Common AJAX Pitfalls in Spring MVC REST Calls
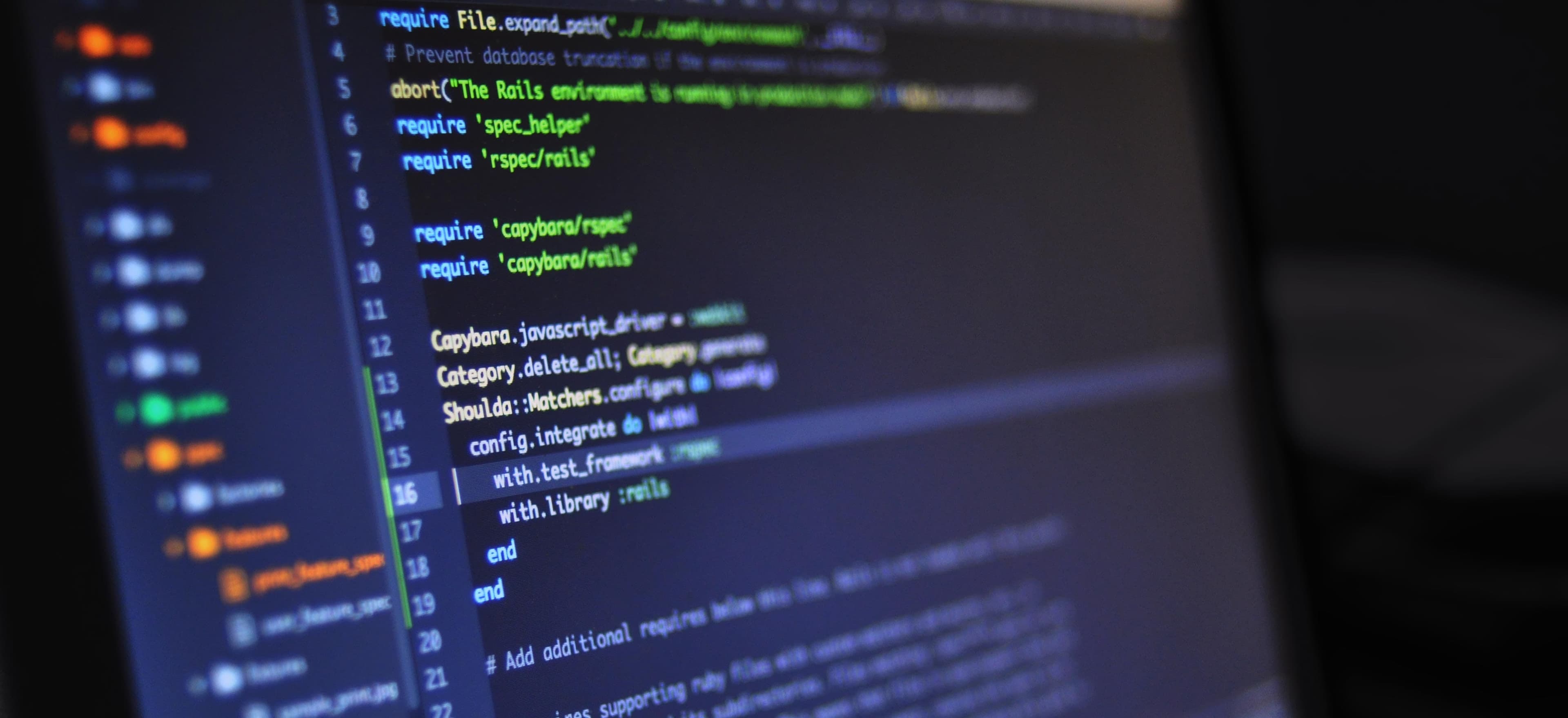
- Published on
Common AJAX Pitfalls in Spring MVC REST Calls
As web developers, we often use AJAX to create dynamic web applications that provide a smooth user experience. In a Spring MVC environment, integrating AJAX with RESTful services can elevate our applications to the next level, facilitating seamless data exchange. However, there are common pitfalls that developers face when implementing these features. In this blog post, we'll explore these pitfalls and their solutions, ensuring you can leverage AJAX effectively in your Spring MVC applications.
Understanding AJAX and Spring MVC
AJAX (Asynchronous JavaScript and XML) allows web applications to send and receive data asynchronously, updating parts of a web page without requiring a full reload. Spring MVC, part of the Spring framework, provides features for backend processing. The combination of AJAX with Spring MVC creates an efficient and responsive user experience.
Setting the Stage with Spring MVC
Before diving into the pitfalls, let's establish a basic Spring MVC REST setup. Here's a simple REST Controller:
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getUsers() {
return userService.getAllUsers();
}
}
In this controller, we have defined a getUsers
endpoint that returns a list of users. We will explore how to connect this endpoint with AJAX calls.
Common AJAX Pitfalls
1. CORS Issues
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to restrict web pages from making requests to a different domain than the one that served the web page. If your AJAX calls are directed to a different domain (including ports), you may get CORS errors.
Solution
You can solve CORS issues in your Spring MVC application by adding the @CrossOrigin
annotation to your controller. For example:
@CrossOrigin(origins = "http://localhost:3000") // Replace with your frontend domain.
@RestController
@RequestMapping("/api")
public class UserController {
//...
}
Alternatively, you can configure global CORS settings in your Spring configuration.
2. Handling Asynchronous Responses
One common pitfall is not handling the asynchronous nature of AJAX calls appropriately. If you send an AJAX request but don't wait for the response properly, you may end up with unexpected results.
Example
Let's look at a simple.ajax request.
$.ajax({
url: '/api/users',
method: 'GET',
success: function(data) {
console.log(data);
},
error: function(xhr, status, error) {
console.error('Error fetching users:', error);
}
});
In this code, if we assume data
has the user information, it's vital to ensure the success
callback handles the response correctly. If you try to manipulate the DOM before the data is returned, you can introduce bugs.
Solution
Always ensure your DOM manipulation logic is within the success
callback. This guarantees that your application reacts with the right data.
3. JSON Parsing Errors
Another common issue arises from JSON parsing. AJAX usually expects responses in JSON format. If your Spring MVC method returns data in a different format, you can encounter parsing errors.
Example
Ensure your response is correctly mapped to JSON using @ResponseBody
or, in a REST controller, simply using @RestController
implicitly does this.
@GetMapping("/users")
public @ResponseBody List<User> getUsers() {
return userService.getAllUsers();
}
Solution
Ensure that your response data is serialized properly and that the content type is set to application/json in the response header. Spring does this automatically for you when you use the right annotations.
4. Error Handling
Neglecting proper error handling in both the Spring backend and the AJAX frontend can lead to a poor user experience. Users can be left confused when something goes wrong without proper indication.
Example
In your AJAX call, it's essential to have an error handling mechanism:
$.ajax({
url: '/api/users',
method: 'GET',
success: function(data) {
// Handle success
},
error: function(xhr, status, error) {
alert('An error occurred: ' + xhr.responseText);
console.error('Error details:', error);
}
});
Solution
Implement comprehensive error handling on the backend as well. You can customize error messages in your Spring Controller:
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
5. Not Using HTTPS
When working with AJAX calls, especially for sensitive data, not using HTTPS can expose your data to vulnerabilities such as man-in-the-middle attacks.
Solution
Always deploy your application over HTTPS. This ensures that your AJAX requests are encrypted, safeguarding user information.
6. Blocking the UI Thread
If you perform heavy computations on the client side after receiving an AJAX response, you can block the UI thread, leading to a poor user experience.
Solution
Use Web Workers for heavy computations or defer processing to a later point when the UI is idle.
7. DRY (Don't Repeat Yourself) Principle Violation
Repeated AJAX call definitions can lead to maintenance challenges. If you've defined the same AJAX call in multiple places, it can be cumbersome to update.
Solution
Encapsulate your AJAX calls in functions:
function fetchUsers() {
return $.ajax({
url: '/api/users',
method: 'GET'
});
}
// Usage
fetchUsers().then(data => {
// Handle success
}).catch(error => {
// Handle error
});
By wrapping your AJAX calls in functions, you promote code reuse and simplify maintenance.
Wrapping Up
Incorporating AJAX calls in Spring MVC REST applications enhances user experience but can come with challenges. Being aware of common pitfalls such as CORS issues, error handling, and response formatting will pave the way for smoother integrations.
For more in-depth knowledge on AJAX and Spring MVC, consider visiting Spring.io, which provides extensive documentation and examples. Additionally, checking out MDN Web Docs on AJAX can enhance your understanding of how AJAX works.
By doing so, you not only improve your technical knowledge but also ensure your web applications are robust, user-friendly, and effective.
Happy coding!
Checkout our other articles