How to Efficiently Load WebView with a Progress Bar in Android
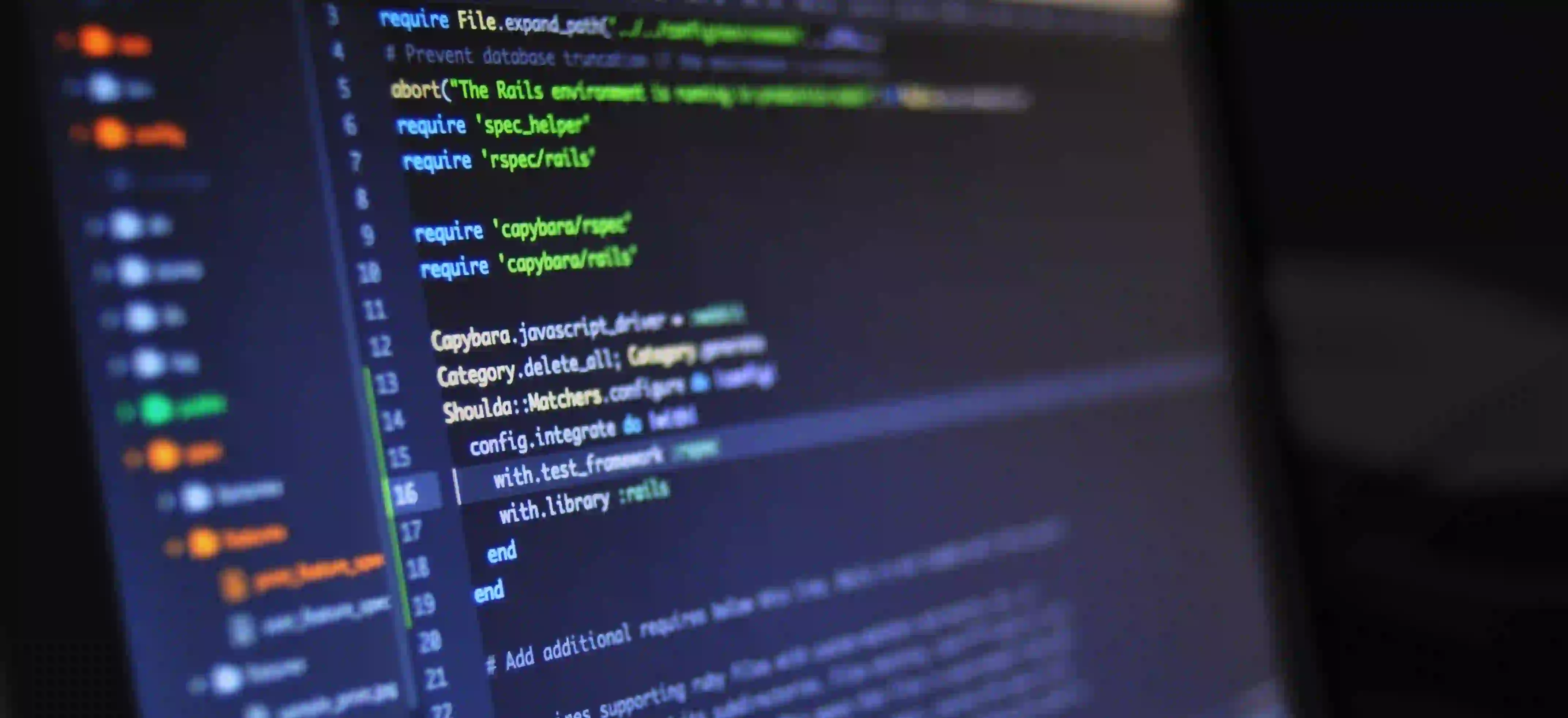
How to Efficiently Load WebView with a Progress Bar in Android
In the world of mobile development, providing a seamless user experience is key. One crucial aspect of this is how your apps handle loading content, particularly when it comes to displaying web pages. In Android, the WebView
component is frequently utilized to load web content. However, without proper handling, your users may be left staring at a blank screen, unsure of what’s happening. In this guide, we will explore how to efficiently load a WebView
with a progress bar in Android, ensuring your users are kept informed while the content loads.
Table of Contents
- What is WebView?
- Setting Up Your Project
- Creating the Layout
- Implementing the WebView and ProgressBar
- Enhancing User Experience
- Conclusion
What is WebView?
WebView
is a powerful component in Android that allows you to display web content within your app. It behaves much like a browser but is embedded within your application. This means you can load URLs, display HTML content, and even execute JavaScript. However, developers must handle loading states effectively. A well-implemented WebView
can significantly enhance user engagement.
Setting Up Your Project
To get started, you need an Android project. If you don't have one already, you can create a new project in Android Studio using the following steps:
- Open Android Studio.
- Create a new project with an Empty Activity.
- Name your project and select your preferred SDK version (Android 5.0 Lollipop or higher is ideal).
Now that you have your project set up, let’s move on to the layout.
Creating the Layout
First, we'll define a simple XML layout containing a WebView
and a ProgressBar
. The ProgressBar
will show users that the content is loading.
<!-- res/layout/activity_main.xml -->
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyleLarge"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:visibility="gone" />
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
Explanation
- ProgressBar: This widget will initially be invisible (
android:visibility="gone"
). It’ll become visible when theWebView
starts loading content. - WebView: The main component that will display the webpage.
Implementing the WebView and ProgressBar
Now, let’s implement the necessary logic to handle the loading states of the WebView
and display the ProgressBar
accordingly.
MainActivity.java
// MainActivity.java
package com.example.webviewprogress;
import android.os.Bundle;
import android.view.View;
import android.webkit.WebChromeClient;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private WebView webView;
private ProgressBar progressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
webView = findViewById(R.id.webView);
progressBar = findViewById(R.id.progressBar);
// Configure the WebView
webView.setWebViewClient(new WebViewClient());
webView.setWebChromeClient(new WebChromeClient());
// Enable JavaScript
webView.getSettings().setJavaScriptEnabled(true);
// Load URL
loadUrl("https://www.example.com");
}
private void loadUrl(String url) {
progressBar.setVisibility(View.VISIBLE); // Show progress bar
webView.loadUrl(url); // Load the requested URL
// Set a WebViewClient to listen for events
webView.setWebViewClient(new WebViewClient() {
@Override
public void onPageFinished(WebView view, String url) {
progressBar.setVisibility(View.GONE); // Hide progress bar when done loading
}
@Override
public void onPageStarted(WebView view, String url) {
progressBar.setVisibility(View.VISIBLE); // Show progress bar while loading
}
});
}
}
Explanation
- WebView and ProgressBar Initialization: We find our
WebView
andProgressBar
in the layout. - JavaScript Support:
webView.getSettings().setJavaScriptEnabled(true)
allows JavaScript execution, which is often essential for modern web pages. - Load URL and ProgressBar Management: When a URL is loaded, the progress bar becomes visible. Once the page finishes loading, the progress bar is hidden.
Enhancing User Experience
While the basic implementation is effective, here are additional strategies to further improve the user experience:
Handling Errors
Web pages may fail to load due to network issues or invalid URLs. Implementing error handling ensures users are informed about issues.
webView.setWebViewClient(new WebViewClient() {
@Override
public void onReceivedError(WebView view, WebResourceRequest request, WebResourceError error) {
progressBar.setVisibility(View.GONE);
// Display a toast or dialog to inform the user
Toast.makeText(MainActivity.this, "Unable to load webpage. Please check your internet connection.", Toast.LENGTH_SHORT).show();
}
});
Redirects and Navigation Control
Instead of letting the user leave the app for external links, you can keep them within your WebView
. Implementing the shouldOverrideUrlLoading
method allows you to handle redirects properly.
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
return true; // Indicates WebView should handle the URL
}
Bringing It All Together
In conclusion, efficiently loading a WebView
with a progress bar enhances the user experience significantly in your Android applications. By giving users feedback while content is loading, you maintain engagement and reduce frustration. With simple yet effective implementations, such as those outlined in this post, your application can navigate the landscape of web content smoothly.
To expand your knowledge on optimizing user experience, take a look at Android's official documentation on WebView and best practices for using WebView
.
Feel free to implement these strategies into your next Android project and watch your user engagement grow. If you have any questions or suggestions, don't hesitate to share in the comments below!