Mastering State Patterns: Common Pitfalls to Avoid
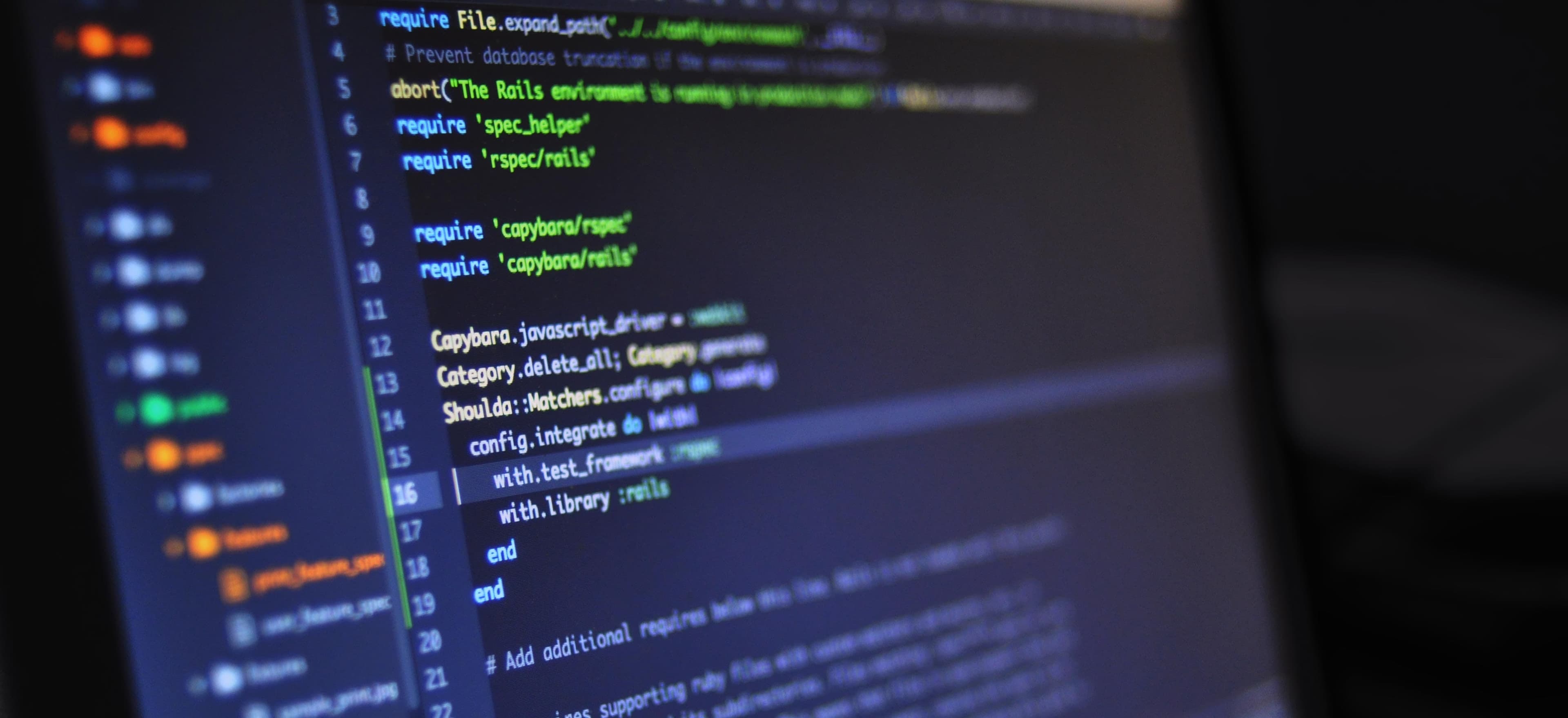
- Published on
Mastering State Patterns: Common Pitfalls to Avoid
State patterns are an invaluable design principle in the world of software engineering. They allow you to manage state-based behavior dynamically. However, implementing state patterns can inadvertently lead to complications that, if not addressed, can create a tangled web of code. This blog post aims to guide you through the common pitfalls when adopting state patterns while providing actionable insights to help streamline your implementation.
What is the State Pattern?
The State pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes. This is often used in systems where an object can be in multiple states, each with distinct behaviors or responses.
Why Use the State Pattern?
Using the State pattern can simplify your code and make it more manageable. It encapsulates state-specific behavior in separate classes, leading to:
- Improved Readability: Behavior related to each state is isolated, making the code easier to follow.
- Enhanced Maintainability: Adding new states or modifying existing ones does not necessitate a significant overhaul of your codebase.
- Greater Flexibility: You can change an object's state dynamically at runtime.
Common Pitfalls in Implementing State Patterns
While the State pattern has many advantages, there are some common pitfalls you should be aware of to prevent introducing complexity into your projects.
1. Overcomplicating Simple States
One of the most frequent mistakes developers make is overengineering states. It’s easy to assume that every state requires its own class. However, not every state warrants a distinct implementation.
Example
// Overcomplicated state representation
public class LightSwitch {
private State currentState;
public LightSwitch() {
this.currentState = new OffState();
}
public void toggle() {
currentState.toggle(this);
}
public void setState(State state) {
this.currentState = state;
}
}
interface State {
void toggle(LightSwitch lightSwitch);
}
class OffState implements State {
@Override
public void toggle(LightSwitch lightSwitch) {
System.out.println("Turning on...");
lightSwitch.setState(new OnState());
}
}
class OnState implements State {
@Override
public void toggle(LightSwitch lightSwitch) {
System.out.println("Turning off...");
lightSwitch.setState(new OffState());
}
}
In the example above, the LightSwitch
could have simply used an enum
instead of separate classes, drastically reducing complexity. Consider this simpler alternative:
public enum LightSwitchState {
ON, OFF
}
public class LightSwitch {
private LightSwitchState currentState;
public LightSwitch() {
this.currentState = LightSwitchState.OFF;
}
public void toggle() {
if (currentState == LightSwitchState.OFF) {
System.out.println("Turning on...");
currentState = LightSwitchState.ON;
} else {
System.out.println("Turning off...");
currentState = LightSwitchState.OFF;
}
}
}
2. Lack of State Transition Management
Another common pitfall is neglecting to manage state transitions adequately. It’s essential to ensure valid transitions between states, preventing the possibility of entering an inconsistent system state.
Example of Missing Transition Management
Ignoring proper management might lead to unexpected behavior:
class Door {
private State currentState;
public Door() {
this.currentState = new ClosedState();
}
public void open() {
currentState.open(this);
}
public void close() {
currentState.close(this);
}
void setState(State state) {
this.currentState = state;
}
}
class OpenState implements State {
@Override
public void open(Door door) {
// Ignoring already open state
}
@Override
public void close(Door door) {
System.out.println("Closing door...");
door.setState(new ClosedState());
}
}
class ClosedState implements State {
@Override
public void open(Door door) {
System.out.println("Opening door...");
door.setState(new OpenState());
}
@Override
public void close(Door door) {
// Ignoring already closed state
}
}
To handle transitions better, explicitly manage state changes:
class Door {
private State currentState;
public Door() {
this.currentState = new ClosedState();
}
public void open() {
if (currentState instanceof ClosedState) {
currentState.open(this);
} else {
System.out.println("Door is already open!");
}
}
public void close() {
if (currentState instanceof OpenState) {
currentState.close(this);
} else {
System.out.println("Door is already closed!");
}
}
void setState(State state) {
this.currentState = state;
}
}
3. Not Considering Complexity in State-Dependent Behavior
Sometimes, developers overlook the complexity in states themselves. States might have additional properties that are intertwined with your application's logic. Failing to consider the state’s complexity can lead to chaotic designs.
Example
class Order {
private State currentState;
public Order() {
this.currentState = new NewState();
}
public void proceed() {
currentState.proceed(this);
}
public void cancel() {
currentState.cancel(this);
}
void setState(State state) {
this.currentState = state;
}
}
// Assume similar state classes for New, Processing, Completed, etc.
In this case, additional properties such as delivery methods or payment states could make the logic convoluted. Isolating complexity into encapsulated states or introducing a state data model can alleviate this burden.
4. Forgetting to Implement Test Cases
The last pitfall involves neglecting proper unit testing for your state management. With multiple states, testing can become complex. Automating the verification of state transitions and behaviors is critical in maintaining dependable code.
Using frameworks like JUnit in Java can help facilitate cleaner tests:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class DoorTest {
@Test
public void testToggleOpenClose() {
Door door = new Door();
door.open();
assertEquals(OpenState.class, door.currentState.getClass());
door.close();
assertEquals(ClosedState.class, door.currentState.getClass());
}
@Test
public void testAlreadyOpen() {
Door door = new Door();
door.open(); // First toggle to open
// Check if opening again gives a proper message
assertTrue(door.open(shouldPrintMessageHere));
}
}
It's critical to have sufficient tests that cover both positive and negative scenarios in your state transition logic.
In Conclusion, Here is What Matters
Mastering the State pattern means understanding both its strengths and its pitfalls. By avoiding overcomplication, adequately managing state transitions, considering complexity, and ensuring thorough testing, you can leverage this pattern to its full potential.
If you'd like to further enhance your knowledge of design patterns, check out the book Design Patterns: Elements of Reusable Object-Oriented Software by Gamma et al here.
The State pattern is a powerful tool when used correctly. By taking the time to address these common pitfalls, you can craft robust, maintainable systems that gracefully navigate the intricacies of state-based behavior. Happy coding!