Selenium 4: Key Features and Deprecated Functions to Know
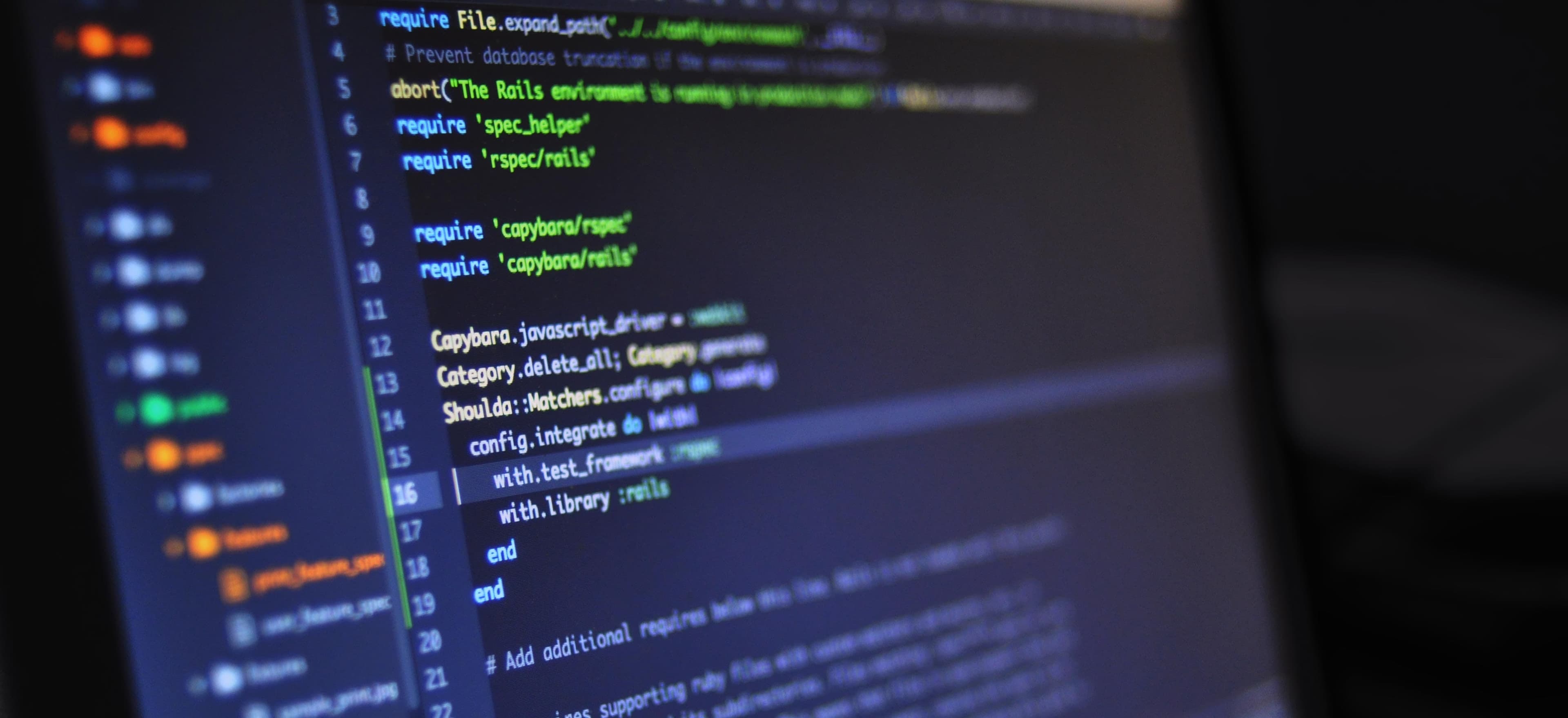
- Published on
Selenium 4: Key Features and Deprecated Functions to Know
Selenium has long been the go-to framework for automating web applications for testing purposes. With the release of Selenium 4, there are some exciting enhancements, new features, and some deprecated functions that developers need to be aware of. This blog post will walk through the essential highlights of Selenium 4, providing examples and insights to help you make the most out of it.
What is Selenium?
Selenium is an open-source framework that allows for the automation of web applications. It supports multiple programming languages, including Java, C#, Python, and Ruby. Initially released in 2004, Selenium has evolved significantly, with Selenium 4 introducing further improvements in automation capabilities and browser compatibility.
Key Features of Selenium 4
1. Selenium Grid Improvements
One of the most significant enhancements in Selenium 4 is the new architecture of Selenium Grid. The new grid is now designed to be a complete server for managing the distribution of tests across multiple browsers and machines.
Key Features of Selenium Grid:
- Simplified Setup: The new Grid has an easy-to-use UI, allowing users to monitor their tests in real-time without requiring any complex configurations.
- Routing: It intelligently routes commands to appropriate nodes based on browser compatibility and project requirements.
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.URL;
public class SeleniumGridExample {
public static void main(String[] args) throws Exception {
WebDriverManager.chromedriver().setup();
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
// Connect to the Selenium Grid hub
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capabilities);
// Automate a simple test
driver.get("http://www.example.com");
System.out.println("Title is: " + driver.getTitle());
driver.quit();
}
}
2. Enhanced WebDriver API
Selenium 4 has introduced a more productive and user-friendly WebDriver API. The new syntax simplifies the interaction with web elements, making your scripts cleaner and easier to navigate.
Key Elements of the New API:
- Relative Locators: The ability to locate elements in relation to others has become more intuitive, making it easier to locate elements using
above
,below
,toLeftOf
, andtoRightOf
.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.locators.RelativeLocator;
public class RelativeLocatorsExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Find an element relative to another
WebElement referenceElement = driver.findElement(By.id("reference-id"));
WebElement nearbyElement = driver.findElement(RelativeLocator.with(By.tagName("h1")).above(referenceElement));
System.out.println("Nearby element text: " + nearbyElement.getText());
driver.quit();
}
}
3. Improved Support for W3C Protocol
Selenium 4 fully supports the W3C WebDriver protocol. This change ensures more secure and consistent behavior across different browsers, enhancing cross-browser compatibility.
Benefits of W3C Protocol Support:
- Greater Stability: The W3C protocol allows for better error handling and reduces inconsistencies when commands are sent to the browser.
- Unified Commands: All commands sent through WebDriver are now standardized, leading to fewer unexpected behaviors.
4. Chrome DevTools Protocol (CDP) Integration
Selenium 4 introduces direct integration with Chrome DevTools Protocol, enabling developers to tap into advanced Chrome functions. This is particularly beneficial for automating actions like performance tracking, network management, and console message monitoring.
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.devtools.DevTools;
import org.openqa.selenium.devtools.v85.network.Network;
public class CDPIntegrationExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
ChromeOptions options = new ChromeOptions();
ChromeDriver driver = new ChromeDriver(options);
// Start a new DevTools session
DevTools devTools = driver.getDevTools();
devTools.createSession();
// Enable network monitoring
devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty()));
devTools.addListener(Network.requestWillBeSent(), request -> {
System.out.println("Request URL: " + request.getRequest().getUrl());
});
driver.get("http://example.com");
driver.quit();
}
}
5. Native Events and Action Chains
Selenium 4 improves how it handles interactions with web elements. The new Action API enables users to create more complex user interactions precisely, such as dragging and dropping, clicking and holding, and much more.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class ActionChainsExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Create an ActionChains object
Actions action = new Actions(driver);
WebElement sourceElement = driver.findElement(By.id("source-id"));
WebElement targetElement = driver.findElement(By.id("target-id"));
// Perform drag and drop
action.dragAndDrop(sourceElement, targetElement).perform();
driver.quit();
}
}
Deprecated Functions in Selenium 4
While Selenium 4 brings many exciting features, it also marks the deprecation of several functions that developers should be aware of to future-proof their code.
1. DesiredCapabilities
DesiredCapabilities
has been largely replaced by a more standardized way of defining capabilities using Options
and Capabilities
. For example, instead of using capabilities, you can now use ChromeOptions
directly:
// Deprecated usage
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("browserName", "chrome");
// Recommended usage
ChromeOptions options = new ChromeOptions();
options.addArguments("--headless");
2. RemoteWebDriver
Connectors
The way RemoteWebDriver connects to a hub has changed. The previous URL format is now streamlined to follow the new W3C protocol rules. The legacy way of connecting is no longer supported and should be updated to avoid issues down the line.
3. wait()
Methods
Older synchronous wait()
methods have been replaced with more robust explicit waits. Using waits like WebDriverWait
or FluentWait
offers better control and reliability for synchronization in your tests.
// Deprecated usage
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Recommended usage
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("element-id")));
To Wrap Things Up
Selenium 4 rolls out with numerous enhancements, making it a more powerful and easier-to-use tool for automation testing. By leveraging the new features, such as improved APIs, native support for W3C standards, and CDP integration, developers can create more effective and efficient test cases.
However, it is paramount to stay updated on the deprecated functions to maintain clean and functional code. There has never been a better time to dive into Selenium. Embrace these changes and elevate your automation testing experience.
For more insights and detailed documentation, visit the official Selenium HQ website. Happy Testing!
Checkout our other articles