Common Pitfalls When Building Your First Serverless Function
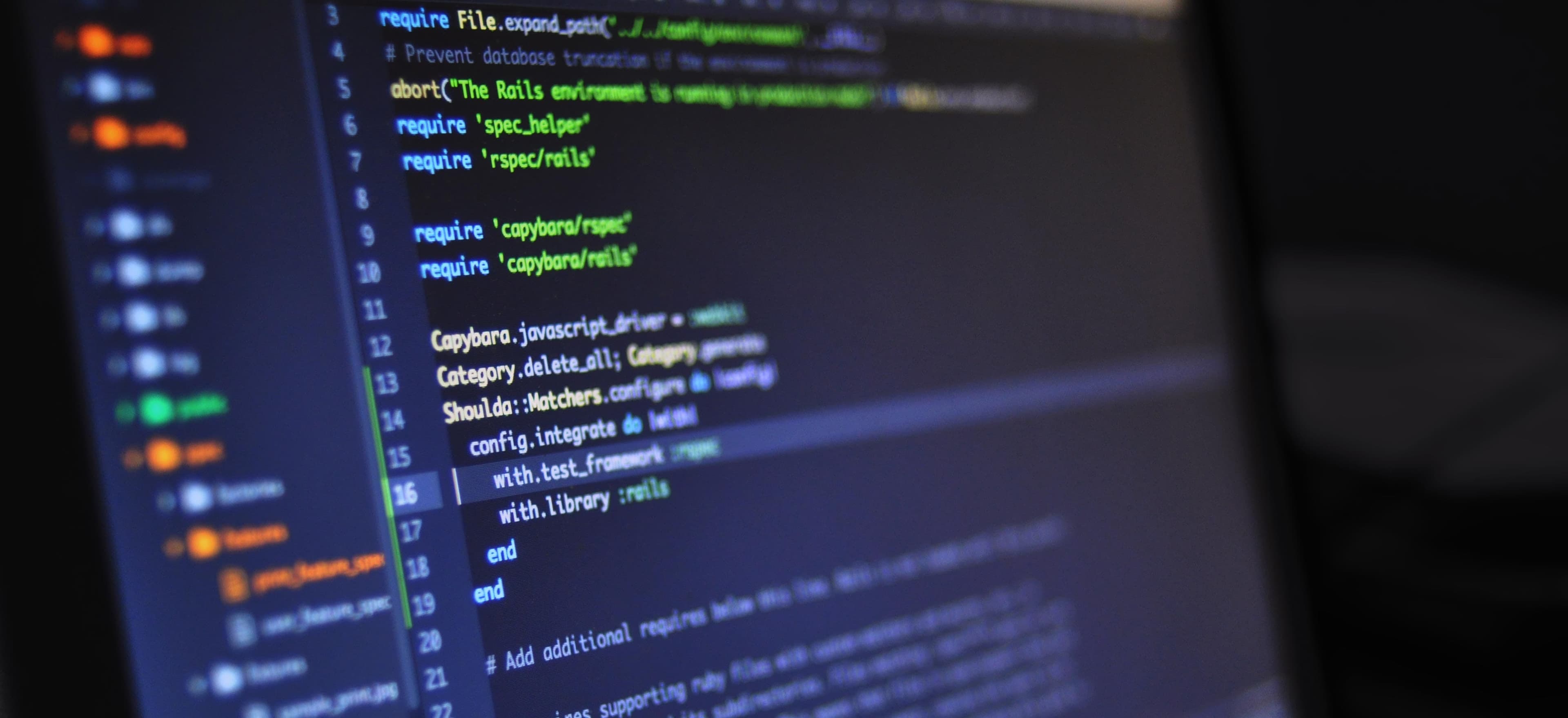
- Published on
Common Pitfalls When Building Your First Serverless Function
In recent years, serverless architecture has revolutionized the way developers build and deploy applications. It allows you to focus predominantly on writing code while abstracting away all the underlying infrastructure management. However, as with any new technology, pitfalls abound. In this blog post, we will discuss the common pitfalls when building your first serverless function, to help you navigate this exciting landscape.
What is Serverless Architecture?
Serverless computing allows you to build and run applications without needing to manage servers. Cloud providers like AWS, Azure, and Google Cloud offer services where you can deploy backend functions that automatically scale and handle events.
For instance, AWS Lambda is one of the most popular serverless offerings. You simply upload your code, configure triggers, and it runs in response to events, whether it's an HTTP request, a file being uploaded to S3, or a message published to a queue.
The Advantages of Serverless
Before diving into pitfalls, let's briefly look at the advantages:
- Cost Efficiency: You pay only for what you use, reducing costs significantly for low-traffic applications.
- Scalability: Your application can automatically scale with demand, handling spikes in traffic without pre-planning.
- Speed of Development: You can focus on writing code rather than managing the infrastructure.
With these advantages in mind, let's explore the common pitfalls you should be vigilant about.
1. Ignoring Cold Start Latency
What Is Cold Start?
A cold start occurs when a serverless function is invoked after a period of inactivity. The function takes time to initialize, resulting in latency. This can be problematic for performance-sensitive applications.
How to Mitigate It
- Keep Functions Warm: You can set up a scheduled event (e.g., a CloudWatch Event in AWS) to activate the function at regular intervals.
- Optimize Code and Dependencies: Keep the function lightweight by minimizing code and external dependencies.
// Example of a simple AWS Lambda function
exports.handler = async (event) => {
console.log("Function starting...");
// Your processing code goes here
return { statusCode: 200, body: JSON.stringify("Hello, World!") };
};
In this example, notice how we are logging the start of the function. Keeping track of such logs is vital for understanding cold start behavior.
2. Overlooking Monitoring and Logging
Monitoring and logging are crucial aspects of any application. However, when transitioning to serverless, developers often underestimate their importance.
Setting Up Monitoring
- Use Built-in Tools: Most cloud providers offer tools. For example, AWS CloudWatch provides logging and monitoring features for AWS Lambda functions.
- Custom Metrics: Incorporate metrics like execution time, invocation count, and error rates to identify performance bottlenecks.
const { CloudWatch } = require('aws-sdk');
exports.handler = async (event) => {
const cloudWatch = new CloudWatch();
const startTime = Date.now();
// Perform operations
const result = await performOperations();
const executionTime = Date.now() - startTime;
console.log(`Function executed in ${executionTime} ms`);
// Send custom metric
await cloudWatch.putMetricData({
MetricName: 'ExecutionTime',
Value: executionTime,
Unit: 'Milliseconds',
Namespace: 'MyServerlessApp',
}).promise();
return result;
};
In this code snippet, we log the execution time of the Lambda function, providing valuable information for monitoring and analysis.
3. Not Handling Errors Properly
Errors are inevitable in any software, and serverless applications can face unique issues, such as timeouts or service unavailability.
Implementing Error Handling
- Retry Logic: Implement retries for transient errors. Serverless platforms usually offer built-in mechanisms for retries based on configuration settings.
- Graceful Failures: Make sure to return meaningful responses even when errors occur.
exports.handler = async (event) => {
try {
// Your main logic goes here
} catch (error) {
console.error("Error occurred:", error);
return {
statusCode: 500,
body: JSON.stringify({ error: "Internal Server Error" }),
};
}
};
In this snippet, we have enhanced error handling to return a user-friendly message while logging the error for debugging.
4. Underestimating Cost Implications
While serverless can save you money, you might inadvertently incur high costs if your functions are inefficiently written or auto-scaling exceeds your budget.
Managing Costs
- Understand Pricing Models: Get familiar with the pricing of functions, including execution time, memory use, and requests.
- Set Up Budgets and Alerts: Use cloud tools to set budgets and alerts as a safety net.
5. Failing to Optimize Function Size
The bigger the function, the longer it takes to initialize. An oversized deployment package can lead to increased latency.
Best Practices for Optimization
- Limit Deployment Size: Minimize package size by only including necessary libraries and code.
- Use Layers: AWS Lambda allows you to use Layers to separate dependencies from your main function, which can lead to better organization and size efficiency.
# Example command to package a Lambda function
zip -r function.zip index.js node_modules
In this example, we create a zip package for an AWS Lambda function, ensuring only what's needed is included.
6. Not Planning for Security
Security often takes a backseat during development, but this can lead to vulnerabilities.
Security Best Practices
- Use Environment Variables: Store sensitive information, like API keys, using environment variables rather than hard-coding them.
- Implement IAM Policies: Define permissions carefully, granting only necessary access to your functions.
// Example to access environment variables
exports.handler = async (event) => {
const secretValue = process.env.SECRET_KEY; // Store API keys in Environment Variables
// Your logic here
};
In this snippet, we demonstrate how to access environment variables, emphasizing best practices for secure coding.
The Bottom Line
Building your first serverless function can be a thrilling experience, but it comes with its own set of challenges. By staying mindful of cold starts, monitoring, error handling, cost management, optimization, and security, you can mitigate these common pitfalls.
For additional resources, consider checking out:
By arming yourself with knowledge about these common pitfalls and employing best practices, you can create efficient, scalable, and secure serverless functions, enhancing your development experience in this modern architectural paradigm. Happy coding!
Checkout our other articles