Overcoming Access Issues When Hosting Maven Artifacts on GCS
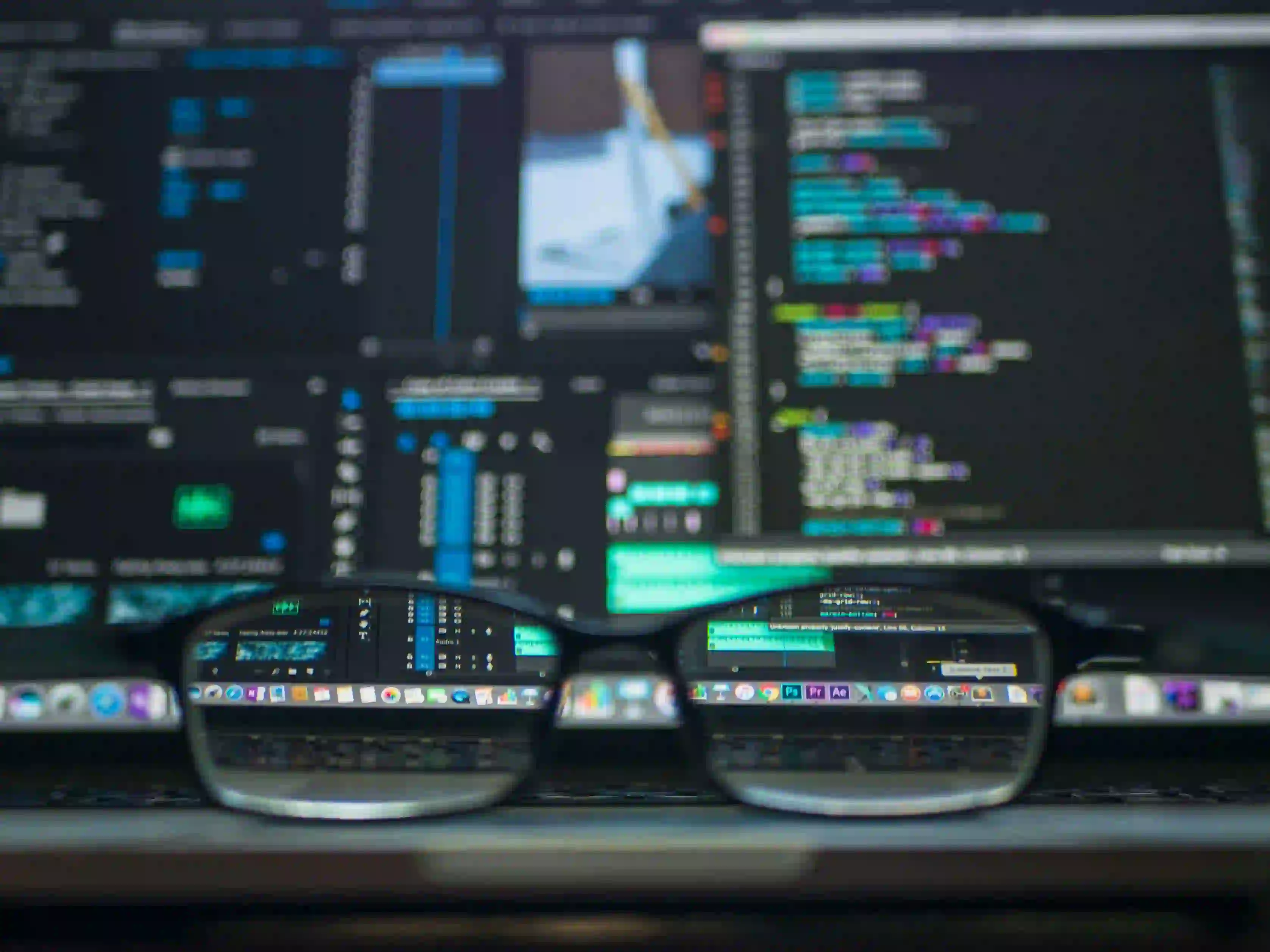
Overcoming Access Issues When Hosting Maven Artifacts on GCS
When it comes to managing Maven artifacts, Google Cloud Storage (GCS) provides a reliable platform for hosting libraries, dependencies, and other such files. However, developers can often face challenges regarding access, permissions, and efficient retrieval. In this post, we will explore some of the common access issues when hosting Maven artifacts on GCS and how to effectively mitigate them.
Understanding Maven and GCS
Maven is a powerful build automation tool primarily used for Java projects. It allows developers to manage dependencies, automate builds, and streamline project organization. The traditional way of managing these artifacts is through repositories such as Maven Central or JFrog Artifactory.
On the other hand, Google Cloud Storage (GCS) serves as a robust object storage service. It provides high availability, security, and easy accessibility. Combining the two creates a powerful development ecosystem, but it also introduces some complexity.
Common Access Issues
Authentication Errors
The first hurdle many developers encounter relates to authentication errors. GCS uses Google Cloud IAM (Identity and Access Management) to control access. This means that if the IAM settings are misconfigured, users may experience permission denied errors.
Solution
To resolve authentication issues, ensure you have created a service account with the necessary roles, such as Storage Admin
:
gsutil iam ch serviceAccount:YOUR_SERVICE_ACCOUNT_EMAIL:roles/storage.admin gs://YOUR_BUCKET_NAME
This command modifies the IAM policy on your bucket, granting the specified service account admin access. Replace YOUR_SERVICE_ACCOUNT_EMAIL
and YOUR_BUCKET_NAME
with your particular service account identifier and your GCS bucket name, respectively.
Incorrect Bucket Policies
Access issues can also arise from improperly set bucket policies. GCS allows you to manage who can access your buckets through predefined roles and permissions.
Solution
To set up the correct policies, you will need to review your bucket's accessibility around anonymous users and service accounts:
gsutil defacl set public-read gs://YOUR_BUCKET_NAME
This command provides public read access for your artifacts. It's crucial to apply the correct policies to maintain security while allowing necessary accessibility. For further details about bucket policies, you can view Google Cloud's documentation on IAM roles.
Networking Restrictions
In some cases, networking issues can affect your connections to GCS, especially in corporate environments where firewalls and proxies come into play.
Solution
You may need to configure your network settings to allow traffic on the required ports (typically port 443 for HTTPS).
Example: Deploying a Maven Artifact to GCS
Now, let's address how you can deploy a Maven artifact to GCS. You can achieve this by using the Maven GCS plugin, which simplifies the deployment process.
Maven POM Configuration
Here’s how to set up the Maven POM configuration:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>maven-gcs-example</artifactId>
<version>1.0-SNAPSHOT</version>
<distributionManagement>
<repository>
<id>gcs-repository</id>
<name>GCS Repository</name>
<url>gs://YOUR_BUCKET_NAME</url>
</repository>
</distributionManagement>
<build>
<plugins>
<plugin>
<groupId>com.google.cloud</groupId>
<artifactId>gcs-maven-plugin</artifactId>
<version>1.0.0</version>
<configuration>
<projectId>YOUR_PROJECT_ID</projectId>
<serviceAccountKey>path/to/service/account/key.json</serviceAccountKey>
</configuration>
</plugin>
</plugins>
</build>
</project>
Explanation
-
Distribution Management: This section defines where to deploy your artifacts. Replace
YOUR_BUCKET_NAME
with your GCS bucket name. -
Plugin Configuration: A service account key is required for authentication. The plugin connects to GCS using the provided credentials. Setting this up correctly will help avoid authentication-related issues.
Deploying the Artifact
Once your POM configuration is complete, you can deploy your Maven artifacts using:
mvn deploy
Final Thoughts
Hosting Maven artifacts on Google Cloud Storage provides a plethora of benefits, including scalability and security. However, access issues, including authentication errors, incorrect bucket policies, and networking restrictions, can impede progress.
By adhering to the proper IAM configurations, setting appropriate bucket policies, and using the Maven GCS plugin, you can ensure a seamless integration between Maven and GCS.
To dive deeper into managing Maven repositories, consider reviewing the following resources:
Implementing the solutions discussed in this blog will empower you to successfully deploy and manage your Maven artifacts on GCS, leading to improved workflows in your development projects. Don't let access issues hold you back; rather, embrace the capabilities of GCS and streamline your Maven building process!