Common Pitfalls in CQRS Read Models with Hibernate
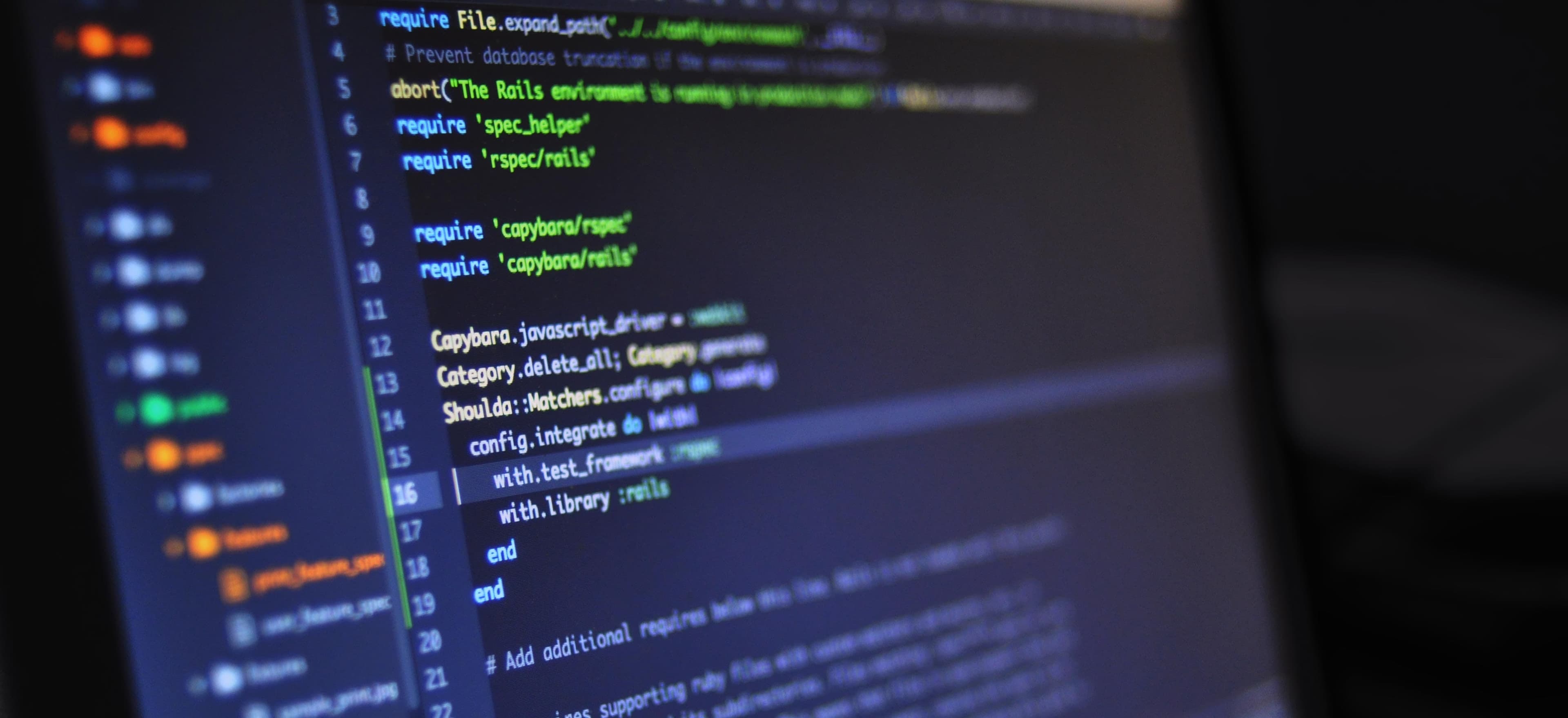
- Published on
Common Pitfalls in CQRS Read Models with Hibernate
The Command Query Responsibility Segregation (CQRS) pattern has become increasingly popular in designing complex systems. It allows for the separation of read and write operations, optimizing each independently to suit their requirements. While this powerful architecture has its advantages, developers often face common pitfalls when implementing the read model, particularly when using Hibernate.
This blog post dives into these pitfalls, offering practical insights and code snippets that illustrate best practices to avoid potential issues. Let's get started!
Understanding CQRS and Read Models
To grasp the pitfalls, let's briefly discuss CQRS. In this pattern, commands (write operations) and queries (read operations) are isolated. The write model handles updates, while the read model is designed specifically for retrieval. When using Hibernate, a popular Object-Relational Mapping (ORM) tool, developers sometimes encounter challenges with optimizing the read model.
It's essential to structure your read model carefully to handle queries efficiently. This post will explore common pitfalls and how to navigate them effectively.
Pitfall 1: Over-fetching Data
Issue
One of the most frequent mistakes is over-fetching data in the read model. This happens when a query retrieves more entities than necessary, leading to performance degradation.
Example
Consider a scenario where you need to fetch a list of users along with their associated roles:
List<User> userList = session.createQuery("FROM User", User.class).list();
In this case, if each User
entity has a collection of Role
entities, Hibernate will perform a cartesian product query under the hood, loading every related record.
Solution
To minimize over-fetching, use projections or DTOs (Data Transfer Objects) to specify only the required fields. Here’s how you can implement this:
List<UserDTO> userDTOList = session.createQuery(
"SELECT new com.example.UserDTO(u.id, u.username) FROM User u", UserDTO.class
).list();
Why This Matters
Using DTOs allows you to retrieve only the information you need for a particular operation. It reduces memory usage and improves query performance, especially when dealing with large datasets.
Pitfall 2: Ignoring Pagination
Issue
When dealing with large datasets, another common mistake is not implementing pagination, leading to performance bottlenecks and poor user experience.
Example
Assume you are fetching all records of users without any pagination:
List<User> userList = session.createQuery("FROM User", User.class).list();
When User
contains thousands of records, this can slow down response times significantly.
Solution
Utilize Hibernate's built-in pagination capabilities with setFirstResult
and setMaxResults
to limit the number of records fetched:
List<User> userList = session.createQuery("FROM User", User.class)
.setFirstResult(page * size)
.setMaxResults(size)
.list();
Why This Matters
Pagination can significantly reduce the load time and memory consumption, ensuring that users have a responsive experience even when working with vast datasets.
Pitfall 3: N+1 Select Problem
Issue
The N+1 Select problem occurs when fetching a list of entities that have associated relationships. For instance, loading a list of Users
and their Roles
one-by-one causes multiple queries to be executed.
Example
List<User> userList = session.createQuery("FROM User", User.class).list();
for (User user : userList) {
// This will trigger a separate query for each user's roles
Set<Role> roles = user.getRoles();
}
This leads to N+1 queries: one for all users and N additional ones for user roles.
Solution
Eager fetching can help mitigate this problem by loading the relationships in a single query:
List<User> userList = session.createQuery("FROM User u JOIN FETCH u.roles", User.class).list();
Why This Matters
Eager fetching can significantly reduce the number of queries executed, optimizing the reading process and enhancing the overall performance of your application.
Pitfall 4: Lack of Consistency in Read Models
Issue
When using CQRS, maintaining consistency between the write and read models is crucial. However, a common mistake is not synchronizing the read model after changes occur in the write model.
Example
If a user is created or updated, failing to update the corresponding read model can result in stale data being returned to the client.
Solution
Consider implementing an event-driven architecture wherein changes in the write model publish events that are handled to update the read model accordingly.
public void userCreated(User user) {
eventPublisher.publish(new UserCreatedEvent(user));
}
// In the event listener for UserCreatedEvent
public void handleUserCreated(UserCreatedEvent event) {
UserDTO userDTO = new UserDTO(event.getUser().getId(), event.getUser().getUsername());
userRepository.save(userDTO); // Saving to the read model
}
Why This Matters
By keeping your read model updated with real-time changes, you ensure that users receive consistent data, enhancing trust in your application.
Pitfall 5: Inefficient Query Design
Issue
Poorly designed queries can lead to significant performance issues. A common pitfall involves using unindexed fields or writing complex joins that can slow down response time.
Example
Using a subquery in a complex query can decrease performance if not carefully implemented.
List<User> userList = session.createQuery(
"SELECT u FROM User u WHERE u.id IN (SELECT ur.userId FROM UserRoles ur WHERE ur.roleId = :roleId)", User.class
)
.setParameter("roleId", someRoleId)
.list();
Solution
Instead, look for ways to simplify the query or create database indexes to optimize the common access paths.
List<User> userList = session.createQuery(
"SELECT u FROM User u JOIN u.roles r WHERE r.id = :roleId", User.class
)
.setParameter("roleId", someRoleId)
.list();
Why This Matters
Efficient query design not only improves performance but also maintains the scalability of your application as it grows.
My Closing Thoughts on the Matter
While using Hibernate to implement CQRS read models offers many benefits, developers must be vigilant in avoiding common pitfalls. By addressing issues such as over-fetching, pagination, N+1 queries, consistency, and query efficiency, you can enhance the performance and reliability of your application.
Consider exploring CQRS Essentials and Hibernate Performance Tuning for deeper insights.
By applying best practices and understanding the potential risks, you can confidently build a robust, efficient application that takes full advantage of the CQRS design pattern. Happy coding!