Mastering Coordination in Akka: Overcoming Common Pitfalls
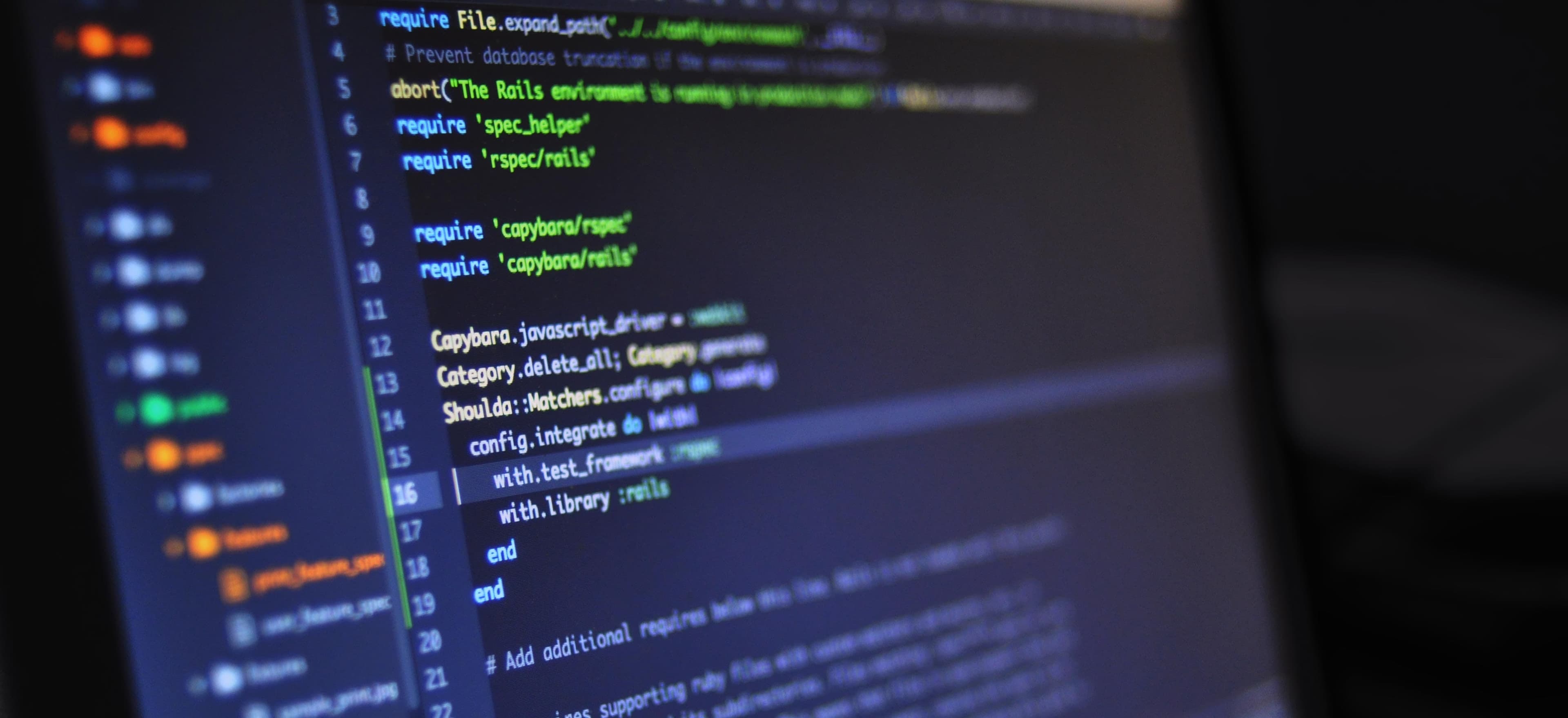
- Published on
Mastering Coordination in Akka: Overcoming Common Pitfalls
In the world of distributed systems and concurrent programming, Akka stands out as a powerful toolkit for building scalable and resilient applications. Its actor-based model provides an elegant way to manage state, concurrency, and communication. However, like any powerful tool, it comes with its complications. This blog post delves into the common pitfalls when coordinating actors in Akka and offers insights on how to overcome them.
Understanding the Actor Model
Before diving into the pitfalls, it is essential to grasp the core principles of the actor model. Actors are the fundamental units of computation in Akka. They encapsulate state and behavior, process messages asynchronously, and manage their own life cycles. By negotiating methods of communication and sharing data, actors can work in harmony to deliver complex functionalities.
For a basic introduction to the actor model, refer to the Akka documentation.
Common Pitfall 1: Message Ordering and Delivery Guarantees
One of the first challenges developers face is ensuring the correct ordering of messages. Actors process messages asynchronously, which means messages sent to an actor will not necessarily be executed in the order they were received. This can lead to problems, especially if an actor relies on the state from previous messages.
Solution
To tackle the message ordering issue, there are several strategies:
-
Use a Stateful Actor: If an actor needs to maintain the order of messages, consider implementing a state machine. Here's a simple example:
import akka.actor.{Actor, Props} class OrderedActor extends Actor { var state: Int = 0 def receive: Receive = { case Increment => state += 1 sender() ! CurrentState(state) case Decrement => state -= 1 sender() ! CurrentState(state) } } object OrderedActor { def props: Props = Props[OrderedActor]() }
This actor maintains its state and ensures that all increments and decrements are processed in order.
-
Implement a Message Queue: If maintaining order is critical across multiple actors, you might consider a queue to manage incoming messages.
The Last Word on Ordering
By using stateful actors or a message queue, you can ensure that messages are processed in the order they should be, avoiding data inconsistencies.
Common Pitfall 2: Actor Supervision and Fault Tolerance
In a distributed environment, actors can fail or behave unexpectedly. One of Akka's strengths is its supervision strategy, which allows you to define how to react to faults.
Solution
To enable robust error handling, define clear supervision strategies. Here’s an example:
import akka.actor.{Actor, ActorRef, ActorSystem, Props}
class Supervisor extends Actor {
val child: ActorRef = context.actorOf(Props[ChildActor])
def receive: Receive = {
case msg => child forward msg
}
override val supervisorStrategy = OneForOneStrategy() {
case _: ArithmeticException => Restart
case _: NullPointerException => Resume
case _: Exception => Stop
}
}
In this code snippet, the supervisor restarts the child actor on an arithmetic exception but resumes it on a null pointer exception. Familiarize yourself with strategies like OneForOneStrategy
and AllForOneStrategy
in the Akka documentation to tailor supervision strategies to suit your needs.
The Last Word on Supervision
Implementing a robust supervision strategy ensures that your actors can recover from failures, thus maintaining system stability.
Common Pitfall 3: Deadlocks and Starvation
Deadlocks and starvation are common issues in concurrent programming when resources are not effectively managed. In Akka, deadlocks can occur if two or more actors are waiting for each other to release resources.
Solution
-
Message Passing: Leverage the non-blocking nature of actors by relying heavily on message passing. This inherently reduces the chances of deadlocks.
-
Designing for Asynchronous Workflows: Consider breaking tasks into smaller, manageable asynchronous units. Here’s an example of how you might handle long-lasting computations:
import akka.actor.{Actor, Props} import scala.concurrent.Future import scala.concurrent.ExecutionContext.Implicits.global class ComputationActor extends Actor { def receive: Receive = { case StartComputation => Future { // Long computation }.pipeTo(sender()) } } object ComputationActor { def props: Props = Props[ComputationActor]() }
This actor performs its computations asynchronously and uses futures to handle long-running tasks.
The Last Word on Deadlocks
By embracing a message-driven or non-blocking architecture, you can effectively reduce the likelihood of deadlocks and starvations in your Akka applications.
Common Pitfall 4: Over-Supervision
While supervision is essential for system reliability, over-supervising actors can lead to resource overhead and degrade performance.
Solution
Combat over-supervision by adopting a layered approach to actor supervision. Use lightweight actors for simple tasks while allowing heavier processing to be handled by dedicated actors. Focus on the divide-and-conquer approach:
class HeavyWorker extends Actor {
def receive: Receive = {
case HeavyTask =>
// Handle heavy tasks
}
}
class LightweightSupervisor extends Actor {
val heavyWorker: ActorRef = context.actorOf(Props[HeavyWorker])
def receive: Receive = {
case task: HeavyTask =>
heavyWorker forward task
}
}
In this architecture, the lightweight supervisor delegates heavy tasks to specialized actors, reducing the supervisory burden.
The Last Word on Over-Supervision
A well-considered supervision strategy can mitigate unnecessary overhead in your Akka applications.
Best Practices to Remember
- Encapsulate State: Ensure actors maintain their state privately. This minimizes side effects and promotes better coordination.
- Inform Task Design: Design your tasks around the actor model's strengths—performance tuning should consider the asynchronous nature of processing.
- Monitor System Health: Use Akka's monitoring features to observe actor states and health for early detection of issues.
The Last Word
Mastering coordination in Akka requires a comprehensive understanding of its strengths and potential pitfalls. From implementing supervision strategies to ensuring message order and avoiding deadlocks, each challenge presents an opportunity for improvement in your software design.
For more in-depth exploration of Akka, consider checking out the official documentation.
By applying these strategies, you will not only enhance your application's resilience but also elevate the overall performance of your Akka-based solutions. Happy coding!