Common Pitfalls in Building a Java Web Framework from Scratch
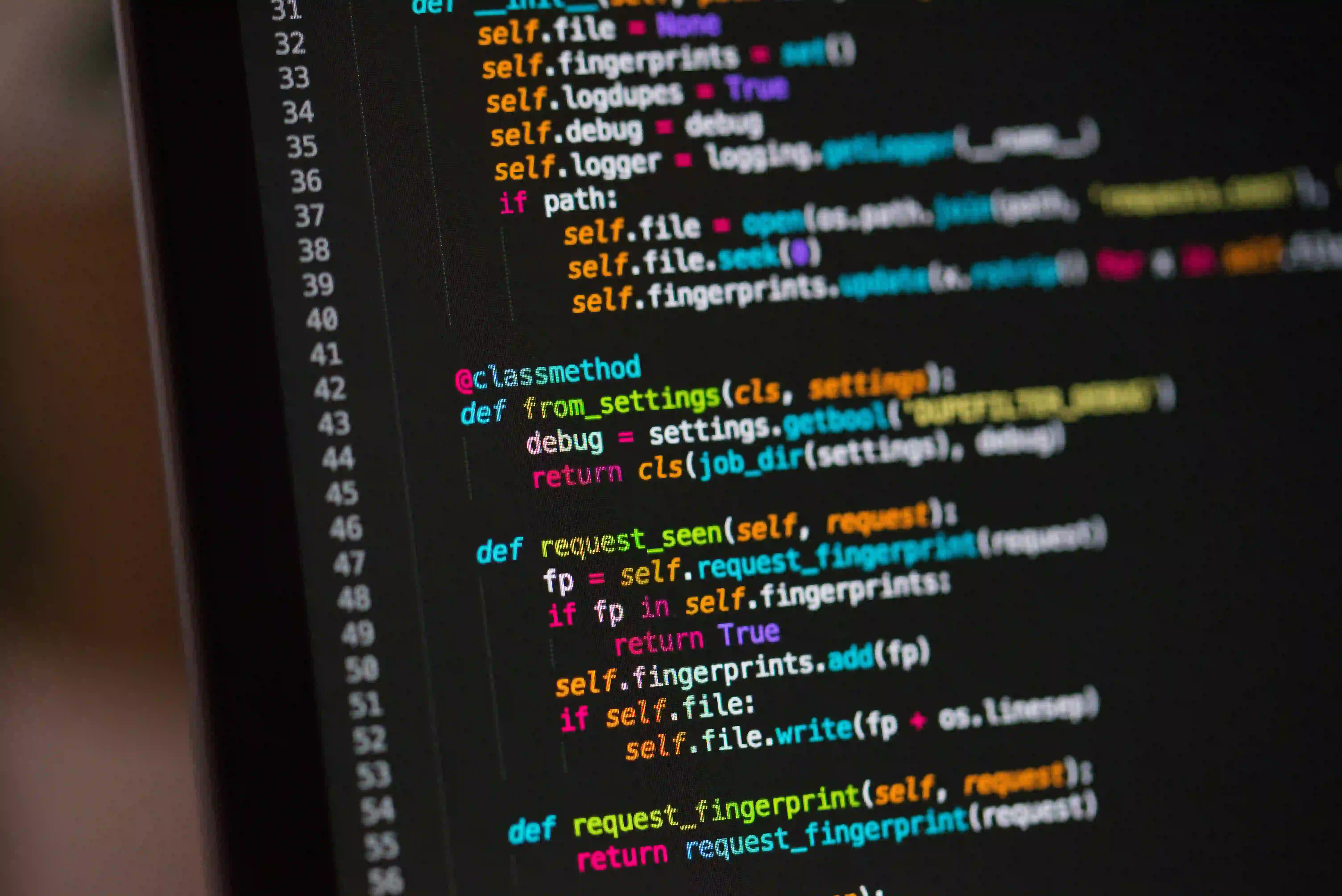
Common Pitfalls in Building a Java Web Framework from Scratch
Creating a Java web framework from the ground up can be an exhilarating project. It’s a fantastic way to deepen your understanding of web application architecture, design principles, and Java itself. However, embarking on such a venture is fraught with challenges. In this blog post, we will explore some common pitfalls developers encounter when building a Java web framework from scratch and how to avoid them.
Understanding the Scope
Before diving in, it is essential to define what a web framework is. A framework provides a foundational structure that developers can build upon. It often simplifies repetitive tasks and offers predefined ways to accomplish standard operations.
The Scope Creep Problem
One of the first pitfalls to avoid is scope creep. As you start building your framework, it's easy to get excited and want to include additional functionalities.
Consider the following best practices:
- Define Clear Objectives: Document the core functionalities your framework should provide.
- Start Small: Begin with the essential features that support the primary goals of your framework.
Example Objective
For instance, your initial goal could be to create a lightweight REST API framework that supports basic CRUD operations. As you move forward, you can iteratively add features like authentication, validation, etc.
Ignoring Design Patterns
Java is a language that thrives on robust design patterns, such as MVC, Singleton, and Factory. Neglecting to incorporate meaningful design patterns can lead to a rigid and unmanageable codebase.
The MVC Pattern
A typical structure for a web framework involves separating concerns through the Model-View-Controller (MVC) design pattern. Here's a simple breakdown:
- Model: Represents the data layer.
- View: Manages the presentation layer.
- Controller: Handles user requests and interactions.
Code Snippet: Simple MVC Structure
// Model
public class User {
private String username;
private String email;
// Getters and Setters...
}
// Controller
public class UserController {
public String createUser(User user) {
// Implementation for user creation
return "User created successfully!";
}
}
// View
public class UserView {
public void printUserDetails(String username) {
// Display user information
System.out.println("User: " + username);
}
}
In this example, separating concerns allows each component to evolve independently, enhancing maintainability.
Underestimating Configuration Management
When you have a custom-built framework, proper configuration management becomes paramount. If your framework requires extensive configuration and lacks a straightforward setup, you risk alienating users.
External Configuration
Leverage external configuration files (e.g., YAML or XML) for settings instead of hardcoding values. This approach enhances flexibility by allowing users to adapt the framework to their needs without altering the core code.
Code Snippet: Loading Configurations
import java.io.InputStream;
import java.util.Properties;
public class Configuration {
private Properties properties = new Properties();
public Configuration(String configFile) {
try (InputStream input = getClass().getClassLoader().getResourceAsStream(configFile)) {
if (input == null) {
System.out.println("Sorry, cannot find " + configFile);
return;
}
properties.load(input);
} catch (IOException ex) {
ex.printStackTrace();
}
}
public String getProperty(String key) {
return properties.getProperty(key);
}
}
This configuration management pattern allows users to specify settings in an external file, making it easier to manage changes.
Overlooking Error Handling
Unexpected errors can occur in any application. A robust error handling mechanism is crucial for a web framework's usability. Your framework should provide clear error messages and appropriate responses.
Exception Handling Strategy
Implement a centralized exception handling mechanism to manage error responses gracefully. For instance, you could create a base error handler class that all controllers can inherit.
Code Snippet: Centralized Exception Handler
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ErrorHandler {
public void handleException(HttpServletRequest request, HttpServletResponse response, Exception e) {
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
response.setContentType("application/json");
response.getWriter().write("{\"error\": \"" + e.getMessage() + "\"}");
}
}
This central error handler ensures that all exceptions are caught in a consistent manner, providing users with necessary feedback without exposing internal application details.
Not Considering Security
The task of building a web framework comes with the responsibility of ensuring its security. Neglecting security considerations can make your framework vulnerable to attacks such as SQL Injection or Cross-Site Scripting (XSS).
Implementing Security Best Practices
- Input Validation: Always validate user input.
- Use Secure Libraries: Employ libraries that provide secure functionalities rather than building everything from scratch.
Here’s an example of input validation code:
public boolean isValidInput(String input) {
return input != null && !input.trim().isEmpty() && input.matches("[A-Za-z0-9]+");
}
This method ensures that the input doesn't contain unintended characters, thus reducing risks.
Poor Documentation and Community Support
Documentation is the lifeblood of any framework. If your framework lacks adequate documentation, it will become less appealing to developers. Additionally, fostering a community around your work can enhance its adoption.
Best Practices for Documentation
- Clear API Specs: Provide comprehensive API documentation, preferably with annotations directly in your code.
- Example Projects: Include examples that developers can run to understand the framework better.
Utilize tools such as Javadocs to automatically generate documentation from your comments.
The Last Word
Building a Java web framework from scratch challenges every aspect of your programming expertise. However, by avoiding common pitfalls – such as creeping scope, design pattern neglect, configuration mismanagement, inadequate error handling, insufficient security, and poor documentation – you set the stage for a successful framework launch.
By adhering to best practices, illustrating clear designs, and maintaining thoughtful documentation, you can create a framework that not only meets your expectations but also those of its users. Remember, the goal is to build a robust, clean, and maintainable framework that maximizes productivity for developers.
Recommended Resources
- Design Patterns in Java
- Spring Framework Documentation
- Effective Java by Joshua Bloch
By learning from the experiences of others, you can craft a more effective framework while elevating your programming skills in the process. So, gear up and start building your dream framework!