Mastering Feature Toggles: Avoiding Common Pitfalls
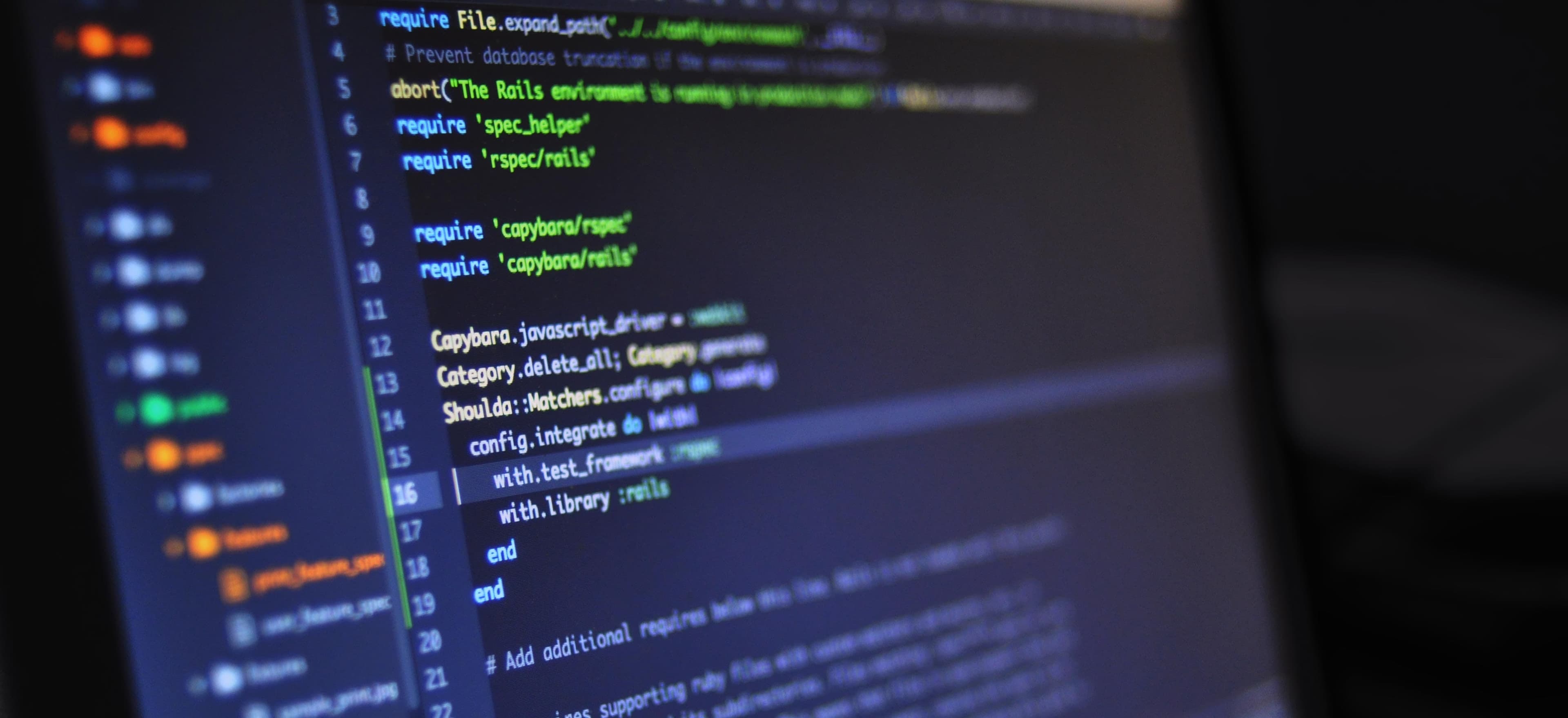
- Published on
Mastering Feature Toggles: Avoiding Common Pitfalls
In today's fast-paced development world, feature toggles—or feature flags—have become an essential tool for managing the deployment of new features. But with great power comes great responsibility. Mismanaging feature toggles can lead to technical debt and complicate your codebase. In this post, we’ll explore the benefits of using feature toggles in Java development, outline common pitfalls developers encounter, and provide code snippets to illustrate best practices.
What Are Feature Toggles?
Feature toggles allow developers to switch features on or off without deploying new code. This means you can deploy unfinished features or conduct A/B testing without customer-facing interruptions. Feature toggles also allow teams to manage risk by controlling exposure to new features.
Why Use Feature Toggles?
- Continuous Delivery: They facilitate continuous integration and delivery practices.
- Testing in Production: You can test new features in a live environment without exposing all users to potential issues.
- Granular Control: Feature toggles allow for targeted rollouts to specific user segments.
- Rollback Capability: If a new feature has issues, it can be quickly turned off.
Common Pitfalls to Avoid
While feature toggles can offer numerous benefits, they can also lead to complications if not managed correctly. Let's discuss a few common pitfalls.
1. Maintenance Overhead
Feature toggles can pile up, leading to cluttered code. It's a common scenario that developers have toggles lingering around well after they're deemed unnecessary.
Solution: Regularly review and clean up your feature toggles.
public class FeatureToggle {
private static final boolean NEW_FEATURE_ENABLED = true; // This should be regularly reviewed.
public static void executeFeature() {
if (NEW_FEATURE_ENABLED) {
// New feature code
System.out.println("New Feature is ON");
} else {
// Old feature code
System.out.println("Old Feature is ON");
}
}
}
Why? A clear distinction and occasional cleanup reduce complexity. You should aim to keep your code clean and readable.
2. Misuse of Booleans
Using a Boolean flag for every feature may seem straightforward, but it quickly becomes unmanageable. When toggles are overused, they dilute the clarity of your code.
Solution: Use a configuration management system, which can better handle complex flags.
import java.util.HashMap;
import java.util.Map;
public class FeatureToggleManager {
private Map<String, Boolean> featureToggles = new HashMap<>();
public void setFeature(String feature, boolean isEnabled) {
featureToggles.put(feature, isEnabled);
}
public boolean isFeatureEnabled(String feature) {
return featureToggles.getOrDefault(feature, false);
}
}
Why? This approach allows for multiple features to be managed transparently instead of relying on a fragmented series of Booleans.
3. Inconsistent Toggles Across Multiple Environments
Inconsistencies between dev, staging, and production environments can lead to unexpected behavior. Developers might think they have deployed a feature, but it behaves differently in production.
Solution: Ensure that feature toggles are applied consistently across all environments.
public class ApplicationContext {
private FeatureToggleManager toggleManager;
public ApplicationContext(FeatureToggleManager toggleManager) {
this.toggleManager = toggleManager;
}
public void run() {
if (toggleManager.isFeatureEnabled("DARK_MODE")) {
// Enable dark mode
System.out.println("Dark Mode is Enabled");
} else {
// Default mode
System.out.println("Default Mode is Active");
}
}
}
Why? Having a single source of truth for feature toggles helps keep all environments in sync.
4. Feature Toggle Spaghetti Code
Complex logic combined with feature toggles can lead to a tangled mess, often referred to as "spaghetti code."
Solution: Segment your code logic based on feature toggles, ideally separating concerns.
public class UserInterface {
private FeatureToggleManager toggleManager;
public UserInterface(FeatureToggleManager toggleManager) {
this.toggleManager = toggleManager;
}
public void display() {
if (toggleManager.isFeatureEnabled("NEW_BUTTON")) {
displayNewButton();
} else {
displayOldButton();
}
}
private void displayNewButton() {
System.out.println("Displaying New Button");
}
private void displayOldButton() {
System.out.println("Displaying Old Button");
}
}
Why? This approach keeps logic tightly aligned with features, making it easier to add or remove toggles.
Best Practices for Managing Feature Toggles
- Keep It Simple: Create toggle checks that are easy to understand.
- Document Your Toggles: Keep an up-to-date list of all feature toggles, their purpose, and when to remove them.
- Regular Cleanup: Set a schedule for removing feature toggles that are no longer in use.
- Use Different Types of Toggles: Use various toggle types (e.g., release toggles, experiment toggles, ops toggles) to align toggles with your development strategy.
For an in-depth read on feature toggle types, check out Martin Fowler's article on Feature Toggles.
Final Considerations
Feature toggles can accelerate your development process and enhance your software's agility, but they come with challenges. By understanding common pitfalls and applying best practices, you can master the art of feature toggling in Java development.
Adopting a systematic approach in managing your feature flags, and implementing practices such as regular reviews and documentation, will ensure that your code remains pristine and maintainable. Embrace feature toggles with caution, and enjoy the benefits they bring to your development lifecycle!
Further Reading
- For more insights into feature flags, check out featureflags.io.
By mastering feature toggles, you can leverage their full potential and mitigate the risks associated with their use. Happy coding!