Common Pitfalls When Starting a Maven Web Project
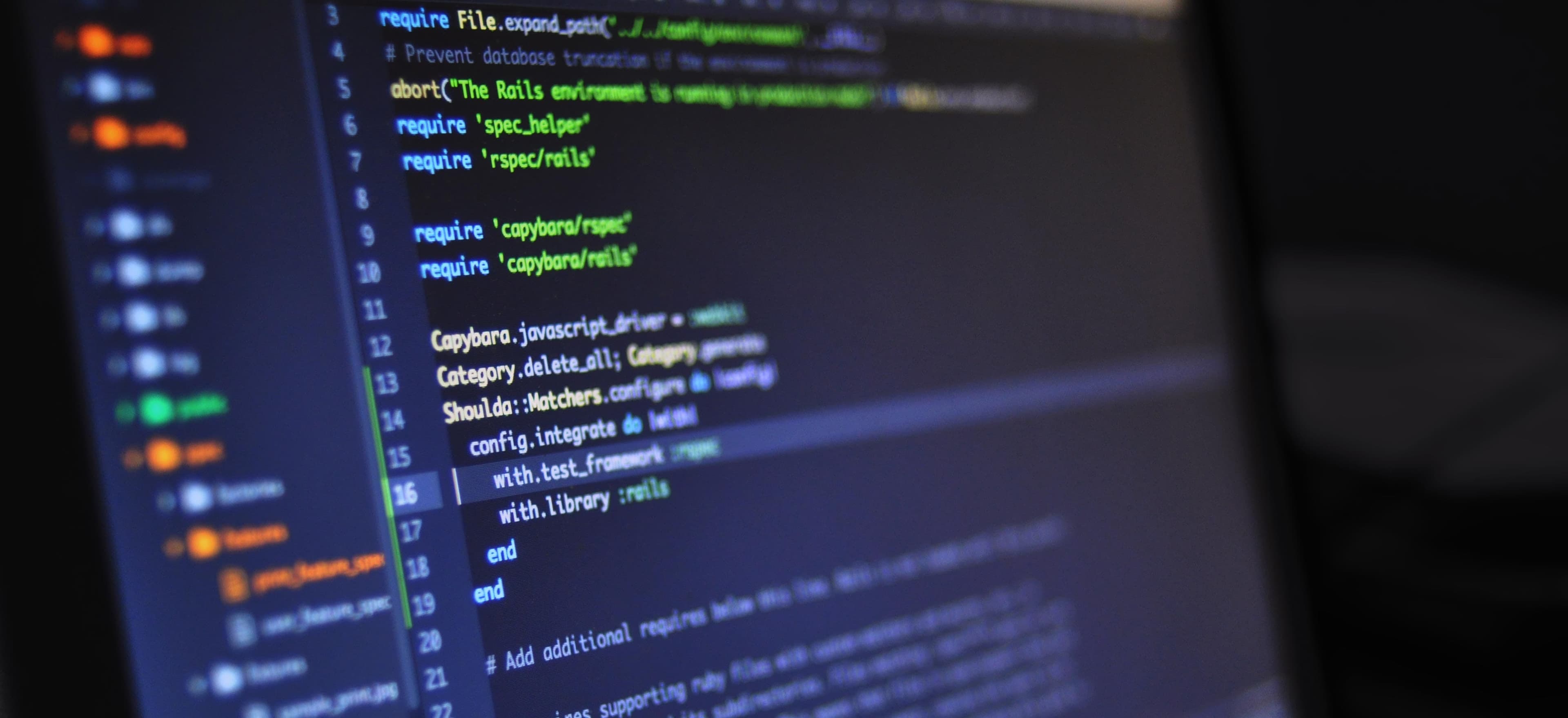
- Published on
Common Pitfalls When Starting a Maven Web Project
Maven is a powerful build automation tool used primarily for Java projects. It simplifies the process of managing dependencies, building the project, and generating documentation. However, newcomers to the Maven ecosystem, especially within the context of web projects, often encounter various pitfalls that can impede their progress. In this blog, we will delve into these common issues and how to avoid them, ensuring a smoother development experience.
Understanding Maven Basics
Before diving into the pitfalls, it is essential to understand what Maven is and how it operates. Maven uses a Project Object Model (POM) file, typically named pom.xml
, which contains information about the project and configuration details used by Maven to build the project.
Key Terminology:
- Dependency: A library that your project requires to function.
- Plugin: A tool that extends Maven's capabilities, often to assist in building processes.
- Repository: A location to store project artifacts, usually central or local repositories.
With this foundation in mind, let's explore common pitfalls.
1. Ignoring Dependency Management
Problem
One of the most frequent mistakes is neglecting to manage dependencies properly within the pom.xml
. Adding libraries without understanding their compatibility can lead to conflicts, known as "dependency hell".
Solution
To manage dependencies effectively, always specify the correct version and scope. Use the <dependencyManagement>
section in your POM to centralize dependency versions, particularly beneficial in multi-module projects.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.8</version>
</dependency>
</dependencies>
</dependencyManagement>
Why? This centralizes the version control and minimizes version conflicts across modules.
2. Not Utilizing the Maven Lifecycle
Problem
Maven has a specific lifecycle consisting of several phases, such as compile
, test
, and package
. New developers may not utilize these phases adequately, leading to missed opportunities for automation.
Solution
Understand and utilize the Maven lifecycle by correctly invoking commands. For instance, you can build and run tests in one command:
mvn clean install
Why? This command cleans the previous builds and prepares the project, ensuring that you always work with the latest code version.
Additional Learning
For more in-depth information on the Maven lifecycle, you can visit Introduction to the Maven Build Lifecycle.
3. Misconfiguring Plugins
Problem
Plugins enhance Maven's capabilities, yet incorrectly configured plugins can lead to build failures. Beginners often skip the plugin configuration details.
Solution
Pay close attention to plugin versions and configurations. For example, to configure the maven-compiler-plugin
, you should specify the Java version as follows:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
Why? This ensures that the correct Java version is used, increasing compatibility and reducing runtime errors.
4. Underestimating the Importance of the .gitignore File
Problem
When starting a web project, developers often forget to create a .gitignore
file, leading to the accidental inclusion of unnecessary files in the version control system (VCS).
Solution
Make sure to create a .gitignore
file to exclude build artifacts and IDE-specific files. A basic .gitignore
for a Maven project might look like this:
target/
*.class
*.log
*.iml
.idea/
Why? By excluding unnecessary files, you keep your repository clean and focused only on relevant source code.
5. Not Leveraging Profiles
Problem
Maven profiles allow developers to customize the build for different environments, but many neglect to utilize them, leading to repetitive configurations.
Solution
Define different profiles for development and production environments. Below is an example profile configuration in your pom.xml
:
<profiles>
<profile>
<id>development</id>
<properties>
<env>dev</env>
</properties>
</profile>
<profile>
<id>production</id>
<properties>
<env>prod</env>
</properties>
</profile>
</profiles>
You can activate a profile using:
mvn clean install -P development
Why? Profiles allow you to define environment-specific properties efficiently, reducing manual configuration.
6. Failing to Read the Documentation
Problem
With large frameworks and tools, some developers take shortcuts instead of referring to official documentation, which often leads to confusion and misconfigurations.
Solution
Always refer to the Maven official documentation before making significant decisions. It is a treasure trove of information for best practices and tool usage.
Why? Reading the documentation helps you stay updated with the latest features and best practices from the developers of Maven themselves.
7. Confusing Local and Central Repositories
Problem
Many beginners do not understand the difference between local and central repositories. This can lead to issues when resolving dependencies.
Solution
Understand that the local repository is typically stored on your machine, while the central repository is a shared collection of libraries available for all projects. If you need to add libraries not available in the central repository, you can install them manually into the local repository:
mvn install:install-file -Dfile=path/to/myfile.jar -DgroupId=com.example -DartifactId=my-artifact -Dversion=1.0 -Dpackaging=jar
Why? By knowing how to properly manage both repositories, you can ensure that dependencies are resolved correctly and efficiently.
The Closing Argument
Starting a Maven web project can be an intimidating process, but recognizing these common pitfalls can greatly improve your experience. By managing dependencies correctly, utilizing the Maven lifecycle, carefully configuring plugins, and more, your projects can run smoothly and efficiently.
Adopting practices like these not only saves time but also leads to a more maintainable codebase. Remember, learning is an iterative process, and each project presents an opportunity to refine your Maven skills.
For those looking to expand their knowledge further, consider exploring additional resources like Maven's official website or the Maven Plugin Documentation.
Happy coding!