Struggling with DAO Complexity? Generics to the Rescue!
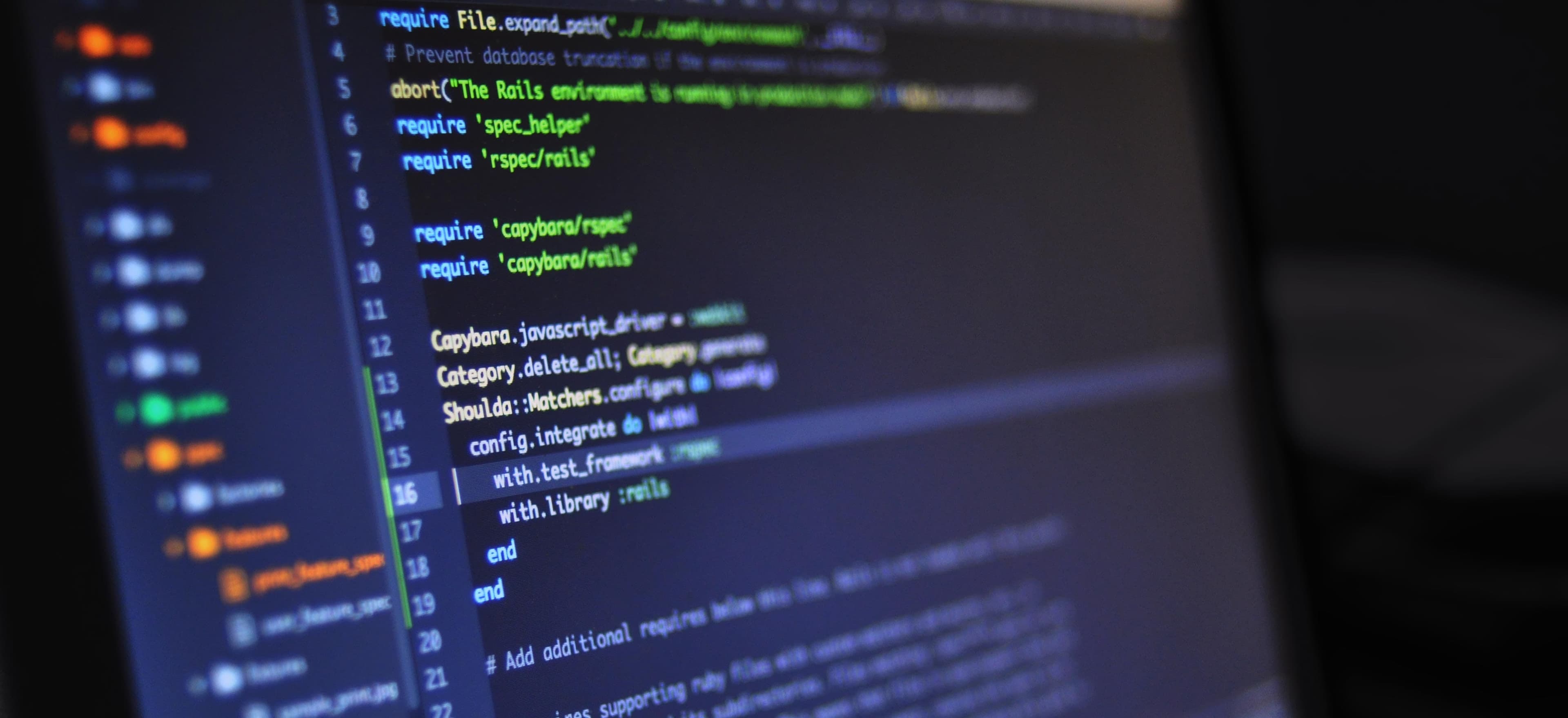
- Published on
Struggling with DAO Complexity? Generics to the Rescue!
In the realm of software development, the Data Access Object (DAO) pattern is a crucial architectural design pattern. It serves as an abstraction for database interactions, allowing developers to manipulate data objects without having to deal with the complexities inherent in different data sources. However, while DAOs streamline data access, they can become cumbersome due to the complexity of managing data types. This is where Java Generics can come to the rescue, providing a more flexible and type-safe way to handle data across various entities.
What is a DAO?
Before diving into the technical aspects of using Generics in DAOs, let's clarify what a DAO is. The DAO pattern provides a way to abstract and encapsulate all access to the data source. A DAO typically includes methods for creating, reading, updating, and deleting (CRUD) records. By separating data access logic from the business logic, you’re left with a cleaner, more maintainable codebase.
Example of a Traditional DAO
Here’s a simple implementation of a traditional DAO for a User
entity:
public class UserDAO {
public void create(User user) {
// Logic to save user to database
}
public User read(int id) {
// Logic to retrieve user from database by ID
return new User(); // Placeholder
}
public void update(User user) {
// Logic to update user in the database
}
public void delete(int id) {
// Logic to delete the user from the database
}
}
While this structure works, it has one significant shortcoming: it’s tightly coupled to the User
type. If you need a similar DAO for different types of entities, you’ll have to duplicate or adjust the code for each one, leading to increased complexity.
The Role of Generics
Java Generics allow us to write a single DAO class that can work with any entity type while maintaining type safety. Instead of being coupled to a single type, you can define a generic type parameter that allows flexibility.
Benefits of Using Generics in DAOs
- Code Reusability: You write a generic DAO once and can reuse it for multiple entities.
- Type Safety: By using Generics, you benefit from compile-time type checking, which reduces runtime errors.
- Cleaner Code: A single, generic DAO implementation makes your codebase more maintainable.
Implementing a Generic DAO
Let’s refactor our UserDAO
to make it a generic DAO.
Step 1: Create a Generic DAO Interface
First, define a generic interface for your DAO:
public interface GenericDAO<T, ID> {
void create(T entity);
T read(ID id);
void update(T entity);
void delete(ID id);
}
Step 2: Implement the Generic DAO Interface
Now, let’s implement it:
import java.util.HashMap;
import java.util.Map;
public class GenericDAOImpl<T, ID> implements GenericDAO<T, ID> {
private Map<ID, T> datastore = new HashMap<>();
@Override
public void create(T entity) {
// Store entity logic (using ID)
}
@Override
public T read(ID id) {
// Logic to retrieve entity by ID
return datastore.get(id);
}
@Override
public void update(T entity) {
// Logic to update entity (using ID)
}
@Override
public void delete(ID id) {
// Logic to delete entity by ID
datastore.remove(id);
}
}
Commentary on Implementation
In the above code snippet, we used a Map
to simulate a simple in-memory datastore. While this is a stubbed example, the core functionality highlights how we can encapsulate CRUD operations for any generic type T
identified by an ID of type ID
.
Step 3: Creating a Specific DAO
Now, let’s create a specific DAO for User
using our generic DAO:
public class UserDAO extends GenericDAOImpl<User, Integer> {
@Override
public void create(User user) {
// Add logic to assign user ID, including validation if necessary.
super.create(user);
}
@Override
public User read(Integer id) {
// Add any extra logic specific to reading User if needed
return super.read(id);
}
// You can override other methods if specific logic is needed.
}
Real-World Use Cases
When using the Generic DAO pattern, consider cases such as:
- Multiple entities: You can implement DAOs for
Product
,Order
, or any other type in a consistent manner. - Unit Testing: Having a generic DAO makes it much easier to mock your database interactions during unit testing, simplifying the entire testing process.
For further exploration, you might want to read up on Data Access Object Pattern in Java to get more insight into best practices.
Best Practices with Generic DAOs
- Limit Generic Types: Only extend the functionality when necessary. Overuse of Generics can lead to less readable code.
- Enforce Constraints: You may use bounded types to restrict the types you can use as parameters.
- Logging Errors: Always ensure you log repository errors that might occur during data access for easier debugging and maintenance.
The Last Word
Using Generics in your DAOs can drastically reduce the complexity of your data access layer while promoting maintainability and reusability. By adopting a generic approach, not only do you simplify your codebase, but you also pave the way for robust, type-safe data handling in your applications.
Feel free to dive into Java's Generics Documentation for an in-depth understanding of generics and unlock their full potential in your Java projects.
With this approach, you'll be well on your way to mastering DAOs with the flexibility of Generics, ensuring your code is both powerful and elegant.
Checkout our other articles