Unpacking the Pitfalls of Java's CompletableFuture.allOf()
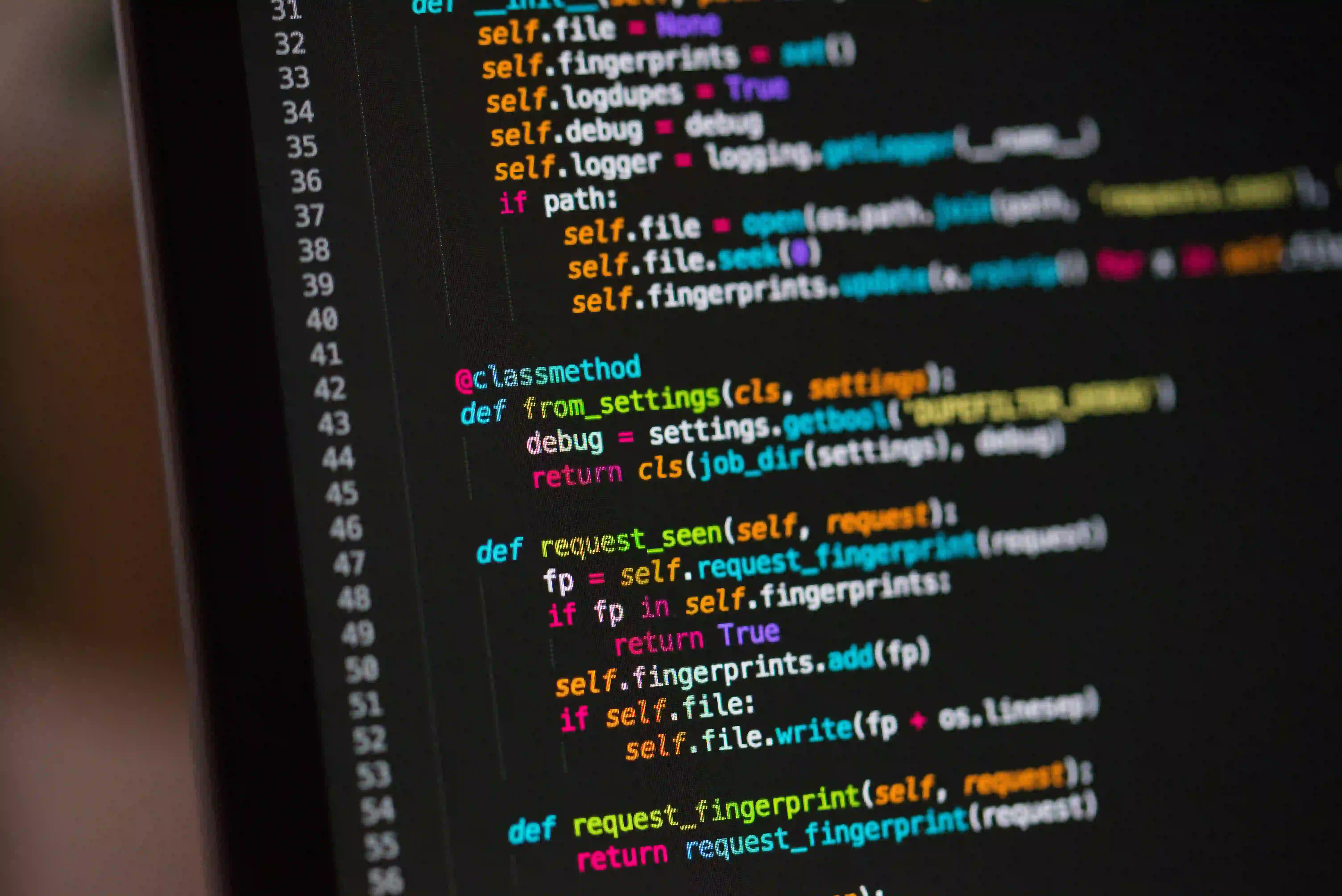
Unpacking the Pitfalls of Java's CompletableFuture.allOf()
Java's CompletableFuture
has transformed the way developers handle asynchronous programming. While it provides a powerful way to deal with future computations, not all of its features are without challenges. Particularly, the CompletableFuture.allOf()
method, which combines multiple futures into a single future, can lead to unexpected pitfalls. In this blog post, we will explore the advantages and potential downsides of using allOf()
, supported by code examples that illustrate the core concepts.
Understanding CompletableFuture.allOf()
Before delving into the pitfalls, let's clarify what CompletableFuture.allOf()
does. This method is designed to take an array of CompletableFuture
instances and return a new CompletableFuture<Void>
. This future will complete when all the futures in the supplied array have completed, be they successfully or exceptionally.
Basic Usage of allOf
Here’s a simple example to illustrate the usage of CompletableFuture.allOf()
:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
sleep(1000);
System.out.println("Task 1 completed");
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
sleep(500);
System.out.println("Task 2 completed");
});
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2);
combinedFuture.join();
System.out.println("All tasks completed");
}
private static void sleep(int milliseconds) {
try {
Thread.sleep(milliseconds);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Commentary on the Code
In this example, we create two asynchronous tasks that simulate work using Thread.sleep()
. The allOf()
method combines these tasks, and the main thread waits for their completion using the join()
method.
This example illustrates an essential use case for combining multiple tasks, but it also sets the stage for understanding some of its shortcomings.
Pitfall #1: Exception Handling
One of the most significant pitfalls when using CompletableFuture.allOf()
is how it handles exceptions. If one of the futures fails, the combined future completes exceptionally. However, it does not propagate the exceptions of individual futures.
Consider the following modification to the previous example, where the second task throws an exception:
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
sleep(1000);
System.out.println("Task 1 completed");
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
throw new RuntimeException("Task 2 failed"); // This throws an exception
});
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2);
try {
combinedFuture.join(); // This will throw an exception
} catch (CompletionException e) {
System.err.println("Error: " + e.getCause().getMessage());
}
Explanation
In this case, when future2
throws a RuntimeException
, the combined future will complete exceptionally. However, the exception from the individual future is wrapped in a CompletionException
that you need to unwrap to handle it correctly. The nuance of error handling can sometimes lead to missing errors if not addressed properly.
Pitfall #2: Mix of Result Types
CompletableFuture.allOf()
returns a CompletableFuture<Void>
, which can be confusing when combining futures that return results. If the futures return complex types, you lose the ability to easily access their results once they have completed.
Take a look at this example:
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> {
sleep(1000);
return 1;
});
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> {
sleep(500);
return 2;
});
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2);
// This does not compile because combinedFuture is of type CompletableFuture<Void>
combinedFuture.thenRun(() -> {
// Result retrieval here won't work
System.out.println("Result 1: " + future1.join());
System.out.println("Result 2: " + future2.join());
});
Commentary on the Issue
In this situation, you need to explicitly handle the result retrieval after awaiting for all futures to complete. If your codebase relies heavily on returning results from multiple futures, it may be worth using CompletableFuture.thenCombine()
instead, which allows you to operate on the results directly.
Best Practices
While CompletableFuture.allOf()
has its pitfalls, it can still be effectively used in many scenarios. Here are some best practices to bear in mind:
-
Handle Exceptions Early: Use exception handlers on each individual future before combining them. This way, you can log or recover from exceptions sooner.
☕snippet.javafuture1 = future1.exceptionally(ex -> { System.err.println("Exception in Task 1: " + ex); return null; }); future2 = future2.exceptionally(ex -> { System.err.println("Exception in Task 2: " + ex); return null; });
-
Evaluate Result Needs: If you need the results, consider alternatives like
thenCombine()
,thenCompose()
, or using anExecutor
that collects results into a structured format. -
Always Check for Completion: Before proceeding, ensure that the combined future has indeed completed. This is particularly important to avoid issues when cascading further actions.
To Wrap Things Up
CompletableFuture.allOf()
offers a powerful way to handle multiple asynchronous tasks in Java. However, its nuances require careful handling, particularly regarding exceptions and result types. By following best practices, you can mitigate many of the pitfalls and ensure that your code remains robust and understandable.
If you're interested in a deeper exploration of Java's concurrency framework, consider reading more on Java's Concurrency Utilities or exploring the official Java documentation for more insights into CompletableFutures.
Happy coding!