Optimizing CSV Parsing and Grouping in Spark Efficiently
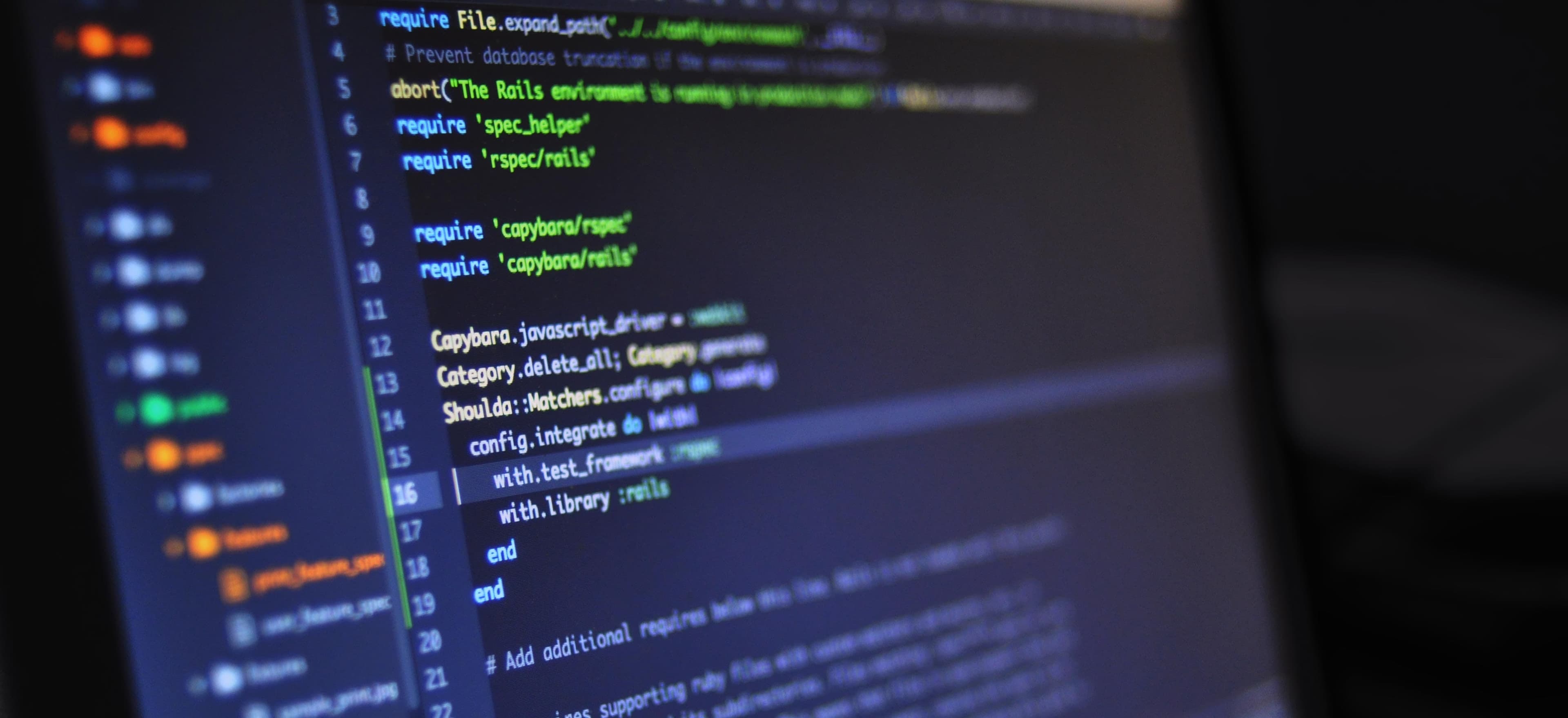
- Published on
Optimizing CSV Parsing and Grouping in Spark Efficiently
Apache Spark has gained immense popularity due to its ability to handle large data sets with low latency. One of the most common tasks in data processing is parsing CSV files and performing data grouping operations. In this blog post, we will explore efficient techniques for CSV parsing and grouping using Spark.
Understanding CSV Parsing and Grouping
CSV (Comma-Separated Values) is a simple text format used for storing tabular data. It’s widely used for its simplicity but can become cumbersome when dealing with large files. Grouping, on the other hand, is the process of aggregating data based on specific columns, which can help in analyzing and summarizing the dataset effectively.
Why Optimize CSV Parsing and Grouping?
- Performance: Large datasets can lead to long processing times. Efficient parsing and grouping can significantly reduce runtime.
- Resource Management: Optimizing operations can minimize the cluster resources consumed, leading to cost savings.
- Scalability: Optimized processes handle growth in data volumes better.
Setting Up Spark
If you haven't set up Spark yet, follow these steps before we dig into parsing and grouping operations.
- Install Apache Spark by following the instructions on the official documentation.
- Ensure that you have the necessary dependencies installed. For Python developers, using PySpark is common, while Java developers can utilize the Spark Java API.
Sample Data
For our demonstration, let’s assume we have a CSV file named employees.csv
with the following structure:
id,name,department,salary
1,John Doe,Engineering,70000
2,Jane Smith,Marketing,80000
3,Emily Davis,Engineering,75000
4,Michael Brown,Marketing,80000
5,David Wilson,HR,50000
Reading CSV in Spark
Before we parse the CSV file, it is essential to understand the best practices for reading it into a Spark DataFrame.
Loading CSV Data
Here’s how to load the CSV file into a DataFrame using Spark:
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class CSVParser {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("CSV Parser")
.master("local")
.getOrCreate();
// Load CSV file
Dataset<Row> employeeData = spark.read()
.option("header", "true") // Use the first line as headers
.option("inferSchema", "true") // Automatically infer data types
.csv("path/to/employees.csv");
// Show data
employeeData.show();
spark.stop();
}
}
Commentary on Code
- Header Option: By setting the header option to true, Spark uses the first row of the CSV file as the DataFrame column names.
- Infer Schema: This option indicates that Spark should automatically determine the data types of the columns, making it easier to work with the different data types present in your dataset.
Efficient Grouping of Data
Once the data is loaded, you might want to group it based on specific columns. For instance, we can group our employee data by the department and calculate the average salary.
Grouping by Department
Here's how to perform the grouping operation in Spark:
import org.apache.spark.sql.functions;
public class CSVGrouping {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("CSV Grouping")
.master("local")
.getOrCreate();
Dataset<Row> employeeData = spark.read()
.option("header", "true")
.option("inferSchema", "true")
.csv("path/to/employees.csv");
// Group by department and calculate average salary
Dataset<Row> avgSalaryByDepartment = employeeData.groupBy("department")
.agg(functions.avg("salary").alias("average_salary"));
avgSalaryByDepartment.show();
spark.stop();
}
}
Explanation of Code
- groupBy(): This method is used to group the data based on the specified column(s). In our case, we’re grouping by the
department
. - agg(): This function is used to perform aggregation on the grouped data. We use the
avg()
function to calculate the average salary. - alias(): This method renames the resulting column for better readability.
Performance Optimization Techniques
To ensure that our parsing and grouping processes are efficient, consider applying the following optimizations:
1. Using the Right Data Format
While CSV is widely used, it may not be the most efficient format for large datasets. Parquet or ORC formats provide better performance for read and write operations due to their columnar storage structure.
Example of Saving as Parquet
After manipulations, you can save your DataFrame in optimal formats:
avgSalaryByDepartment.write()
.mode("overwrite")
.parquet("path/to/average_salaries.parquet");
2. Caching DataFrames
If you’re going to carry out multiple operations on the same DataFrame, it might be worthwhile to cache it in memory.
employeeData.cache();
3. Adjusting Spark Configuration
Tuning the Spark engine’s configuration can heavily impact performance. Some configurations worth considering include:
spark.sql.shuffle.partitions
: Adjust the number of partitions used when shuffling data for joins or aggregations.spark.executor.memory
: Increase the executor memory to handle larger computations.
The Closing Argument
Efficiently parsing CSV files and performing grouping operations in Apache Spark is essential for large data processing tasks. By following the above techniques outlined in this blog post, you can reduce runtime, manage resources effectively, and ultimately scale your data workflows.
For further reading, consult the following resources:
- Apache Spark Documentation
- Optimizing Apache Spark SQL
By understanding and implementing these practices, you can ensure that your data processing pipelines remain efficient and scalable as your data needs grow. Happy coding!