Debugging Common Lambda Expression Errors in Java 8
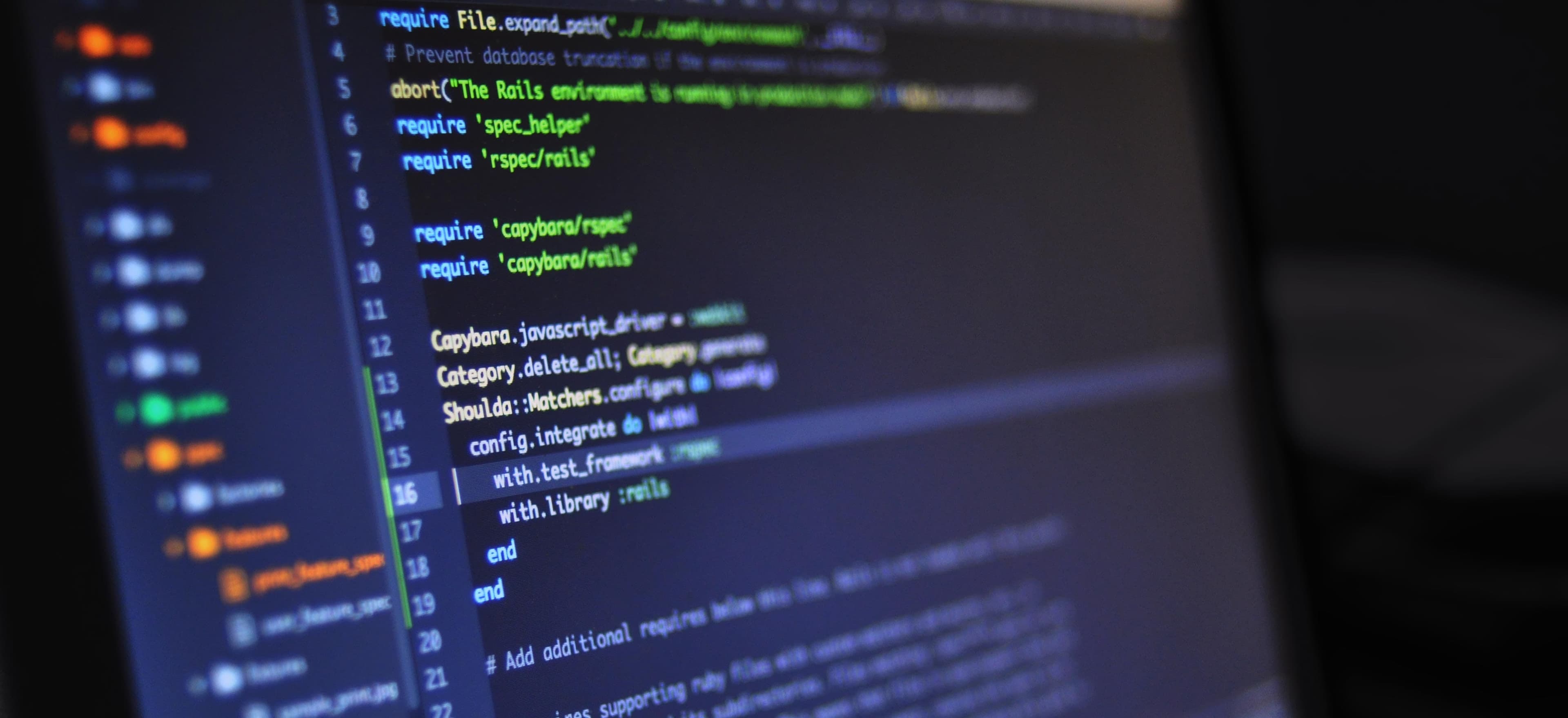
- Published on
Debugging Common Lambda Expression Errors in Java 8
Java 8 broke grounds by introducing lambda expressions, granting developers a streamlined way to write functions, particularly when dealing with collections and event handling. However, with great power comes great responsibility—or in this case, great potential for confusion. In this blog post, we'll delve into the common lambda expression errors encountered by Java developers, and more importantly, how to debug and resolve them effectively.
Understanding Lambda Expressions
Lambda expressions provide a clear and concise way to represent functional interfaces (interfaces with a single abstract method) using an expression instead of an anonymous class. Here’s a quick example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach(name -> System.out.println(name));
In this case, name -> System.out.println(name)
is a lambda expression that prints each name in the list.
Why Use Lambda Expressions?
- Conciseness: Reduces boilerplate code, improving readability.
- Functionality: Enhances capabilities of the Java Collections framework with a more functional programming style.
- Ease of Use: Facilitates usage with Stream API to perform bulk operations on collections.
While lambda expressions are powerful, they also come with pitfalls. Below are some common errors that developers encounter, along with practical solutions.
Common Lambda Expression Errors
1. Target Type Cannot Be Inferred
One of the frequent errors encountered is the "Target type cannot be inferred" error.
Example of the error:
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach(name -> name.length());
Why this happens: The compiler cannot determine the target type for name.length()
since it relies on context that is not provided here.
Solution: Provide context by indicating the type explicitly.
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach((String name) -> System.out.println(name.length()));
Explicitly stating the type (String name
) helps the compiler understand what is being processed, thereby eliminating the error.
2. Non-Effective Final Local Variables
Lambda expressions can capture variables from their enclosing context, but these variables must be effectively final.
Example of the error:
int a = 10;
Runnable r = () -> System.out.println(a);
a = 20; // This will cause a compilation error.
Why this happens: The lambda tries to capture a
, which has been modified after its declaration.
Solution: Use final
keyword or ensure no modification occurs post declaration.
final int a = 10;
Runnable r = () -> System.out.println(a);
3. Incompatible Types
Incompatible types error arises when the lambda expression’s return type does not match the expected type.
Example of the error:
Function<Integer, String> func = (value) -> value; // This line causes a compile-time error.
Why this happens: The expected type for func
is String
, but the lambda only returns an Integer
.
Solution: Convert the return type inside the lambda.
Function<Integer, String> func = (value) -> String.valueOf(value);
Here, you explicitly convert Integer
to String
using String.valueOf()
, ensuring type compatibility.
4. Ambiguous Method Call
When overloaded methods exist, the compiler may not know which method to use based on the lambda expression passed.
Example of the error:
public class Example {
void doSomething(String s) {
System.out.println("String: " + s);
}
void doSomething(Integer i) {
System.out.println("Integer: " + i);
}
public void test() {
// Without specifying the target type
doSomething(x -> x + 1); // Error: ambiguity
}
}
Solution: Specify the target type within the function call to eliminate ambiguity.
public void test() {
doSomething((String x) -> x + "...");
doSomething((Integer x) -> x + 1);
}
By declaring the expected types, you guide the compiler in choosing the appropriate method.
5. Using Lambdas in Async Operations
Sometimes, when adding a lambda to an asynchronous call, like a timer, you may experience unpredicted behavior or even errors.
Example of a common mistake:
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
// Do something
}
}, 1000); // This runs correctly but...
Attempting to replace the anonymous inner class with a lambda expression can inadvertently introduce synchronization issues.
Solution: Use local variables inside the lambda that are inherently thread-safe.
final String message = "Task executed!";
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
System.out.println(message);
}
}, 1000);
Ensuring variables used in lambda expressions within asynchronous contexts do not alter state inadvertently is crucial for predictable behavior.
Best Practices for Using Lambda Expressions
Knowing common errors is important, but cultivating best practices is essential to avoid these errors:
- Favor Clear Intent: Write lambda expressions that are easy to read and understand.
- Limit Scope: Use local variables effectively, making sure they are either final or effectively final.
- Utilize IDE Features: Modern IDEs like IntelliJ and Eclipse offer excellent support for identifying lambda issues before compilation. Use them to your advantage.
Closing Remarks
Java 8's lambda expressions significantly enhanced the language's expressiveness and functional programming capabilities, but they come with their own set of challenges. By understanding the common errors and following the best practices outlined in this post, developers can navigate the complexities of lambda expressions with ease.
For more in-depth analysis on functional programming in Java, you may want to explore Oracle's Java Documentation or delve deeper into Java Streams and their use with lambda expressions.
By sharing your own experiences with lambda expressions, you can contribute to a richer understanding of this essential component of Java 8. Happy coding!
Checkout our other articles