Overcoming Common Pitfalls in Strategy Pattern Design
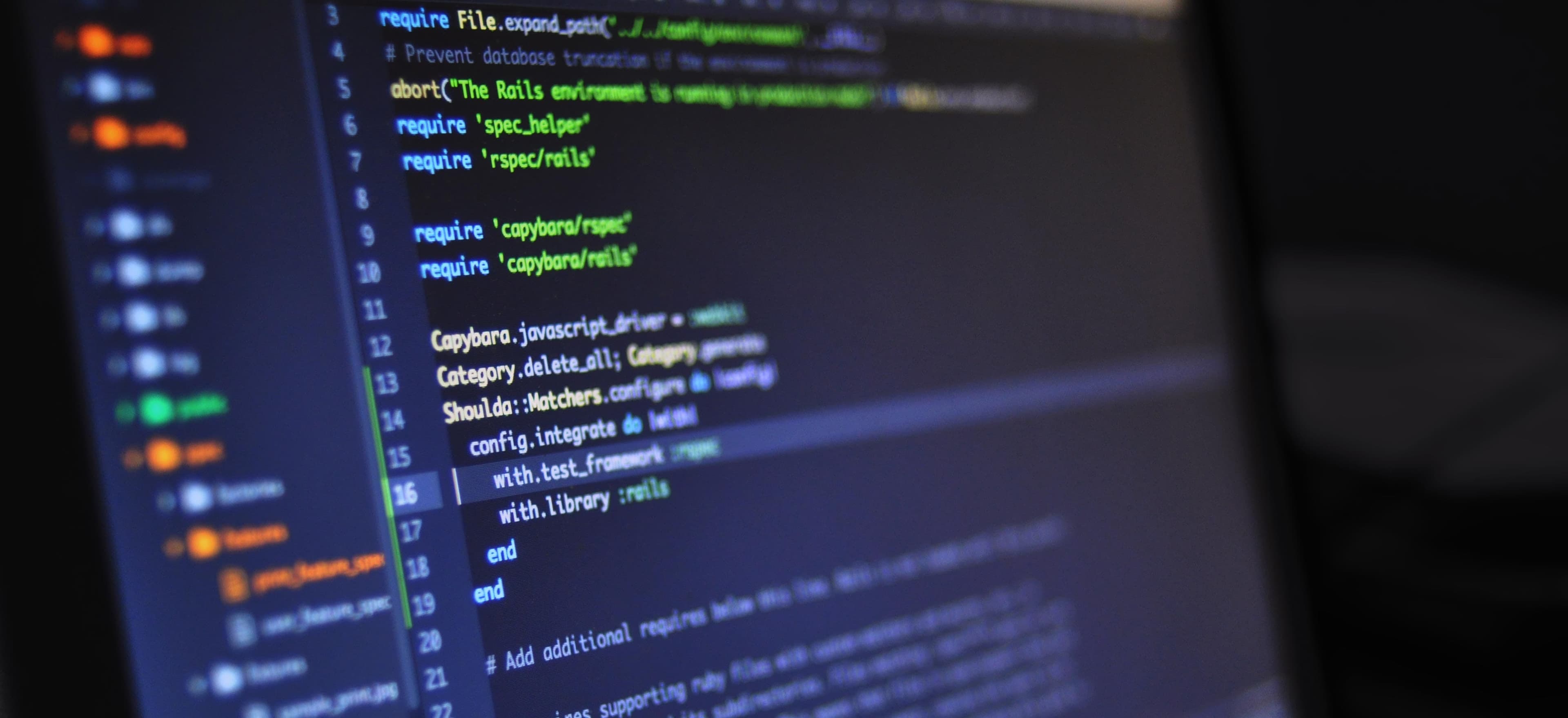
- Published on
Overcoming Common Pitfalls in Strategy Pattern Design
When it comes to software design patterns, the Strategy Pattern stands out as an effective way to manage algorithms and behaviors dynamically. This pattern allows you to define a family of algorithms, encapsulate each one, and make them interchangeable. It promotes the idea of "strategy" on how the algorithm should be configured or executed at runtime, keeping code organized and easy to maintain. However, despite its versatility and strength, it's not immune to pitfalls.
In this blog post, we will explore common mistakes developers make when implementing the Strategy Pattern in Java. We will provide insights and actionable advice to overcome these issues.
What is the Strategy Pattern?
The Strategy Pattern is a behavioral design pattern that enables selecting an algorithm at runtime. Imagine you have different ways to sort a list, such as QuickSort or MergeSort. Instead of hardcoding the sorting algorithm within your class, you can encapsulate these algorithms in separate classes and select one at runtime, leading to more flexible and maintainable code.
Key Components of the Strategy Pattern:
- Context: The client that utilizes the strategy.
- Strategy Interface: Declares a common interface for all concrete strategies.
- Concrete Strategies: Implement the Strategy interface.
Here's a simple implementation of the Strategy Pattern:
// Strategy Interface
public interface SortingStrategy {
void sort(int[] array);
}
// Concrete Strategy: Bubble Sort
public class BubbleSort implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implementation of Bubble Sort
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - 1 - i; j++) {
if (array[j] > array[j + 1]) {
swap(array, j, j + 1);
}
}
}
}
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
// Context
public class SortingContext {
private SortingStrategy strategy;
public SortingContext(SortingStrategy strategy) {
this.strategy = strategy;
}
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void sortArray(int[] array) {
this.strategy.sort(array);
}
}
In this example, the SortingContext
class acts as the context that uses various sorting strategies. The different sorting algorithms are encapsulated in different classes implementing the SortingStrategy
interface.
Why Go for the Strategy Pattern?
- Flexibility and Reusability: Strategies can be swapped easily.
- Single Responsibility Principle: Each class has one responsibility.
- Open/Closed Principle: New strategies can be added without altering existing code.
Common Pitfalls and How to Overcome Them
1. Overusing the Strategy Pattern
One of the most frequent mistakes developers make is overusing the Strategy Pattern. When your application has only one algorithm or behavior, it may not be necessary to implement this design pattern.
Solution: Assess the complexity of your algorithms and avoid introducing unnecessary abstractions. The Strategy Pattern is best utilized when multiple interchangeable behaviors are present.
2. Ignoring the Context Class
Sometimes developers neglect the importance of the context class, treating it merely as a holder for strategies rather than a functional component of the design.
Solution: The context should manage and coordinate different strategies. Ensure it has all the necessary logic to utilize the strategies effectively, which can include switching strategies based on specific conditions.
3. Strategy Creation Logic in the Context
Let’s say your context class is responsible for creating instances of strategies based on certain parameters or input. This can lead to a violation of the Single Responsibility Principle.
Solution: Consider using a Factory Pattern or Dependency Injection to handle strategy creation. This way, the context class remains clean and focused on strategy execution.
Here's a revised implementation using a Factory Pattern:
// Strategy Factory
public class SortingStrategyFactory {
public static SortingStrategy getStrategy(String strategyType) {
switch (strategyType.toLowerCase()) {
case "bubblesort": return new BubbleSort();
case "quicksort": return new QuickSort();
// Add more strategies
default: throw new IllegalArgumentException("Unknown strategy type");
}
}
}
// Usage in Context
public class SortingContext {
private SortingStrategy strategy;
public SortingContext(String strategyType) {
this.strategy = SortingStrategyFactory.getStrategy(strategyType);
}
public void sortArray(int[] array) {
this.strategy.sort(array);
}
}
4. Not Defining a Clear Strategy Interface
Another pitfall is failing to create a clear and comprehensive Strategy interface, which can lead to confusion and disrupt the interchangeability of the strategies.
Solution: Clearly define the methods you want your strategies to implement. Use descriptive names and omit unnecessary parameters to keep the interface clean.
5. Excessive Number of Concrete Strategies
Adding too many strategies can clutter the codebase and make it difficult to manage. Each time a new variation of an algorithm is needed, a new class may be written.
Solution: Organize strategies logically. If certain strategies are variations of one another, you may achieve reusability through inheritance or composition. Consider how they differ and whether they can share code effectively.
6. Lack of Documentation
When employing design patterns, adequate documentation becomes crucial. A lack of comments can result in misunderstandings for other developers trying to comprehend the strategy's purpose and implementation.
Solution: Comment your code adequately. Explain why a strategy is chosen and how it works compared to other strategies, enhancing maintainability and collaboration.
7. Performance Concerns
Performance can degrade if a Strategy utilizes inefficient algorithms or if context is continually swapping strategies without valid reasons, leading to overhead.
Solution: Profile your application to understand performance impacts and use the most efficient strategy for your use case. In cases where performance is critical, consider caching results when appropriate.
Final Thoughts
Implementing the Strategy Pattern can greatly enhance your Java application, providing an intuitive way to manage interchangeable behaviors. However, common pitfalls may hinder its effectiveness.
By being conscious of overuse, maintaining clear interfaces, using context appropriately, avoiding unnecessary complexity, and documenting your choices, you can fully leverage the benefits of the Strategy Pattern.
For further reading on design patterns in Java, you can check out Refactoring Guru or the classic "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al.
Remember, good design isn’t just about patterns; it's about understanding when and how to use those patterns effectively. Keep these pitfalls in mind, and your implementation of the Strategy Pattern will serve your project well. Happy coding!
Checkout our other articles