Common Pitfalls When Building an RSS Reader with Rome
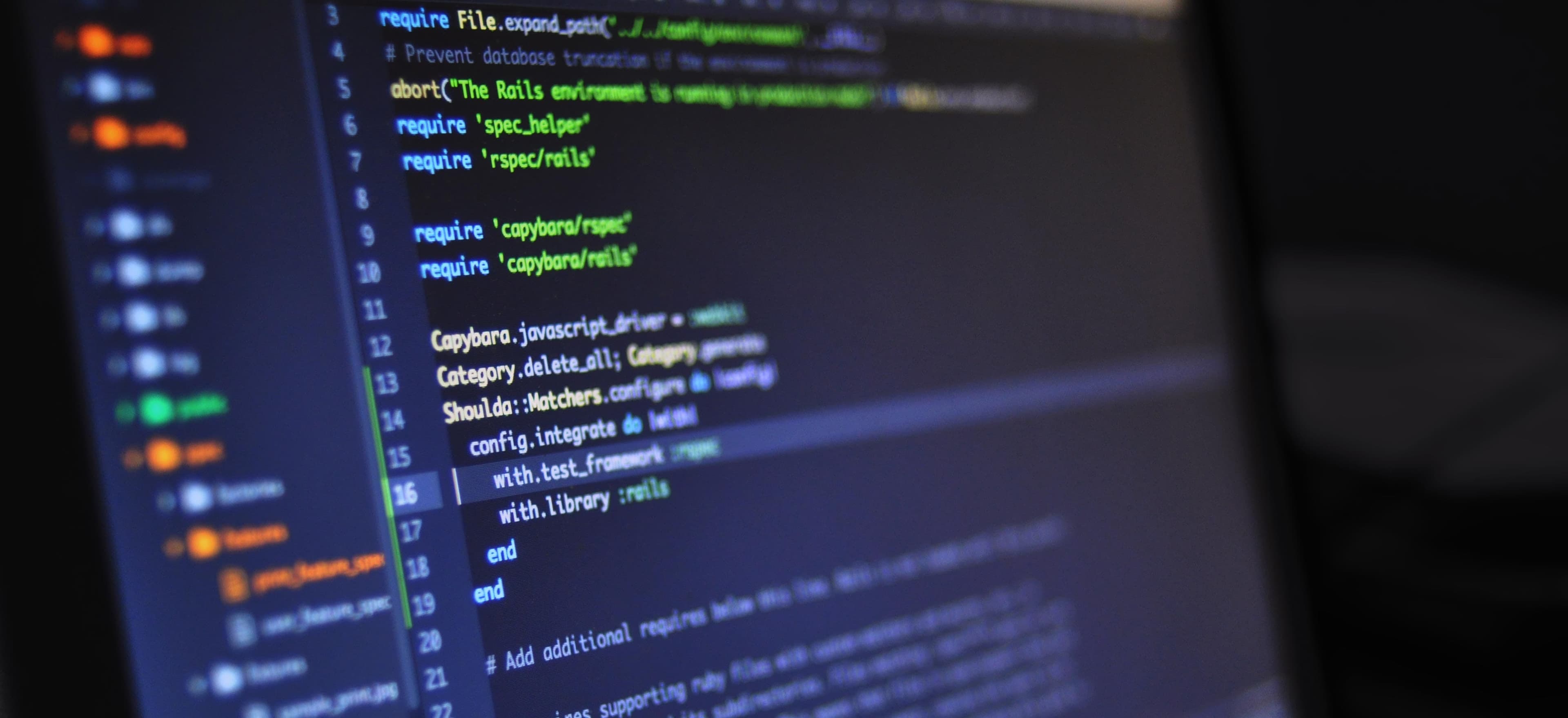
- Published on
Common Pitfalls When Building an RSS Reader with Rome
Building an RSS reader can be an exciting project. It allows you to aggregate content, keep up with news, and serve as a hands-on introduction to working with XML and Java libraries. One such library is Rome, a popular Java library that simplifies the parsing of RSS feeds. However, even seasoned developers can encounter issues when working with this library. This blog post will discuss some common pitfalls you may face when building an RSS reader using Rome.
Understanding RSS and Rome
An RSS (Really Simple Syndication) feed is an XML file that allows users to access updates to websites in a standardized format. Rome provides a simple way to parse this XML and extract relevant data.
Before diving into the code, it’s crucial to understand the basic structure of an RSS feed. An RSS feed consists of a channel element that can have various child elements such as title, link, description, and item, among others.
Pitfall #1: Not Handling Exceptions Properly
When you work with external feeds, exceptions are inevitable. Failing to handle them can lead your application to crash unexpectedly.
Code Example
import com.rometools.rome.feed.synd.SyndFeed;
import com.rometools.rome.feed.synd.SyndEntry;
import com.rometools.rome.io.SyndFeedInput;
import com.rometools.rome.io.XmlReader;
import java.net.URL;
import java.util.List;
public class RSSReader {
public void readFeed(String feedUrl) {
try {
URL url = new URL(feedUrl);
SyndFeedInput input = new SyndFeedInput();
SyndFeed feed = input.build(new XmlReader(url));
List<SyndEntry> entries = feed.getEntries();
for (SyndEntry entry : entries) {
System.out.println(entry.getTitle());
}
} catch (Exception e) {
System.err.println("Error reading feed: " + e.getMessage());
}
}
}
Commentary
In the code above, we wrap our feed reading logic in a try-catch block. The catch block captures any exceptions and logs an informative message. This not only prevents your application from crashing but also helps in debugging and maintaining the program in the long run.
Pitfall #2: Ignoring Feed Types and Variations
RSS comes in different formats like RSS 2.0, Atom, and more. Rome supports these variations, but ensuring that you handle each type correctly is essential.
Code Example
public void processFeed(String feedUrl) {
try {
URL url = new URL(feedUrl);
SyndFeedInput input = new SyndFeedInput();
SyndFeed feed = input.build(new XmlReader(url));
if ("rss".equals(feed.getFeedType())) {
processRSSFeed(feed);
} else if ("atom_1.0".equals(feed.getFeedType())) {
processAtomFeed(feed);
} else {
System.err.println("Unsupported feed type: " + feed.getFeedType());
}
} catch (Exception e) {
System.err.println("Error processing feed: " + e.getMessage());
}
}
private void processRSSFeed(SyndFeed feed) {
// Process RSS-specific features
}
private void processAtomFeed(SyndFeed feed) {
// Process Atom-specific features
}
Commentary
Here, we check the feed type before processing it. This ensures that your application can adapt to different feed types and handle specific nuances correctly. In practice, this flexibility can save a significant amount of time and prevent errors when working with various news sources.
Pitfall #3: Forgetting to Validate Input URLs
Not validating the feed URLs can lead to runtime errors, especially with malformed URLs. Always check for proper formatting before attempting to parse.
Code Example
import java.util.regex.Pattern;
public boolean isValidUrl(String urlString) {
String urlPattern = "^(http|https)://.*$";
return Pattern.matches(urlPattern, urlString);
}
public void readFeed(String feedUrl) {
if (!isValidUrl(feedUrl)) {
System.err.println("Invalid URL: " + feedUrl);
return;
}
// Proceed to read the feed...
}
Commentary
In this snippet, we implement a URL validation method using a regex pattern. The use of regex checks for basic URL formatting, preventing invalid URLs from proceeding to the RSS feed reading logic. This simple validation can save you troubleshooting time later.
Pitfall #4: Not Caching Feed Data
RSS feeds can be quite dynamic, but that does not mean you should continually request updates for the same content. Caching feed data minimizes network calls and speeds up the user experience.
Code Example
import java.util.HashMap;
import java.util.Map;
public class FeedCache {
private Map<String, SyndFeed> cache = new HashMap<>();
public SyndFeed getFeed(String feedUrl) {
return cache.get(feedUrl);
}
public void putFeed(String feedUrl, SyndFeed feed) {
cache.put(feedUrl, feed);
}
}
// In your RSSReader:
FeedCache feedCache = new FeedCache();
public void readFeed(String feedUrl) {
SyndFeed cachedFeed = feedCache.getFeed(feedUrl);
if (cachedFeed != null) {
// Use cached feed
return;
}
// Logic to read and cache the new feed
feedCache.putFeed(feedUrl, feed);
}
Commentary
In this example, we implement a caching layer using a map. By checking if a feed is already cached before attempting to read it, we improve efficiency and reduce unnecessary network calls. This can have a significant impact, especially when dealing with multiple feeds.
In Conclusion, Here is What Matters
Building an RSS reader with Rome can be both fun and educational, but pitfalls are lurking around every corner. From exception handling to ensuring feed validity, handling feed types, and establishing caching, let these common pitfalls serve as warning signs in your journey. Avoiding them will lead to a more robust and reliable application.
For further reading on working with RSS feeds in Java, you can check out this article on parsing XML with Rome to learn more about its capabilities. With these insights, you’re well on your way to developing a successful RSS reader. Happy coding!
Checkout our other articles