Mastering Spring Boot: Change Default Port Like a Pro
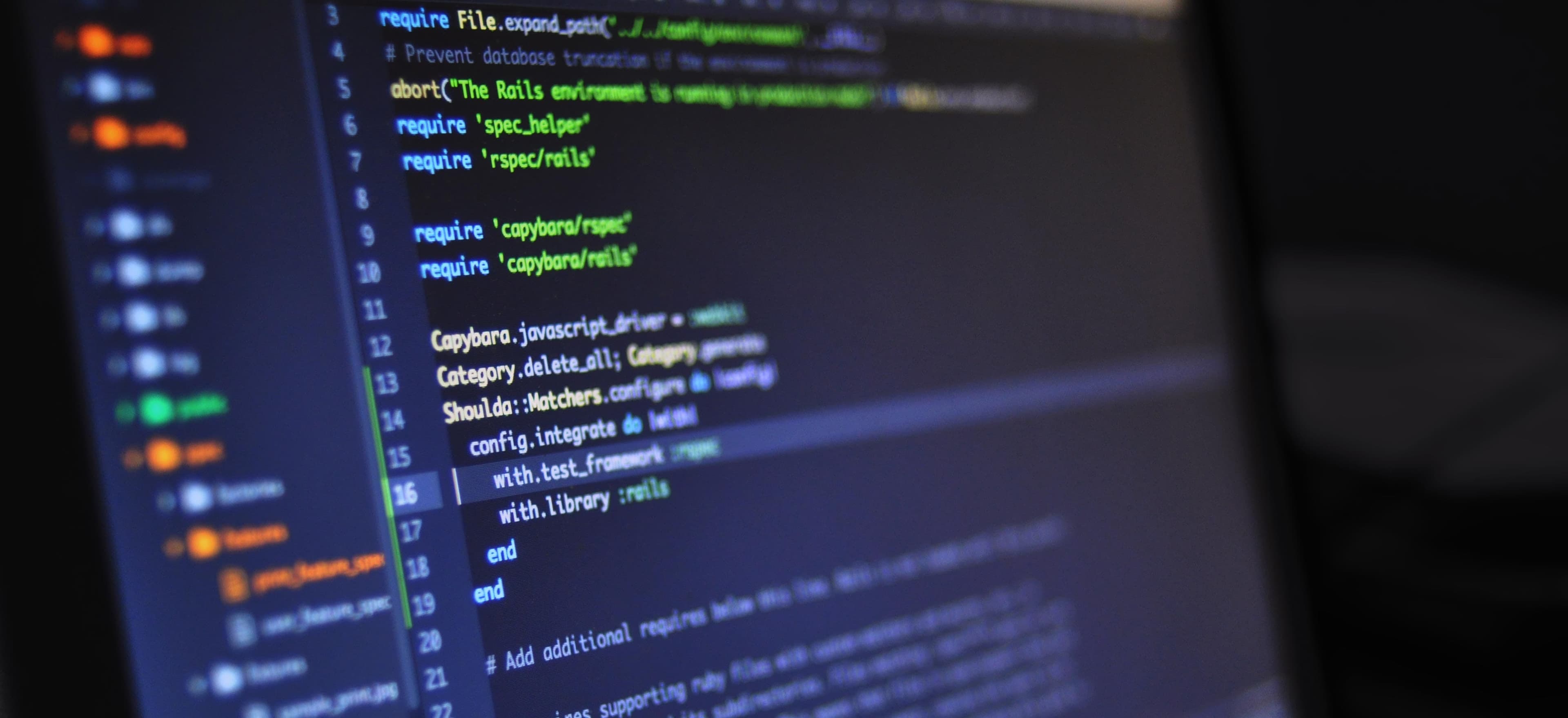
- Published on
Mastering Spring Boot: Change Default Port Like a Pro
Spring Boot has revolutionized Java development, making it faster and easier to build production-ready applications. One of the common configurations developers often need to adjust is the server port. By default, Spring Boot applications run on port 8080. However, in many cases, you might want to change the default port for various reasons, such as avoiding port conflicts, aligning with organizational standards, or custom development setups.
In this blog post, we will explore several methods for changing the default port in a Spring Boot application. We'll focus on practical implementation and share code snippets with detailed explanations.
Why Change the Default Port?
Before diving into the methods, it's crucial to understand why changing the default port can be beneficial:
- Avoid Port Conflicts: If another application is already running on port 8080, changing the port avoids conflicts and ensures your application runs smoothly.
- Development Flexibility: Different projects may have different requirements or dependency setups that necessitate using a specific port.
- Production Configurations: In a production environment, the standard practices often require using specific ports for applications for security or monitoring purposes.
Methods to Change the Default Port
1. Application Properties
The simplest way to change the default port in a Spring Boot application is by modifying the application.properties
file found in the src/main/resources
directory.
# Set the server port to 9090
server.port=9090
Why This Works: Spring Boot automatically loads configuration properties from the application.properties
file, and the server.port
property tells Spring Boot to listen on port 9090 instead of the default 8080.
2. Application YAML
If you prefer to use YAML (YAML Ain't Markup Language) instead of properties, you can achieve the same by using the application.yml
file:
server:
port: 9090
Why Use YAML: YAML provides a more readable structure, especially when you have nested properties. It also allows you to group related configurations together.
3. Command Line Arguments
You can also set the port when running your Spring Boot application using command-line arguments. This can be especially useful in CI/CD pipelines or when you want to override configuration dynamically:
java -jar your-app.jar --server.port=9090
Why Command Line: This approach prioritizes flexibility. You can specify different ports without changing any files, making it perfect for varying environments such as development, testing, and production.
4. Environment Variables
Another powerful way to change the server port is via environment variables. This method is particularly useful in containerized environments, such as with Docker:
export SERVER_PORT=9090
In your application.properties
, you can use the following configuration to reference the environment variable:
server.port=${SERVER_PORT:8080}
Why Environment Variables: They enable configuration management and secret handling best practices. In production environments, it's often necessary to keep sensitive information out of the codebase, making this method very clean.
5. Programmatic Configuration
If you need more complex logic to determine the port (such as reading it from a database or an external configuration service), you can configure it programmatically:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.boot.web.embedded.tomcat.ConfigurableTomcatWebServerFactory;
import org.springframework.boot.web.server.WebServerFactoryCustomizer;
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@Bean
public WebServerFactoryCustomizer<ConfigurableTomcatWebServerFactory> serverPortCustomizer(ConfigurableEnvironment env) {
return factory -> {
String port = env.getProperty("SERVER_PORT", "8080");
factory.setPort(Integer.parseInt(port));
};
}
}
Why Programmatic Configuration: This method offers maximum flexibility and is especially useful for applications that need to adapt configurations based on various conditions or application states.
6. Using the Spring Cloud Config Server
In more complex microservices architectures, using a configuration management system, such as Spring Cloud Config Server, can simplify managing properties shared across multiple applications. You can externalize the configuration and update the port there.
Why Use Spring Cloud Config: It centralizes configuration management across various environments and eliminates duplication of properties across multiple applications.
Testing the Port Change
After implementing any of the above methods, it is essential to test if the port has been changed successfully. Run your Spring Boot application and navigate to http://localhost:9090
in your web browser. You can check the server logs to confirm that your application is running on the configured port.
A Final Look
Knowing how to change the default port in a Spring Boot application is a fundamental skill that can make your development process much smoother. Choosing the right method depends on your project's needs and development practices. Whether you prioritize simplicity, flexibility, or organization, there's a suitable option for everyone.
If you're interested in learning more about Spring Boot, check out the official Spring Boot documentation for further guidance and resources.
By mastering these configurations, you can ensure your applications are well-optimized and tailored to fit your specific development environment.
Happy coding!
Checkout our other articles