Overcoming Session Loss in Tomcat Clustering: A Guide
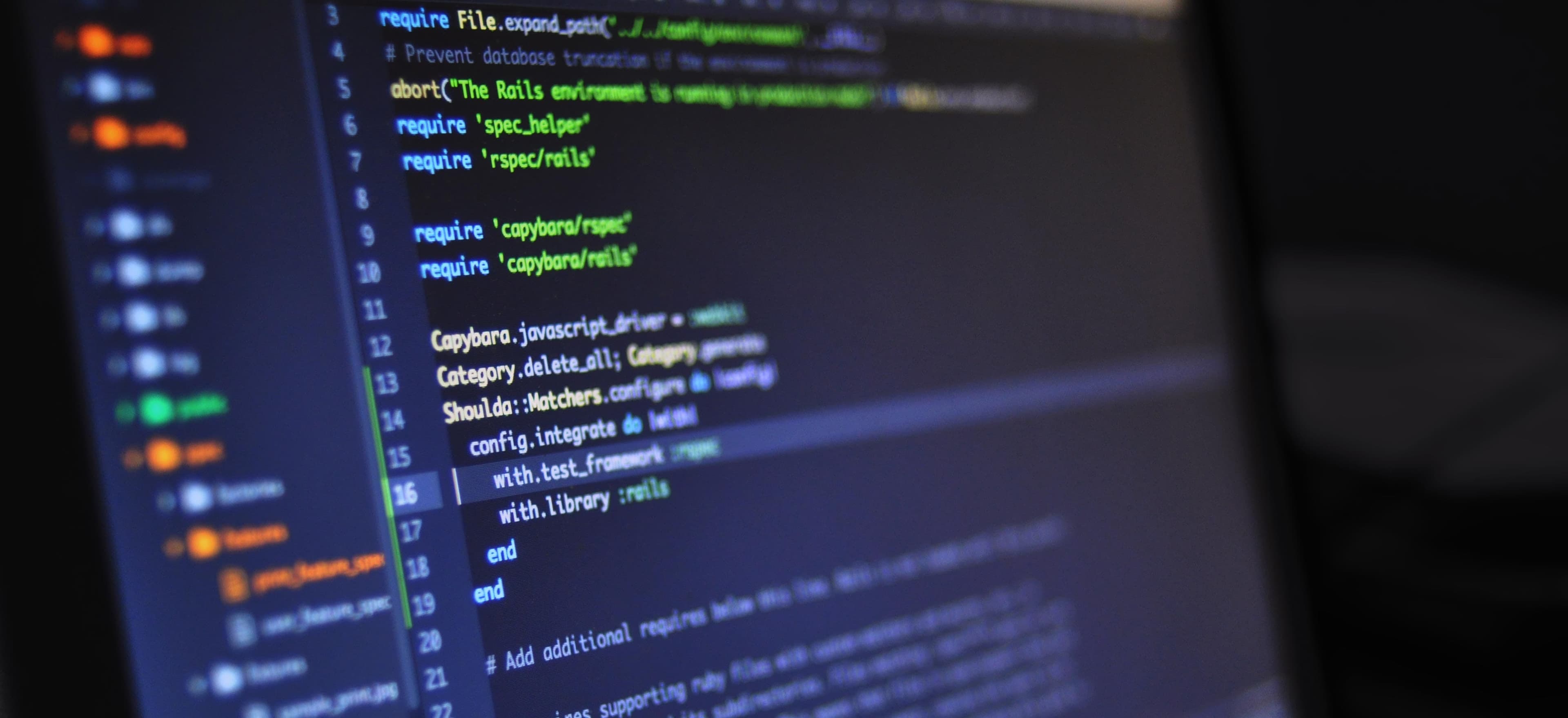
- Published on
Overcoming Session Loss in Tomcat Clustering: A Guide
Let us Begin
In today's world, web applications are expected to maintain a high level of availability and performance. As traffic increases, so does the necessity for load balancing across multiple server instances, and this is where clustering comes into play. Apache Tomcat, a widely-used web server and servlet container, provides clustering capabilities that enable applications to scale. However, session loss during server failovers or load balancing can pose a significant challenge. This guide discusses strategies for mitigating session loss in Tomcat clustering while maintaining your application's performance.
Understanding Tomcat's Clustering Architecture
Tomcat's clustering architecture enables multiple Tomcat instances to work together, allowing for session replication and failover. When a user logs in and a session is created, it can potentially be replicated across multiple nodes in a cluster. This way, if one server fails, another can take over without losing session data.
Key Features of Tomcat Clustering
- Session Replication: Sharing session data across cluster nodes.
- Failover: Transferring sessions to another active node when one goes down.
- Load Balancing: Distributing incoming requests across multiple servers.
It’s crucial to note that clustering does not guarantee complete elimination of session loss; however, it significantly mitigates the risk.
Configuring Clustering in Tomcat
To enable clustering in Tomcat, you need to configure the server.xml
and context.xml
files. Here’s how to set it up:
Step 1: Modify server.xml
Locate and edit the server.xml
file in the conf
directory. Here’s an example of adding a cluster configuration:
<Cluster className="org.apache.catalina.ha.session.DeltaManager"
expireSessionsOnShutdown="false"
expireSessionsOnShutdown="true"
notifyListenersOnReplication="true">
<Manager className="org.apache.catalina.ha.session.DeltaManager"
maxActiveSessions="-1"
minSpareSessions="5"
maxIdleSwap="100"
minIdleSwap="50"
fallbackToNonSerializable="true">
</Manager>
</Cluster>
Explanation of the Code
- DeltaManager: The manager used for session replication. It operates efficiently by requiring minimal overhead.
- expireSessionsOnShutdown: This should be set to
false
to maintain sessions if a server is stopped unexpectedly. - maxActiveSessions: Set to
-1
to allow infinite active sessions.
Step 2: Modify context.xml
Now, update the context.xml
file located in the META-INF
folder of your web application:
<Context>
<Manager className="org.apache.catalina.ha.session.DeltaManager"
sessionIdGeneratorClassName="org.apache.catalina.ha.session.SESSION_ID_GENERATOR"
replicationMode="full">
</Manager>
</Context>
Explanation of the Code
- full: This replication mode ensures that the full session is replicated across the cluster. This could mean higher resource usage but guarantees complete session integrity in the event of a node failure.
Session Serialization
When you implement session replication in a clustered environment, it’s essential to serialize the session attributes. Non-serializable objects will prevent sessions from being replicated, leading to potential session loss.
Example of Serialization
import java.io.Serializable;
public class UserSession implements Serializable {
private String username;
private String email;
public UserSession(String username, String email) {
this.username = username;
this.email = email;
}
// Getters and Setters
public String getUsername() {
return username;
}
public String getEmail() {
return email;
}
}
Why Serialization Matters
Making your session data serializable allows it to be transferred across network boundaries without loss. Always ensure that session attributes are serializable or marked as transient if necessary.
Load Balancing
Another critical aspect to consider eliminating session loss is load balancing. Tomcat can be deployed behind a load balancer capable of handling sticky sessions.
Sticky Sessions
Sticky sessions (or session affinity) ensure that a user is always redirected to the same server, minimizing the risk of session loss. Here’s a simple configuration example in your load balancer (for example, using NGINX):
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
location / {
proxy_pass http://backend;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
# Enable sticky sessions
proxy_cookie_path / "/; HTTPOnly; Secure; SameSite=Strict";
}
}
The Role of Sticky Sessions
With sticky sessions, users don't experience session-related issues, such as session timeout or data loss, especially in cases where they are redirected to different servers in a cluster.
Monitoring and Health Checks
Reliable clustering requires monitoring each node’s health. Incorporating health checks ensures that requests are only sent to healthy instances.
Configuring Health Checks in Tomcat
To configure health checks, you can set up health checks within your load balancer or use Tomcat's built-in capabilities like the Manager
app. Regular health assessments will redirect traffic only to functioning servers.
Example of a Health Check Configuration
For a Java-based application using Spring Boot:
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
@Component
public class CustomHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// Logic to check for health
boolean isHealthy = checkServiceHealth();
return isHealthy ? Health.up().build() : Health.down().build();
}
private boolean checkServiceHealth() {
// Implement health check logic
return true;
}
}
The Last Word
In summary, overcoming session loss in Tomcat clustering involves a combination of proper session management, serialization of session data, enabling sticky sessions, and continuous health monitoring of the server instances. Proper configuration of your Tomcat installation, as outlined in this guide, can significantly enhance your application's resilience and performance.
By using these strategies consistently, you can ensure that your clustered Tomcat environment maintains user sessions effectively, delivering a seamless experience to end-users. For further reading, you can explore the Apache Tomcat Documentation on clustering and session management.
Feel free to reach out with questions or for more detailed explanations on specific topics discussed here! Happy coding!
Checkout our other articles