Mastering Waste Management: Common Garbage Collector Mistakes
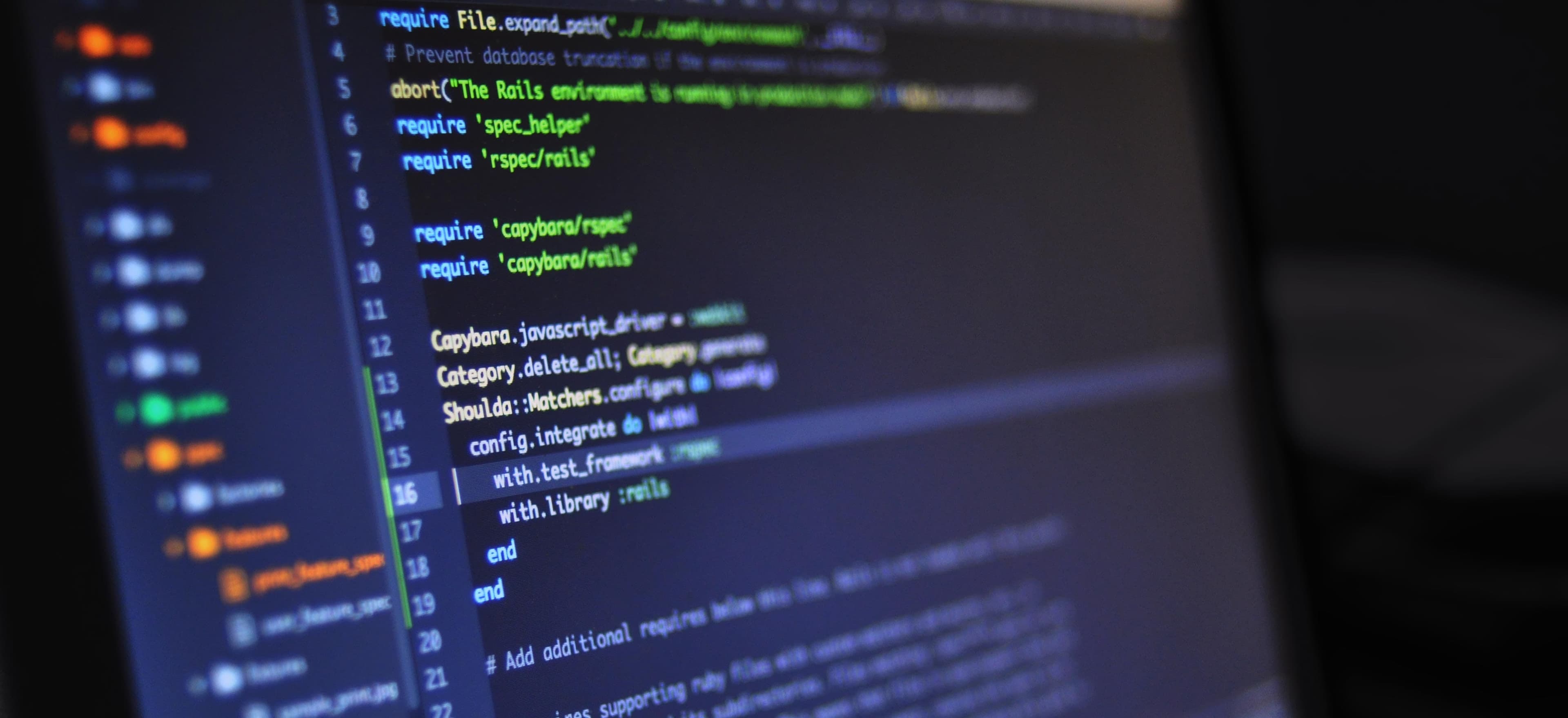
- Published on
Mastering Waste Management: Common Garbage Collector Mistakes in Java
Garbage collection is one of the core functionalities of Java that makes life easier for developers. It frees up memory that is no longer in use, allowing programmers to focus on building great software without needing to manage memory manually. However, misunderstandings and common mistakes related to garbage collection can lead to performance issues and application crashes. In this article, we will explore these mistakes, how to identify them, and best practices for effective garbage collection management.
Understanding Garbage Collection in Java
Before delving into common mistakes, it’s essential to understand what the Garbage Collector (GC) does. Java’s GC automatically recycles memory, preventing memory leaks and reducing the chances of OutOfMemoryError
. However, it’s not infallible, and errors can happen if developers don’t grasp how it operates.
Key Concepts of Garbage Collection
-
Heap Memory: The area where Java objects reside. It is divided into Young Generation (for new objects) and Old Generation (for long-lived objects).
-
Garbage Collector Types:
- Serial GC: Best for small applications.
- Parallel GC: Good for maximizing throughput.
- Concurrent Mark-Sweep (CMS): Minimizes pause times for applications requiring responsiveness.
- G1 GC: Designed for large applications needing predictable response time.
Understanding these aspects will give you a solid foundation for identifying mistakes.
Common Garbage Collector Mistakes
Mistake 1: Ignoring the Garbage Collection Logs
One of the most common mistakes is not examining the garbage collection logs. Garbage collection logs provide insights into how memory is allocated and reclaimed, identifying when and how often the GC is triggered.
How to fix it: Use the -Xlog:gc*
option to enable detailed GC logging.
java -Xlog:gc*:file=gc.log -jar YourApplication.jar
With this log, you will see detailed information about each GC event.
Mistake 2: Underestimating the Impact of Object Creation
Creating too many temporary objects can lead to performance degradation. The GC is invoked more frequently when many short-lived objects are generated, leading to increased pause times.
Solution: Consider using object pooling or existing objects over creating new ones when possible.
Example of Object Pooling:
public class ObjectPool {
private List<MyObject> available = new ArrayList<>();
private int maxSize = 10;
public MyObject acquire() {
if (available.isEmpty() && available.size() < maxSize) {
available.add(new MyObject());
}
return available.remove(available.size() - 1);
}
public void release(MyObject obj) {
available.add(obj);
}
}
Here, ObjectPool
reduces the need to repeatedly create new MyObject
instances.
Mistake 3: Misunderstanding Reference Types
Java has four types of references: strong, weak, soft, and phantom. A misunderstanding of these reference types can lead to unintended memory retention or premature object deallocation.
Solution: Understand when to use which reference.
- Strong Reference: Default reference type; prevents GC.
- Weak Reference: Used for caches; allowed to be collected.
- Soft Reference: Held until memory is needed—ideal for memory-sensitive caching.
- Phantom Reference: Used to determine when an object has been collected.
Utilizing soft or weak references for cached objects can help manage memory more effectively.
Mistake 4: Not Tuning the JVM Options
Every application has unique needs. If the default JVM settings for garbage collection are used without adjustment, performance can suffer.
Solution: Tune JVM options such as -Xms
, -Xmx
, and GC settings to suit your application’s needs.
Example:
java -Xms512m -Xmx4g -XX:+UseG1GC -jar YourApplication.jar
In this case, we define a minimum heap size of 512MB and a maximum of 4GB with G1 GC enabled. Adjust these settings based on your application’s performance metrics.
Mistake 5: Neglecting to Upgrade Java Version
Java releases frequently include performance improvements to the GC algorithms. Sticking with an outdated Java version may result in missed optimizations.
Solution: Regularly update your Java version to leverage advancements in garbage collection.
Additional Best Practices
-
Avoid Memory Leaks: Always ensure that long-lived objects do not hold references to short-lived objects which could lead to memory not being reclaimed.
-
Profile Your Application: Use profiling tools like VisualVM or Java Mission Control to observe memory utilization and GC behavior.
-
Minimize Use of Finalizers: Finalizers can lead to longer GC pauses, which affect application performance.
Bringing It All Together
Garbage collection in Java may seem automatic and foolproof, but it's crucial to understand its intricacies to avoid common pitfalls. By observing GC logs, managing object creation, understanding reference types, tuning JVM settings, and staying updated with Java releases, you can effectively master waste management in your Java applications.
If you would like to dive deeper into garbage collection and performance tuning, consider checking out the following resources:
- Oracle's Garbage Collection Tuning Guide
- Java Performance: The Definitive Guide
By implementing these best practices, you'll not only improve performance but also create more efficient, memory-conscious Java applications. Happy coding!
Checkout our other articles