Mastering Error Handling with CompletableFuture in Java 8
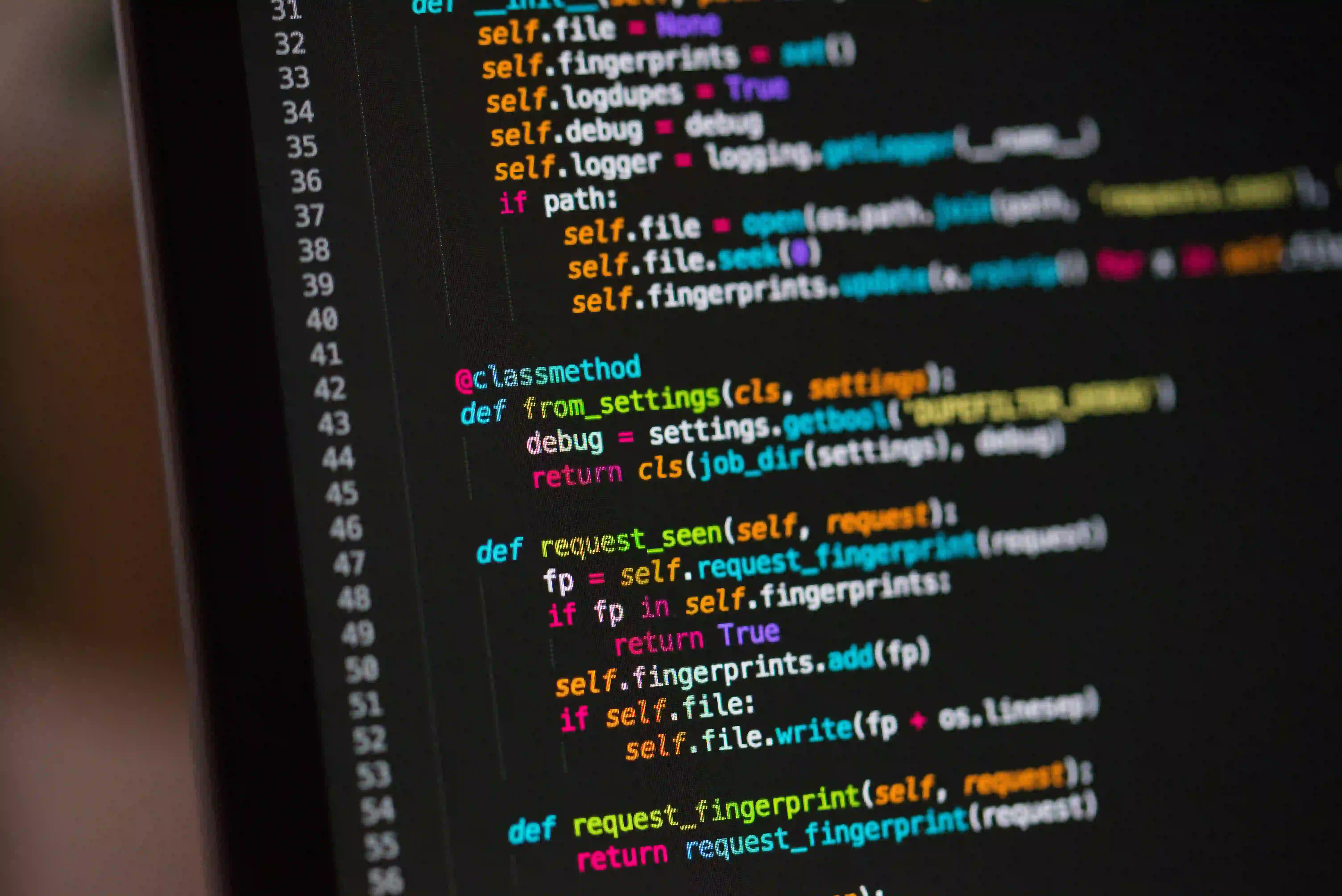
Mastering Error Handling with CompletableFuture in Java 8
Java 8 introduced CompletableFuture
, a powerful tool that enables asynchronous programming by simplifying the handling of side effects and enabling better error management. Prior to Java 8, writing asynchronous code was often complicated, leading to callback hell or hard-to-manage threads. With the introduction of CompletableFuture
, error handling can be more intuitive and manageable.
In this blog post, we will delve deep into mastering error handling with CompletableFuture
. We will explore how to create them, how to handle exceptions, and how to apply best practices to ensure robust applications.
What is CompletableFuture?
CompletableFuture
is part of the java.util.concurrent
package and provides a way to write asynchronous non-blocking code. It is an enhancement of the Future
interface that allows the writing of asynchronous code in a more readable and manageable way by using chained methods.
Benefits of CompletableFuture
- Non-blocking: It allows other tasks to continue running without blocking the main thread.
- Composability: You can easily chain operations and combine multiple
CompletableFuture
instances. - Better error handling: Built-in methods allow you to handle exceptions more gracefully.
Creating a CompletableFuture
Let's start with a simple example of creating a CompletableFuture
. This will serve as our foundational code for subsequent error handling discussions.
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
sleep(2000);
return "Hello, CompletableFuture!";
});
// Handling result or error
future.thenAccept(result -> System.out.println("Result: " + result))
.exceptionally(ex -> {
System.err.println("Error: " + ex.getMessage());
return null;
});
// Adding a delay to allow asynchronous execution to complete before the main thread exits
sleep(3000);
}
private static void sleep(long millis) {
try {
Thread.sleep(millis);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why Use CompletableFuture.supplyAsync()
?
- The
supplyAsync()
method runs the task in a separate thread without blocking the main thread. - It eventually returns a
CompletableFuture
which represents the result of that computation once it's done, allowing for subsequent operations.
Chaining Methods and Error Handling
Chaining methods is one of the strengths of CompletableFuture
. Following our example, we can see how we handle either the result of our computation or any exceptions that arise.
- Result Handling: We use
thenAccept()
to handle successful completion. - Error Handling: The
exceptionally()
method is called if the computation throws an exception.
Handling Exceptions with CompletableFuture
The Exception Handling Methods
Apart from exceptionally()
, which deals with exceptions right after the computation, there are other methods for error handling:
- handle(): This method lets you handle both the result and exception in one go.
- whenComplete(): This method is executed once the
CompletableFuture
completes, regardless of whether it completed normally or exceptionally.
Example of handle()
CompletableFuture<String> futureWithHandle = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Something went wrong");
})
.handle((result, ex) -> {
if (ex != null) {
System.err.println("Error Handled: " + ex.getMessage());
return "Default Value";
}
return result;
});
Key Points about handle()
- The method takes a
BiFunction<T, Throwable, R>
, whereT
is the type of the result andThrowable
is the exception. - It returns a new
CompletableFuture
that contains either the successful result or a default value if an exception occurred.
Best Practices for Error Handling
Handling errors correctly is vital for creating robust applications. Here are some best practices:
-
Be Specific with Exceptions: Catch specific exceptions rather than general ones to provide more informative error messages.
-
Logging: Make sure to log exceptions as they occur. This helps in debugging during development and provides insight when running in production.
-
Chain Exception Handling: Make use of
handle()
orexceptionally()
effectively to handle exceptions gracefully and maintain application stability. -
Use CompletableFuture to avoid blocking: Leverage non-blocking calls to ensure your application stays responsive.
-
Combine Multiple Futures Carefully: If you combine several
CompletableFuture
instances using methods likethenCombine()
, ensure you handle exceptions at both levels.
Common Use Cases of CompletableFuture
-
Web Services Invocation: Use
CompletableFuture
to call multiple APIs simultaneously and process their responses collectively. -
Data Processing Pipelines: You can create processing steps for data that can be chained together numerically and can also handle exceptions at each stage.
-
Dynamic Application Features: You can keep your application lightweight by performing expensive computations in the background.
Final Thoughts
Mastering error handling in CompletableFuture
can significantly enhance the quality and reliability of your Java applications. As we explored, by utilizing the built-in methods for exception handling and applying best practices, you can create robust asynchronous code that remains maintainable.
To further deepen your understanding of asynchronous programming and CompletableFuture
, consider checking out the Java 8 Tutorial and Java Concurrency in Practice.
Implementing these concepts will certainly make your applications not only faster but also easier to debug and maintain, paving the way for a more resilient software architecture. Happy coding!