5 Steps to Safely Refactor Your Monolithic Codebase
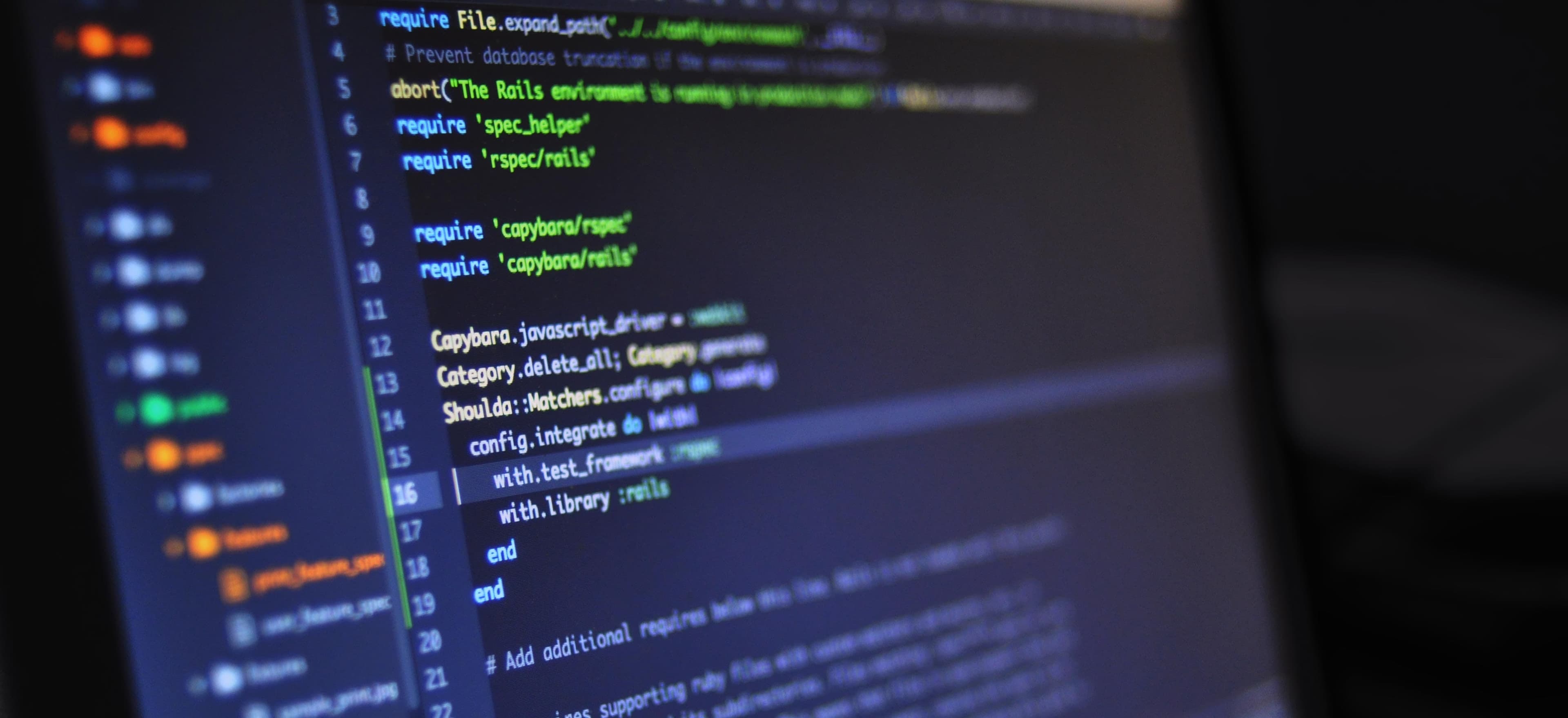
- Published on
5 Steps to Safely Refactor Your Monolithic Codebase
Refactoring a monolithic codebase can feel like both an art and a science, and rightly so. With the right approach, you can enhance the code's maintainability, readability, and scalability without the chaos that often accompanies such endeavors. In this blog post, we'll walk through five actionable steps to safely refactor your monolithic codebase while minimizing risks.
Why Refactor?
Before diving into the steps, let’s clarify why refactoring is essential. Monolithic applications are often tightly coupled and can become unwieldy over time. This can lead to:
- Increased difficulty in adding new features
- Higher chances of introducing bugs
- Longer onboarding times for new developers
Refactoring addresses these issues by breaking down complexities and improving the overall structure of the code.
Step 1: Assess Your Codebase
The first step in the refactoring process is accurately assessing your current codebase. This involves understanding its architecture, identifying the most problematic areas, and documenting the current functionalities.
Analyze Code with Tools
Consider utilizing tools such as SonarQube or Code Climate for static code analysis. They can highlight code smells, technical debt, and areas that need improvement.
Example:
// Example of a code smell: Long method
public void processOrder(Order order) {
validateOrder(order);
calculateTotal(order);
sendEmailConfirmation(order);
// More logic
}
Long methods like the one above create unmanageable blocks of logic that confuse developers. Aim to break such methods into smaller, more focused functions.
Why This Matters
By assessing your codebase with analytical tools, you gain invaluable insight. You can prioritize which areas require urgent attention, making your refactoring process more efficient.
Step 2: Create a Refactoring Plan
With assessment complete, it’s time to create a robust refactoring plan. This plan should outline the scope and timeline for your efforts.
Identify Patterns and Standards
Define coding standards and refactoring techniques that your team will use during the process. Pattern-based refactoring, as outlined in Martin Fowler's Refactoring: Improving the Design of Existing Code, can provide a structured way to approach common refactoring tasks.
Example Patterns
- Extract Method: Useful for breaking long methods into smaller parts.
public void someMethod() { doPart1(); doPart2(); } private void doPart1() { // logic for part 1 } private void doPart2() { // logic for part 2 }
- Introduce Parameter Object: Helps reduce the number of parameters in methods.
// Instead of public void createUser(String name, String email, String password) {...} // You can create a User class public class User { String name; String email; String password; } public void createUser(User user) {...}
Why This Matters
A well-defined plan allows for easier tracking of progress and facilitates team collaboration. It's a visual guide that can help align everyone's focus on common goals.
Step 3: Incremental Refactoring
Now comes the execution part, which should be done incrementally. Instead of attempting to refactor large sections of the code at once, breaking the task into smaller, manageable chunks ensures that changes are easier to test and review.
Use Feature Flags
Feature flags allow you to toggle functionality on and off without deploying new code. This can be particularly useful in a monolithic architecture, as you can experiment with changes without disrupting all users.
Example:
if (featureFlag.isEnabled("newFeature")) {
// New feature's implementation
} else {
// Old feature logic
}
Why This Matters
Incremental refactoring reduces risk. By isolating your changes, you can more easily identify which modifications have introduced issues, allowing you to revert when necessary.
Step 4: Include Automated Testing
Integrating automated testing into your refactoring process is not just beneficial; it's essential. Having a comprehensive suite of tests helps ensure that your refactoring does not break existing functionality.
Types of Tests
- Unit Tests: Test individual components for expected behavior.
- Integration Tests: Ensure that different components interact as expected.
- End-to-End Tests: Verify that the entire application flow works.
Here's a simple JUnit test for our earlier example:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class UserTest {
@Test
void testCreateUser() {
User user = new User("John Doe", "john@example.com", "password123");
assertEquals("John Doe", user.name);
}
}
Why This Matters
Automated tests are your safety net. They allow you to make changes with confidence, knowing that you can quickly identify any issues. This is especially critical in a monolithic codebase where one modification can ripple through the entire application.
Step 5: Document Your Changes
Finally, documentation is often overlooked during refactoring but is crucial. Proper documentation serves multiple purposes, such as aiding onboarding for new developers and ensuring that your team understands the architectural decision-making process.
Update Code Comments and Documentation
Whenever you refactor, update inline comments and relevant documentation. Highlight not just the 'how' but also the 'why' behind your changes.
Example:
/**
* Creates a new user from the provided User object.
*
* @param user The User object containing user details.
*/
public void createUser(User user) {
// User creation logic
}
Why This Matters
Effective documentation ensures that future modifications are easier to comprehend and implement. It can also prevent redundant work or conflicting changes among team members who may not be aware of recent updates.
The Last Word
Refactoring a monolithic codebase may seem daunting, but following these five steps—assessing your code, creating a plan, incrementally refactoring, implementing automated testing, and documenting changes—can streamline the process and significantly reduce risks.
With a thoughtful approach, your efforts can lead to a more maintainable, scalable, and robust application.
For more information about best practices in software development and code quality, check out resources like Martin Fowler's website and Clean Code. Happy coding!
Checkout our other articles