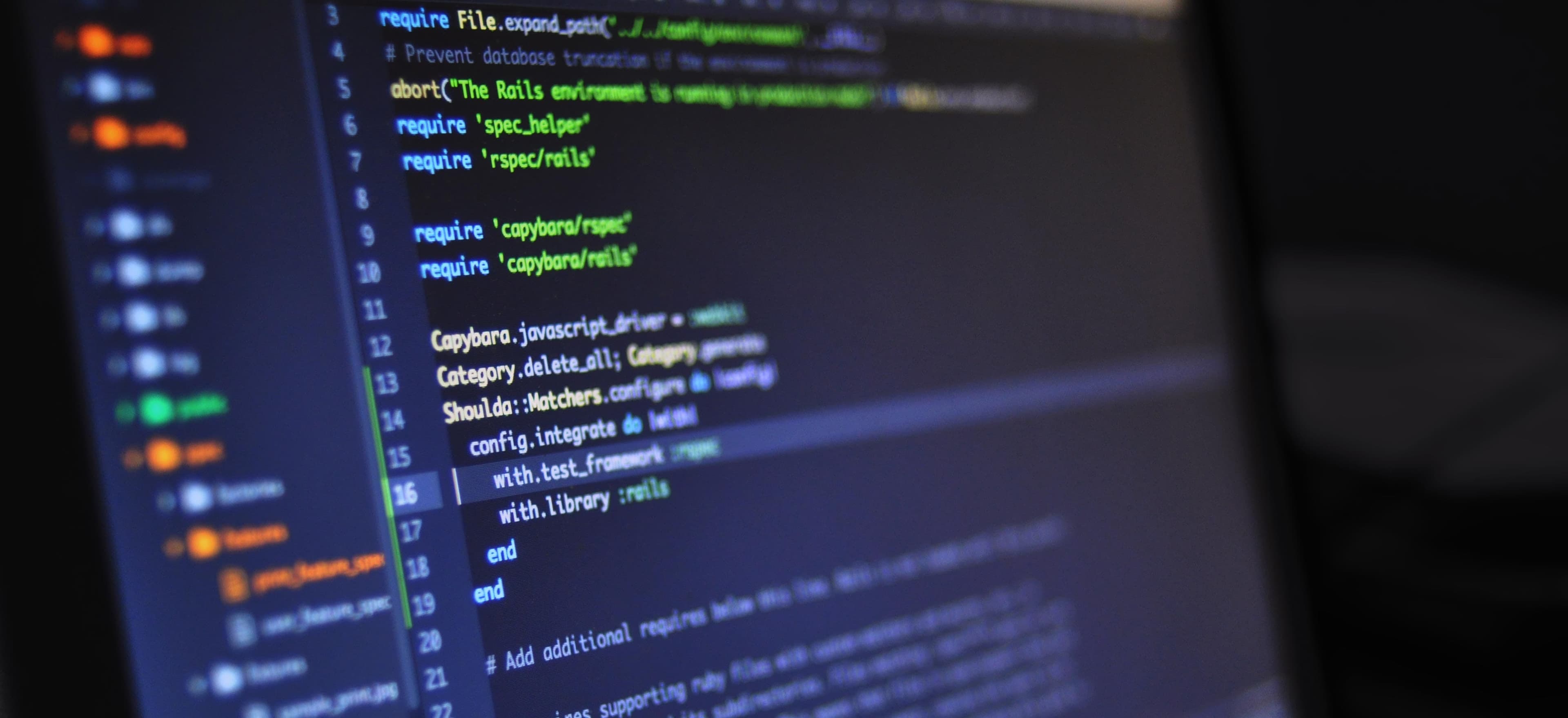
- Published on
Common Mistakes Beginner Java Programmers Make
Java is a powerful programming language that powers everything from mobile apps to large-scale enterprise systems. While it is loved for its versatility and design, beginners often stumble upon some common pitfalls that can hinder their learning process. In this blog post, we will discuss the most frequent mistakes made by novice Java programmers so that you can avoid them and improve your coding skills faster.
1. Ignoring the Basics
Why it Matters
It’s tempting for beginners to rush into complex projects, but this often results in a shaky foundation. Understanding the fundamentals of Java—such as data types, control structures, and object-oriented programming (OOP)—is crucial.
Key Concepts to Master
- Data Types: Java is a statically typed language, meaning you must define the type of data a variable will hold.
- Control Structures: Mastery of
if
,switch
,for
,while
, anddo-while
statements is essential.
Example Code Snippet
public class BasicExample {
public static void main(String[] args) {
int score = 85;
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else {
System.out.println("Grade: C");
}
}
}
Explanation
In this example, we are using an if-else
structure to determine a letter grade based on a score. Understanding how control structures work is vital for creating decision-making in your applications.
2. Overlooking Java Naming Conventions
Why it Matters
Java has specific naming conventions that enhance readability and maintainability of code. Beginners often neglect these, leading to confusion down the road.
Naming Best Practices
- Classes: Start with a capital letter (e.g.,
StudentInfo
). - Methods and variables: Start with a lowercase letter (e.g.,
calculateAverage
).
Example Code Snippet
public class Student {
private String studentName;
public Student(String name) {
this.studentName = name;
}
public void printName() {
System.out.println("Student Name: " + studentName);
}
}
Explanation
Here, we follow basic naming conventions, which help others understand the code at a glance. Naming conventions are not just stylistic choices; they can greatly affect collaboration in team environments.
3. Not Using Comments Wisely
Why it Matters
While writing code, it's common to fall into the trap of thinking that the code itself is self-explanatory. However, well-placed comments can clarify the purpose of complex sections.
Comments: A Guiding Light
- Explain why: Use comments to explain the reasoning behind the code, especially if it’s not immediately obvious.
- Avoid redundancy: Don’t comment on what is clear from the code itself.
Example Code Snippet
public class Calculator {
// Method to calculate the sum of two integers
public int add(int a, int b) {
return a + b; // Adding two numbers
}
}
Explanation
In this code, we efficiently use comments to explain the purpose of the method without cluttering the logic. Proper commenting can simplify the debugging process and improve the learning curve for others reviewing the code.
4. Misunderstanding Object-Oriented Programming
Why it Matters
Java is an object-oriented programming (OOP) language, and beginners often misuse or misunderstand the principles of OOP, such as encapsulation, inheritance, and polymorphism.
Essential OOP Concepts to Learn
- Encapsulation: Keep the data (attributes) safe within a class.
- Inheritance: Create a new class based on an existing class.
- Polymorphism: Allow methods to do different things based on the object it is acting on.
Example Code Snippet
class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
public void sound() {
System.out.println("Dog barks");
}
}
public class OOPExample {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // Outputs "Dog barks"
}
}
Explanation
In this snippet, we create a base class Animal
and a subclass Dog
that overrides the sound
method. Understanding inheritance and polymorphism is crucial for effective Java programming, especially when dealing with frameworks and APIs.
5. Neglecting Exception Handling
Why it Matters
Beginners often forget to implement proper exception handling. Java provides a robust mechanism to handle errors and exceptions, and neglecting it can lead to application crashes.
Basic Exception Handling
- Try-Catch Blocks: Use these to catch exceptions and handle them gracefully.
- Throwing Exceptions: Learn to throw exceptions for error conditions.
Example Code Snippet
public class ExceptionExample {
public void divide(int numerator, int denominator) {
try {
int result = numerator / denominator;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero");
}
}
}
Explanation
In this example, we handle an ArithmeticException
via a try-catch
block. Proper exception handling prevents your program from crashing and helps users understand errors without frustration.
6. Failing to Use Version Control
Why it Matters
One of the most crucial tools for any developer is version control, yet many beginners overlook it. This leads to lost progress and difficulty in collaborating with others.
Why Use Git?
- Tracks changes: You can see what has changed over time.
- Collaboration: It allows multiple developers to work on the same codebase.
- Easy rollback: If something goes wrong, you can revert to a previous state.
Example Command Line
git init
git add .
git commit -m "Initial commit"
Explanation
These commands initialize a Git repository, stage files, and commit your changes. Mastering Git and GitHub will significantly improve your development workflow.
The Closing Argument
As a beginner Java programmer, it’s easy to make these common mistakes, but understanding and learning from them is key to becoming a proficient developer. Focus on mastering the basics, adhering to naming conventions, utilizing comments, grasping OOP principles, handling exceptions properly, and using version control.
By avoiding these pitfalls, you will not only enhance your coding skills but also set a solid foundation for your future in software development.
For further reading, check out Java Programming Language and Effective Java for more insights into best practices in Java programming.
Happy coding!