Common JBoss Core Java Web Services Pitfalls to Avoid
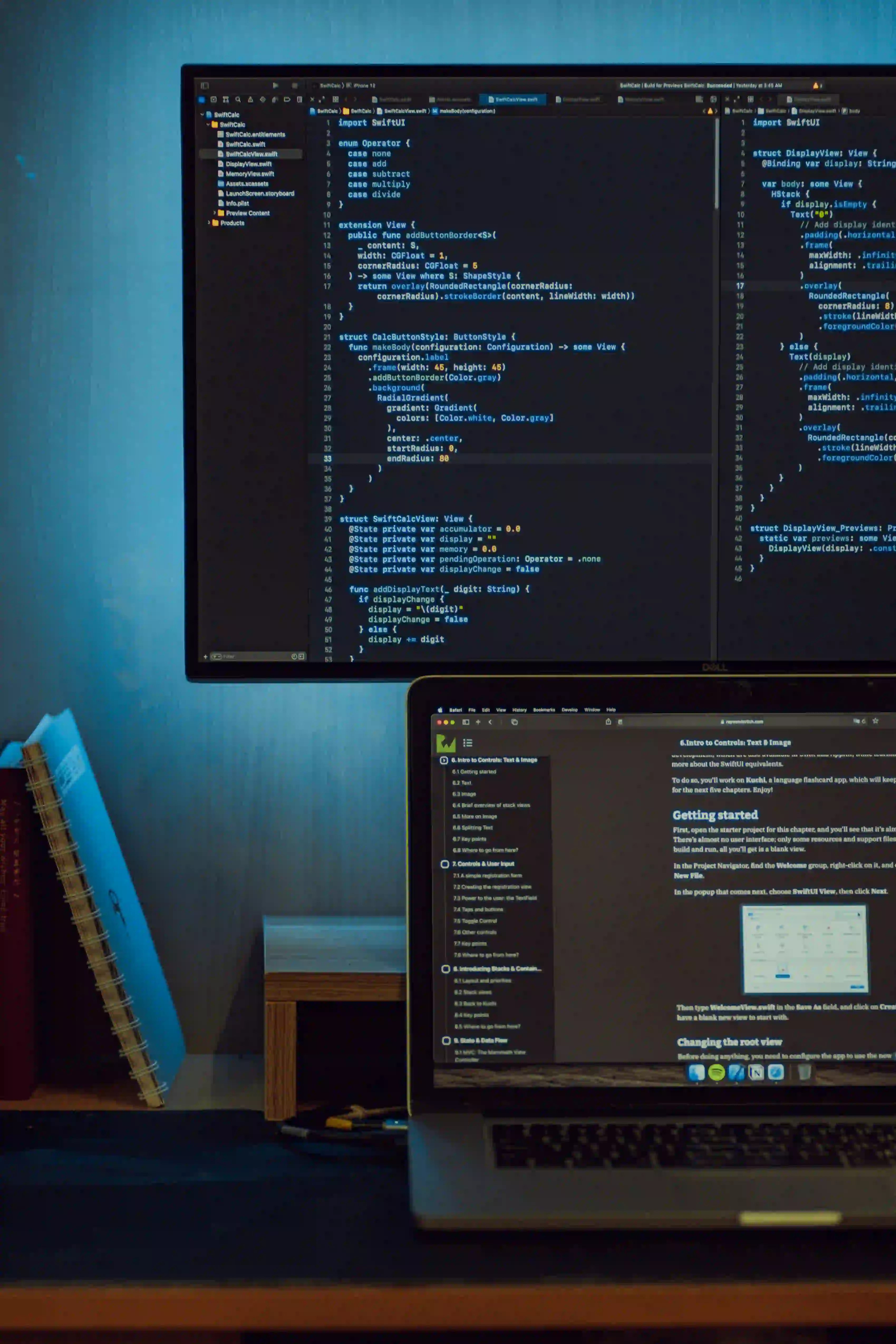
Common JBoss Core Java Web Services Pitfalls to Avoid
Web services are integral to modern application development, allowing disparate systems to communicate and share data seamlessly. JBoss, now known as WildFly, is a popular open-source Java application server that simplifies the deployment of Java web services. However, developers can encounter common pitfalls when working with JBoss Core for their web services. In this article, we'll discuss these pitfalls and provide practical solutions to avoid them.
Table of Contents
- Understanding JBoss Core
- Common Pitfalls
- Misconfiguration of JAX-WS and JAX-RS
- Ignoring Security Practices
- Not Managing Dependencies Correctly
- Failing to Implement Proper Logging and Monitoring
- Handling Exceptions Ineffectively
- Best Practices
- Conclusion
Understanding JBoss Core
JBoss Core, also known as WildFly, is a powerful application server used for building and hosting Java applications. It offers robust features like EJB (Enterprise Java Beans), JPA (Java Persistence API), and JAX-RS/JAX-WS for RESTful and SOAP web services, respectively. However, leveraging these features comes with its own challenges.
Common Pitfalls
1. Misconfiguration of JAX-WS and JAX-RS
One of the most common mistakes is misconfiguring web services. In JAX-WS (for SOAP web services), you need to define @WebService
annotations carefully. For JAX-RS (for RESTful services), using the correct resource annotations like @Path
, @GET
, @POST
, etc., is crucial.
Example of JAX-WS Configuration
import javax.jws.WebService;
@WebService
public class CalculatorService {
public int add(int a, int b) {
return a + b;
}
}
Why this matters: Proper annotations define how the web service behaves and how it can be accessed. Misconfiguration can lead to service failures or incorrect service behavior.
2. Ignoring Security Practices
Security should never be an afterthought. Many developers fail to implement proper security protocols to protect their web services. Malicious actors can exploit vulnerabilities if web services do not validate inputs or authenticate users effectively.
Secure Web Service Implementation
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/secure/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>admin</role-name>
</auth-constraint>
</security-constraint>
Why this matters: Implementing security constraints ensures that sensitive resources are protected and accessible only by authorized users.
3. Not Managing Dependencies Correctly
Dependency management is critical in any Java project. With JBoss, leveraging Maven or Gradle can simplify this process. However, developers often overlook dependency conflicts, leading to classpath issues.
Example of Maven Dependency Management
<dependency>
<groupId>org.jboss.spec.javax.ws.rs</groupId>
<artifactId>jboss-jaxrs-api</artifactId>
<version>2.0.1.Final</version>
<scope>provided</scope>
</dependency>
Why this matters: Properly defining dependencies ensures that your application has access to the right versions of libraries, minimizing the risk of conflicts.
4. Failing to Implement Proper Logging and Monitoring
Logging is crucial for debugging and monitoring web services. Many developers either neglect this or implement insufficient logging.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public User getUser(int userId) {
logger.info("Fetching user with ID: " + userId);
// Fetch user logic
return user;
}
}
Why this matters: Effective logging provides insight into the application’s behavior, helping you track issues and performance bottlenecks.
5. Handling Exceptions Ineffectively
Exception handling in web services is frequently mishandled. If exceptions aren’t caught properly, they can lead to unexpected crashes or security vulnerabilities.
Example of Custom Exception Handling
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.Response;
public class UserNotFoundException extends WebApplicationException {
public UserNotFoundException(String message) {
super(Response.status(Response.Status.NOT_FOUND).entity(message).build());
}
}
Why this matters: Custom exception handling allows for clearer error messages and better user experience throughout your application.
Best Practices
- Follow the Principle of Least Privilege: Always limit access rights for accounts and roles in your application.
- Keep Dependencies Updated: Regularly check for updates in dependencies to patch security vulnerabilities.
- Implement Caching: Use JBoss cache mechanisms to improve performance in data retrieval.
- Document Your APIs: Use tools like Swagger to document REST APIs, making them easier to understand and use.
- Thoroughly Test Your Services: Implement unit and integration tests to ensure your services function as expected in various scenarios.
Final Considerations
In summary, navigating JBoss Core web services can be fraught with pitfalls. Misconfiguration, security neglect, dependency mismanagement, poor logging practices, and ineffective exception handling can all contribute to suboptimal web service performance and security.
By understanding these common problems and implementing the best practices outlined above, you can enhance the robustness and reliability of your Java web services running on JBoss.
Feel free to explore more about JBoss/WildFly documentation or consider checking out the Java EE tutorial for comprehensive guidelines. Happy coding!
This blog post aims to equip you with the knowledge to sidestep potentially detrimental pitfalls while building web services using JBoss Core. It emphasizes both practical coding and overarching strategies that foster long-term project success.