Troubleshooting Common Issues with Custom Java Annotations
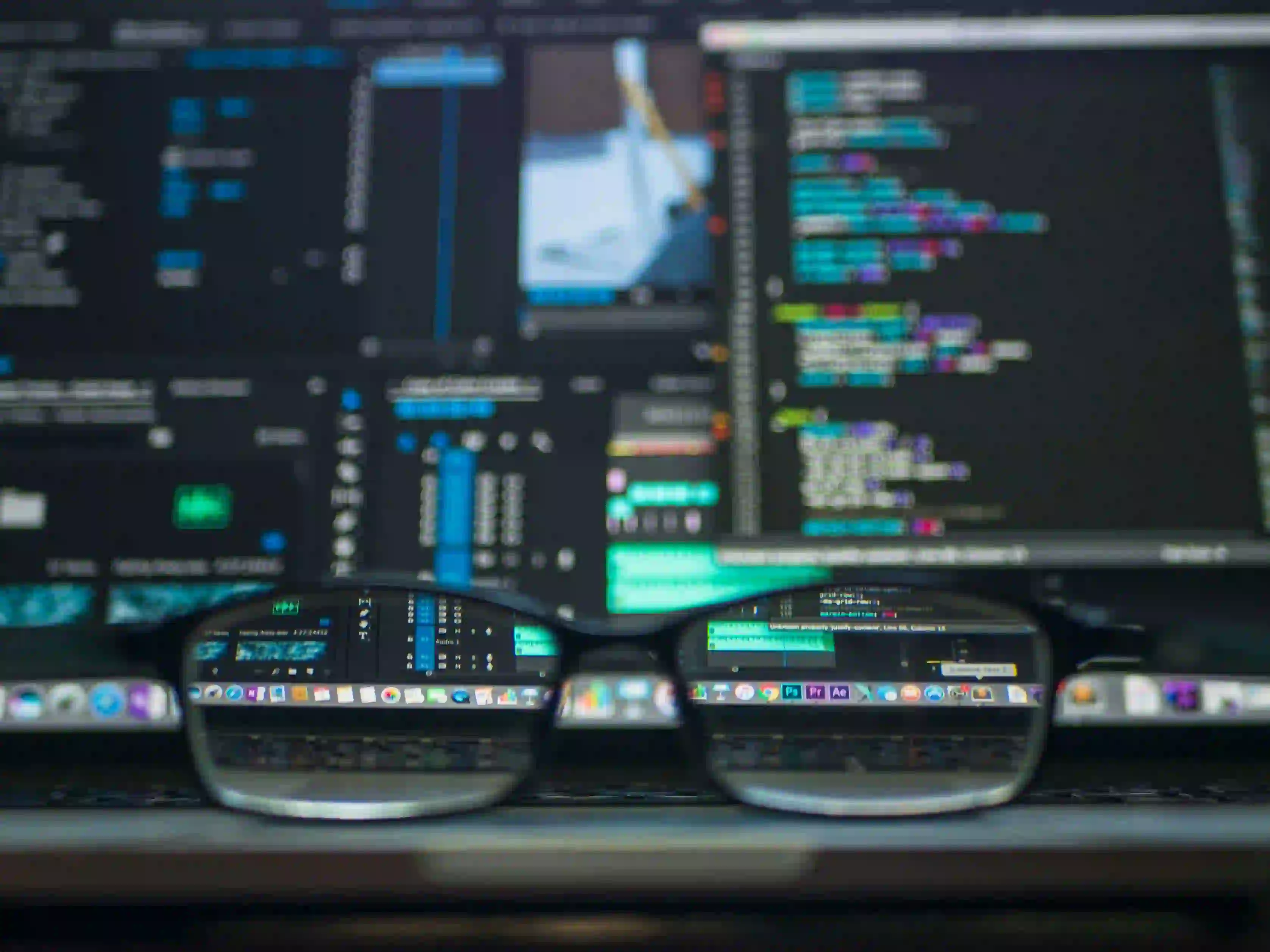
Troubleshooting Common Issues with Custom Java Annotations
Java annotations are a powerful feature that allows developers to add metadata to their code. This metadata can influence compile-time processing, runtime behavior, and even documentation. While annotations can simplify your code and make it more readable, implementing custom Java annotations may lead to some common pitfalls. In this blog post, we’ll explore these issues and provide practical troubleshooting strategies.
What Are Annotations in Java?
Annotations are a form of metadata that provide data about a program but are not part of the program itself. In Java, annotations are defined using the @interface
keyword. They can be used to convey information about code to the compiler or to frameworks, such as Spring or Hibernate.
Example of a Custom Annotation
Before diving into troubleshooting, let’s start with a simple custom annotation example.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyCustomAnnotation {
String value();
}
In this code snippet:
- @Retention specifies that the annotation should be available at runtime.
- @Target indicates that it can be applied to methods.
Common Issues and How to Troubleshoot Them
1. Annotation Not Being Detected at Runtime
A fairly common issue is that your annotations don’t seem to be recognized at runtime. This usually stems from improper retention policy settings. If the retention policy is set to SOURCE
, the annotations will be discarded by the compiler and not available in the bytecode.
Solution: Ensure that the retention policy is set to RUNTIME
, as shown in the example above.
Example:
// Wrong Retention Policy - will cause runtime issues
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.METHOD)
public @interface MyCustomAnnotation {
String value();
}
2. Incorrect Target Specification
If your annotation is meant to be applied to methods but you apply it to fields or classes, your program won’t behave as expected. Always validate that you've set the correct target.
Solution: Check the @Target
annotation to confirm it includes the appropriate ElementTypes.
Example:
@Target(ElementType.FIELD) // Incorrect for methods
public @interface MyCustomAnnotation {
String value();
}
3. Annotation Processor Not Being Invoked
If you’ve implemented an annotation processor but it’s not being triggered during compilation, it might be due to incorrect implementation or lack of configuration.
Solution: Verify that your annotation processor is properly registered in your project’s build file (like Maven’s pom.xml
or Gradle’s build.gradle
).
Example in Maven:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<annotationProcessorPaths>
<path>
<groupId>com.example</groupId>
<artifactId>my-annotation-processor</artifactId>
<version>1.0</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
</build>
4. Building Custom Logic with Annotations
Sometimes developers struggle to effectively utilize custom annotations within their logic. For example, while fetching annotated methods, they might overlook consideration for accessibility (like private or protected methods).
Solution: Make sure to handle all accessible levels when retrieving methods with your annotation.
Example of Fetching Annotated Methods
import java.lang.reflect.Method;
public class AnnotationProcessor {
public void processAnnotations(Class<?> clazz) {
for (Method method : clazz.getDeclaredMethods()) {
if (method.isAnnotationPresent(MyCustomAnnotation.class)) {
MyCustomAnnotation annotation = method.getAnnotation(MyCustomAnnotation.class);
System.out.println("Found method: " + method.getName() + ", annotation value: " + annotation.value());
}
}
}
}
// Usage
public class Test {
@MyCustomAnnotation("Test method")
public void myAnnotatedMethod() {
// Some logic
}
}
5. Using Multiple Annotations or Combining Annotations
Managing multiple annotations can lead to confusion. If one annotation depends on another, it’s crucial to ensure that they’re compatible and properly configured.
Solution: Keep your annotations in a clear hierarchy and document their purpose, usage, and any dependencies they may have.
Example of Combining Annotations
@Target(ElementType.TYPE)
public @interface CustomClassAnnotation {
String description();
}
// Class with multiple annotations
@MyCustomAnnotation("Main class")
@CustomClassAnnotation(description = "This class does XYZ")
public class MainClass {
// Implementation
}
Best Practices for Custom Annotations
-
Keep It Simple: Only create an annotation when necessary. Avoid over-engineering your solution.
-
Document Annotations: Utilize Javadoc effectively to describe what your annotations do. This will help others understand their application and maintenance.
-
Consistency is Key: Follow naming conventions and keep a uniform structure in your annotations. This will improve code readability and maintenance.
-
Testing Annotations: Always write unit tests to verify that your annotations behave as expected.
-
Use Libraries When Possible: For common needs, consider existing libraries (like Lombok) before creating custom annotations.
Closing the Chapter
Custom Java annotations can significantly improve the functionality and readability of your code when implemented correctly. By avoiding common pitfalls and leveraging effective troubleshooting techniques, you can harness the power of annotations while avoiding potential issues.
For more in-depth information on Java annotations and their use cases, consider reading Oracle’s Java Documentation for a detailed guide.
As you gain experience with Java annotations, you will appreciate their utility and flexibility in various coding scenarios. Happy coding!