Top 5 Metrics Every Microservices Architect Should Track
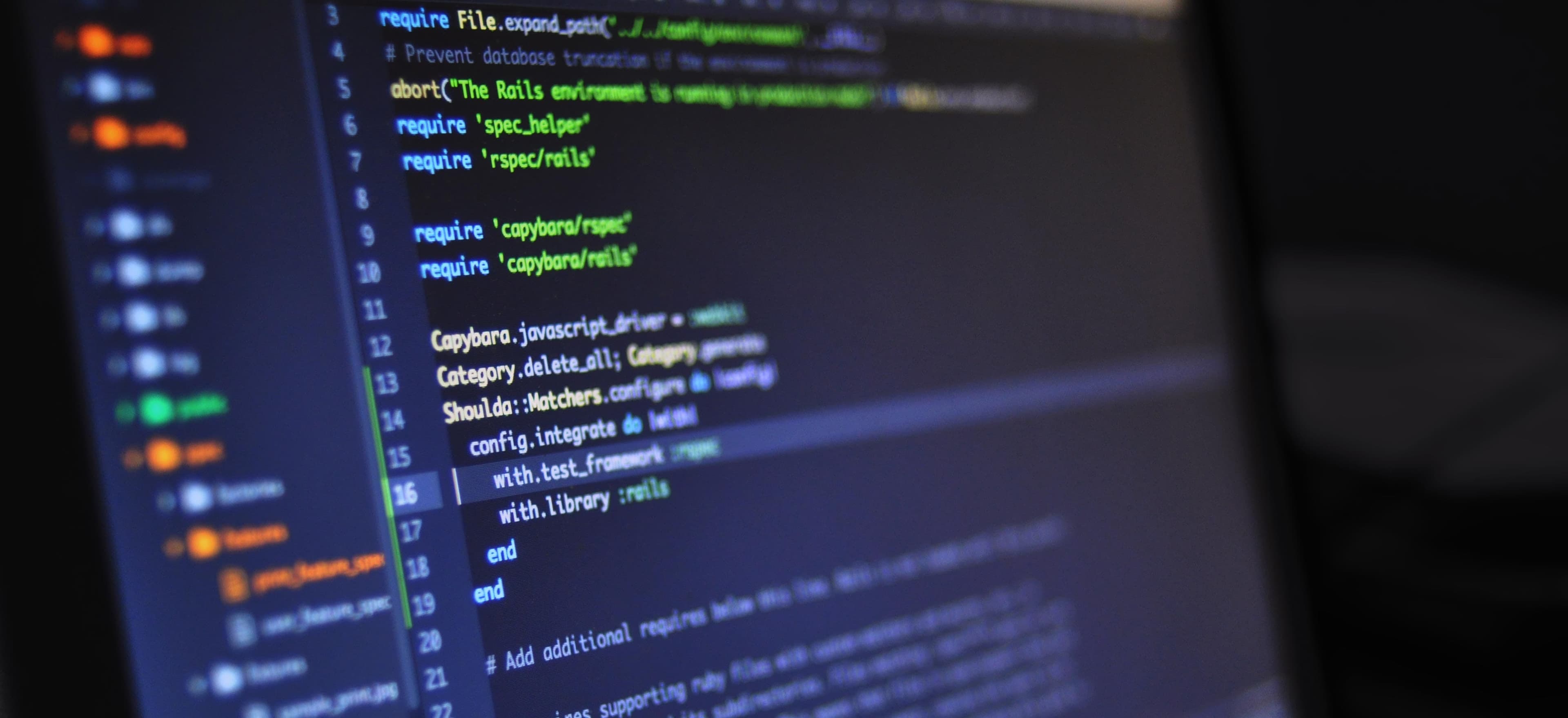
- Published on
Top 5 Metrics Every Microservices Architect Should Track
Microservices architecture has gained immense popularity due to its scalability, agility, and ease of deployment. However, managing microservices can be complex and challenging. To ensure your microservices-based applications are performing optimally, it is crucial to track specific metrics. This blog post will delve into the Top 5 Metrics Every Microservices Architect Should Track, helping you maintain high performance and reliability.
1. Response Time
Why Track Response Time?
Response time is a critical metric for understanding how quickly your microservices respond to requests. A slow response time can lead to user dissatisfaction and a decrease in application performance. Monitoring response time allows you to identify bottlenecks in your services.
How to Measure
You can measure response time by tracking the time from when a request is made until the response is received. This can be done using tools like Prometheus or New Relic.
Example Code
Here's a simple example using Spring Boot to log response time:
@RestController
public class GreetingController {
@GetMapping("/greeting")
public ResponseEntity<String> greeting() {
long startTime = System.currentTimeMillis();
String response = "Hello, World!";
long responseTime = System.currentTimeMillis() - startTime;
log.info("Response Time: " + responseTime + " ms");
return ResponseEntity.ok(response);
}
}
Commentary
In this code snippet, we use System.currentTimeMillis()
to capture the time before and after processing the request. This allows us to compute the response time and log it for analysis. Regularly monitoring this information can help you spot trends and make data-driven decisions for performance improvements.
2. Error Rate
Why Track Error Rate?
The error rate is a crucial indicator of the reliability of your microservices. A high error rate can lead to application downtime and a poor user experience. By tracking error rates, architects can quickly identify and address issues before they escalate.
How to Measure
Track the number of failed requests over a defined period and calculate the error rate as follows:
Error Rate = (Number of Errors) / (Total Requests) * 100
Example Code
Here’s how you can achieve this in Java with Spring AOP:
@Aspect
@Component
public class ErrorTrackingAspect {
private int totalRequests = 0;
private int errorCount = 0;
@Around("execution(* your.package..*(..))")
public Object trackError(ProceedingJoinPoint joinPoint) throws Throwable {
totalRequests++;
try {
return joinPoint.proceed();
} catch (Exception e) {
errorCount++;
log.error("Error in method: " + joinPoint.getSignature(), e);
throw e; // Rethrow the exception to propagate the error
} finally {
log.info("Error Rate: " + (float) errorCount / totalRequests * 100 + "%");
}
}
}
Commentary
This aspect tracks the total number of requests and counts errors within them. By employing AOP (Aspect-Oriented Programming), you can seamlessly integrate error tracking without modifying business logic. This provides a clear view of your service's reliability.
3. Throughput
Why Track Throughput?
Throughput measures the number of requests processed over a specific time frame. A declining throughput can indicate underlying issues, such as server performance or service limitations. Understanding throughput is vital for planning resource scaling.
How to Measure
You can use monitoring tools like Grafana or DataDog to visualize and track throughput metrics.
Example Code
Here’s how you can log the throughput in a Spring Boot application:
@RestController
public class ThroughputController {
private AtomicLong requestCounter = new AtomicLong();
@GetMapping("/throughput")
public ResponseEntity<String> handleRequest() {
requestCounter.incrementAndGet();
return ResponseEntity.ok("Processed successfully");
}
@Scheduled(fixedRate = 1000)
public void logThroughput() {
long throughput = requestCounter.getAndSet(0);
log.info("Throughput: " + throughput + " requests/second");
}
}
Commentary
In this snippet, we use an AtomicLong
to maintain a request counter and log the throughput every second using a scheduled task. This approach helps you monitor your service’s capacity and make proactive scaling decisions.
4. Service Dependency Health
Why Track Service Dependency Health?
Microservices are interconnected. The health of one service may depend on another. Monitoring dependency health helps you identify failures in dependent services that could lead to cascading issues across your entire architecture.
How to Measure
Establish health checks for each service and monitor their status. Tools like Spring Boot Actuator can help.
Example Code
Here’s a Spring Boot actuator setup for service health checks:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public HealthIndicator myCustomHealthIndicator() {
return () -> {
// Check the health of the service dependency here
boolean healthy = // logic to check health condition
return healthy ? Health.up().build() : Health.down().build();
};
}
}
Commentary
This custom health indicator will help you assess the status of dependent services. Monitoring health indicators allows you to quickly address issues and maintain overall system reliability.
5. Latency
Why Track Latency?
Latency measures the delay before a transfer of data begins following an instruction for its transfer. High latency can significantly affect the user experience and can indicate issues in the system, such as network bottlenecks or slow dependencies.
How to Measure
You can monitor latency through application logs or by employing APM (Application Performance Management) tools like Dynatrace.
Example Code
Basic latency tracking can be implemented as follows:
@RestController
public class LatencyController {
@GetMapping("/latency")
public ResponseEntity<String> getLatencyData() {
long startTime = System.currentTimeMillis();
// Simulate processing
try {
Thread.sleep(100); // Simulate some latency
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
long latency = System.currentTimeMillis() - startTime;
log.info("Latency: " + latency + " ms");
return ResponseEntity.ok("Latency logged");
}
}
Commentary
In this example, we simulate some latency with Thread.sleep()
. Logging the latency provides insights into how delays in a microservice can impact the overall performance.
My Closing Thoughts on the Matter
Tracking the right metrics in a microservices architecture is vital for ensuring high performance, reliability, and user satisfaction. By focusing on response time, error rate, throughput, service dependency health, and latency, microservices architects can gain valuable insights into their applications.
To gain a comprehensive overview of your microservices and enable continuous improvement, consider integrating monitoring tools like Prometheus, Grafana, or New Relic. The right balance of metrics allows you to proactively respond to issues, ensuring your microservices architecture thrives in today’s competitive landscape.
For further reading on microservices and their management, consider exploring articles on Martin Fowler's website and books like "Building Microservices" by Sam Newman.
By following these best practices, you can turn metrics into actionable intelligence, leading your microservices to a path of sustained greatness.
Checkout our other articles