Why Java 9's New Features Might Not Impact Your Workflow
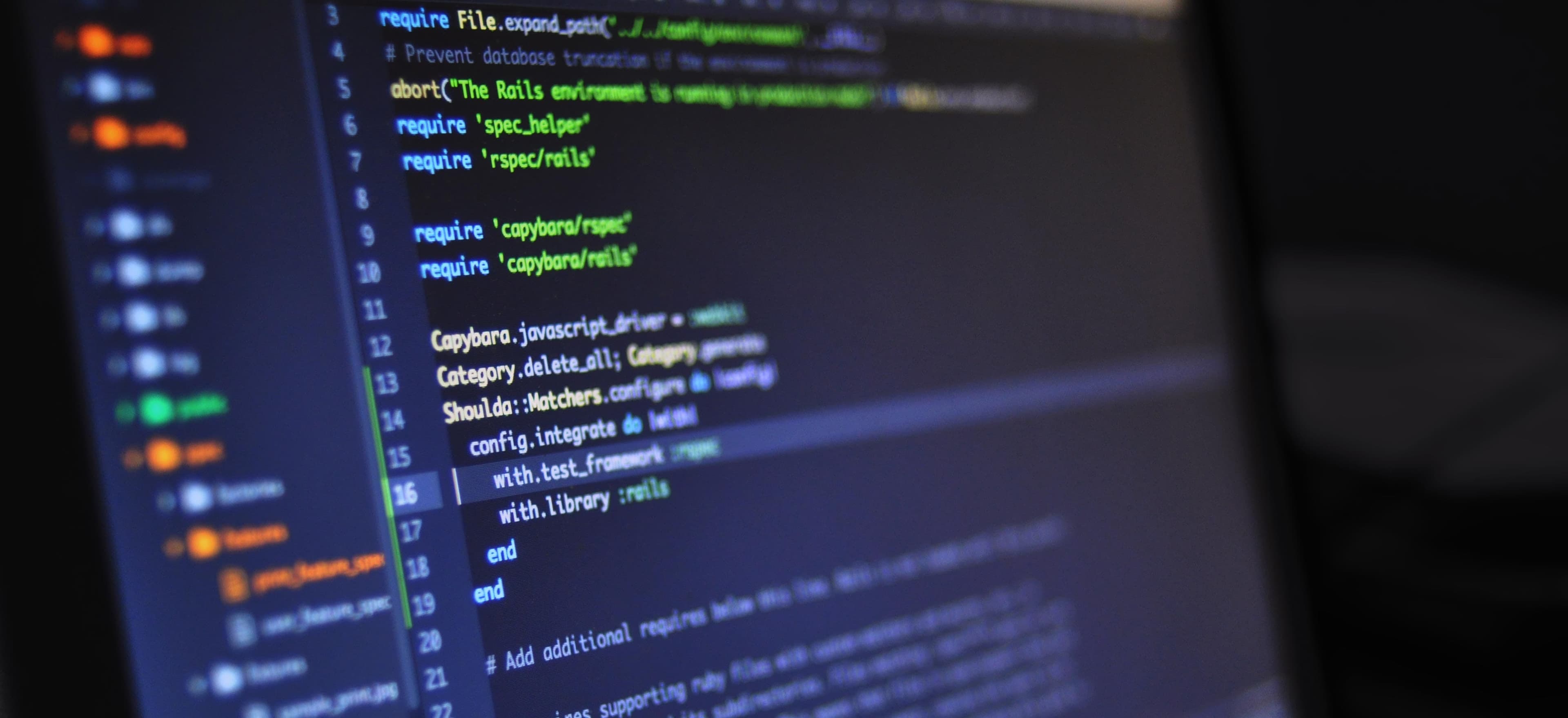
- Published on
Why Java 9's New Features Might Not Impact Your Workflow
Java has long been a staple in the world of programming, revered for its platform independence and extensive libraries. As the Java community continually evolves, new versions often bring exciting features that promise to enhance development capabilities. With the release of Java 9, the spotlight was on several key features aimed at improving performance and usability. However, you might be wondering whether these updates genuinely influence your everyday workflow. This blog post will explore the new features of Java 9, discussing their significance and why they may or may not impact your actual work.
A Quick Overview of Java 9 Features
Here are some of the most notable features that came with Java 9:
- Modular System (Project Jigsaw)
- JShell: The Java Shell
- Improved Stream API
- Private Methods in Interfaces
- Enhanced @Deprecated Annotation
- New HTTP/2 Client
Each of these features provides developers with new tools and processes, but how do they translate into practical usage? Let’s dive into each one.
1. Modular System (Project Jigsaw)
The most acclaimed feature of Java 9 is its modular system, also known as Project Jigsaw. This modularization allows developers to create more maintainable and scalable applications.
Example Code
module com.example.hello {
exports com.example.hello;
}
Commentary
Creating modules involves defining a module descriptor in a file named module-info.java
. This descriptor lists packages to be exported and required modules. The advantages include:
- Encapsulation: Only specified packages are visible to other modules.
- Reduced Footprint: Applications become smaller and faster since only required modules are loaded.
Why It Might Not Impact Your Workflow: If you work on smaller applications or maintain legacy code written in earlier Java versions, the modular system may not be immediately applicable. Migrating existing projects to a modular structure can require significant time and effort, causing reluctance to adopt this feature. Furthermore, many organizations maintain a traditional monolithic style of development.
2. JShell: The Java Shell
JShell is an interactive tool for quickly testing Java code snippets. It allows for a more exploratory programming style, enabling developers to iterate and refine ideas rapidly.
Example Code
jshell> int x = 10
jshell> int y = 20
jshell> x + y
Commentary
In a console application, JShell allows you to execute snippets without needing to encapsulate them inside a class or method. You can immediately see results, which fosters rapid prototyping.
Why It Might Impact Your Workflow: JShell is great for learners or those looking to experiment with code quickly. However, if your primary development revolves around established codes and frameworks, the benefits might be less pronounced.
3. Improved Stream API
Java 9 enhances the Stream API by adding new methods that simplify operations like creating streams from various sources and accumulating elements.
Example Code
List<String> list = Arrays.asList("A", "B", "C", "D");
Stream<String> stream = list.stream();
stream.takeWhile(s -> s.compareTo("C") < 0)
.forEach(System.out::println);
Commentary
The takeWhile
method allows you to process elements while a condition is met. This can lead to cleaner and more efficient code.
Why It Might Impact Your Workflow: If you frequently work with complex data transformations and collections, these enhancements can streamline your work. However, for simpler projects, existing stream methods may suffice.
4. Private Methods in Interfaces
Java 9 introduces private methods in interfaces that help to reduce code duplication among default methods.
Example Code
interface MyInterface {
default void defaultMethod() {
privateMethod();
}
private void privateMethod() {
System.out.println("This is a private method.");
}
}
Commentary
These private methods can be utilized to encapsulate logic that is shared between default methods, making your code cleaner and more maintainable.
Why It Might Not Impact Your Workflow: If you mostly implement straightforward interfaces, this feature may seem superfluous. It becomes beneficial in larger systems where interfaces grow in complexity, leading to potential bloating and code reuse.
5. Enhanced @Deprecated Annotation
Java 9 improved the @Deprecated
annotation with more descriptive messages, allowing developers to specify the reason and suggest alternatives.
Example Code
@Deprecated(since = "9", forRemoval = true)
public void oldMethod() {
// Old implementation
}
Commentary
This makes it easier for other developers to understand the reason behind deprecating a method and what alternatives are available.
Why It Might Impact Your Workflow: If you’re part of a large team or maintaining public APIs, these enhancements can improve communication and code quality. However, if your environment is small or predominantly internal, the value may not be as pronounced.
6. New HTTP/2 Client
Java 9 introduces a new HttpClient
API supporting HTTP/2, providing a modern approach to handle HTTP requests.
Example Code
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://example.com"))
.build();
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println);
Commentary
This API is designed for asynchronous and reactive programming models, boosting the efficiency of network operations.
Why It Might Not Impact Your Workflow:
If your work relies on libraries that abstract HTTP request handling or if you are already satisfied with your current setup, transitioning to the new HttpClient
may not be a priority.
The Last Word
Java 9 introduced a plethora of exciting features that can revolutionize software development in many ways. However, the extent to which these features impact your daily workflow largely depends on your development context, team structure, and the nature of your projects.
Adopting new technologies often entails a learning curve and shifts in workflow processes. While some of Java 9's features may offer significant improvements, others might cater to specific programming paradigms or project types.
As you continue to evolve your programming skills, evaluate when and where to implement these features based on your project necessities. Adapting to every change in the Java landscape isn’t always feasible, but being informed gives you the power to enhance your development process strategically.
Additional Resources
- Getting Started with Modular Programming
- Official Java Documentation on JShell
- Stream API Improvements in Java 9
By staying aware of these advancements, you ensure that your development practices remain relevant and efficient—balance is key!