Troubleshooting Common Spring Boot Elasticsearch Integration Issues
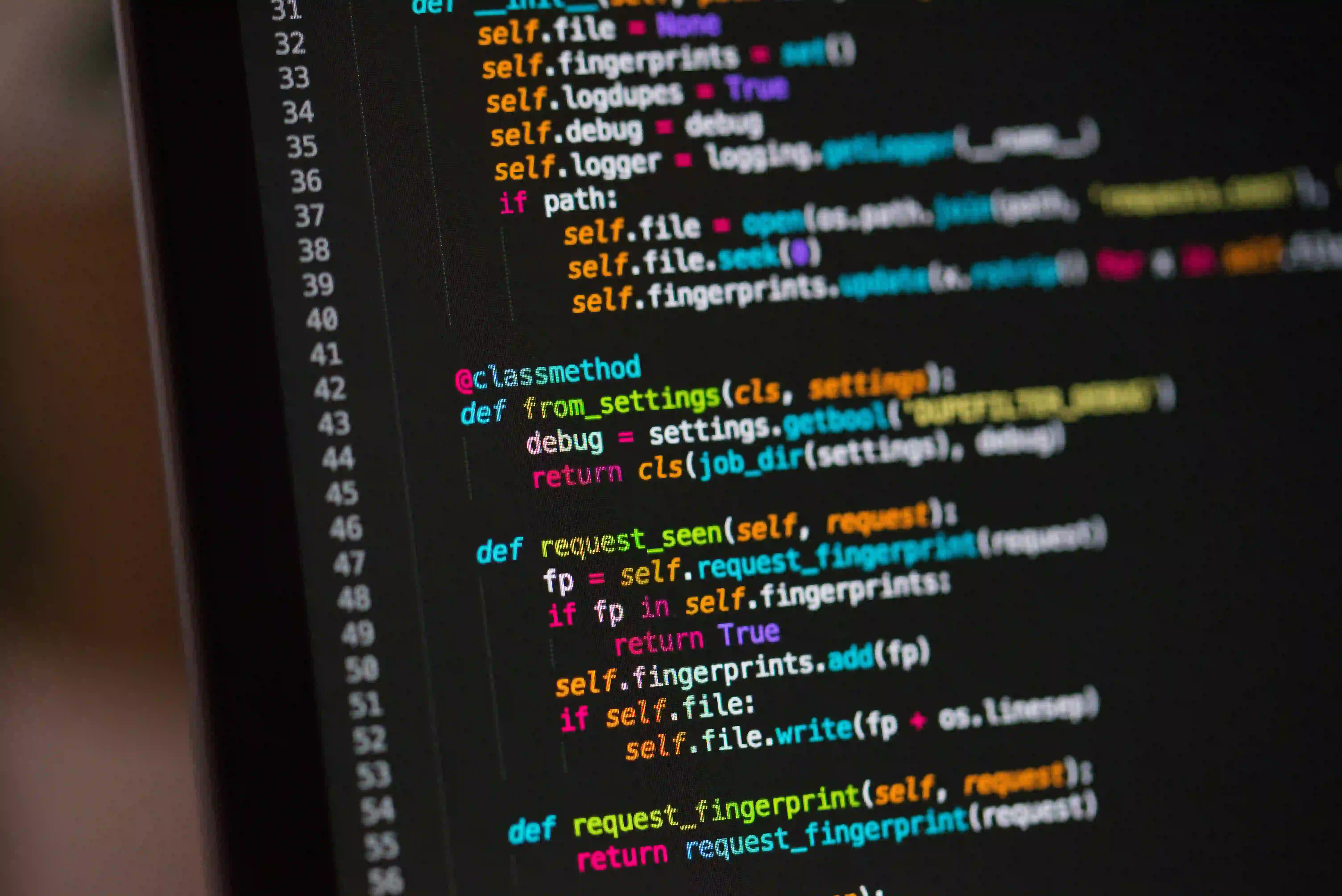
Troubleshooting Common Spring Boot Elasticsearch Integration Issues
Spring Boot has surged in popularity among developers thanks to its robust capabilities and seamless integration with various technologies. One of the most in-demand integrations is with Elasticsearch, a powerful search and analytics engine. However, as with any technology stack, issues can arise. This blog post will guide you through common Spring Boot and Elasticsearch integration issues and their solutions.
Table of Contents
- Understanding the Basics of Spring Boot and Elasticsearch
- Common Issues and Troubleshooting Techniques
- Best Practices for Spring Boot and Elasticsearch Integration
- Conclusion
Understanding the Basics of Spring Boot and Elasticsearch
What is Spring Boot?
Spring Boot is a project from the Spring Framework that simplifies the process of setting up and deploying Spring applications. It offers pre-configured settings out of the box, reducing boilerplate code and enhancing productivity.
What is Elasticsearch?
Elasticsearch is a distributed, RESTful search and analytics engine designed for horizontal scalability, reliability, and real-time search capabilities.
How They Work Together
Combining Spring Boot with Elasticsearch allows developers to create applications that can leverage the powerful search and analytical features of Elasticsearch while benefiting from Spring Boot's simplicity.
Common Issues and Troubleshooting Techniques
1. Elasticsearch Service Not Starting
Symptoms: When attempting to run your Spring Boot application, you may encounter errors indicating that the Elasticsearch service is not running or cannot be reached.
Solution:
-
Verify Elasticsearch Installation: Ensure that Elasticsearch is installed correctly and the service is running. On Unix-based systems, you can typically start Elasticsearch with:
🔧snippet.shsudo service elasticsearch start
For Windows users, make sure to run it as a Windows service.
-
Check Service Status: Verify that the service status is "active (running)" using:
🔧snippet.shsudo systemctl status elasticsearch
-
Firewall Settings: Sometimes, firewall settings may prevent access to Elasticsearch. Ensure the necessary ports (default is 9200) are open.
2. Incorrect Elasticsearch Configuration
Symptoms: You may encounter Connection refused
errors or fail to connect to the Elasticsearch server.
Solution:
-
Check Application Properties: In your
application.properties
orapplication.yml
, double-check your Elasticsearch configuration settings.Example configuration:
📄snippet.txtspring.data.elasticsearch.cluster-nodes=localhost:9200 spring.data.elasticsearch.repositories.enabled=true
-
Cluster Health: Verify the health of your Elasticsearch cluster by accessing
http://localhost:9200/_cluster/health
in your browser or using a tool like Postman. -
Version Compatibility: Ensure that the versions of Spring Boot, Spring Data Elasticsearch, and Elasticsearch are compatible. Check the Spring Data Elasticsearch documentation for version compatibility.
3. Dependency Issues
Symptoms: You might encounter ClassNotFoundException
or NoSuchMethodError
.
Solution:
-
Maven Dependencies: Ensure you have the correct Spring Data Elasticsearch dependency in your
pom.xml
file.📄snippet.txt<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-elasticsearch</artifactId> </dependency>
-
Examine Dependency Tree: Use the Maven command to visualize your dependencies and check for conflicts:
🔧snippet.shmvn dependency:tree
-
Clear Local Repository: Sometimes, corrupted local cache can cause issues. Clear your local Maven repository using:
🔧snippet.shmvn clean install
4. Data Not Being Indexed
Symptoms: You might find that your indexed documents are missing or not appearing in Elasticsearch.
Solution:
-
Check Document Structure: Make sure that the data being sent to Elasticsearch conforms to the expected document structure. Use an explicit mapping if necessary.
Example of a simple mapping:
☕snippet.java@Document(indexName = "users") public class User { @Id private String id; private String name; // getters and setters }
-
Inspect Logs: Check the logs for any errors or warnings related to indexing. The logs might indicate if there were issues with serialization or connection problems.
-
Manual Indexing: For testing, try to manually index a document using curl or Postman to confirm the server is functioning:
🔧snippet.shcurl -X POST "localhost:9200/users/_doc/1" -H 'Content-Type: application/json' -d' { "name": "John Doe" } '
5. Search Queries Returning No Results
Symptoms: Even though documents are indexed, searching returns no results.
Solution:
-
Correct Query Format: Ensure that your search queries are appropriately formed based on Elasticsearch's DSL.
Example of a search query:
☕snippet.javaSearchRequest searchRequest = new SearchRequest("users"); SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder(); searchSourceBuilder.query(QueryBuilders.matchQuery("name", "John")); searchRequest.source(searchSourceBuilder);
-
Refresh Index: If you index data but do not refresh the index, it may not be available for search. You can force a refresh with:
🔧snippet.shcurl -X POST "localhost:9200/users/_refresh"
-
Examine Filters: Ensure that there aren’t overly restrictive filters applied that might exclude valid results.
Best Practices for Spring Boot and Elasticsearch Integration
-
Version Management: Always use compatible versions of Spring Boot, Spring Data Elasticsearch, and Elasticsearch. Regularly check for updates.
-
Use the RestHighLevelClient: This client is more aligned with modern standards and better handles asynchronous operations.
-
Error Handling: Implement robust error handling in your service methods to catch and manage specific exceptions related to Elasticsearch.
-
Testing: Always write integration tests to verify that your Spring Boot application interacts correctly with Elasticsearch.
-
Monitor Health: Use tools like Kibana or Elasticsearch's built-in monitoring features to keep track of your Elasticsearch cluster’s health.
Wrapping Up
Integrating Spring Boot with Elasticsearch can significantly enhance your application's search capabilities. However, it's vital to be mindful of common pitfalls during the integration process. By following the troubleshooting steps and best practices outlined in this post, you can mitigate these issues and build robust applications with seamless search functionality.
For further reading, check out these useful resources:
Embrace the power of Spring Boot and Elasticsearch to deliver exceptional search experiences in your applications!