Securing Your Spring App: Common JWT Pitfalls to Avoid
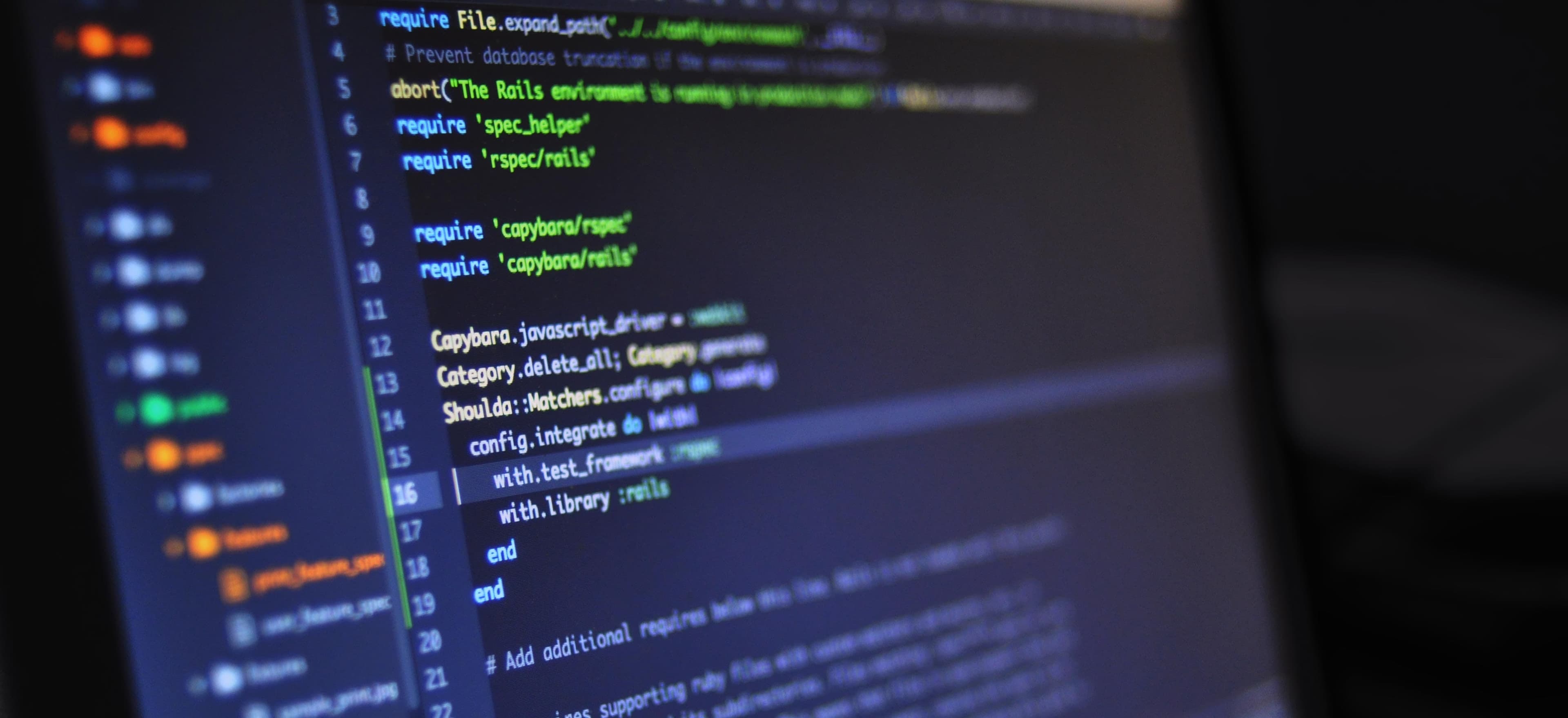
- Published on
Securing Your Spring App: Common JWT Pitfalls to Avoid
In modern web applications, security is of utmost importance. One method that has gained immense popularity for handling authentication and authorization is JSON Web Tokens (JWT). They are a compact and self-contained way to securely transmit information between parties. Spring, a widely used Java framework, offers robust support for JWT. However, while implementing JWT in your Spring applications, common pitfalls can lead to vulnerabilities. This article will guide you through these pitfalls and how to avoid them.
What is JWT and Why Use It?
Before we delve into the pitfalls, let’s clarify what JSON Web Tokens are. A JWT is a string consisting of three parts: header, payload, and signature. It enables secure transmission of information as a JSON object. The JWT's compact size makes it an ideal choice for mobile applications and is widely employed for stateless authentication.
Why use JWT?
- Stateless: No server-side sessions are required.
- Interoperability: JWTs support various programming languages, making them versatile.
- Efficiency: Compact and URL-safe, suitable for transmission in HTTP headers.
JWT Structure
A JWT is typically structured as follows:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
- Header: Contains the type of token and signing algorithm.
- Payload: Contains the claims, or information about the user and other data.
- Signature: Created by taking the encoded header and payload, a secret, and hashing them.
Common JWT Pitfalls in Spring
While JWT offers a robust solution for authentication, several pitfalls can compromise security if not careful. Below are the common pitfalls with strategies to avoid them.
1. Public Key Management
One of the most crucial aspects of JWT is the key used to sign the token. If you are using asymmetric keys, ensure proper management and security of the public-private key pair.
Avoid:
- Hardcoding key values directly in the codebase.
- Putting sensitive configurations in version control or deploying them without encryption.
Do:
Use environment variables or a secure vault service, such as HashiCorp Vault, AWS Secrets Manager, or Spring Cloud Config Server, to manage keys securely.
@Configuration
public class JwtConfig {
@Value("${jwt.secret.key}")
private String secretKey;
public String getSecretKey() {
return secretKey;
}
}
In the example above, we retrieve the secret key from the environment. This keeps the key out of the code base, reducing the risk of exposure.
2. Token Expiration Management
JWT is not meant to last forever. A common pitfall is setting a longer expiration time to avoid user re-authentication.
Avoid:
- Using overly long expiration times (like a week or a month).
- Ignoring token expiration checks.
Do:
Implement short expiration times and use refresh tokens for continuous sessions.
public String createToken(String username) {
return Jwts.builder()
.setSubject(username)
.setExpiration(new Date(System.currentTimeMillis() + JWT_EXPIRATION_TIME))
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
This code snippet ensures that the JWT includes an expiration claim (exp
). By keeping track of the expiration, you can avoid long-lived tokens which could be exploited.
3. Failing to Validate JWT
One of the most common pitfalls is neglecting the validation process of the JWT. Without proper validation, the application can accept forged tokens.
Avoid:
- Skipping checks for token expiration and signature verification.
Do:
Implement comprehensive validation checks in your authentication middleware.
public Claims validateToken(String token) {
try {
return Jwts.parser()
.setSigningKey(secretKey)
.parseClaimsJws(token)
.getBody();
} catch (JwtException e) {
throw new RuntimeException("Invalid or expired JWT");
}
}
Here, we validate the token by parsing it with the secret key. It ensures that invalid tokens are promptly rejected.
4. Information Disclosure in JWT Payload
The JWT payload can be decoded easily by anyone who has access to the token. Consequently, be cautious about the information you include in the payload.
Avoid:
- Exposing sensitive user data like passwords, social security numbers, or personal information.
Do:
Include minimal information in the payload while ensuring it suffices for authentication.
public String createToken(String username) {
return Jwts.builder()
.setSubject(username)
.claim("role", "user") // only essential claims
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
In this snippet, we intentionally include minimal information, demonstrating the principle of least privilege.
5. Weak Signing Algorithms
Another frequent oversight is not using strong signing algorithms for the token.
Avoid:
- Using none or weak algorithms (e.g., none, HS256 with a weak secret).
Do:
Always opt for strong algorithms, such as RS256, with appropriately sized keys.
public String createToken(String username) {
KeyPair keyPair = generateKeyPair();
return Jwts.builder()
.setSubject(username)
.signWith(keyPair.getPrivate(), SignatureAlgorithm.RS256)
.compact();
}
Using a public-private key pair greatly enhances security compared to using a simple shared secret.
Wrapping Up
Implementing JWT in your Spring applications can provide a powerful and efficient method of handling authentication and authorization. However, the effectiveness of JWT depends on careful management and security measures.
By avoiding the common pitfalls outlined above, you can significantly enhance the security of your application. Remember that security is an ongoing process; invest time continuously reviewing and updating your authentication mechanisms.
For further reading, check out the official Spring Security documentation on JWT here and a comprehensive guide on securing REST APIs with JWT here.
Stay safe and code secure!