Overcoming Challenges in JUnit 5 Custom Execution Conditions
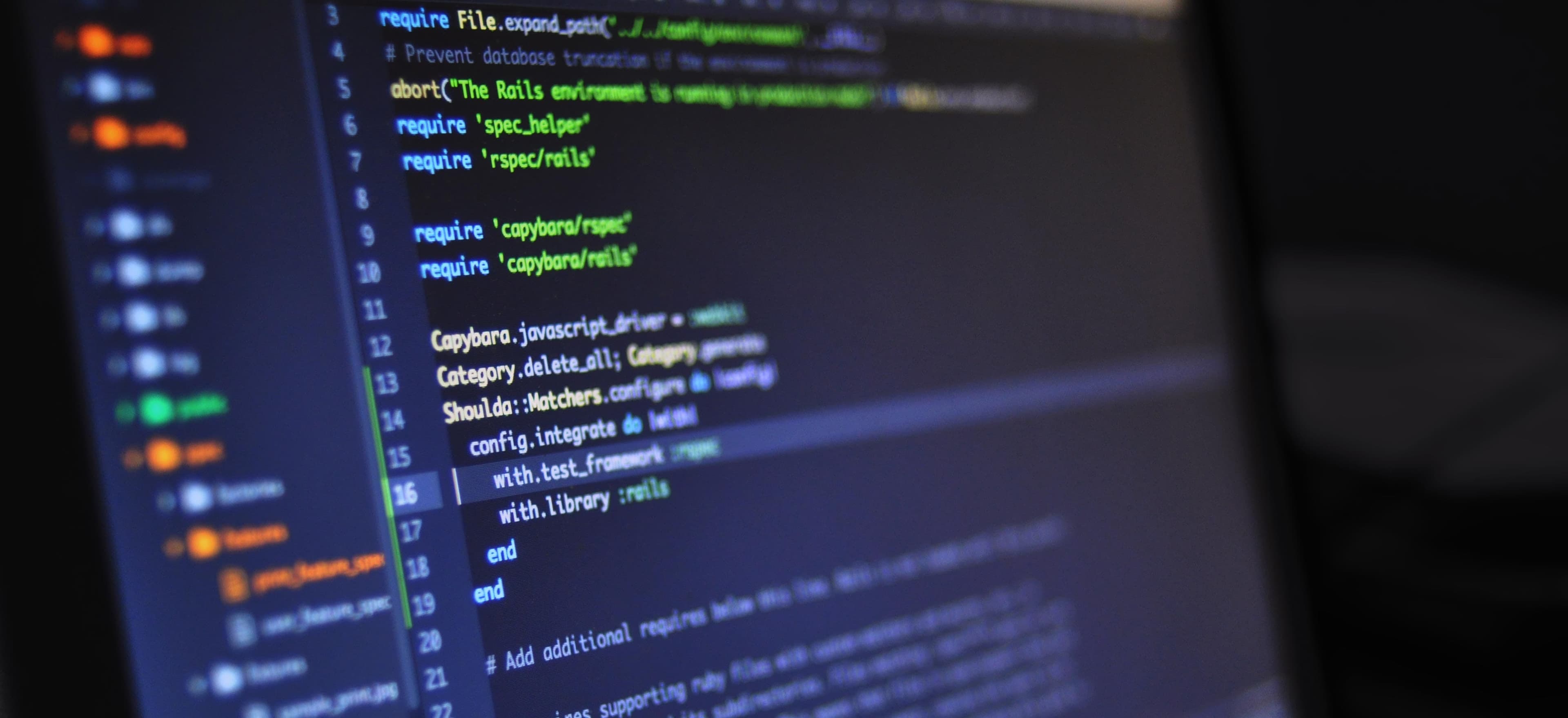
- Published on
Overcoming Challenges in JUnit 5 Custom Execution Conditions
In the realm of software development, testing is a crucial component for guaranteeing the quality and functionality of applications. JUnit, one of the most widely used testing frameworks in Java, has evolved significantly with its fifth iteration (JUnit 5). Among its advancements is the introduction of custom execution conditions, which allow you to dictate when tests should run based on specific criteria. While this feature grants developers greater flexibility, it may also present challenges. In this blog post, we will explore how to create custom execution conditions in JUnit 5, the potential challenges one might face, and, importantly, how to overcome them.
Understanding JUnit 5 Execution Conditions
JUnit 5 introduces the concept of Extension which allows developers to create reusable functionalities. One important type of extension is the ExecutionCondition, which determines if a test should execute. By implementing the ExecutionCondition
interface, you can create a custom condition that checks whether a test should run or be skipped based on runtime criteria.
Why Use Custom Execution Conditions?
Custom execution conditions become particularly beneficial in scenarios where:
- You have tests that are dependent on external features (like an API).
- Certain tests should only be executed on specific environments (development, testing, production).
- You want to skip tests based on configuration settings.
Creating a Custom Execution Condition
Let's start with the basics of creating a custom execution condition in JUnit 5. Below is a simple example that demonstrates how to skip tests based on the presence of a configuration property.
Step 1: Implementing the Custom Condition
import org.junit.jupiter.api.condition.EnabledIf;
import org.junit.jupiter.api.extension.ExecutionCondition;
import org.junit.jupiter.api.extension.ExtensionContext;
import java.lang.reflect.Field;
public class ConfigEnabledCondition implements ExecutionCondition {
@Override
public ConditionEvaluationResult evaluateExecutionCondition(ExtensionContext context) {
try {
// Access a specific configuration field
Class<?> testClass = context.getRequiredTestClass();
Field field = testClass.getDeclaredField("ENABLE_TEST");
field.setAccessible(true);
boolean isEnabled = field.getBoolean(null);
if (isEnabled) {
return ConditionEvaluationResult.enabled("Test is enabled based on configuration.");
} else {
return ConditionEvaluationResult.disabled("Test is disabled based on configuration.");
}
} catch (NoSuchFieldException | IllegalAccessException e) {
return ConditionEvaluationResult.disabled("Configuration field not accessible.");
}
}
}
Commentary
-
Reflection Usage: The
ConfigEnabledCondition
class retrieves the value of theENABLE_TEST
field using reflection. Though powerful, reflection can introduce challenges such as decreased performance and potential security concerns, so it should be used judiciously. -
Condition Evaluation: Depending on whether the condition is met (i.e., the field is true), it returns either
enabled
ordisabled
. It’s essential to provide meaningful messages to facilitate debugging.
Step 2: Applying the Execution Condition
Once you define your custom execution condition, you can use it in your test classes like so:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.condition.EnabledIf;
public class MyServiceTest {
public static final boolean ENABLE_TEST = true; // Modify this to control test execution
@Test
@EnabledIf("io.myapp.ConfigEnabledCondition") // Use the condition
void testServiceFunctionality() {
// Your test logic here
}
}
In this code:
- The test
testServiceFunctionality
will only run ifENABLE_TEST
is set totrue
. Otherwise, it will be skipped.
Challenges and Solutions
While the approach outlined above seems straightforward, several challenges can arise:
Challenge 1: Understanding the Lifecycle
JUnit 5 has a diverse lifecycle for tests. Implementing custom execution conditions might introduce confusion if not used correctly.
Solution: Study the Lifecycle Events
Familiarize yourself with JUnit 5 lifecycle events. Knowing when your conditions are evaluated helps in deciding where to implement your logic effectively.
Challenge 2: Performance Concerns
Using reflection can slow down your tests if overused or misapplied.
Solution: Minimize Reflection Usage
Use reflection only when absolutely necessary. Where possible, consider alternative methods such as configuration management libraries (like Spring's @Value
or MicroProfile’s config).
Challenge 3: Complexity and Readability
Custom conditions can complicate test readability, causing confusion among team members primarily unfamiliar with the code.
Solution: Documentation and Naming Conventions
Document your conditions thoroughly. Use clear and intuitive names for your conditions that describe their purpose.
Additional Examples
Conditional Test for Environment-Based Execution
Here’s another real-world scenario where you want tests to run only in a specific environment, e.g., only in a production-like environment.
import org.junit.jupiter.api.extension.ExecutionCondition;
import org.junit.jupiter.api.extension.ExtensionContext;
public class ProductionOnlyCondition implements ExecutionCondition {
@Override
public ConditionEvaluationResult evaluateExecutionCondition(ExtensionContext context) {
String env = System.getProperty("app.environment", "dev");
if ("prod".equalsIgnoreCase(env)) {
return ConditionEvaluationResult.enabled("Running in production environment.");
} else {
return ConditionEvaluationResult.disabled("Not in production environment.");
}
}
}
Applying ProductionBased Condition
@Test
@ExtendWith(ProductionOnlyCondition.class)
void testOnlyInProduction() {
// Test logic that should run only in prod
}
Wrapping Up
Custom execution conditions in JUnit 5 offer a powerful way to manage test executions based on various criteria. While they hold potential for flexibility, they can introduce challenges in terms of performance, complexity, and lifecycle understanding.
By following best practices and leveraging effective documentation, you can mitigate these challenges, creating tests that are both dynamic and reliable. Custom execution conditions empower us to run our tests more intelligently, ensuring that they are both relevant and contextually appropriate.
If you want to dive deeper into JUnit 5, consider checking out the official JUnit 5 User Guide for more insights on extensions and testing techniques.
In case you find yourself grappling with JUnit 5 configurations or wish to share your experiences regarding challenges faced with execution conditions, feel free to add your comments below! Happy testing!
Checkout our other articles