Overcoming Complexity in Multilevel Adapter Patterns
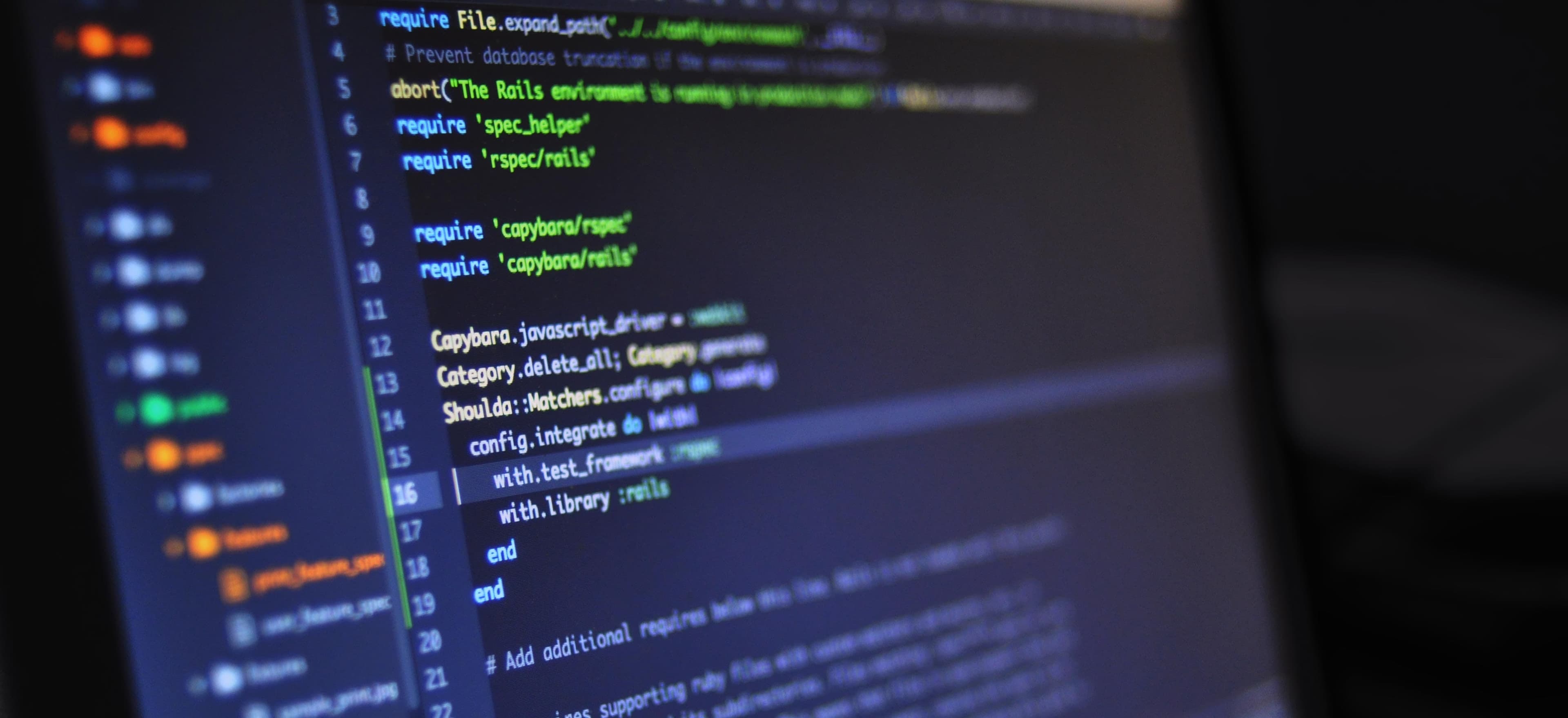
- Published on
Overcoming Complexity in Multilevel Adapter Patterns
In the world of software design, adoption of design patterns can lead to more maintainable, understandable, and reusable code. Among these patterns, the Adapter Pattern stands out for its utility in bridging the gap between incompatible interfaces. However, when scaled to a multilevel approach, complexity can often arise. In this article, we will explore the multilevel adapter patterns in Java, identify the challenges they pose, and present strategies for overcoming these complexities.
Table of Contents
- Understanding the Adapter Pattern
- Introduction to Multilevel Adapter Patterns
- Challenges of Multilevel Adapters
- Example of Multilevel Adapter Pattern
- Tips for Managing Complexity
- Conclusion
Understanding the Adapter Pattern
The Adapter Pattern is a structural design pattern that enables objects with incompatible interfaces to work together. This is achieved by creating an adapter class that essentially 'adapts' one interface to another, allowing for seamless communication.
Key Concepts:
- Target Interface: The interface that the client expects.
- Adapter: The class that implements the target interface and translates calls to the adaptee.
- Adaptee: The class that has the functionality but lacks an interface compatible with the client.
Practical Example:
// Target interface
interface Target {
void request();
}
// Adaptee class
class Adaptee {
public void specificRequest() {
System.out.println("Specific request.");
}
}
// Adapter class
class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void request() {
adaptee.specificRequest();
}
}
// Client code
public class Client {
public static void main(String[] args) {
Adaptee adaptee = new Adaptee();
Target target = new Adapter(adaptee);
target.request(); // Output: Specific request.
}
}
In this example, the adapter class serves as a bridge between the client and the adaptee, ensuring the client can use the functionality without needing to understand the specifics of the adaptee.
Essentials at a Glance to Multilevel Adapter Patterns
Multilevel Adapter Patterns extend the basic concept of the Adapter Pattern by introducing multiple layers of adapters. They are particularly useful in systems where you may need to integrate diverse components with varying levels of abstraction.
For instance, consider system integration scenarios in microservices architecture where different services expose varying APIs. Here, a multilevel adapter can serve as an abstraction that simplifies interaction with these services.
Example Scenario
- Service A: Provides a JSON API.
- Service B: Exposes a SOAP API.
You may require an adapter for each service as well as an overarching adapter to present a unified interface to the client.
Challenges of Multilevel Adapters
While multilevel adapters can simplify interaction with multiple interfaces, they introduce several complexities.
-
Increased Code Complexity: More layers of adapters can lead to confusing relationships between classes. As the number increases, tracing through the layers can become difficult.
-
Performance Overhead: Each layer requires method invocations, which can accumulate overhead and affect performance, especially if the adapters perform additional processing.
-
Harder to Test: Testing becomes arduous with multiple layers, as you often have to mock or stub several components.
-
Difficulties in Maintenance: As you add or change services, updating several adapters can lead to a cascading effect of changes, potentially introducing bugs.
Example of Multilevel Adapter Pattern
To clarify how multilevel adapter patterns are structured, let’s create a more comprehensive example involving two adapters.
Base Interfaces
// Base interface for Service A
interface ServiceA {
String getData();
}
// Base interface for Service B
interface ServiceB {
String retrieveData();
}
Adaptee Implementations
// Concrete implementation of Service A
class ServiceAImpl implements ServiceA {
@Override
public String getData() {
return "Data from Service A";
}
}
// Concrete implementation of Service B
class ServiceBImpl implements ServiceB {
@Override
public String retrieveData() {
return "Data from Service B";
}
}
Adapters
// Adapter for Service A
class ServiceAAdapter implements ServiceB {
private ServiceA serviceA;
public ServiceAAdapter(ServiceA serviceA) {
this.serviceA = serviceA;
}
@Override
public String retrieveData() {
// Converting Service A's response to Service B's expected format
return "Adapted: " + serviceA.getData();
}
}
// Adapter for Service B
class ServiceBAdapter implements ServiceA {
private ServiceB serviceB;
public ServiceBAdapter(ServiceB serviceB) {
this.serviceB = serviceB;
}
@Override
public String getData() {
// Converting Service B's response to Service A's expected format
return "Adapted: " + serviceB.retrieveData();
}
}
Client Code
public class MultiAdapterClient {
public static void main(String[] args) {
ServiceA serviceA = new ServiceAImpl();
ServiceB serviceB = new ServiceBImpl();
ServiceB adaptedServiceA = new ServiceAAdapter(serviceA);
ServiceA adaptedServiceB = new ServiceBAdapter(serviceB);
System.out.println(adaptedServiceA.retrieveData()); // Output: Adapted: Data from Service A
System.out.println(adaptedServiceB.getData()); // Output: Adapted: Data from Service B
}
}
In this example, we create two services and define an adapter for each so that they can communicate with their opposite types. Thus, we have a clean abstraction where the client interacts without concern for the underlying implementations.
Tips for Managing Complexity
-
Centralized Documentation: Document the relationship between adapters to provide clear guidelines on how they relate to each other.
-
Layered Architecture: Ensure that each adapter layer has a clearly defined purpose, separating responsibilities as much as possible.
-
Use Interfaces: Interfaces can help limit coupling between classes. Only expose necessary methods in your adapters.
-
Testing Strategies: Adopt strategies like the Template Method or Strategy patterns for testing adapters, enabling you to isolate components better.
-
Performance Metrics: Monitor performance to ensure that the overhead introduced by adapters does not adversely impact the application.
Final Considerations
Navigating the complexities introduced by the multilevel adapter pattern need not be a daunting task. By recognizing the intricacies involved and implementing effective strategies for management, we can harness the benefits of adapters effectively. The power of design patterns, when utilized with an understanding of their strengths and weaknesses, can lead to robust and adaptable software architecture.
For more profound insights into adapter patterns and their applications, you may explore resources such as Refactoring Guru or dive into the official Java Tutorials that cover more advanced topics in Java development.
Happy coding!
Checkout our other articles